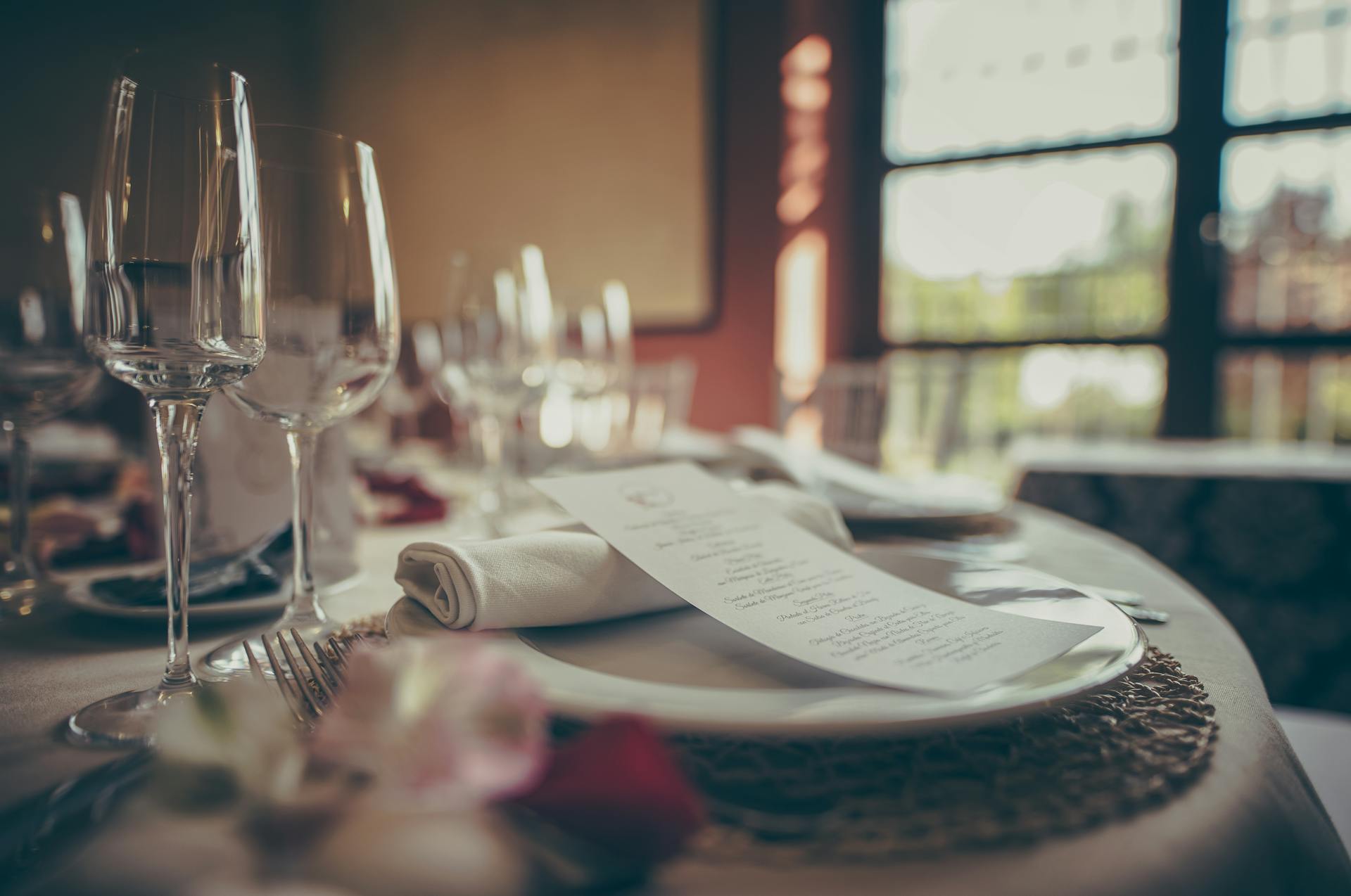
Dropdown menus with multiple levels can be a bit tricky to set up, but with the right tools, it's definitely doable.
In React, we can use a combination of state and conditional rendering to create dropdown menus with multiple levels. This allows us to dynamically render different levels of dropdown menus based on user interactions.
To achieve this, we'll need to create a nested structure of dropdown menus, where each level has its own set of options. This can be done using a recursive function or a loop that iterates over an array of options.
The key to making this work is to use a consistent naming convention for our state variables and to keep track of the current level of the dropdown menu.
