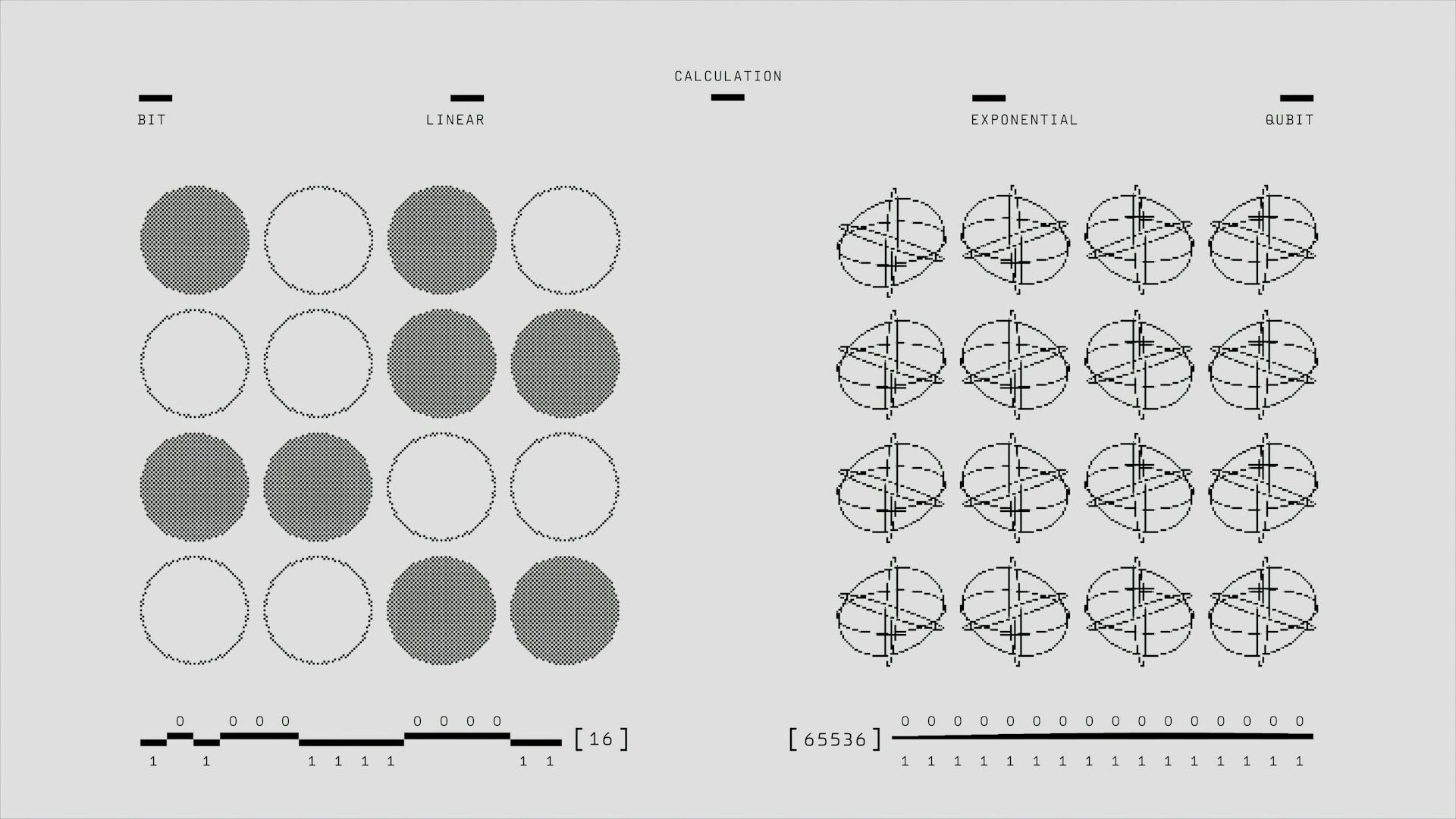
Css Grid Row Height Layouts and Sizing Options can be achieved through various techniques.
You can use the grid-template-rows property to set the height of rows in a grid container. This property accepts a track sizing function that can be a length, a percentage, or a repeat function.
The auto keyword can also be used to set the height of rows, where the row height will be automatically calculated based on the content.
A row can be set to a fixed height using a length value, such as 100px. You can also set the row height to a percentage value, like 50%.
