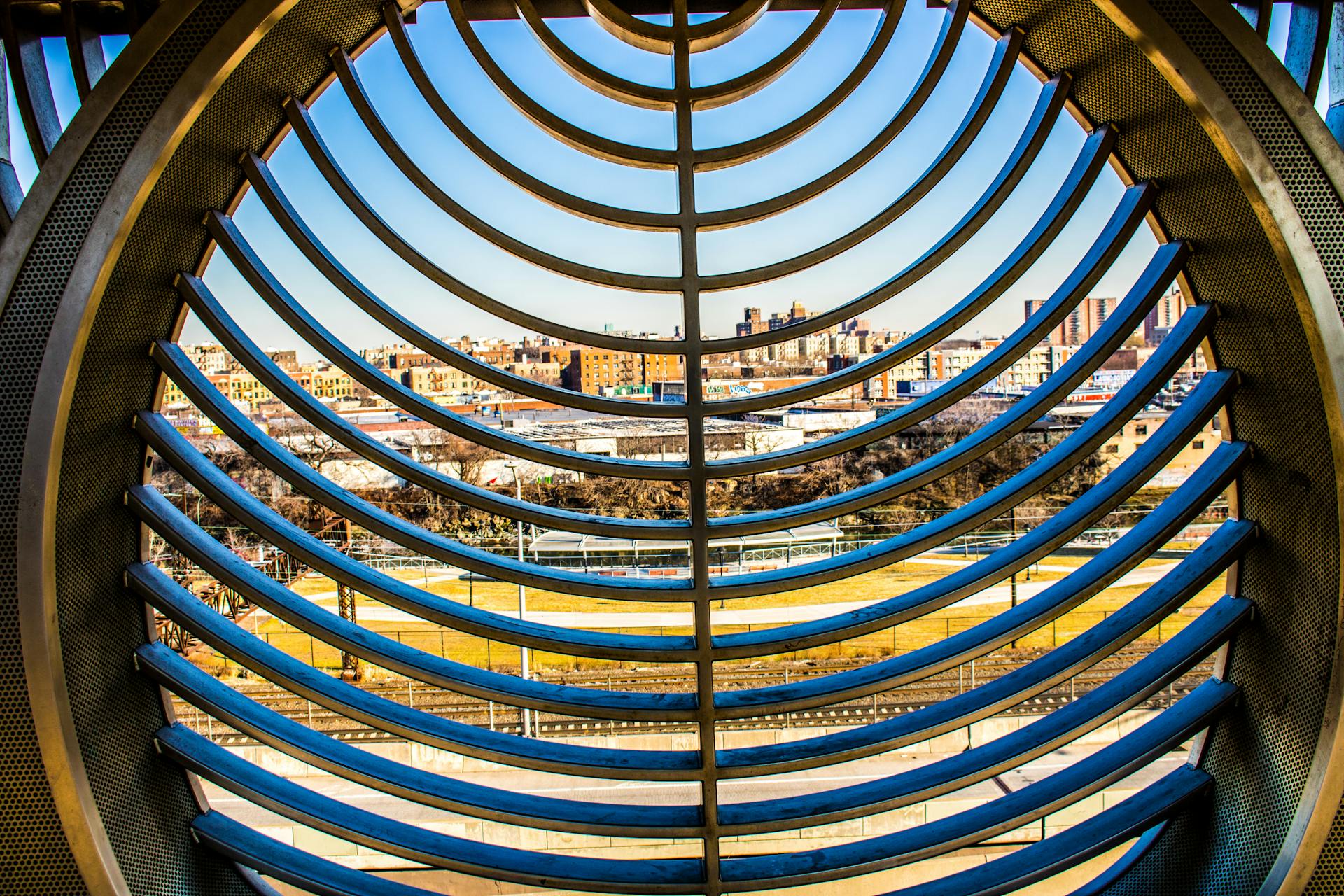
CSS Grid allows you to create complex layouts with ease, but it can be tricky to manage extra space. To avoid clutter and maintain a clean design, use the `grid-template-columns` property to define the number of columns and their widths.