A unique perspective: Why Use Tailwind Css
Setting Up
To set up a dropdown menu with multiple levels in React using Tailwind CSS, you'll need to start by creating a basic dropdown component.
First, create a new file for your dropdown component and import the necessary dependencies.
For another approach, see: Dropdown Menu Html Css Responsive
Next, define the basic structure of your dropdown menu using HTML and Tailwind CSS classes.
You can use the `nav` element as the container for your dropdown menu and apply the `flex` class to create a horizontal layout.
Inside the `nav` element, create a `button` element to trigger the dropdown menu.
This button should have a `type` attribute set to `button` and a `class` attribute that includes `inline-flex justify-center w-8 h-8` to style it as a small button.
Here's an interesting read: Tailwind Css Nav Styling
Menu Structure
To achieve a multilevel dropdown menu, you recursively render the menu items. This is done by delegating the rendering of the menu items to a separate component, such as the MenuItems component.
The Dropdown component can import the MenuItems component and pass the submenu via the items prop. This allows for the creation of nested dropdown menus.
A dropdown item can have menu items, another dropdown item, and so on, making it necessary to detect the dropdown depth level to position the dropdown logically.
This can be achieved by using a reference object to access the DOM elements of the dropdown and detect its depth level.
Keyboard Navigation
Keyboard navigation is a crucial aspect of making dropdown menus accessible and user-friendly. By allowing users to navigate through menu items using the arrow keys, we can improve the overall experience.
To achieve this, we can add a focusedIndex state to track the currently focused item, enabling users to navigate through the menu items using the arrow keys. This state is set to null initially, indicating that no item in the dropdown is focused.
We can define handlers for the onKeyDown event for both the button and menu items to manage keyboard interactions. These handlers help users navigate through options using the keyboard.
By including tabIndex and id attributes in the li element, we can assign a unique id based on its index and dynamically set the tabIndex value based on whether the current item is focused. This allows users to navigate the dropdown using keyboard arrow keys.
ARIA attributes like aria-haspopup, aria-expanded, and aria-controls provide a clear context for assistive technologies regarding the dropdown's function and state. The dropdown menu has the role of menu and an id that is linked to the button via aria-labelledby, clarifying their relationship.
In a useEffect Hook, we can locate the menu item corresponding to the focusedIndex and set keyboard focus on that specific item. This ensures keyboard navigation and proper focus management.
A well-designed dropdown menu guarantees that focus is restored to the dropdown button after a selection is made or the menu is closed during keyboard navigation. To achieve this, we can utilize useRef to link the reference object to the menu button.
By updating the handleItemKeyDown function to ensure the button receives focus when the user presses the Enter, Space, or Escape keys, we can return focus to the dropdown button on close. This ensures a seamless user experience.
Recommended read: Tailwind Css Sidebar Navigation React
Item Interaction
To enhance user experience, we can improve dropdown menu interaction by adding hover and keyboard navigation features. We can also make it easier to navigate through submenus by setting focus on the submenu item.
By implementing onMouseEnter and onMouseLeave event handlers, submenus will open and close dynamically as users hover over the menu item, providing a more intuitive experience. This feature is achieved by adding the onMouseEnter and onMouseLeave events to the primary li element.
When navigating through submenus using the keyboard, we can set focus on the submenu item by its id, enabling users to navigate through submenus with ease. This is done by adding the following code above the return statement.
Here's an interesting read: Tailwind Css Hover
Item Header
Item Header is a crucial aspect of how users interact with items on your application. To style them as headers, you can add a header property to TEDropdownItem in the dropdown.
Adding a header property to TEDropdownItem is a simple yet effective way to make your menu items stand out. This property can be used to style the header in various ways.
By incorporating a header property, you can create a visually appealing and user-friendly interface that guides the user through your application.
You might like: Tailwind Css Header
Item Interaction Enhancements
We can improve the dropdown menu experience by adding hover interactions for submenus, making it easier for users to navigate.
By implementing onMouseEnter and onMouseLeave event handlers, the submenu will open and close dynamically as users hover over the menu item.
We'll update the primary li element to include the onMouseEnter and onMouseLeave events, along with an updated className. This will ensure clearer visual feedback for users.