A different take: Css Grid Row
Grid Basics
As of March 2017, most browsers shipped native support for CSS Grid, making it a game-changer for web development.
To get started with CSS Grid, you need to define a container element as a grid with display: grid, which will implicitly convert all direct child elements into grid items.
How to Make a Basic
To make a basic grid, you'll first need to define a grid container, which can be any individual parent element, such as a div.
Pass the display property with either a value of grid or inline-grid to an element's style block to define a grid container.
The value grid makes the parent grid element act like an un-styled div, spanning the full width of a page by default.
To set an element to a grid container, simply pass the display property with either grid or inline-grid to its style block.
Setting an element to a grid container alone won't immediately affect how the child elements are displayed, as you still need to specify how the layout should look.
To change the layout of the grid items within a grid, you must explicitly define the rows and columns you want your grid to have.
Curious to learn more? Check out: Css Grid Container
Background and Motivation
Websites used to be simple documents, but as they evolved into complex, interactive applications, layout techniques like floats didn't quite cut it. Authors had to use workarounds like tables, JavaScript, or measuring floated elements to achieve desired layouts.
Layouts that adapted to available space often resulted in counter-intuitive behavior as space became constrained. This led many authors to opt for fixed layouts that couldn't take advantage of changes in the available rendering space on a screen.
Grid layout provides a mechanism for authors to divide available space into columns and rows using predictable sizing behaviors.
For more insights, see: Css Grid Extra Space
Grid Properties
Grid properties are used to define the layout and behavior of CSS Grid. The `grid-template-columns` property is used to define the columns of a grid, and it can take multiple values separated by space or comma.
The `grid-template-rows` property is used to define the rows of a grid, and it works similarly to `grid-template-columns`. You can use the `fr` unit to define flexible grid items that take up equal space.
Grid properties can be used to create complex layouts, but they can also be used to create simple and clean layouts by using the `grid` shorthand property.
A fresh viewpoint: Css Grid Space between
Container
A grid container is the element on which display: grid is applied, and it's the direct parent of all the grid items.
The size of a grid container is determined by the rules of the formatting context it participates in. As a block-level box in a block formatting context, it's sized like a block box that establishes a formatting context.
In both inline and block formatting contexts, the grid container's auto-block size is its max-content size. The max-content size of a grid container is the sum of the grid container's track sizes (including gutters) in the appropriate axis.
Here are the sizing rules for grid containers in different formatting contexts:
The explicit grid of a grid container is defined by the properties grid-template-rows, grid-template-columns, and grid-template-areas. These properties specify the explicit grid tracks.
The size of the explicit grid is determined by the larger of the number of rows/columns defined by grid-template-areas and the number of rows/columns sized by grid-template-rows/grid-template-columns.
A different take: Css Grid Template
Display
The display property is a fundamental aspect of CSS Grid, and it's used to define an element as a grid container. It establishes a new grid formatting context for its contents.
You can use the display property to create either a block-level grid or an inline-level grid. The values for this property are straightforward: grid generates a block-level grid, while inline-grid generates an inline-level grid.
For example, if you want to create a grid that spans the full width of its parent element, you would use the grid value. On the other hand, if you want to create a grid that can be placed alongside other elements, you would use the inline-grid value.
Here are the values for the display property in a concise list:
- grid – generates a block-level grid
- inline-grid – generates an inline-level grid
Note that the ability to pass grid parameters down through nested elements has been moved to level 2 of the CSS Grid specification, but that's a topic for another time.
Align-Items
The align-items property is a crucial part of grid properties, and it's used to align grid items along the block (column) axis.
This property applies to all grid items inside the container, and it's the default behavior for grid items.
The align-items property has several values, including stretch, start, end, center, and baseline. The stretch value fills the whole height of the cell, while the start and end values align items to the start and end edges of their cell, respectively.
The center value aligns items in the center of their cell, and the baseline value aligns items along the text baseline.
There are also modifier keywords for align-items: safe and unsafe. The safe keyword tries to align items like the specified value, but not if it means moving them into inaccessible overflow areas. The unsafe keyword, on the other hand, allows items to be moved into inaccessible areas.
Here are the possible values for the align-items property:
- stretch – fills the whole height of the cell
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- baseline – align items along text baseline
- first baseline – use the baseline from the first line in the case of multi-line text
- last baseline – use the baseline from the last line in the case of multi-line text
Column-Start
Column-Start is a crucial property in CSS grid systems. It determines the starting position of a grid column.
