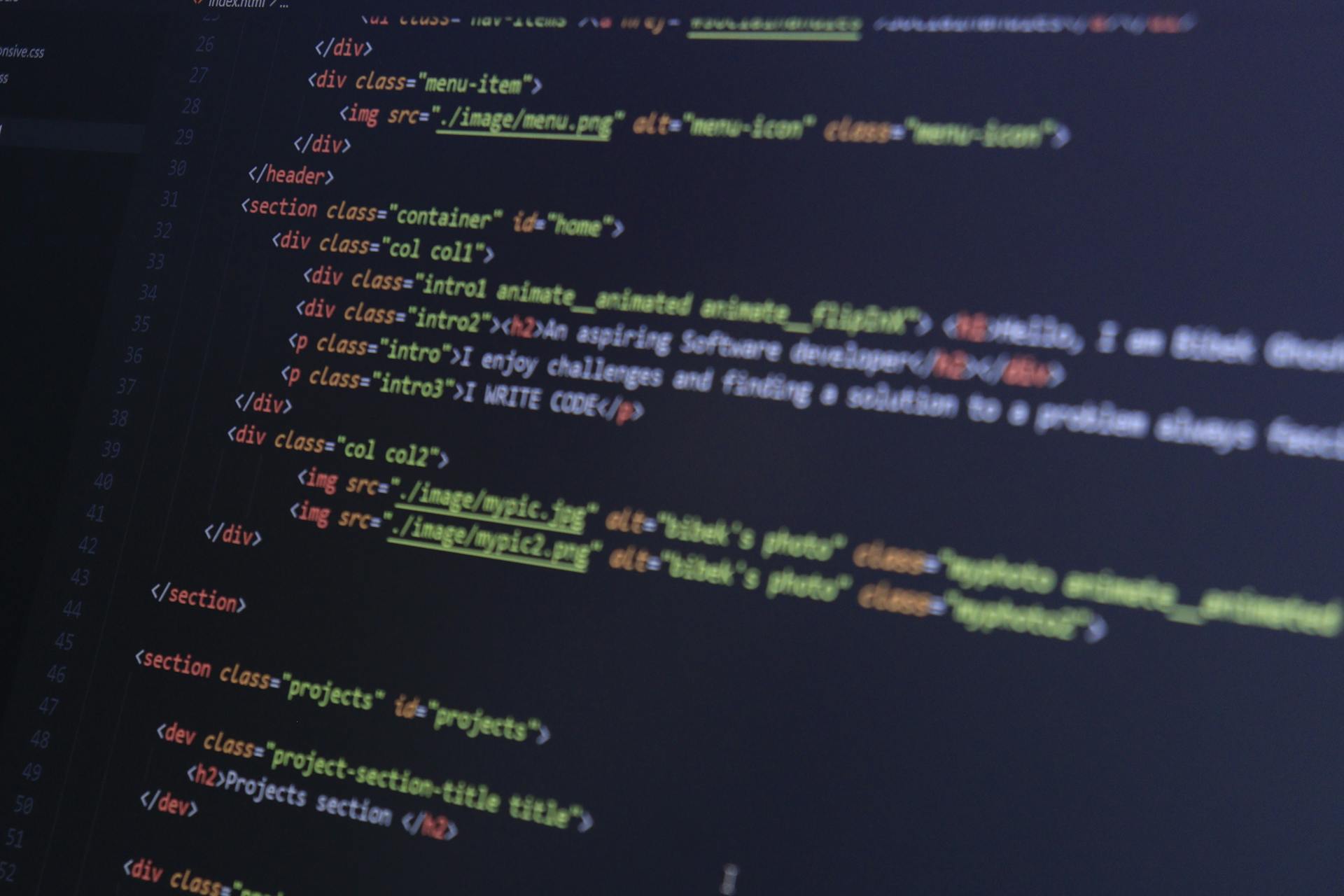
Implementing a Next.js theme is a great way to give your application a consistent look and feel. You can create a theme object with various properties, such as colors and typography.
To enable dark mode, you can add a `theme` object to your `next.config.js` file with a `darkMode` property set to `true`. This will allow users to toggle between light and dark modes.