When working with uneven grid tracks, it's essential to use the `grid-template-columns` property with the `fr` unit to allocate fractional units of space. This ensures that the remaining space is distributed evenly among the tracks.
To avoid overlapping content, make sure to set the `grid-auto-flow` property to `row` or `column` and use the `grid-template-rows` or `grid-template-columns` property to define the grid tracks.
By following these best practices, you can create a well-structured and visually appealing layout that effectively utilizes the extra space in your CSS Grid design.
Related reading: Onedrive Extra Storage
Grid Terminology
Grid comes with a bunch of new terminology as it's the first time CSS has had a real layout system. This means you'll need to learn some new vocabulary to effectively use Grid.
Grid terminology can be overwhelming at first, but don't worry, it's worth the investment of time to learn. The terminology will help you to better understand how to use Grid to create flexible and responsive layouts.
Some key terms to get familiar with include the grid container, grid items, and grid tracks.
Terminology
Grid brings a new layout system to CSS, and with it, a whole bunch of new terminology.
The first time CSS has had a real layout system, Grid is a game-changer for web design.
Grid terminology can be overwhelming, especially for those new to CSS layout.
The good news is that once you learn the basics, you'll be able to create complex and beautiful layouts with ease.
Grid comes with a bunch of new terminology, so let's break it down and make it more manageable.
Grid terminology is a departure from the traditional CSS layout methods, which is why it can feel so new and unfamiliar.
The more you work with Grid, the more comfortable you'll become with its unique terminology.
Grid is designed to help you create responsive and flexible layouts, which is a huge advantage over traditional methods.
Rows and Columns
Rows and columns are the building blocks of a grid. They can be defined with specific lengths or auto sizing.
To create a basic grid, you can define a grid with three column tracks and two row tracks. A 10 pixel gap between the tracks is also possible.
Each track uses a different length unit, and tracks are auto sized by default. This means that if an element has child items, they will immediately lay out on this grid.
You can see this in action in the demo below. The grid overlay in Chrome DevTools can help you understand the various parts of the grid.
If you want to create a 12 column track grid with equal columns, you could use the following CSS. This will create a grid with 12 equal-sized columns.
Here's a breakdown of the grid tracks:
- Column tracks: 3 tracks with different length units
- Row tracks: 2 tracks, one with a length unit and the other auto
Each row track can be named for easier reference. For example, you can name the first row track "row1-start" and set it to "auto". This will create a grid with a named row track.
Recommended read: Css Grid Row Height
The Fr Unit
The fr unit is a flexible length that describes a share of the available space in the grid container. It works in a similar way to using flex: auto in flexbox, distributing space after the items have been laid out.
To have three columns that all get the same share of available space, you can use the fr unit. For example, grid-template-columns: 1fr 1fr 1fr will share the available space equally among the three columns.
The fr unit can be combined with a fixed size gap or fixed size tracks. For instance, grid-template-columns: 200px 1fr will have a component with a fixed size element and the second track taking up whatever space is left.
Using different values for the fr unit will share the space in proportion. Larger values will get more of the spare space.
Here's an example of how to use the fr unit to share space among three columns:
The fr unit is a powerful tool in CSS Grid, allowing you to create flexible and responsive layouts.
Negative Line Numbers
Negative line numbers can be used with explicit grids to place items from the end line, allowing them to span across the entire grid with grid-column: 1 / -1. This is particularly useful for creating items that stretch across multiple columns.
You might think that using negative line numbers with grid-row would have the same effect, but it doesn't work with implicit grids. The tracks are created in the implicit grid, making it impossible to reach the end of the grid using -1.
See what others are reading: Css Grid Align Items
Grid Layout
Grid Layout is a powerful tool in CSS that allows for flexible and responsive design.
CSS Grid Layout examples, such as those provided by web designer Rachel Andrew, demonstrate its capabilities.
