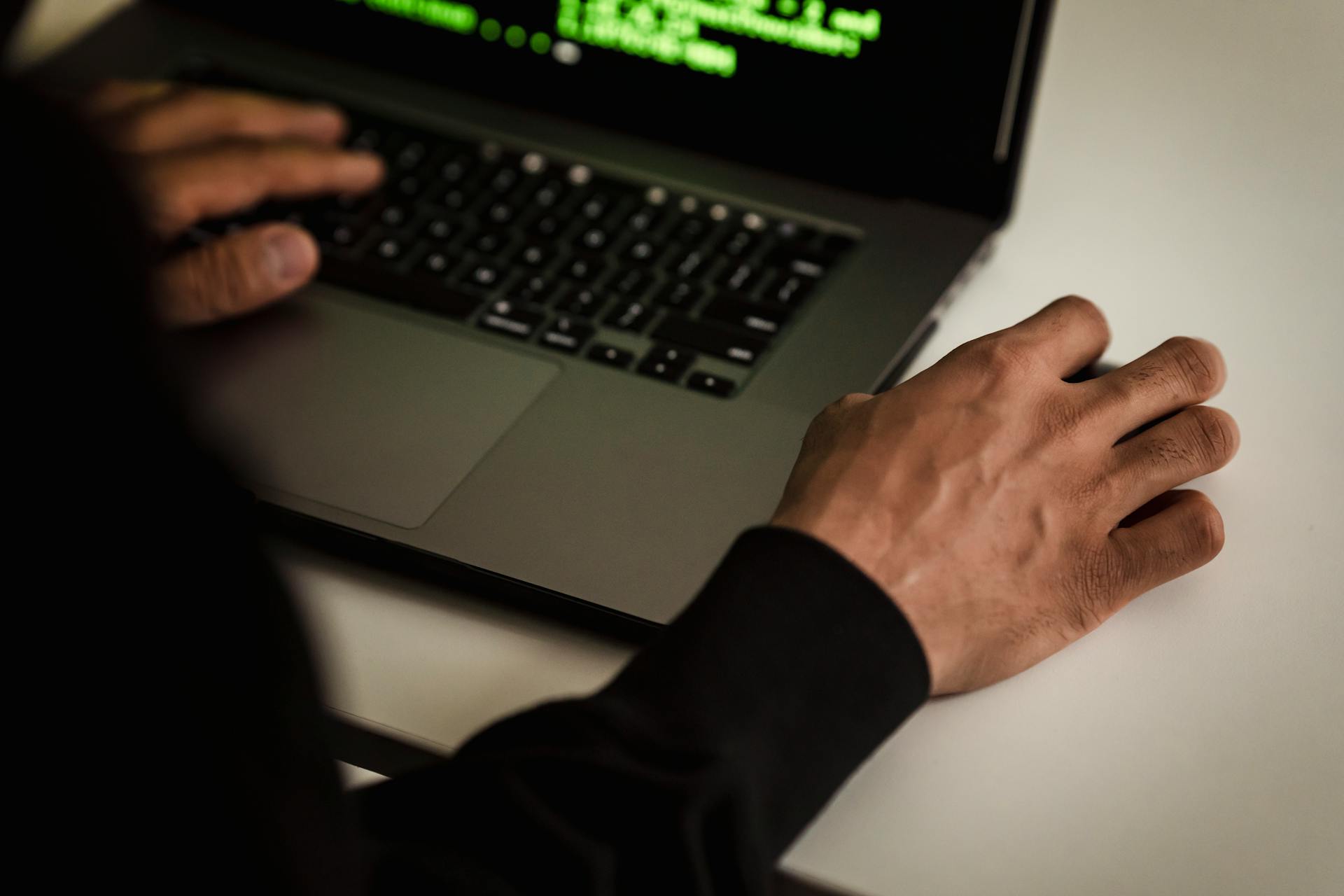
Nextjs is a React-based framework that allows you to build server-rendered and statically generated websites and applications. It's known for its speed and performance.
To get started with Nextjs Sass, you'll need to install the required packages, including sass and next-sass. This will enable you to write Sass code in your Nextjs project.
With Nextjs Sass, you can separate your styles from your JavaScript code, making it easier to maintain and update your project. This is especially useful for large-scale applications.
By following the installation process and setting up your project, you'll be able to take advantage of the benefits of Nextjs Sass and create efficient and scalable applications.
Readers also liked: Nextjs Code Block
Getting Started
To get started with Next.js and Sass, you'll need to install the sass package from npm. This will enable Sass support in your Next.js project.
First, you need to install the sass package. You can do this by running the following command inside your project: npm install sass.
Consider reading: Install Next Js 13
Next, you'll need to install the sass package using npm or yarn. The command is the same for both: npm install sass.
Installing Sass is a straightforward process. To get started, simply run the command npm install sass inside your project.
After installing Sass, you can start using it in your Next.js project. Notice how the darken() function can be used to create a hover state without defining another variable.
Additional reading: Using State in Next Js
Setting Up Sass
Setting Up Sass is surprisingly easy in Next.js, thanks to the framework's ability to do a lot of the heavy lifting for us.
