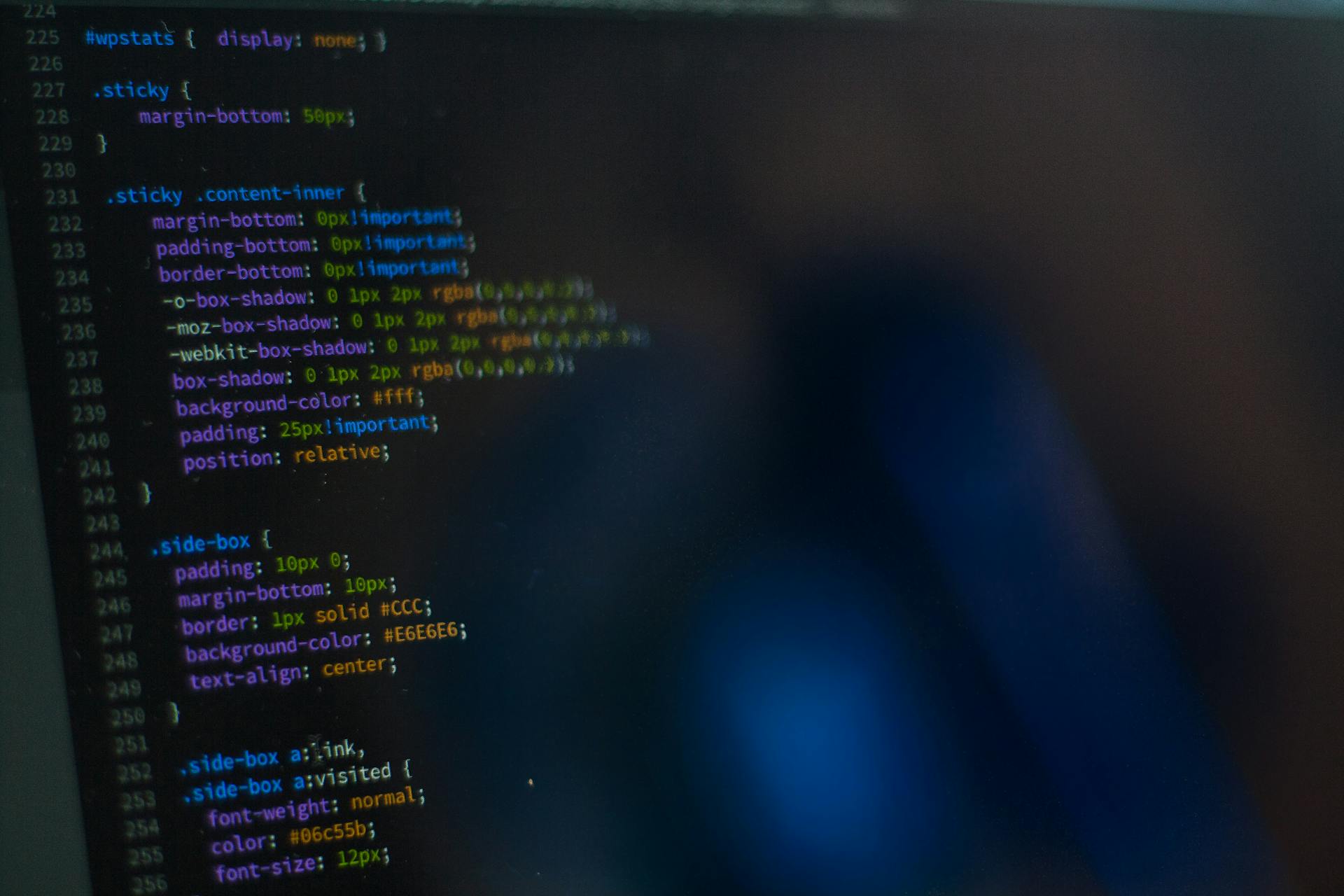
Hiding text in CSS can be a useful technique for various purposes, such as creating a cleaner design or hiding sensitive information.