To set the starting position of a grid column, you can use the column-start property. This property takes a value that represents the column number where the grid column should start. For example, column-start: 3; starts the grid column at the third column position.
Grid columns can be set to start at different positions using the column-start property. This flexibility is useful for creating complex grid layouts.
Here's an interesting read: Css Grid 2 Columns
Row-Start
The grid-row-start property is a powerful tool for specifying the vertical starting position of a grid item along the horizontal (row) grid lines within the grid container. It sets the line where the grid item begins.
This property can be used to control the placement of grid items, ensuring they align perfectly with the grid lines. The grid-row-start property is a key component in creating visually appealing and well-structured grid layouts.
A fresh viewpoint: Css Grid Row Span
By setting the grid-row-start property, you can dictate where a grid item starts its vertical position, giving you precise control over the layout of your grid. This is particularly useful when working with complex grid systems or responsive designs.
In the code above, the grid-row-start property is used to set the vertical starting position of the second div element to row line 1. This demonstrates how this property can be used to specify the exact starting point of a grid item.
Recommended read: Css Grid Vertical Align
Tracks
Tracks are the building blocks of a grid, and understanding how they work is crucial to creating a well-designed layout. A grid track is the space between two adjacent grid lines.
Each grid track is assigned a sizing function, which controls how wide or tall the column or row may grow. This function can be a length, a percentage, or a fraction of the free space in the grid using the fr unit.
In the grid-template-columns and grid-template-rows properties, the values represent the track size, and the space between them represents the grid line. You can use a space-separated list of values to define the columns and rows of the grid.
Grid lines are automatically assigned positive numbers from these assignments, but you can choose to explicitly name the lines using the bracket syntax. This can be useful when you need to reference specific lines in your CSS.
The fr unit allows you to set the size of a track as a fraction of the free space of the grid container. For example, this will set each item to one third the width of the grid container: 1fr 1fr 1fr.
Here's a breakdown of how the fr unit works:
The free space is calculated after any non-flexible items, so if you have a grid container with a non-flexible width of 100px, the free space will be the remaining width of the container.
For another approach, see: Css Grid Column Width
Auto-Columns Property
The auto-columns property is a crucial part of grid layout, and it's essential to understand how it works. The grid-auto-columns property specifies the size of tracks not assigned a size by grid-template-rows or grid-template-columns.
To be more specific, if multiple track sizes are given, the pattern is repeated as necessary to find the size of the affected tracks. This means that the first track after the last explicitly-sized track receives the first specified size, and so on forwards.
Here's a simple way to think about it: if you have three track sizes specified, the first track will get the first size, the second track will get the second size, and the third track will get the third size. This pattern continues until all tracks are assigned a size.
Note that if a grid item is positioned into a row or column that is not explicitly declared, implicit grid tracks are created to hold it. This can happen either by explicitly positioning into a row or column that is out of range, or by the auto-placement algorithm creating additional rows or columns.
A fresh viewpoint: Css Not Class
Centralizing Properties
The grid property is a shorthand way to set multiple grid container properties at once. It's a game-changer for simplifying your CSS code.
You can use the grid property to set values for grid-template-rows, grid-template-columns, and grid-template-areas in a single declaration. This can save you a lot of time and make your code more readable.
Consider reading: Grid Template Areas Css
Explicit Track Sizing: Template-Columns
The grid-template-columns property specifies the track list for the grid's columns, which defines the size and layout of the grid's columns. This property is a crucial part of creating a grid layout.
You can specify the track list for the grid's columns using a space-separated list of values, which can include lengths, percentages, or fractions of the free space in the grid using the fr unit.
The fr unit allows you to set the size of a track as a fraction of the free space of the grid container. For example, this will set each item to one third the width of the grid container.
A unique perspective: Css Grid Fr
You can use the repeat() notation to streamline your definition if it contains repeating parts. For instance, repeat(3, 1fr) is equivalent to 1fr 1fr 1fr.
If multiple lines share the same name, they can be referenced by their line name and count. The fr unit is calculated after any non-flexible items, so the total amount of free space available to the fr units doesn’t include the 50px in this example.
Consider reading: Css Grid Repeat
Grid Layout
Grid Layout is a powerful tool in CSS that allows us to create complex layouts with ease. It's based on a grid system with rows and columns that can be explicitly defined.