To get started, you'll need to install the sass package from npm. You can do this using npm: npm install sass, or using yarn: yarn add sass.
Next.js will now be able to import and compile Sass files for you, making it easy to define variables, mixins, and other Sass constructs in your .scss files.
To set up Sass in your Next.js project, simply define some variables in a file like styles/Home.module.scss.
On a similar theme: Next Js Npm Install
Installing Sass is the first step to getting started with Sass in your Next.js app. To do this, run the command npm install sass or yarn add sass in your project directory.
After installing Sass, start back up your development server and reload the page to see the results. You'll notice that nothing has happened yet, which is a good thing - it means everything is working as expected.
Writing Sass Code
To write Sass code, start by creating a file with a .scss or .sass extension, typically placed in the styles directory or alongside components. This file will hold your global styles.
You can create a file named globals.scss for global styles, and add your Sass styles to it. This is a great place to define reusable styles that can be used across your application.
Import the Sass module into your component and use the styles as an object, as shown in Example 3. This will allow you to access your Sass styles in your JavaScript code.
Intriguing read: Nextjs Project Structure
Rename to .scss
As you start working with Sass, you'll need to rename your CSS files to use the SCSS extension. This is a crucial step in getting started with Sass-specific features.
To rename your files, look for the .css extension and replace it with .scss. For example, you might have a file called styles/globals.css, which you'll need to rename to styles/globals.scss.
Here are some specific file renames to keep in mind:
- styles/globals.css → styles/globals.scss
- styles/Home.module.css → styles/Home.module.scss
Remember to update the corresponding import statements in your code to match the new file extensions.
Importing Files
To import files in a Next.js app, you need to import Sass files with either the .sass or .scss extension. This is because Next.js supports both Sass and SCSS syntax.
To get started, rename any Sass file with the .sass extension to .scss. This will ensure that Next.js can compile the file correctly.
In your pages/_app.js file, import global styles by importing the globals.scss file. This will apply the global styles throughout your application.
For each component that requires scoped styles, create a corresponding CSS Module file with the .module.css extension. This will allow you to add component-specific styles to the file.
You can also import Sass files from third-party libraries in your pages/_app.js or specific component files.
Explore further: Next Js Pages
Using Variables
Variables in Sass allow you to easily manage colors or other values throughout your application.
You can define a variable at the top of your page, like $color-primary, and use it in your styles.
Updating the variable value allows you to change the color without altering the CSS directly.
Variables are just the start to the superpowers Sass gives your CSS.
You can define a set of variables for each theme, like light and dark modes, and create a mixin that applies the appropriate theme based on a class.
This enables you to toggle the theme class on the body element and switch between themes.
Sass variables can be used with CSS variables to create a refined theming experience in Next.js applications.
Initializing styles with Sass variables and overriding them with CSS variables allows for real-time modifications without a page refresh.
Efficient utilization of CSS variables minimizes the risk of performance hitches, keeping animations and transitions sleek.
Consistent naming conventions and a singular source, typically via a _variables.scss file, serves as a scalable foundation for theme customization.
A different take: Nextjs Build Fails Environment Variables
Managing Styles
Managing styles in Next.js with Sass can be a breeze. You can use CSS Modules for component-level styles, creating a CSS file with the .module.css extension for styles scoped to specific components.
To import and use the styles in your component, simply import the CSS file and use the styles as needed. This ensures that component-scoped styles remain isolated.
You can also use Sass for component-specific styles by creating a Sass file with the .module.scss extension. For example, create a file named MyComponent.module.scss and add your Sass styles to it.
CSS Modules can be combined with Sass for scoped styles by naming your files with the .module.scss extension. This ensures styles are scoped locally to components.
Global styles can be optimized with CSS-in-JS, combining with CSS-in-JS libraries like styled-components for component-level styles while keeping global styles in Sass. This provides flexibility and avoids style conflicts.
Regularly reviewing and refactoring styles is crucial for maintainability. Use tools to identify unused CSS and optimize styles accordingly.
Readers also liked: Nextjs Server Actions File Upload
To maintain global styles, import the global CSS file into your custom App component, which is defined in pages/_app.js. This ensures that the global styles are applied across all pages.
For dynamic theming, use CSS variables to manage different themes within your application. This allows you to change themes without altering CSS directly.
Sass variables can be used to easily manage colors or other values throughout your application. For example, you can update each location that uses a color in your home CSS file to your new variables.
To implement theming with Sass variables, define a set of variables for each theme and create a mixin that applies the appropriate theme based on a class. You can then toggle the theme class on the body element to switch between themes.
Consider reading: Nextjs Theme
Advanced Sass Features
Advanced Sass Features are a game-changer for Next.js projects, allowing developers to write more maintainable and efficient code. Variables for storing reusable values like colors, fonts, and spacing are a great place to start.
Some of my favorite Sass features include variables, mixins, nesting, functions, and inheritance. These features can be used to encapsulate styles, write more concise code, and share styles between selectors.
Here are some key benefits of using these features:
- Variables for storing reusable values
- Mixins for encapsulating styles
- Nesting for writing more concise code
- Functions for complex operations and dynamic values
- Inheritance for sharing styles between selectors
By leveraging these features, developers can write more maintainable and efficient code, and enjoy the benefits of a more responsive and agile build process.