Fluid columns can be created without media queries, as seen in the fluid width columns snippet, which breaks into more or less columns as space is available.
Area
Grid areas are created by causing an item to span over multiple tracks. This allows for more flexibility in layout design.
Rachel Andrew has provided some examples of grid layouts, which demonstrate how grid areas can be used to create complex and interesting designs.
A grid area is essentially a group of grid cells together. This can be useful for creating larger sections of a layout that need to be styled consistently.
Grid areas can be thought of as a single unit that can be manipulated and styled as a whole. This makes it easier to work with complex layouts and design systems.
Take a look at this: Grid Template Areas Css
Justify-Items
Justify-Items is a crucial property in Grid Layout that determines how grid items are aligned along the inline (row) axis. You can apply this property to all grid items inside a container.
The justify-items property has four values: start, end, center, and stretch. The stretch value is the default, which means it fills the whole width of the cell.
To align items to the start edge of their cell, use the start value. This is useful when you want to create a grid with items flush against the left edge.
For your interest: Css Grid Justify Content
For aligning items to the end edge of their cell, use the end value. This is helpful when you want to create a grid with items flush against the right edge.
The center value aligns items in the center of their cell, creating a balanced look.
Here are the justify-items values in a concise table:
Line-Based Placement Layout
You can use the grid-column and grid-row properties to position items in a grid, and they can be used in a shorthand form to make your code more concise.
To have an item span multiple columns, you can define the grid-column shorthand property by making the first value a "1" and the second value a "3", for example.
Grid items can also span multiple rows, and to achieve this, you can define the grid-row shorthand property by making the first value a "1" and the second value a "3".
The grid below will contain only four grid items, and one item will span more than one column, while another will span more than one row.
You only need to define the start and end values for the grid items that you want to span multiple tracks, which makes the code more efficient.
Worth a look: Css Grid Row Span
Auto-Columns Auto-Rows
=====================
Auto-columns and auto-rows are two powerful properties that allow you to control the size of implicit grid tracks. Implicit tracks get created when there are more grid items than cells in the grid or when a grid item is placed outside of the explicit grid.
You can specify the size of auto-generated grid tracks using the grid-auto-columns and grid-auto-rows properties. These properties can take a length, a percentage, or a fraction of the free space in the grid, using the fr unit.
To create all implicit rows at a minimum size of 10em and a maximum size of auto, you can use the following CSS: grid-auto-rows: minmax(10em, auto);
Implicit columns can also be controlled using the grid-auto-columns property. To create implicit columns with a pattern of 100px and 200px wide tracks, you can use the following CSS: grid-auto-columns: 100px 200px 100px 200px;
The grid-auto-flow property controls how the auto-placement algorithm works. It can take three values: row, column, and dense. The default value is row, which tells the auto-placement algorithm to fill in each row in turn, adding new rows as necessary.
Here's a summary of the grid-auto-flow values:
Column Row
You can specify the size of any auto-generated grid tracks using the grid-auto-columns and grid-auto-rows properties.
These properties are useful when you have more grid items than cells in the grid, or when a grid item is placed outside of the explicit grid. You can define the size of these implicit tracks as a length, a percentage, or a fraction of the free space in the grid.
For example, if you define .item-b to start on column line 5 and end at column line 6, but you never defined a column line 5 or 6, implicit tracks with widths of 0 are created to fill in the gaps.
You can use the grid-auto-columns and grid-auto-rows properties to specify the widths of these implicit tracks.
Here's a list of values you can use for the grid-auto-columns and grid-auto-rows properties:
- track-size – can be a length, a percentage, or a fraction of the free space in the grid (using the fr unit)
Grid lines can also be named instead of using numbers. This can make your CSS code more readable and easier to understand.
For example, you can define the grid-template-columns and grid-template-rows properties with line names and values. Each column will be named "col1-start", "col2-start", and "col3-start", respectively, and set to 100px. Each row will be named "row1-start" and "row2-start", respectively, and set to "auto."