You can hide text using the `display: none` property, which completely removes the element from the layout.
To use `display: none`, simply add the following code to your CSS: `display: none;` This method is easy to implement and works well for hiding text that should not be visible at all.
However, there are other methods for hiding text, such as using the `visibility: hidden` property, which hides the text but still takes up space in the layout.
This method is particularly useful when you want to hide text but still want the layout to adjust accordingly.
If this caught your attention, see: Css How to Override Style Class Using Stylesheet
Display Property
The display property is a powerful tool for hiding text in CSS, but it's not as straightforward as it seems. It completely removes the element from the DOM, making it invisible to the user and inaccessible to screen readers.
Using display: none will prevent event listeners from registering, so if you have a button with a click event, it won't work if the button is hidden with display: none.
One of the most interesting facts about display: none is that it affects image requests differently than other elements. If an image is applied with a background property, it won't be downloaded, but if it's applied with a display property set to none, it will still be downloaded.
Here's a quick rundown of the key differences between display: none and visibility: hidden:
As you can see, display: none is a more extreme solution that removes the element entirely, while visibility: hidden simply makes it invisible.
How to Hide Elements
Hiding elements can be a bit tricky, but with the right tools, it's a breeze. You can use the Inspect tool in your browser to find the CSS ID or Class of the element you want to hide.
To do this, right-click on the element and select Inspect. This will open the Dev Tools pane, letting you find the correct selectors to use in your CSS file.
If you're working with WordPress, you'll need to find the Page ID Class for the specific page you want to hide the element on. You can do this by looking at the URL for the edit page - the number listed in the URL is the Page ID.
You can use this ID in your CSS selector to target elements on that specific page and nowhere else on the site. For example, if the Page ID is 1337, you can use the CSS selector `.page-id-1337` to hide the element on that page.
If you're using Tailwind CSS, you can use breakpoints to hide elements on specific screens. For example, you can use the `lg` breakpoint to hide an element on large screens, but show it on smaller screens.
The `:empty` pseudo-class is also a great tool for hiding elements. It matches any element that has no children, and can be used in combination with the `display` property to hide an element if it's empty.
A different take: Css Selector Text Contains
You can also use the `:not()` pseudo-class in combination with `:has()` to hide a parent element that doesn't contain a certain child. For example, you can use `:not(:has(.result))` to hide a `.container` element if it doesn't contain a `.result` element.
It's worth noting that `display: none` is still a valid solution for hiding elements, but it's been superseded by more flexible and animatable options like `transform` and `opacity`.
Visibility Property
The visibility property is a powerful tool in CSS that allows you to hide an element without removing it from the DOM. It's incredibly different from display: none, as the element is still generated and rendered, but invisible.
When you set an element's visibility property to hidden, it's called "visually hidden." This means the element's box model is present, giving it dimensions that continue to occupy space on the screen, even though it doesn't appear to be there.
The element's box model being present is a key difference between visually hidden and not displayed. This means that the element will still take up space in the design, unlike display: none which removes the element entirely.
Take a look at this: Box around Text Html Css
Here's a breakdown of how visually hidden elements behave in terms of accessibility and event triggers:
As you can see, visually hidden elements are inaccessible to screen readers and won't register events. However, they will still take up space in the design and affect the document layout. This makes visibility:hidden a useful tool when you want to remove an element's visibility but still maintain its space in the design.
Discover more: Space between Text Css
Accessibility
Visually hiding an element may not always hide the content from assistive technologies, so be aware that a screen reader could still announce tiny transparent text.
To truly hide content, you may need to use additional CSS properties or ARIA attributes, such as aria-hidden="true".
Some people may experience disorientation, migraines, seizures, or other physical discomfort due to animations, so consider using a prefers-reduced-motion media query to switch off animations when specified in user preferences.
This can be done to make your website more accessible and comfortable for users with certain needs.