The grid-template-rows property lets us specify the height of each row in a grid container, and it accepts one or more non-negative CSS length values. These values represent the height of each row in the grid container, from the first to the last.
In the code above, values of 100px and 200px are passed to the grid-template-rows property, giving the first row a height of 100px and the second row a height of 200px. This is a great way to control the height of specific rows in a grid container.
Implicit rows are created when grid items wrap into new lines, and the height of these rows is determined by the size of their content, not the grid-template-rows property.
Curious to learn more? Check out: Text Height in Css
Cell
In a grid layout, the grid cell is the space between two adjacent row and two adjacent column grid lines. It's a single unit of the grid.
The grid cell is defined by its position between row grid lines and column grid lines. For example, the grid cell between row grid lines 1 and 2, and column grid lines 2 and 3, is a specific unit of the grid.
A grid cell can be thought of as a container for content, such as text, images, or other elements.
Template-Columns
Grid-template-columns is a property that defines the columns of a grid with a space-separated list of values. Each value represents the track size, and the space between them represents the grid line.
The values can be a length, a percentage, or a fraction of the free space in the grid using the fr unit. For example, grid-template-columns: 200px 1fr 2fr creates a three-column grid layout with a fixed width of 200px and two flexible columns that take up 1 and 2 parts of the available space, respectively.
Explore further: Css Grid Template Column
You can choose to explicitly name the lines, and grid lines are automatically assigned positive numbers from these assignments. However, if you define repeating parts, you can use the repeat() notation to streamline things.
The fr unit allows you to set the size of a track as a fraction of the free space of the grid container. For example, 1fr equals 300px when the remaining available width is 900px.
Here's a breakdown of the values you can use in grid-template-columns:
- track-size: can be a length, a percentage, or a fraction of the free space in the grid using the fr unit
- line-name: an arbitrary name of your choosing
Template
In a grid layout, the template is what defines the structure of the grid. The grid-template-columns and grid-template-rows properties work together to define the columns and rows of the grid with a space-separated list of values.
You can specify the track size using a length, percentage, or a fraction of the free space in the grid using the fr unit. For example, setting each item to one third the width of the grid container is done using the fr unit.
Intriguing read: Css Grid Rows Auto
Grid lines are automatically assigned positive numbers, but you can choose to explicitly name the lines using the bracket syntax. This allows you to reference specific lines in your grid.
The repeat() notation can be used to streamline definitions with repeating parts. For instance, this is equivalent to the following definition.
Grid-template is a shorthand for setting grid-template-rows, grid-template-columns, and grid-template-areas in a single declaration. It's a convenient way to define the grid template, but it doesn't reset the implicit grid properties.
You can use the grid-template property to set the grid template with a simpler syntax. For example, this is equivalent to the following definition.
The grid-template-rows property sets the height of each row in a grid container, but it doesn't control the number of rows. Instead, it only sets the height of each row, allowing the grid container to implicitly create new rows as needed.
Here are some examples of how to use the grid-template-rows property:
The grid-template-rows property is a powerful tool for creating complex grid layouts. By understanding how it works, you can create grids that are both functional and visually appealing.
Auto-Flow
Auto-flow is a game-changer for grid layout, allowing you to automatically place items on the grid without specifying every single spot. This property is controlled by grid-auto-flow, which has three main values: row, column, and dense.
The default value is row, which tells the auto-placement algorithm to fill in each row in turn, adding new rows as necessary. This is what happens when you only specify spots for two items out of five, and the remaining three items flow across the available rows.
If you set grid-auto-flow to column, the algorithm will instead fill in each column in turn, adding new columns as necessary. This results in a different layout, where the three unplaced items flow down the columns.
The dense value is a bit more nuanced, as it attempts to fill in holes earlier in the grid if smaller items come up later. However, this can cause items to appear out of order, which is bad for accessibility.
Here are the three main values of grid-auto-flow in a nutshell:
- row: fills in each row in turn, adding new rows as necessary
- column: fills in each column in turn, adding new columns as necessary
- dense: attempts to fill in holes earlier in the grid if smaller items come up later
Align Self
Align Self is a property that helps you control how grid items are aligned within their cells. It's a way to fine-tune the layout of your grid, making sure everything looks just right.