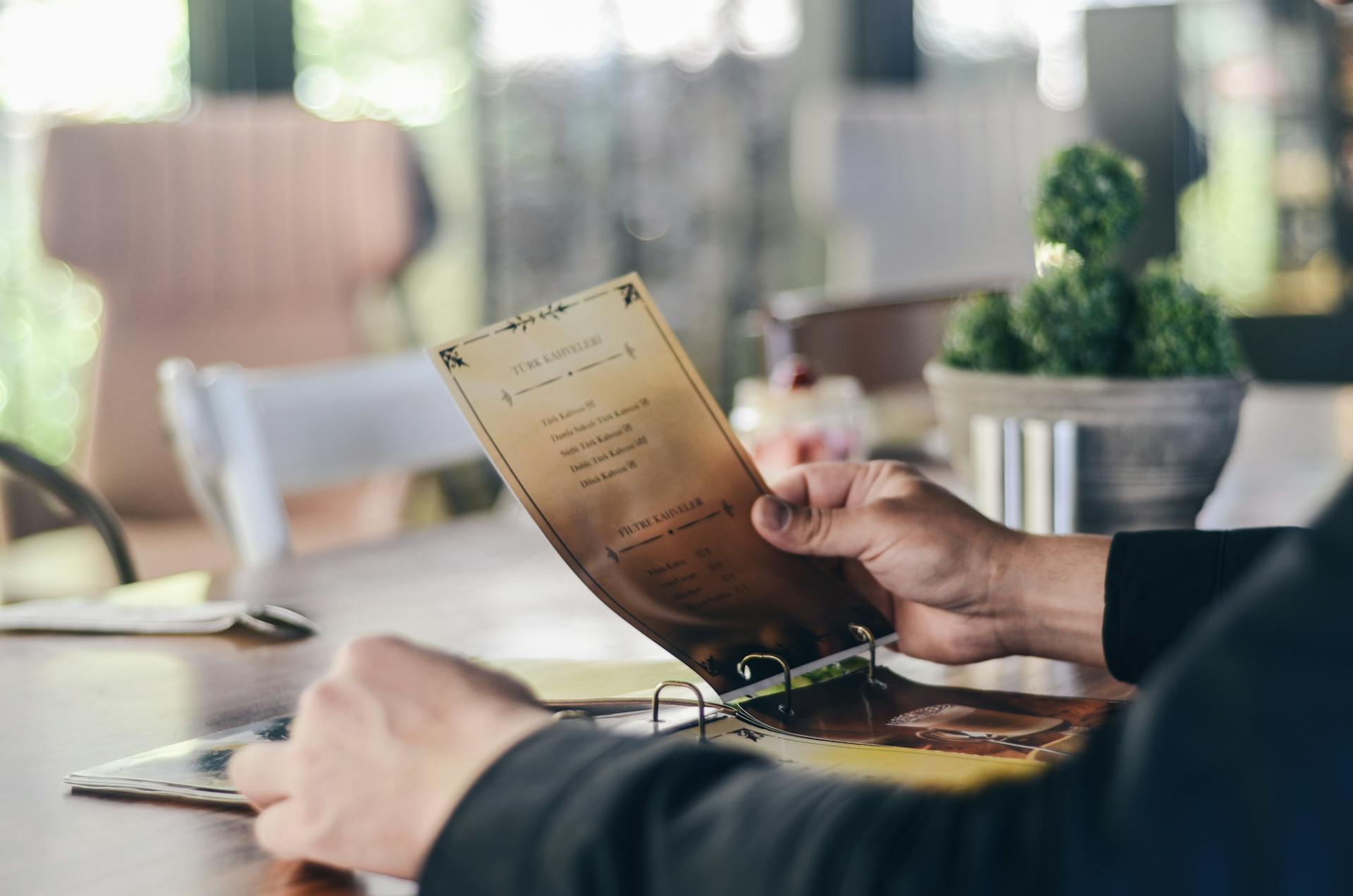
To enhance the dropdown, we can add icons, links, and actions, making it more versatile for different use cases. Update the li element to display an icon, a clickable link, or an action button.
If an item has a URL, it displays as a clickable link. Otherwise, it renders as a button that triggers an optional action. This adds flexibility and interactivity to the dropdown.
To improve user experience, we ensure the dropdown closes after an item is clicked by calling setOpen(false).
Recommended read: Tailwind Css Snippets Link
Rendering and Layout
Rendering a multilevel dropdown menu requires recursively rendering menu items. This is achieved by delegating the rendering of menu items to a separate component, like the MenuItems component.
To position submenus logically, we can detect the dropdown depth level. This is crucial when submenus overlap each other. For instance, when clicking the "web development" submenu, its dropdown should be positioned to the right.
Managing keyboard navigation within submenus is also essential. The handleSubmenuKeyDown function handles keyboard interactions, allowing users to move between items with the arrow keys, select an item, or close the submenu with the escape key. This enhances the overall user experience.