Here's an interesting read: Hide a Class Css
Putting It All Together
We got to a solution that will visually hide content but still be accessible. This solution involves using a combination of CSS and ARIA attributes.
To visually hide content, display: none is still the best way to go. It completely hides the element from view.
If you want to hide something from a screen reader, the aria-hidden="true" attribute is the way to go. This will hide the content from screen readers, but not visually.
A complete table comparing all the approaches is provided to guide your decisions on how to hide content. This table will help you choose the right method for your specific situation.
See what others are reading: Table Stylesheet Css Examples
Finding and Wrapping Up
To find the element you want to hide, start by right-clicking on it and selecting Inspect. This opens the Dev Tools pane in your browser, allowing you to identify the CSS ID or Class it uses.
The element will be highlighted as you hover over and click the correct lines in the inspect tool to the right. You'll see the selector syntax attached to the element on hover, which is what you'll use in your CSS file or field.
Knowing the difference between CSS properties visibility and display can help you customize your pages. You might need to hide an image or remove meta-data on a specific page.
For another approach, see: Hover Css Effects Text
Finding the Element
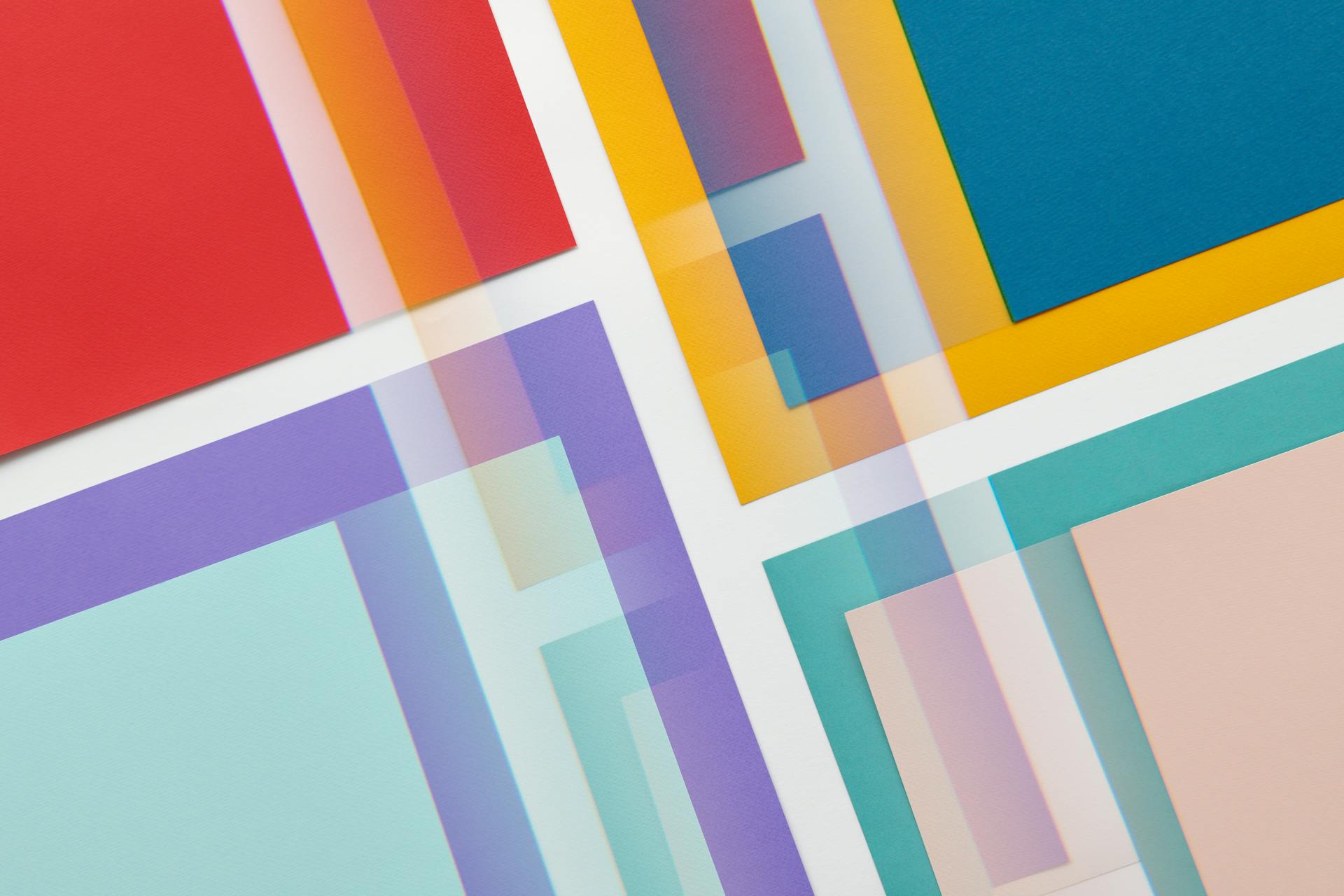
If you know which element you want to hide but not what to call it, you can right-click on it and select Inspect.