The align-self property can be set to start, end, center, or stretch. If you set it to start, the grid item will be aligned to the top of its cell. This is useful when you want to create a header or title area.
If you set align-self to end, the grid item will be aligned to the bottom of its cell. This is great for creating a footer or a call-to-action area.
When you set align-self to center, the grid item will be centered vertically within its cell. This is perfect for creating a balanced and visually appealing layout.
The default value for align-self is stretch, which means the grid item will fill the entire height of its cell. This is useful when you want to create a grid that takes up the full height of its container.
Check this out: Center Grid Css
Here are the possible values for align-self:
- start – aligns the grid item to the top of its cell
- end – aligns the grid item to the bottom of its cell
- center – aligns the grid item in the center of its cell
- stretch – fills the whole height of the cell
Note that the align-self property can also be set on the grid container via the align-items property. This allows you to control the alignment of all grid items at once.
Additional reading: Css Text Align
Creating a Layout
Creating a Layout is the first step in harnessing the power of CSS grid. To do this, you can turn any layout element into a grid layout by setting its Display value to grid.
This element will become your Grid Container, which is the foundation of your grid layout. Every direct child element of your Grid container will be a Grid Item.
Grid Items can be laid out in rows by default, covering the full width of the grid container.
Layout Concepts
Grid layout is a powerful tool for arranging content on a web page. It's based on a grid system with horizontal and vertical lines that divide the space into grid areas.
A grid container is the top-level element that contains the grid layout, and it's where the grid is defined. The grid is made up of an intersecting set of horizontal and vertical grid lines that divide the container's space into grid areas.
Grid lines are the horizontal and vertical dividing lines of the grid, and they can be referred to by numerical index or by an author-specified name. This means you can use numbers to identify specific lines, or give them names for easier reference.
Grid items, on the other hand, are the elements that are placed within the grid. They can be positioned and aligned using various properties, such as grid-column-start and grid-row-start.
To specify the height of each row in a grid container, you can use the grid-template-rows property. This property lets you set the height of each row, but it doesn't control the number of rows that are created. Instead, it's up to the grid container to create new rows as needed to accommodate the content.
Here's a quick summary of the different grid properties:
By using these properties, you can control the size and location of grid items within the grid, and create a wide range of layouts and designs.
Grid Sizing
Grid sizing is a crucial aspect of CSS Grid, determining the size of all grid tracks and, by extension, the entire grid. Each track has specified minimum and maximum sizing functions, which can be fixed, intrinsic, or flexible.
The grid sizing algorithm defines how to resolve these sizing constraints into used track sizes. This involves considering the sizes of the grid items, available space, and specified sizing functions.
Grid containers are sized using the rules of the formatting context in which they participate. In a block formatting context, they're sized like a block box, with an auto inline size calculated as for non-replaced block boxes. In an inline formatting context, they're sized as an atomic inline-level box.
The max-content size of a grid container is the sum of the grid container's track sizes (including gutters) in the appropriate axis, when the grid is sized under a max-content constraint. This is true for both inline and block formatting contexts.
You might like: Css Grid Max Columns
Here are the ways to specify track sizes:
- A fixed sizing function (e.g., 100px or 50%)
- An intrinsic sizing function (e.g., min-content, max-content, or auto)
- A flexible sizing function (e.g., 1fr)
Note that the size of the grid is not purely the sum of the track sizes, as row-gap, column-gap, and justify-content, align-content can add additional space between tracks.
For your interest: Css Grid Fit Content
Sizing Algorithm
The sizing algorithm is a crucial part of CSS Grid, determining the size of all grid tracks and ultimately the entire grid. It's a complex process, but we can break it down into simpler components.
Each track has specified minimum and maximum sizing functions, which can be fixed, intrinsic, or flexible. Fixed sizing functions use lengths or resolvable percentages, intrinsic functions use min-content, max-content, auto, or fit-content, and flexible functions use flex values.
The grid sizing algorithm defines how to resolve these sizing constraints into used track sizes. This involves calculating the size of each track based on its minimum and maximum sizing functions, as well as any flexible sizing functions.
Here's a step-by-step breakdown of the sizing algorithm:
- Calculate the minimum and maximum sizes of each track based on its sizing functions.
- Calculate the total size of all tracks, including any flexible sizing functions.
- If the total size is less than the grid container's width, the remaining space is distributed among the flexible tracks.