Classes
Custom classes can be passed to elements via the theme prop, allowing for more control over the layout and styling of your application.
To do this, you'll need to create an object with the classes you want to apply, like this: `NameDefaultDescriptiondropdownrelativeSets styles to the TEDropdown element.`
This object can then be passed to the theme prop, giving you fine-grained control over the styling of your application.
The classes available for styling the TEDropdown element include dropdown, relative, and others, which can be used to create a unique and customized layout.
Here's a list of some of the classes you can use:
By using these classes, you can create a customized layout that meets the specific needs of your application.
Rendering the Items
Rendering the items is a crucial part of creating a seamless user experience. To achieve this, we can use a technique called recursive rendering, which involves delegating the rendering of menu items to a separate component, as we see in the MenuItems component.
By passing the submenu via the items prop, we can render menu items with ease. This approach is particularly useful when dealing with multilevel dropdown menus.
To further enhance the user experience, we can use the handleSubmenuToggle function to toggle the open state of the submenu based on the clicked item using its index. This allows users to easily navigate through the menu items.
The handleSubmenuKeyDown function is also essential in managing keyboard navigation within the submenu. It enables users to move between items with the arrow keys, select an item, or close the submenu with the escape key.
To set focus on the submenu item, we can use the useRef to access the DOM elements of the dropdown. This allows us to logically position the dropdown by detecting the dropdown depth level.
By using these techniques, we can create a dropdown menu that is both functional and visually appealing.
Multilevel Menu
To create a multilevel menu in React, you can start by setting up a project with a multilevel dropdown menu. Let's open the src/menuItems.js file and update the data to include multilevel submenu components.
We can achieve this design by recursively rendering the menu items. In the Dropdown component, delegate the rendering of the menu items to the MenuItems component by passing the submenu via the items prop.
To logically position the dropdown menus, we need to detect the menu depth level. We can do this by accessing the depthLevel and using it to display dropdown arrows in the MenuItems component.
For the depthLevel greater than 0, we display a right arrow using an HTML entity name, », else we add an .arrow class name to style a custom down arrow. This allows us to dynamically add varying arrows to show that a dropdown exists.
We can also use the depthLevel to detect a “second and above” level dropdown, hence logically positioning them to the right of the submenu. In the components/Dropdown.js file, access the depthLevel prop, increment it, and check if the value is greater than 1 so we can add a custom class to the dropdown.
To further enhance the multilevel menu, we can update the handleItemKeyDown function to handle keyboard interactions within the primary dropdown. Specifically, we’ll enhance it by adding support for the “Arrow Right” key and updating the behavior for the Enter and Space keys to open submenus when available.
By following these steps, you can create a fully functional multilevel menu in React, complete with keyboard navigation and dynamically positioned dropdowns.
Related reading: Tailwind Css Display Card
A Linkable Button
A linkable button can be achieved by ensuring the dropdown menu includes its URL path. This allows the button to point to its own page while still showing dropdown items on large screens.
To make a dropdown menu button linkable, we need to check not only the submenu but also the URL. This is done by expanding the conditional check in the components/MenuItems.js file.
The button should be linkable, so we will ensure the button element is implemented like so. This will make the button functional and point to its own page.
By including the URL path in the dropdown menu, we can create a linkable button that meets our needs.
Behavior and Timing
In dropdown menus, timing is everything. You can use the data-dropdown-delay={milliseconds} data attribute to set the delay on when to show or hide the dropdown menu when using hover.
This delay can be adjusted to suit your interface's needs, and the default delay is 300 milliseconds. For example, adding 500 milliseconds can give users more time to interact with your menu before it hides.
The right timing can make a big difference in user experience, so it's worth taking the time to get it just right.
