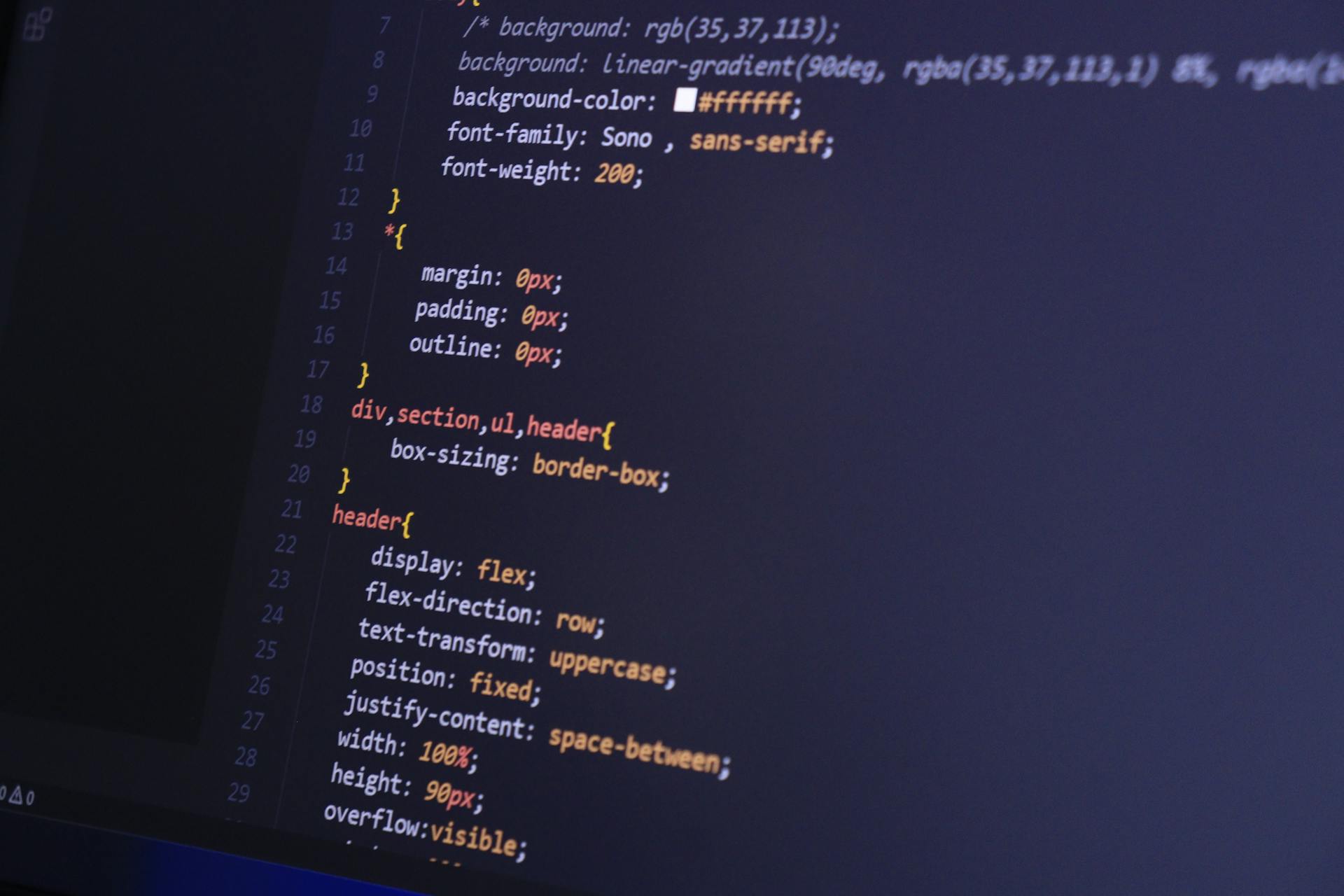
When using CSS selectors that contain text, it's essential to keep it simple and efficient.
A common best practice is to use the `:contains()` pseudo-class to select elements that contain specific text. This can be particularly useful when working with dynamic content.
For instance, if you have a list of items and you want to target the ones that contain the word "sale", you can use the `:contains()` pseudo-class like this: `li:contains('sale')`. This will select all list items that contain the word "sale".
Using the `:contains()` pseudo-class can help reduce the complexity of your CSS selectors.
Suggestion: Css Select Data Attribute in Pseudo Selector
CSS Selectors Basics
CSS selectors are like a special language that helps you find and style specific elements on a webpage. You can use them to target elements by their tag name, ID, class, and even attributes.
To start with, you can use the tagname selector to find elements by their tag name, like `div` or `p`. You can also use the `#id` selector to find elements by their ID, like `#logo`.
Related reading: Css Selector for Id
The `.class` selector is another way to find elements by their class name, like `.masthead`. This is super useful when you want to style a group of elements with the same class.
You can also use attribute selectors to find elements with specific attributes, like `[href]` or `[width=500]`. These selectors are really powerful and can help you target elements with specific values or patterns.
Here are some examples of attribute selectors:
These selectors can be used in combination with other selectors to create more complex rules. For example, you can use the `[attr^=value]` selector to find elements with an attribute value starting with a specific value, like `[href*=/path/]`.
Remember, the key to mastering CSS selectors is to practice and experiment with different selectors and combinations. With time and patience, you'll become a pro at finding and styling specific elements on a webpage.
