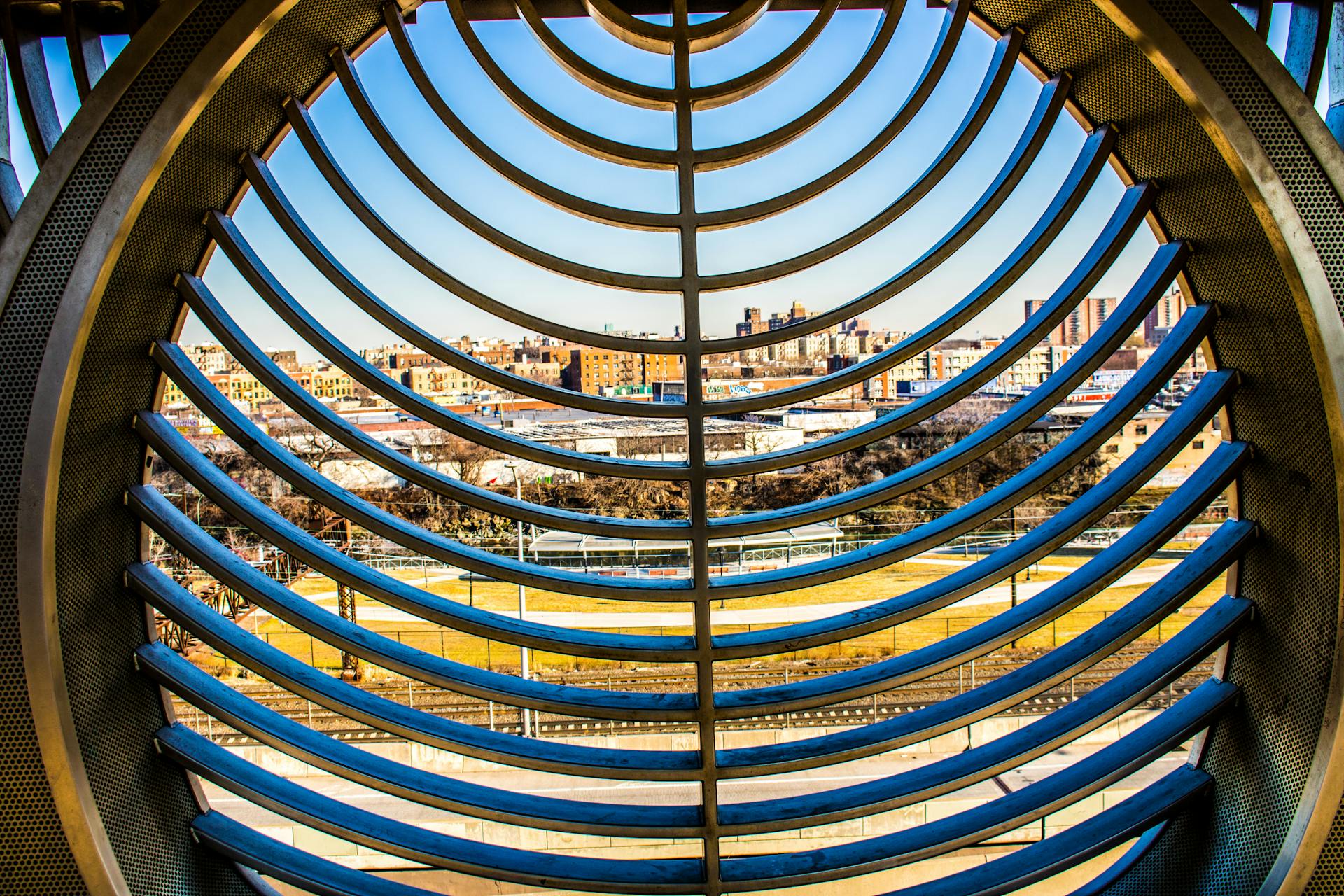
Mastering CSS Grid Repeat is a game-changer for web development.
This property allows you to repeat a grid template area or tracks, making it easier to create responsive and dynamic layouts.
The CSS Grid Repeat property can be used to repeat a grid template area, as shown in the example where we repeat the "header" grid template area three times.
This is particularly useful for creating a grid that adapts to different screen sizes.
To repeat a grid template area, you use the repeat() function, which takes the number of times you want to repeat it as an argument.
For example, repeat(3) would repeat the grid template area three times.
Using CSS Grid Repeat, you can create complex and responsive layouts with minimal code.
Additional reading: Responsive Width Css
Responsive Grid Layout
Responsive grid layout is a powerful tool in CSS grid repeat. It allows you to create flexible and dynamic grids that adapt to different screen sizes and devices.
You can use the repeat() function to create a repeating number of columns in a grid row. This is useful when you want to pack in as many columns as possible before the grid wraps to a new line.