Enhancing the
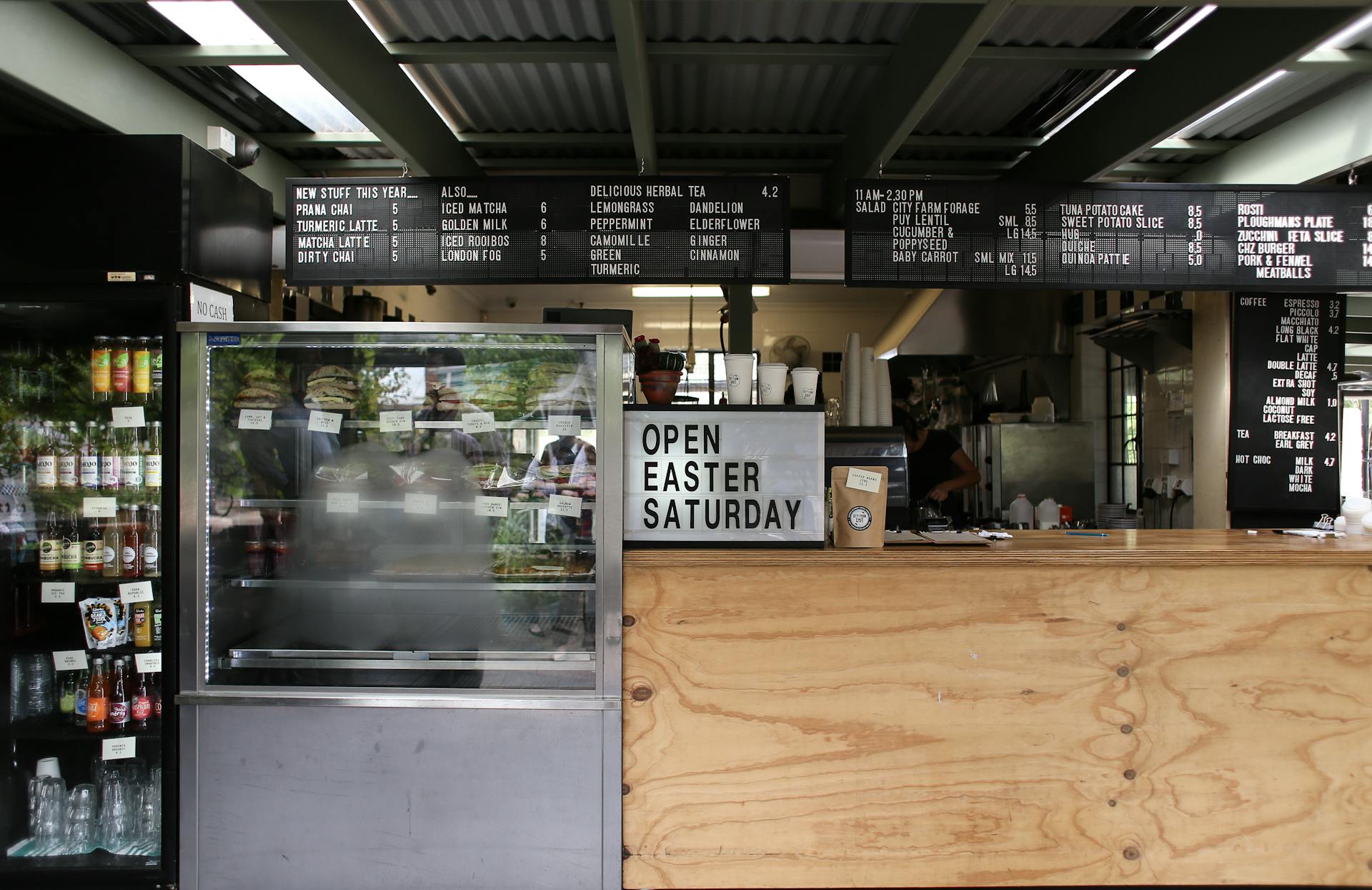
Enhancing the dropdown to support icons, links, and actions can make it more versatile for different use cases. By adding icons to each item, we can make the dropdown more visually appealing and easier to use.
If an item has a URL, it displays as a clickable link, otherwise it renders as a button that triggers an optional action. This flexibility is achieved by dynamically rendering either navigation links or action buttons.
To improve user experience, the dropdown closes after an item is clicked by calling setOpen(false). This prevents the dropdown from staying open after an item is selected.
Adding icons to the dropdown can also make it more intuitive to use. For example, the IoIosArrowForward icon from the react icons library can be used to indicate a link or a button.
By making the dropdown more interactive, we can create a more engaging user experience. This is especially important for use cases where the dropdown is used to navigate to different pages or perform actions.