Grid Tracks
Grid tracks are the building blocks of a CSS grid layout, and understanding how to size them is crucial for creating responsive and flexible designs. By default, implicit tracks are auto-sized, but you can control their sizing using the grid-auto-rows and grid-auto-columns properties.
To create all implicit rows at a minimum size of 10em and a maximum size of auto, you can use the grid-auto-rows property. For example, grid-auto-rows: minmax(10em, auto); will achieve this.
Implicit columns can be created with a pattern of 100px and 200px wide tracks by using the grid-auto-columns property, as shown in the example: grid-auto-columns: 100px 200px.
Flexible units like fr and minmax are essential for creating responsive grid layouts. Instead of specifying fixed pixel values, you can use these units to make your layout adapt to different screen sizes. For instance, using minmax() to set a minimum width of 200px and a maximum width of 1fr will ensure your layout remains consistent and visually appealing across various devices.
Here's a summary of the flexible units:
By using these flexible units, you can create grid layouts that are truly responsive and flexible, making your designs more efficient and user-friendly.
Spanning Tracks
You can cause some or all of the items in an auto-placed layout to span more than one track by using the span keyword plus the number of lines to span as a value for grid-column-end or grid-row-end.
The span keyword can be used to specify the number of columns or rows an item spans, and it's especially useful when you only need to define one value.
You can also specify the same thing using the shorthand grid-column property, which is a convenient shortcut for setting both grid-column-start and grid-column-end.
Items that span multiple tracks can be defined using the span keyword, where the end value is specified as "span" followed by the number of columns or rows to span.
You might like: Css Grid Column Width
Setting Up Tracks
Setting up tracks is a crucial step in creating a grid layout. You can create implicit tracks in a grid, which will be auto-sized by default.
To control the sizing of rows, use the grid-auto-rows property, and for columns, use grid-auto-columns. This allows you to specify the minimum and maximum size of implicit tracks.
Suggestion: Css Grid Auto Fit
For example, you can create all implicit rows at a minimum size of 10em and a maximum size of auto. You can also create implicit columns with a pattern of 100px and 200px wide tracks.
To create a responsive layout, use flexible units like fr or minmax instead of fixed pixel values. Flexible units dynamically adjust their dimensions based on the available space, ensuring a consistent and visually appealing layout across various devices.
For instance, you can use minmax() to specify that the cards should have a minimum width of 200px and a maximum width of 1fr. This approach ensures a flexible and responsive card layout.
You can also set up explicit grid tracks by defining the grid column and row tracks using the grid-template-columns and grid-template-rows properties. For example, you can create a three-column grid layout with the following settings:
grid-template-columns: 200px 1fr 2fr
This creates a grid with a fixed width of 200px for the first column, and 1fr and 2fr for the second and third columns, respectively. The fr unit takes up a fraction of the available space, and its width is calculated based on the remaining space after subtracting non-fr values and gaps.
Here's an interesting read: Css Grid Minmax
Grid Template
Grid Template is a powerful tool in CSS that allows you to define the layout of your grid container in a single place. This makes it easy to redefine the layout using media queries.
You can name areas of the grid and place items onto those named areas using the grid-area property. The name can be anything you like, except for the keywords "auto" and "span". Once you've named your items, you can use the grid-template-areas property to define which grid cells each item will span.
Grid-template-areas is a property that defines the layout of your grid container. It's a complete grid with no empty cells, and each row is defined within quotes. To span tracks, you simply repeat the name, and the areas created by repeating the name must be rectangular and cannot be disconnected.
To leave white space on the grid, you can use a dot (.) or multiple dots with no white space between them. For example, to leave the very first cell on the grid empty, you could add a series of dots.
Take a look at this: Css Grid Span All Columns
Grid-template is a shorthand for grid-template-rows, grid-template-columns, and grid-template-areas. It's a convenient way to define the layout of your grid container in a single property.