For example, you can use repeat() in combination with auto-fit to fill all of the available space of the grid. This is achieved by using the auto-fit keyword, which allows the grid to automatically fit the available space.
Using repeat() and auto-fit together creates a flexible grid that can adapt to different screen sizes. This is especially useful for responsive design, where you want your layout to work well on both desktop and mobile devices.
The combination of repeat() and auto-fit also allows you to set a minimum width for each column. This is achieved by using the minmax() function, which sets a minimum and maximum value for each column. For instance, you can set each column to be at least 250px wide, while also allowing it to take up an equal fraction of space.
This type of layout is perfect for creating a dynamic and responsive grid that adapts to different screen sizes and devices.
Curious to learn more? Check out: Css Grid Auto-fit
Grid Properties
Grid Properties are a key part of CSS Grid Repeat, allowing you to define the size of your grid rows or columns in a concise and efficient manner.
You can use the repeat function with grid-template-rows to define the size of your grid rows. The syntax is the same as with grid-template-columns, for example, "grid-template-rows: repeat(3, 100px);" will create a grid with three rows each of 100px height.
Grid Properties support various sizing options, including fixed lengths, content-based keywords, and flexible auto keyword, providing versatility in responsive design. For instance, you can use the repeat function with length values to define a consistent size for your grid tracks while repeating them across the grid container.
Here are some common length values you can use with the repeat function:
- Fixed lengths (px, em, %)
- Content-based keywords (min-content, max-content)
- Flexible auto keyword
These options allow you to create complex and responsive grid layouts efficiently, without the need for media queries.
Flex
Flex is a non-negative value expressed in fr units that allows you to create flexible grid tracks by specifying the size of a track as a fraction of the available space in the grid container.
This makes for a more responsive grid, as the grid changes widths or heights, so do the columns or rows.
One fraction of the available space is 200px, which can be calculated by dividing the remaining space by the number of fractions.
For example, if the available space is 600px and there are two fractional columns, one fraction would be 200px.
A grid takes any gaps that have been added between grid tracks into account when figuring out how much available space there is to distribute to the fractional columns or rows.
This means that gaps, like the 30px worth of gaps in a grid with a 15px gap between each column or row, have to be factored into the available space.
The benefit of using flex is that it takes care of the math, so you don't have to do a lot of manual calculations.
Take a look at this: Css Grid Rows Auto
Fit-Content (<Length-Pecentage>)
The fit-content() function is a powerful tool in CSS Grid, allowing you to create flexible grid layouts that adapt to the size of their content. It takes a single argument, which represents the maximum size a track can grow to.
For your interest: Css Grid Fit Content
The fit-content() function uses the space available and accepts a percentage or length value as the maximum size allowed for a grid track. This means that the track can be flexible up to that point, but not beyond.
For example, if you set three columns and fit-content(120px), the column widths will be responsive up until a width of 120px. The fit-content() function is particularly useful for scenarios where you want the grid to be responsive to varying content lengths but within a certain range of sizes.
Here are some key points to keep in mind when using the fit-content() function:
- The fit-content() function takes a single argument, which represents the maximum size a track can grow to.
- The fit-content() function uses the space available and accepts a percentage or length value as the maximum size allowed for a grid track.
- The track can be flexible up to the maximum size, but not beyond.
- The fit-content() function is particularly useful for scenarios where you want the grid to be responsive to varying content lengths but within a certain range of sizes.
In the example code, .grid-container{display:grid;grid-template-columns:repeat(3,fit-content(200px));}, the grid-template-columns property uses the CSS Grid repeat() function to create three columns, and each column's width is determined by the fit-content(200px) function. The fit-content() function calculates the minimum size required to contain the content within an element.
On a similar theme: Css Grid Justify Content
Can I the?
You can use the repeat function with percentage values to create a grid with columns that take up a specific portion of the container's width. For example, "grid-template-columns: repeat(2, 50%);" will create a grid with two columns that each take up half of the container's width.
Recommended read: Grid Container Css
The repeat function can be used with media queries to create responsive grid layouts. You can define different grid layouts for different screen sizes using the repeat function inside a media query.
You can use media queries to display a different number of columns on small screens versus large screens. For instance, you might want to display two columns on small screens and four columns on large screens.
The code for this would be: "@media (max-width: 600px) { .grid { grid-template-columns: repeat(2, 1fr); } } @media (min-width: 601px) { .grid { grid-template-columns: repeat(4, 1fr); } }".
A different take: Css Grid Template Areas
Grid Layout Techniques
Grid layouts can be created with a dynamic number of columns by combining the powers of repeat(), auto-fit, and minmax(). This allows for a flexible and responsive grid layout that can adapt to different screen sizes and content types.