Customizing your theme is also a breeze. For example, you can define a `colors` object with different color schemes for light and dark modes. This way, you can ensure that your application's UI remains visually appealing regardless of the mode.
By following these steps, you can create a Next.js theme that's both functional and visually stunning.
See what others are reading: Why Theme Is Important
Getting Started
To get started with a Next.js theme, you'll need to initialize a new Next.js application using Node.js. Ensure you have Node.js installed on your machine.
You can initialize a new Next.js application by running a command in your terminal. This will set up the basic structure for your project.
If this caught your attention, see: Can Nextjs Be Used on Traditional Web Application
Next.js applications require a layout to display content, so you'll need to create a layout component. You can store both your layout and header inside the components directory for organization.
The first step in implementing a layout is to create the HTML and CSS files. You can find an example of a layout implementation in the GitHub repository.
For another approach, see: Nextjs Nested Layout
Enable Color Scheme
You can indicate to browsers which color scheme is used for built-in UI like inputs and buttons by enabling the color scheme.
This is done by using the enableColorScheme property.
The color scheme will be applied based on the user's preference, whether it's light or dark mode.
To manage colors for both Light and Dark mode, create a CSS file, such as /styles/colors.css.
In this file, set your colors using CSS variables, selecting the root pseudo-class to apply to Light mode and using the [data-theme='dark'] attribute for Dark mode.
This way, the colors will be updated whenever the user toggles the theme.
To use CSS variables in your stylesheets, replace hard-coded colors with CSS variables, like the example code shows.
A fresh viewpoint: Tailwindcss Themes
Layout and Structure
A well-structured Next.js theme is key to a seamless user experience. This is achieved through the use of a modular layout.
The theme's layout is divided into sections, each with its own set of components. The layout is defined in the `pages/_app.js` file.
The theme's structure is based on a combination of CSS and JavaScript. This allows for a high degree of flexibility and customization.
If this caught your attention, see: Use Theme in Server Components Nextjs
Layout and Structure" would best fit with the heading "Create Component
To create a well-structured layout, you need to organize your project's files and components. This involves creating a components directory where you'll store your reusable UI components, such as the layout and header.