- If the total size is greater than the grid container's width, the excess space is removed from the flexible tracks.
- The final track sizes are calculated based on the minimum and maximum sizes, as well as any flexible sizing functions.
This algorithm ensures that the grid tracks are sized correctly, taking into account any flexible or intrinsic sizing functions. By understanding how the sizing algorithm works, you can create more effective and responsive grid layouts.
Fit-Content()
Fit-content() is a function that operates similarly to minmax(), but with a different twist. It sets the size of a grid track to its intrinsic minimum width, which is the size of the smallest piece of content or text in its grid items.
The intrinsic minimum width is the size of the smallest piece of content or text in the grid items, but it can't be larger than the value specified in the function. This means that if the value of the intrinsic minimum width exceeds the value supplied to the function, the size of the grid track will be set to the value passed to the fit-content() function.
The size of the grid track will be equal to the size of the smallest piece of content or text in its grid items, but if that becomes greater than the value supplied to the fit-content() function, then the size of the columns will be set to the value passed to the fit-content() function.
For example, if you create three columns with widths of 200px, 300px, and 400px using the fit-content() function, the size of each column will be equal to the size of the smallest piece of content or text in its grid items.
You might enjoy: Css Grid Justify Content
Grid Concepts
Grid Concepts are the foundation of CSS Grid, and understanding them is crucial for mastering row height. A Grid Container is the HTML element where the grid layout is applied.
Grid Items are the direct child elements of the Grid Container, and they occupy grid cells by default. Grid Cells are the smallest units of a grid, and they're created by the intersection of column and row grid lines.
Grid Lines are the horizontal and vertical dividing lines that make up the structure of the grid. A grid with four columns will have five column lines, with one after the last column. Grid Tracks are the areas between two adjacent grid lines, and they determine the width and height of our grid items.
Grid Areas are rectangular spaces made up of one or more grid cells, created when a grid item spans over multiple grid tracks.
Discover more: Tailwind Css Min Height
Grid Placement
Grid placement is determined by the grid-row-start, grid-column-start, grid-row-end, and grid-column-end properties. These properties contribute a line, a span, or nothing (automatic) to the grid item's grid placement.
A unique perspective: Css Grid Properties
The grid container has an explicit grid with two grid lines, numbered 1 and 2. You can specify the grid item's column-end edge using the grid-column-end property, which can generate two lines in the endward side of the implicit grid if the value is a line number greater than the number of explicit grid lines.
The grid-row-start and grid-column-start properties can be used to place the grid item between the lines indicated by index. If a name is given as a custom-ident, only lines with that name are counted. If not enough lines with that name exist, all implicit grid lines are assumed to have that name for the purpose of finding this position.
Here's a summary of the grid placement properties:
Note that if a name is given as a custom-ident, only lines with that name are counted. If not enough lines with that name exist, all implicit grid lines on the side of the explicit grid corresponding to the search direction are assumed to have that name for the purpose of counting this span.
Related reading: Css Grid Lines
Row-End
The grid-row-end property lets us specify the vertical ending position of a grid item along the horizontal (row) grid lines within the grid container. This makes it easy to control the height of a grid item down the grid.
If you want a grid item to span down a certain number of grid lines, you can use the grid-row-end property with a span value. For example, if you want a grid item to start on the first-row line and span down three grid lines, ending on line 4, you can use the grid-row-end property with the value 4.
Grid items can overlap each other, and you can use z-index to control their stacking order. However, if you don't specify the grid-row-end property, the grid item will span 1 track by default.
Here are some examples of how to use the grid-row-end property:
- grid-row-end: 4
- grid-row-end: span 3
- grid-row-end: auto
Note that if you use a name as a value for the grid-row-end property, only lines with that name are counted. If not enough lines with that name exist, all implicit grid lines are assumed to have that name for the purpose of finding this position.
Placing Items
Grid placement is all about organizing your content into individual grid items and assigning them to specific areas in the grid. These grid items can be explicitly placed using coordinates through grid-placement properties.
Grid items are equivalent to flex items, and they can be placed using grid-row and grid-column declarations. You can also use the grid-row-start, grid-row-end, grid-column-start, and grid-column-end longhands to further specify their placement.
Grid items can be implicitly placed into empty areas using auto-placement, which is a convenient feature that saves you time and effort.
Grid Shorthands
CSS Grid shorthands are a game-changer for writing more concise and readable style sheets, saving time and energy.