Here are the different parts of the grid-template property:
- grid-template-rows defines the rows of the grid
- grid-template-columns defines the columns of the grid
- grid-template-areas defines the layout of the grid
You can use the repeat() notation to streamline your grid-template-columns and grid-template-rows definitions. For example, if you have a grid with two repeating parts, you can define it like this: repeat(2, 1fr 2fr).
The fr unit allows you to set the size of a track as a fraction of the free space of the grid container. For example, this will set each item to one third the width of the grid container: 1fr 1fr 1fr.
For your interest: Css Grid Container
Grid Alignment
Grid alignment is a crucial aspect of CSS grid, and it's essential to understand the properties that control it.
The alignment properties in grid layout are similar to those in flexbox. They include justify-content, align-content, justify-self, align-self, justify-items, and align-items.
These properties can be used to distribute additional space in the grid container or to move grid items around inside the grid area. The key is to understand which properties are used on the inline axis and which are used on the block axis.
Broaden your view: Css Grid Fit-content
The inline axis is the direction in which sentences run in your writing mode, while the block axis is the direction in which blocks are laid out. This is important to keep in mind when choosing which properties to use.
To align a grid item inside a cell, you can use the align-self property. This property aligns the grid item along the block axis, and it has several values, including start, end, center, and stretch.
Here are the values for the align-self property:
- start – aligns the grid item to be flush with the start edge of the cell
- end – aligns the grid item to be flush with the end edge of the cell
- center – aligns the grid item in the center of the cell
- stretch – fills the whole height of the cell (this is the default)
To align all the items in a grid, you can set this behavior on the grid container via the align-items property. This property aligns grid items along the block axis, and it has several values, including stretch, start, end, center, and baseline.
Here are the values for the align-items property:
- stretch – fills the whole height of the cell (this is the default)
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- baseline – align items along text baseline
The align-content property is used to align the grid along the block axis, and it has several values, including start, end, center, stretch, space-around, space-between, and space-evenly.
A fresh viewpoint: Css Grid Vertical Align
Here are the values for the align-content property:
- start – aligns the grid to be flush with the start edge of the grid container
- end – aligns the grid to be flush with the end edge of the grid container
- center – aligns the grid in the center of the grid container
- stretch – resizes the grid items to allow the grid to fill the full height of the grid container
- space-around – places an even amount of space between each grid item, with half-sized spaces on the far ends
- space-between – places an even amount of space between each grid item, with no space at the far ends
- space-evenly – places an even amount of space between each grid item, including the far ends
By understanding these properties and values, you can effectively align your grid and make the most of the extra space in your layout.
Grid Placement
Grid Placement is a powerful feature in CSS that allows you to precisely control the position of grid items within a grid container. You can use the grid-column and grid-row shorthand properties to define the start and end values for grid items that span multiple tracks.
For example, to have a grid item span two columns, you can define the grid-column shorthand property by making the first value a "1" and the second value a "3." This will place the grid item from column 1 to column 3.
To have a grid item span two rows, you can define the grid-row shorthand property by making the first value a "1" and the second value a "3." This will place the grid item from row 1 to row 3.
You can also use the place-content shorthand property to set both the align-content and justify-content properties in a single declaration. The first value sets align-content, and the second value sets justify-content. If the second value is omitted, the first value is assigned to both properties.
You might enjoy: Css Grid 3 Columns
Grid Gaps
Grid gaps are a crucial aspect of CSS Grid, and understanding how they work can help you create more efficient and visually appealing layouts.
Gaps can be thought of as the space between grid tracks, which can be sized like regular tracks. However, you can't place content into a gap, but you can span grid items across it.
Grid gaps can be filled using the `grid-auto-flow` property, which can be set to `dense` to enable dense packing mode. This mode takes items later in the layout and uses them to fill gaps, potentially changing the display order.
The `gap` shorthand property is a convenient way to set both `row-gap` and `column-gap` at the same time. It's worth noting that the unprefixed `gap` property is already supported in Chrome 68+, Safari 11.2 Release 50+, and Opera 54+.