You might like: Tailwind Css Icon
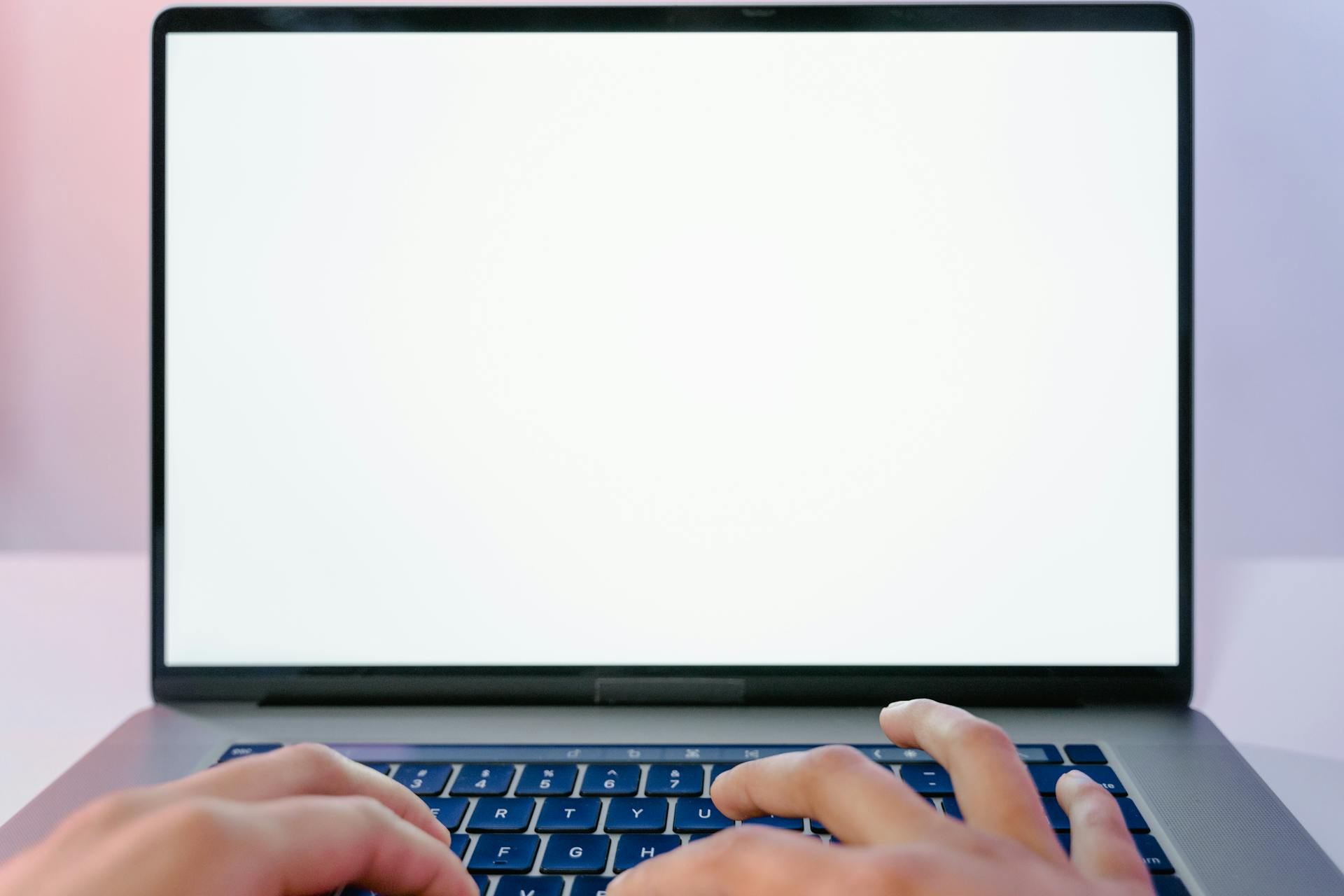
In some cases, we may want a dropdown menu button to point to its own page while still showing the dropdown items when we hover over it on a large screen. To achieve this, we need to ensure the dropdown menu includes its URL path.
By checking for the presence of a URL and a submenu, we can determine whether to render a button or a link. This allows us to create a more flexible and user-friendly dropdown menu.
Restore Focus to Button on Close
Restoring focus to the button on close is crucial for a seamless user experience. By utilizing useRef, we can link the reference object to the menu button, ensuring it receives focus when the user presses the "Enter", "Space", or "Escape" keys.
This approach was used in the dropdown menu example, where the reference object was linked to the menu button and updated in the handleItemKeyDown function. The result is a smooth transition back to the button after the dropdown closes.