Leveraging Functions and Mixins
Functions and mixins are powerful tools in Sass that can help you write more efficient and maintainable code. They allow you to create reusable blocks of code that can be used throughout your project, reducing duplication and making it easier to update styles.
You can create custom functions and mixins to handle complex styles and calculations, improving maintainability and consistency. For example, you can define a mixin for responsive design, which can be used to create styles that adapt to different screen sizes.
Here are some examples of how you can use functions and mixins:
By using functions and mixins, you can write more concise and readable code, and make it easier to manage complex styles and layouts.
Build-Time Optimization
Next.js will handle Sass compilation and optimization automatically during the build process. Ensure you run next build to apply these optimizations for production.
You can effectively integrate Sass into your Next.js project by following these steps, enabling advanced styling capabilities and enhancing the maintainable of your styles.
Next.js will handle Sass compilation and optimization automatically during the build process. This means you can focus on writing your Sass code without worrying about the complexities of compilation.
Advanced build-time optimizations can be configured using Next.js plugins or custom Webpack configurations to reduce CSS file sizes and enhance performance.
Optimizing Sass builds can make a significant difference in the performance of your application, especially in large-scale projects.
By leveraging the power of SWC, Next.js 14 alleviates concerns about Sass compilation requirements, offering improvements in build times which are especially palpable in larger projects.
Next.js will automatically handle Sass compilation and optimization during the build process, so you can focus on writing your Sass code without worrying about the complexities of compilation.
With the introduction of SWC's Sass support in Next.js 14, configurations are drastically simplified or, in many cases, rendered unnecessary, removing a friction point in project setup.
Suggestion: Nextjs Open Source Personal Projects
Best Practices
To get the most out of Next.js with Sass, follow these best practices.
Separate your Sass files into separate directories for each component or page to keep your code organized and easy to maintain.
Use CSS modules to avoid global CSS variables and ensure each component has its own unique styles. This approach also helps prevent CSS conflicts and makes it easier to reuse components.
Remember to import your Sass files correctly by using the `@use` directive instead of `@import`. This will help you avoid unnecessary overhead and improve performance.
Consider reading: Apply Css Nextjs
Performance
Optimize your website's performance by extracting and inlining critical CSS for above-the-fold content using tools like critical or integrating with build-time optimizers.
This technique can significantly improve page load performance by loading the most important CSS styles first.
Extracting critical CSS is a simple yet effective way to speed up your website.
Expand your knowledge: Nextjs Performance
Regularly Review and Refactor Styles
Regularly Review and Refactor Styles is a crucial part of maintaining a clean and efficient codebase.
This process helps ensure your styles remain organized as your project evolves. You can use tools to identify unused CSS and optimize styles accordingly.
By regularly reviewing and refactoring your styles, you'll catch any unnecessary code and simplify your codebase.
This will make it easier to make changes and updates in the future. It's a good idea to schedule regular reviews to maintain your styles.
Tools can help you identify unused CSS, which can be removed to optimize your styles.
Integrating with Next.js
Integrating with Next.js is a breeze, thanks to its native support for CSS and Sass. Next.js automatically compiles and optimizes your styles during the build process, so you don't need to worry about any additional configuration.
To get started, you can set up Sass in a Next.js project by importing the globals.scss file in your custom App component, located in pages/_app.js. This ensures that the global styles are applied throughout your application.
With Next.js, you can use both CSS and Sass features to enhance your styling. CSS features like direct use of CSS for straightforward styling and media queries are available, while Sass features like variables, nesting, mixins, and partials offer more advanced styling capabilities.
Next.js also supports CSS Modules and Sass Modules, which allow you to scope styles to individual components, reducing the risk of style conflicts and making it easier to manage styles in large applications.
Here are some key benefits of integrating CSS and Sass in a Next.js project:
- CSS Features: Directly use CSS for straightforward styling with basic selectors, properties, and media queries.
- Sass Features: Leverage advanced Sass features like variables, nesting, mixins, and partials to write more modular, maintainable, and reusable styles.
When working with Sass, you can use the .sass or .scss extension. For example, you can change the import of the styles object in pages/index.js to @import 'globals.scss'; to use the SCSS syntax.
Next.js 14 has also introduced advancements in Sass processing, using the SWC-based compiler, which offers faster compilation times and improved performance. This means that developers can expect their style changes to become visible in their applications more rapidly during development, streamlining the debugging and iteration processes.
Sources
- https://betterprogramming.pub/setting-up-a-next-js-project-with-typescript-sass-and-jest-d9b2d3bfb34a
- https://medium.com/@farihatulmaria/how-to-integrate-css-and-sass-in-next-js-6264e75bc268
- https://www.freecodecamp.org/news/how-to-use-sass-with-css-modules-in-next-js/
- https://www.bomberbot.com/sass/supercharging-your-next-js-styling-workflow-with-sass-and-css-modules/
- https://borstch.com/blog/development/efficiently-using-sass-in-nextjs-14
Featured Images: pexels.com