Using these shorthand properties, we can set the values of multiple CSS Grid properties simultaneously, making our code more efficient.
Grid shorthands are like a shortcut for setting multiple properties at once, allowing us to write less code and focus on the design.
The grid shorthand property is particularly useful for setting the grid-template-columns and grid-template-rows properties at the same time.
Grid Explicit
The explicit grid is defined by three properties: grid-template-rows, grid-template-columns, and grid-template-areas. These properties work together to specify the explicit grid tracks.
The size of the explicit grid is determined by the larger of the number of rows/columns defined by grid-template-areas and the number of rows/columns sized by grid-template-rows/grid-template-columns. If grid-template-areas defines a row/column but grid-template-rows/grid-template-columns doesn't size it, its size will be determined by grid-auto-rows/grid-auto-columns.
The grid-template-columns property specifies the track list for the grid's columns, while grid-template-rows specifies the track list for the grid's rows. The grid-template property is a shorthand that can be used to set all three explicit grid properties at the same time, and it resets properties controlling the implicit grid.
Discover more: List Styling in Css
Column-End
The grid-column-end property determines the end edge of a grid item's grid area.
You can specify a line number to indicate where the item ends. For example, grid-column-end: 4 means the item ends at line 4.
If you omit the grid-column-end property, the item will span 1 track by default.
The grid container has an explicit grid with two grid lines, numbered 1 and 2. If you specify grid-column-end: 4, two lines are generated in the endward side of the implicit grid.
Negative integers or zero are invalid for grid-column-end.
Here are some examples of valid grid-column-end values:
- grid-column-end: 4
- grid-column-end: span 3
- grid-column-end: span foo
Note that if you specify grid-column-end: span foo and there's no "foo" line in the grid, the only possibility is a line in the implicit grid.
You might like: Tailwind Css Line Height
The Explicit
The Explicit Grid is defined by three properties: grid-template-rows, grid-template-columns, and grid-template-areas. These properties work together to specify the explicit grid tracks of a grid container.
The size of the explicit grid is determined by the larger of the number of rows/columns defined by grid-template-areas and the number of rows/columns sized by grid-template-rows/grid-template-columns. Any rows/columns defined by grid-template-areas but not sized by grid-template-rows/grid-template-columns take their size from the grid-auto-rows/grid-auto-columns properties.
A unique perspective: Css Grid Auto Columns
A grid with an explicit grid will always contain at least one grid line in each axis, even if no explicit tracks are defined.
The grid-placement properties use numeric indexes that count from the edges of the explicit grid. Positive indexes count from the start side (starting from 1 for the start-most explicit line), while negative indexes count from the end side (starting from -1 for the end-most explicit line).
Here's a summary of the grid-placement property indexes:
The grid and grid-template properties are shorthands that can be used to set all three explicit grid properties at the same time. The grid shorthand also resets properties controlling the implicit grid, whereas the grid-template property leaves them unchanged.
Grid Implicit
The implicit grid is a feature of CSS Grid that automatically generates additional tracks for grid items that fall outside of your explicitly defined grid. This happens when you use grid items with an explicit position.
You might enjoy: Css Grid Align Items
You can define the column and row sizes of this implicit grid using the Grid auto columns (grid-auto-columns) and Grid auto rows (grid-auto-rows) settings of your grid container. This is useful when you want to control the size of the implicit grid tracks.
The grid-auto-flow property controls auto-placement of grid items without an explicit position. Once the explicit grid is filled, auto-placement will also cause the generation of implicit grid tracks.
A different take: Css Grid Auto Fit
Frequently Asked Questions
How to change grid row height in CSS?
To change grid row height in CSS, use the grid-row-end or grid-row-start property in combination with grid-template-rows in the parent container. This allows you to specify the height of each row in your grid layout.
How do you define row height in CSS?
To define row height in CSS, use the grid-template-rows property with a space-separated list of values specifying the height of each row. This property allows you to customize the layout of your grid by setting the height of each row.
What is the grid row height property?
The grid row height property is a setting that determines the height of each row in a grid. It's set using the rowHeight property, which accepts a positive number value.
How do you make all grid rows the same height in CSS?
To make all grid rows the same height in CSS, set the grid-auto-rows property to 1fr. This will distribute the available space evenly among all rows, ensuring they have equal height.
Featured Images: pexels.com