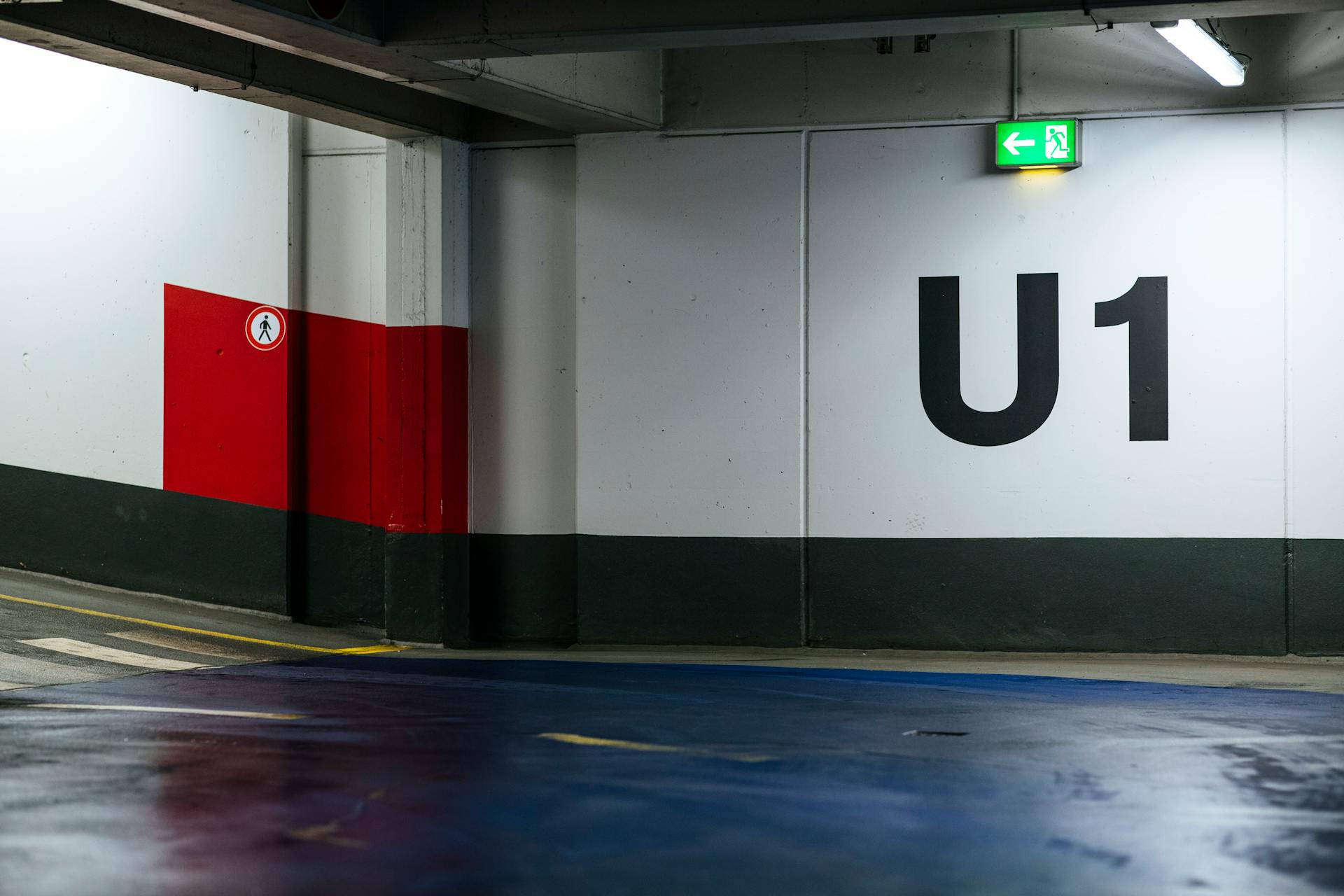
The focus outline added to the menu button provides a clear visual indication during keyboard navigation, which is essential for accessibility. This is especially important when users need to see which element is focused on.
By restoring focus to the button on close, we can improve the overall user experience and provide a more predictable interaction. This is achieved by maintaining the intended state of the dropdown and its submenus upon toggling.
You might enjoy: Tailwindcss Button
Closing Item on Click
Closing an item on click is an essential aspect of creating a seamless user experience. By setting the dropdown state back to its default value of false, we can ensure that the dropdown panel closes when its item is clicked.
This functionality is crucial for maintaining a predictable user experience. We can achieve this by adding a click event to the menu item, which invokes a function to set the dropdown state back to its default value.
In our code, this is implemented by updating the handleToggle function to reset the focus and submenu states when the dropdown is closed. This provides a more intuitive experience for users.
By incorporating this feature, we can enhance the overall usability of our application. The dropdown management is improved, ensuring a complete reset of the focus and submenu states when the dropdown is closed.
In addition to this, we can also define the logic that detects when a dropdown menu item is clicked, allowing us to dynamically display or hide the dropdown box. This is achieved by adding a state and updating it on the dropdown menu click.