Here's an interesting read: Css Selector That Targets a Specific Property Declaration
Attribute Selectors
Attribute selectors are a powerful tool in CSS, allowing you to select elements based on their attributes. You can use the square brackets [] to specify an attribute, and then use various selectors to filter the results.
You might enjoy: Css Selector for Data Attribute
To select an element based on the presence of an attribute, regardless of its value, simply include the attribute name in square brackets. For example, a[target] selects an element if the given attribute is present.
You can also use the asterisk character * to select elements based on part of an attribute value, but not an exact match. For instance, a[href*="login"] selects an element if the given attribute value contains at least once instance of the value stated. This is particularly useful when you need to select elements based on a partial match.
Here's a quick rundown of the different attribute selectors:
These attribute selectors can be combined with other selectors to create more complex and specific rules.
Attribute
Attribute selectors allow you to target elements based on their attributes.
You can use square brackets, [], to select an element based on if an attribute is present or not, regardless of any actual value. For example, a[target]{...} will select an element with a target attribute, even if its value is empty.
Here's an interesting read: Pseudo Element
The asterisk character, *, can be used within the square brackets of a selector to find an element based on part of an attribute value, but not an exact match. For example, a[href*="login"]{...} will select an element with an href attribute value that contains the string "login".
Here are some examples of attribute selectors:
Attribute Spaced Selector
Attribute Spaced Selectors are used to match attribute values that are whitespace-separated. You can use the tilde character, ~, within the square brackets of a selector between the attribute name and equals sign to denote an attribute value that should be whitespace-separated.
For example, a[rel~="tag"] would select an element if the given attribute value is whitespace-separated with one word being exactly as stated, like in the example:a[rel~="tag"]{...}. This is useful when you want to match attribute values that have multiple words separated by spaces.
The tilde character is a special character in CSS selectors that indicates a whitespace-separated value. It's a powerful tool for matching attribute values that have multiple words or phrases.
Here's a quick reference to help you remember how to use Attribute Spaced Selectors:
Pseudo Selectors and Alternatives
Pseudo selectors are a powerful tool in CSS, allowing you to select elements based on various criteria. For example, the :has selector finds elements that contain elements matching a specified selector, such as `div:has(p)`.
The :has selector is relational, meaning it selects an element based on its contents, not just its attributes or position in the document. This is in contrast to other CSS selectors, which are primarily attribute-based.
Some pseudo selectors are indexed, meaning they use a 0-based system to select elements based on their position in the DOM tree. For example, `:lt(n)` selects elements whose sibling index is less than `n`, while `:gt(n)` selects elements whose sibling index is greater than `n`.
Here are some pseudo selectors that can be used to find elements containing specific text:
- :contains(text): find elements that contain the given text, with case-insensitive search
- :containsOwn(text): find elements that directly contain the given text
- :matches(regex): find elements whose text matches the specified regular expression
- :matchesOwn(regex): find elements whose own text matches the specified regular expression
Note that the :has selector is not supported in browsers, so alternatives like JavaScript, jQuery, or CSS combinators like child or sibling selectors may be needed.
Pseudo Selectors
Pseudo selectors are a powerful tool in web development, allowing you to target specific elements on a webpage. They're like superpowers for your CSS.
You can use the :has(selector) pseudo selector to find elements that contain elements matching the selector. For example, div:has(p) will find any div element that contains a paragraph.
The :is(selector) pseudo selector is useful for finding elements that match any of the selectors in the list. For instance, :is(h1, h2, h3, h4, h5, h6) will find any heading element.
The :not(selector) pseudo selector does the opposite, finding elements that do not match the selector. For example, div:not(.logo) will find any div element that is not a logo.
The :contains(text) pseudo selector is great for finding elements that contain specific text. For instance, p:contains(jsoup) will find any paragraph element that contains the text "jsoup".
You can also use the :lt(n), :gt(n), and :eq(n) pseudo selectors to find elements based on their sibling index. For example, td:lt(3) will find any table data element that is before the third table data element.
Additional reading: Css Find Position of Selector
Here's a quick rundown of the indexed pseudo-selectors:
Note that these indexed pseudo-selectors are 0-based, so the first element is at index 0, the second at 1, and so on.
Alternatives to Has Selector
The :has selector is a powerful tool, but it's not supported in all browsers. This means you might need to use alternatives depending on your needs.
If you're looking for a similar effect, you could use JavaScript or jQuery. These options can achieve the same result as the :has selector, but they require more code and can be slower.
CSS combinators like the child or sibling selectors can also be used as alternatives. However, they're not as flexible as the :has selector and might not cover all your needs.
If you need to select elements that contain specific text, you could use the :contains or :containsOwn pseudo-selectors. These are case-insensitive and can be used to find elements that contain the given text.
Discover more: Css Selector the Last 2 Child Elements
Here are some examples of pseudo-selectors that can be used as alternatives to the :has selector:
- :is(selector) can be used to find elements that match any of the selectors in the selector list.
- :not(selector) can be used to find elements that do not match the selector.
- :matches(regex) and :matchesOwn(regex) can be used to find elements whose text matches the specified regular expression.
- :lt(n), :gt(n), and :eq(n) can be used to find elements based on their sibling index.
These pseudo-selectors can be used to achieve similar effects to the :has selector, but they might require more code and can be slower.
Featured Images: pexels.com