The layout implementation is an HTML and CSS task, so you can find the code in the GitHub repository. However, the header component is where the magic happens, especially when it comes to toggling between light and dark themes.
To create the header component, you'll need to create a theme-switch.jsx file in the components directory. This file will contain the code that switches the theme when the button is clicked.
Readers also liked: Next Js Components Folder
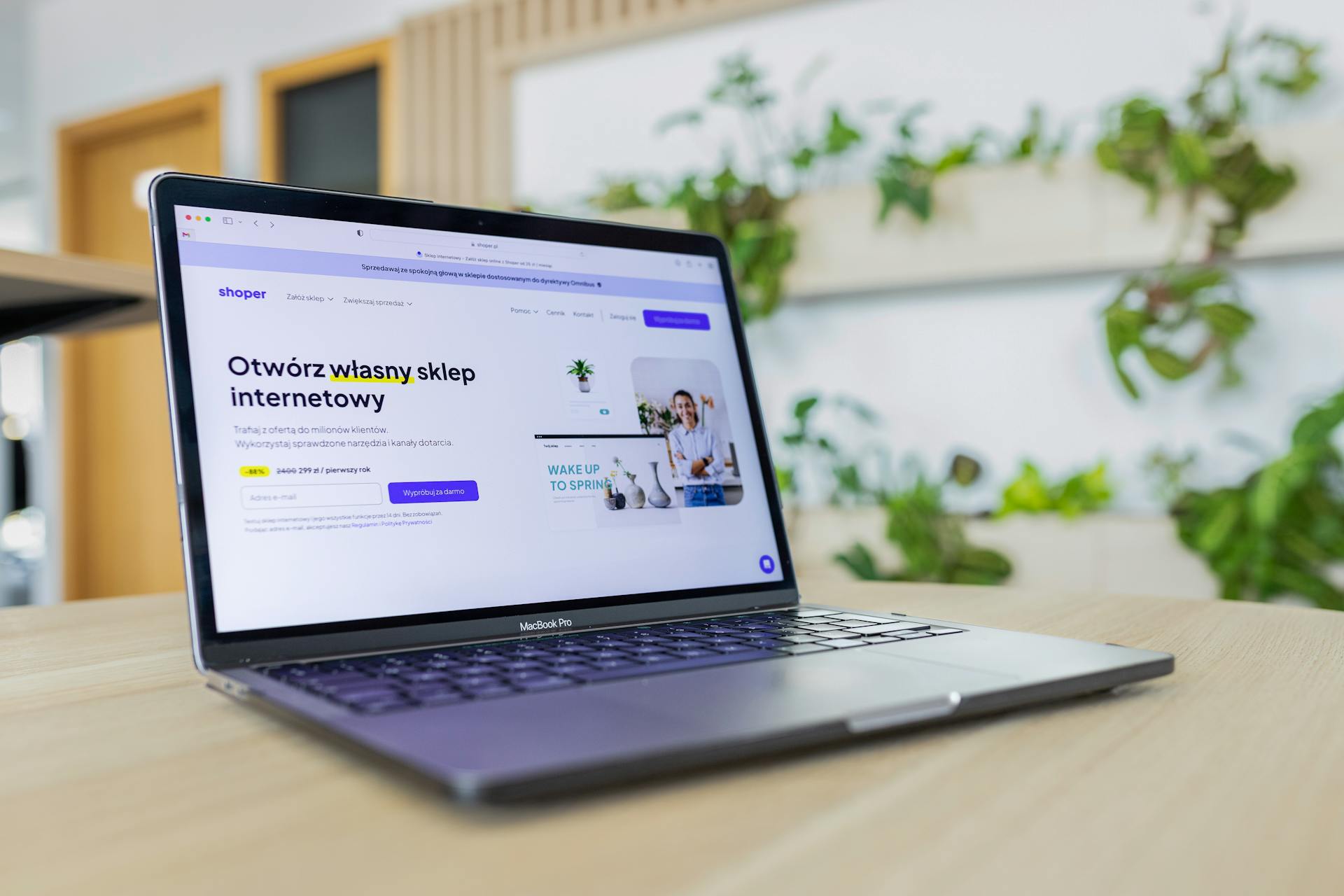
The theme-switch.jsx file is where you'll define the logic for switching between light and dark themes. You can use the following code as a starting point:
- The code uses the data-theme attribute on the HTML element to keep track of the current theme.
- The theme variable is used to determine whether the system setting is light or dark.
Here's a brief overview of the steps involved in creating the theme-switch component:
1. Create a new file called theme-switch.jsx in the components directory.
2. Add the code that switches the theme when the button is clicked.
3. Use the theme variable to determine the current theme.
By following these steps, you'll be able to create a theme-switch component that allows users to toggle between light and dark themes.
Worth a look: Next Js Component Library
Font Loading
Font loading is a crucial aspect of a well-designed layout. To ensure font loads globally, add a global style to styles in _document_.tsx: NextTamaguiProvider.tsx. This simple step can make a big difference in the overall user experience.
The global style is a key element in achieving this. It allows you to define a consistent look and feel across your application, including font loading.
Here's an interesting read: Nextjs Google Fonts
Resetting CSS
Resetting CSS can be a game-changer for consistent styles across browsers.
There is an optional CSS reset available that helps normalize styling. You can import it into your app.
The getNewCSS helper in Tamagui will keep track of the last call and only return new styles generated since the last usage.
To ensure font loads globally, add a global style to useServerInsertedHTML in NextTamaguiProvider.tsx.
App/Layout.tsx
In your app's layout file, you'll want to add a new component to include the TamaguiProvider. This involves creating a new component to wrap your app with the TamaguiProvider.
You'll need to add the skipNextHead prop to your NextThemeProvider to avoid any issues with internal usage of next/head in the app directory. This is a simple fix that will get your app up and running.
To avoid warnings about mismatched content during hydration in dev mode, you can use the suppressHydrationWarning prop.
This is a straightforward step that will help you set up your app's layout correctly.