This will open the Dev Tools pane in your browser, letting you find the CSS ID or Class it uses.
The element will be highlighted as you hover over and click the correct line(s) in the inspect tool to the right.
You can use the selector syntax attached to the element on hover to adjust with CSS.
The in-line selectors in the inspect tool are what’s rendered by the browser.
Expand your knowledge: Css Hover Text
Wrapping Up
You might need to hide elements on specific WordPress pages for various reasons, such as removing an image that's not needed on a certain page.
Knowing the difference between CSS properties visibility and display is key to customizing your WordPress site. This knowledge can help you highlight specific pages and make them stand out.
Sometimes, you might need to remove meta-data or dates from a blog post. In such cases, using a variant of hide element CSS can help you achieve your goal.
CSS properties like visibility and display can be used to customize and highlight different pages on your WordPress site.
You can use hide element CSS to remove elements that are not needed on a particular page, giving your site a more streamlined look.
If this caught your attention, see: Css Highlight Text
Hiding in WordPress
You can find the page or post ID in the URL for the edit page by hovering over the link and seeing the preview URL. This ID is a CSS class selector, not a CSS ID.
The page or post ID can also be found in the URL bar of any Edit or Preview page. It's the number listed in these URLs that you'll use in the CSS selector to target elements on that specific page.
To hide elements on a specific page in WordPress, you'll need to use the page ID class. This class is in the format .page-id-1337, where 1337 is the page ID.
The code to remove a header only on the page with a specific ID is straightforward. It's a simple CSS selector that targets the element on that specific page.
You can use breakpoints to hide elements on specific screens in Tailwind CSS. This is useful when you want to show an element on larger screens but hide it on smaller screens.
Curious to learn more? Check out: Jquery Apply Css Class
Key Takeaways and Choices
Hiding elements in CSS can be done in multiple ways, each with varying effects on accessibility, layout, animation, performance, and event handling.
The opacity and filter: opacity() properties can make an element fully transparent, but the element remains on the page and can still trigger events.
Using the transform property is an excellent choice for hiding elements, as it offers excellent performance and hardware acceleration by moving the element off-screen or scaling it down.
The display property is a common method to hide elements, but it can't be animated and can trigger a page layout, making it a less desirable choice in many cases.
Other methods to hide elements include the HTML hidden attribute, absolute position, overlaying another element, and reducing dimensions, each with their own pros and cons.
Here are some key differences between these methods:
Sources
- https://css-tricks.com/comparing-various-ways-to-hide-things-in-css/
- https://www.elegantthemes.com/blog/wordpress/hide-element-css
- https://tw-elements.com/learn/te-foundations/te-ui-kit/hiding-elements/
- https://tobiasahlin.com/blog/hiding-an-element-if-its-empty/
- https://www.sitepoint.com/hide-elements-in-css/
Featured Images: pexels.com