To create a grid layout with a repeating pattern of track sizes, you can use the CSS Grid repeat() function, which takes two main arguments: the number of repetitions and the track size. The first value can be a length value, auto-fill, or the auto-fit keyword, while the second value can be a length value, min-content, max-content, auto, or one of the other options listed in the article.
Here are some common track size options for the CSS Grid repeat() function:
- Length value (px) or percentages
- min-content keyword
- max-content keyword
- auto keyword
- auto-fit and auto-fill keywords
- Named lines
- fit-content() function
- minmax() function
- min() or max() function
Explicit vs Implicit Layout
In CSS Grid, layouts are classified into two types: explicit and implicit. These terms refer to how the grid tracks (rows and columns) are defined and created within a grid container.
Explicit grid layouts are created using CSS properties like grid-template-columns and grid-template-rows, where you explicitly specify the number and size of rows and columns. This is shown in the example code where grid-template-columns creates three columns with relative widths, and grid-template-rows creates four rows with fixed heights.
You determine how exactly the grid should be structured, including its dimensions and layout. In the example code, the grid-template-columns property creates three columns with relative widths, and the grid-template-rows property creates four rows with fixed heights.
The implicit grid layout is automatically created to accommodate additional content beyond what is explicitly defined. This is particularly useful when there are more items to place in the grid than the number of tracks that have been explicitly defined.
Suggestion: Grid Template Css
The implicit grid adapts to the added content, automatically creating additional rows or columns as needed. In the example code, the grid-auto-rows property defines the height of the rows in the implicit grid, and additional rows are created automatically.
Here's a comparison of explicit and implicit grid layouts:
The implicit grid is useful when you have more items to place in the grid than the number of tracks that have been explicitly defined. However, a point to note is that even if you don’t define the size of these implicitly created tracks using grid-auto-columns and grid-auto-rows, they will still be created, but their size will be based on the default value of auto.
Worth a look: Css Grid Auto Columns
Exploring Different Techniques
The CSS Grid repeat() function is a powerful tool for creating flexible and dynamic grid layouts. It allows you to specify the number of repetitions and the track size, making it easier to create consistent layouts.
You can use the repeat() function to create a grid layout with a dynamic number of columns that will fill all available space. For example, you can use repeat() with auto-fit to create a grid layout that adapts to different screen sizes.
The repeat() function can also be used with length values, such as pixels or percentages, to create a grid layout with a fixed number of columns. For instance, you can use repeat(3, 1fr) to create a grid layout with three equal-sized columns.
Here are some common use cases for the repeat() function:
- Creating a grid layout with a dynamic number of columns that will fill all available space.
- Creating a grid layout with a fixed number of columns.
- Creating a grid layout with equal-sized columns.
The repeat() function can be used in combination with other CSS Grid functions, such as auto-fill and auto-fit, to create more complex grid layouts. For example, you can use repeat() with auto-fill to create a grid layout that fills all available space while maintaining a minimum width.
By mastering the repeat() function, you can create more efficient and maintainable grid layouts that adapt to different screen sizes and types of content.
Grid Size and Variations
Grid size is determined by the number of grid tracks defined in the grid template, which can be set using the grid-template-rows and grid-template-columns properties.
A grid container can have a maximum of 10 grid tracks per row or column.
Grid templates can be repeated to create a grid layout with multiple rows or columns.
The grid-template-rows property can be used to define a repeating grid template, where the grid template is repeated for each row.
The grid-template-columns property can be used to define a repeating grid template, where the grid template is repeated for each column.
Additional reading: Css Grid Equal Width Columns
Grid Functions and Properties
Grid functions are a powerful tool for creating complex layouts with CSS Grid. You can use the repeat function with grid-template-rows to define the size of grid rows.
The syntax for repeat with grid-template-rows is the same as with grid-template-columns. For example, "grid-template-rows: repeat(3, 100px);" creates a grid with three rows each of 100px height.
A different take: Css Grid Columns
Frequently Asked Questions
The repeat function in CSS Grid is a shorthand method for defining multiple grid tracks of the same size.
This makes your code cleaner and easier to read. For example, instead of writing "grid-template-columns: 100px 100px 100px 100px;", you can simply write "grid-template-columns: repeat(4, 100px);".
You can use the repeat function to define the number of tracks and their size in one go. This is particularly useful for creating grids with a large number of tracks.
The repeat function is a shorthand method, which means it's more efficient and easier to maintain than writing out each track individually.
Sources
- https://css-tricks.com/almanac/properties/g/grid-template-columns/
- https://www.sitepoint.com/css-grid-repeat-function/
- https://www.lambdatest.com/blog/css-grid-repeat/
- https://css-tricks.com/almanac/properties/g/grid-template-rows/
- https://dev.to/mateuszkirmuc/css-grid-repeat-function-beyond-basics-32ce
Featured Images: pexels.com