Explore further: Nextjs Pathname Server Component
Dark Mode
Dark Mode is a game-changer for user experience. Enabling it in TailwindCSS is as simple as adding `darkmode: 'class'` to the config, which includes all the necessary CSS utility classes for dark mode.
To test dark mode in a Next.js app, you'll need to make a few changes to the `/src/_document.tsx` source file. Add the `dark` class to the HTML element to enable dark mode for the entire application.
The `darkMode` config property in TailwindCSS has two options: `class` and `media`. The `class` option allows you to select and toggle the mode, while the `media` option uses the `prefers-color-scheme` media query to automatically switch between light and dark themes based on the user's system settings.
Toggling between light and dark modes with a click is a breeze with the `useTheme` hook from `next-themes`. You'll need to import the hook, manage some state, and wire it all together in a button.
For your interest: Css3 Themes
Here's a quick rundown of the `darkMode` config options:
- `class`: Select and toggle the mode manually.
- `media`: Automatically switch between light and dark themes based on user preferences.
Enabling dark mode can also lead to slow builds due to the massive CSS file generated by TailwindCSS + Dark Mode. To alleviate this issue, you can turn on purging for the dev environment in `tailwind.config.css` by setting `purge` to an object instead of an array.
To customize the typography in dark mode, you'll need to override the defaults in your `tailwind.config.js` file by adding a `typography` property under `extend` in the `theme` section. This will allow you to extend the `@tailwindcss/typography` plugin and add a `dark` property with a CSS property that sets the color to white.
Lastly, don't forget to import Tailwind into your main CSS file and add the necessary configuration to your `tailwind.config.js` file to enable dark mode.
Take a look at this: Nextjs File Upload Api
Components and Files
In Next.js theme development, components play a crucial role in creating a seamless user experience. A Theme Switch component is created to toggle between light and dark mode.
This component is implemented in a separate file, specifically in the /components/theme-switch.jsx file. The code for this component is pasted into the file, allowing for easy switching between light and dark mode.
The Theme Switch component updates the data-theme attribute on the HTML element each time the button is clicked, effectively switching between light and dark mode.
Explore further: Can Amazon S3 Take in Nextjs File
Components and Files
You can create a CSS file for managing colors, specifically for Light / Dark mode, by creating a /styles/colors.css file. This file will store all the colors for both modes.
The root pseudo-class, such as html tag, is used to select the root element, and variables in this scope will be applied to Light mode. This is done using the :root pseudo-class.
You can apply CSS variables to your stylesheets by replacing hard-coded colors with CSS variables. This is demonstrated in an example code that shows how to write CSS variables.
Recommended read: Next Js File Upload
To toggle between light and dark mode, you need to create a Theme Switch component. This component is created by creating a /components/theme-switch.jsx file and pasting the code below.
The Theme Switch component switches the value of the data-theme attribute on the html element every time you click on the button. This is how the current theme is determined.
You can use a package called @tamagui/next-theme to properly support SSR light/dark themes that also respect user system preference. This package is pre-configured in the create-tamagui starter.
Toggle Header Component
To toggle the theme on the header component, you'll need to import the useContext hook, which takes your context as an argument.
The useContext hook is used to access the custom context that controls the theme, allowing you to change it when the toggle button is clicked.
Tailwind classes are used heavily to customize the button's style, with the dark: prefix used to recognize and apply the class after dark.
Check this out: Next Js Fetch Data save in Context and Next Route
The dark: prefix is a syntax recognized by Tailwind that uses the class after dark if a class is appended to the body of the page called dark.
You can append a class to the body of your page called dark to apply the style defined by the dark: prefix.
Expand your knowledge: Next Js 14 Redirect to Another Page Loading Indicator
Sources
- https://tamagui.dev/docs/guides/next-js
- https://webtech-note.com/posts/how-to-implement-light-dark-mode-css-vars-next-js
- https://egghead.io/blog/tailwindcss-dark-mode-nextjs-typography-prose
- https://next-auth.js.org/configuration/options
- https://javascript.plainenglish.io/how-to-implement-dark-light-themes-in-a-next-js-app-using-context-hook-tailwindcss-336558dd4579
Featured Images: pexels.com