The size of grid lines, which creates the gutters between columns and rows, can be set using the `column-gap` and `row-gap` properties. These properties can be set to a length value, and the gutters are only created between the columns and rows, not on the outer edges.
Consider reading: Css Grid Properties
Grid Sizing
The grid-auto-rows and grid-auto-columns properties allow you to control the sizing of implicit tracks in a grid.
You can use the grid-auto-rows property to create all implicit rows at a minimum size of 10em and a maximum size of auto.
To create implicit columns with a pattern of 100px and 200px wide tracks, use grid-auto-columns.
Flexible units like fr and minmax are essential for responsive grid layouts. They enable your layouts to adapt to different screen sizes.
The fit-content() function uses the space available, but never less than min-content and never more than max-content.
The minmax() function sets a minimum and maximum value for what the length is able to be, which is useful for in combination with relative units.
Here's a summary of the different functions:
By using flexible units and the minmax() function, you can create responsive grid layouts that adapt to different screen sizes.
Auto-Fill & Auto-Fit Keywords
Auto-Fill & Auto-Fit Keywords are a game-changer for responsive grid layouts. They allow us to create flexible grids without the need for media queries.
Expand your knowledge: Css Grid Automatic Columns
You can use the auto-fill or auto-fit keywords in your grid-template-columns property to achieve this. The difference between them lies in their behavior when there's not enough space for all the tracks. Auto-fill creates empty tracks, while auto-fit collapses them down to 0 size.
The syntax for using auto-fill or auto-fit is as follows: repeat(auto-fill, minmax(200px, 1fr)). This will create as many 200 pixel tracks as will fit in the container. If you add the minmax() function, you can request a minimum size of 200 pixels and a maximum of 1fr.
Here's a comparison of auto-fill and auto-fit:
In practice, this means that auto-fill will leave a gap on the end until there's enough space for another 200 pixel track, while auto-fit will make the flexible tracks grow to consume the space.
Worth a look: Css Grid Auto Rows
The Minmax() Function
The minmax() function is a powerful tool in CSS Grid that allows you to set a minimum and maximum value for a track. It's useful for in combination with relative units, like you may want a column to be only able to shrink so far.
You can use the minmax() function to set a minimum and maximum value for a track, like minmax(200px, 1fr). This means the track will have a minimum width of 200px and a maximum width of 1fr, which is a share of the remaining space.
The minmax() function is especially useful when you want to create a flexible grid layout that adapts to different screen sizes. By using minmax(), you can ensure that your grid layout remains consistent and visually appealing across various devices.
Here are some examples of how you can use the minmax() function:
- To create a grid layout with a minimum width of 200px and a maximum width of 1fr, you can use the following code: grid-template-columns: repeat(auto-fill, minmax(200px, 1fr)).
- To create a grid layout with a minimum width of 100px and a maximum width of 2fr, you can use the following code: grid-template-columns: repeat(auto-fill, minmax(100px, 2fr)).
By using the minmax() function, you can create flexible and responsive grid layouts that adapt to different screen sizes and devices.
Shorthand Properties
Shorthand properties can save you time and make your code look cleaner. They allow you to set many of the grid properties in one go, which can be a huge timesaver.
To use the shorthand grid-column property, you need to combine the grid-column-start and grid-column-end properties into one line. This is done by separating the values with a slash, where the first number represents the column line where the grid item starts and the second number represents the column line where the grid item ends.
Intriguing read: 2 Column Grid Css
For example, if you want to set a grid item to start at column line 2 and end at column line 5, you would use the shorthand property `grid-column: 2/5;`.
The same applies to the grid-row shorthand property, where you can combine grid-row-start and grid-row-end into one line.
Here's a summary of how to use the shorthand properties:
- grid-column: [start column]/[end column]
- grid-row: [start row]/[end row]
Frequently Asked Questions
How do you add space in CSS grid?
To add space in CSS grid, use the grid-gap property to specify the size of the gap between rows and columns. Adjust the value as needed to add more or less space.
Featured Images: pexels.com