To implement this, we add a state variable called dropdown with a default value of false and a setDropdown updater to toggle the state when the dropdown button is clicked. This allows us to dynamically add value to the aria-expanded attribute to indicate if a dropdown box is expanded or collapsed.
On a similar theme: How to Add Custom Css in Tailwind
Delay Duration
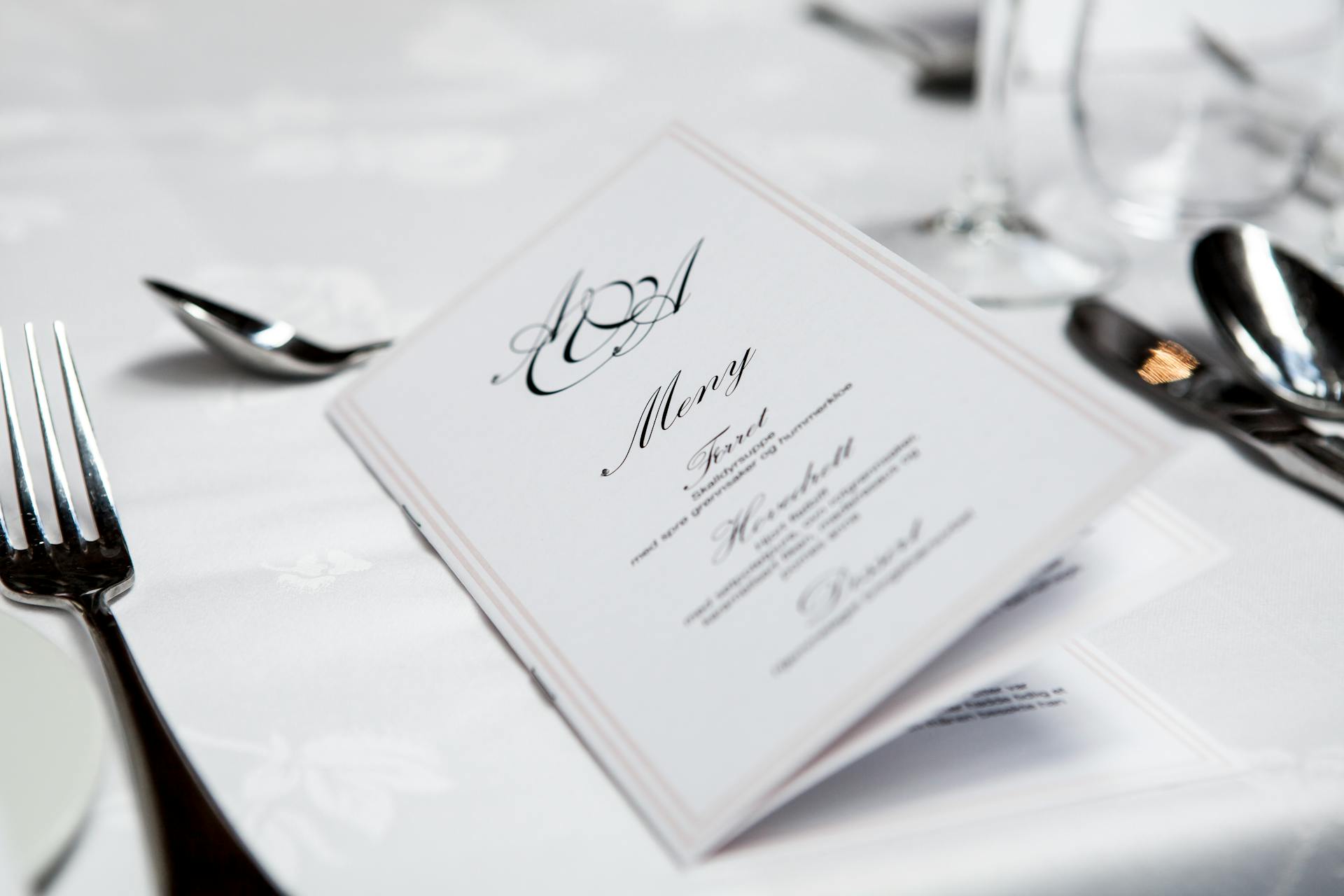
Delay Duration is a crucial aspect of user interface design, and it's essential to understand how to control it. You can use the data-dropdown-delay={milliseconds} data attribute to set the delay on when to show or hide the dropdown menu when using hover.
This attribute allows you to customize the default delay of 300 milliseconds. For example, adding 500 milliseconds can significantly impact user interaction with your interface.
The choice of delay duration depends on how users interact with your interface.
Sources
Featured Images: pexels.com