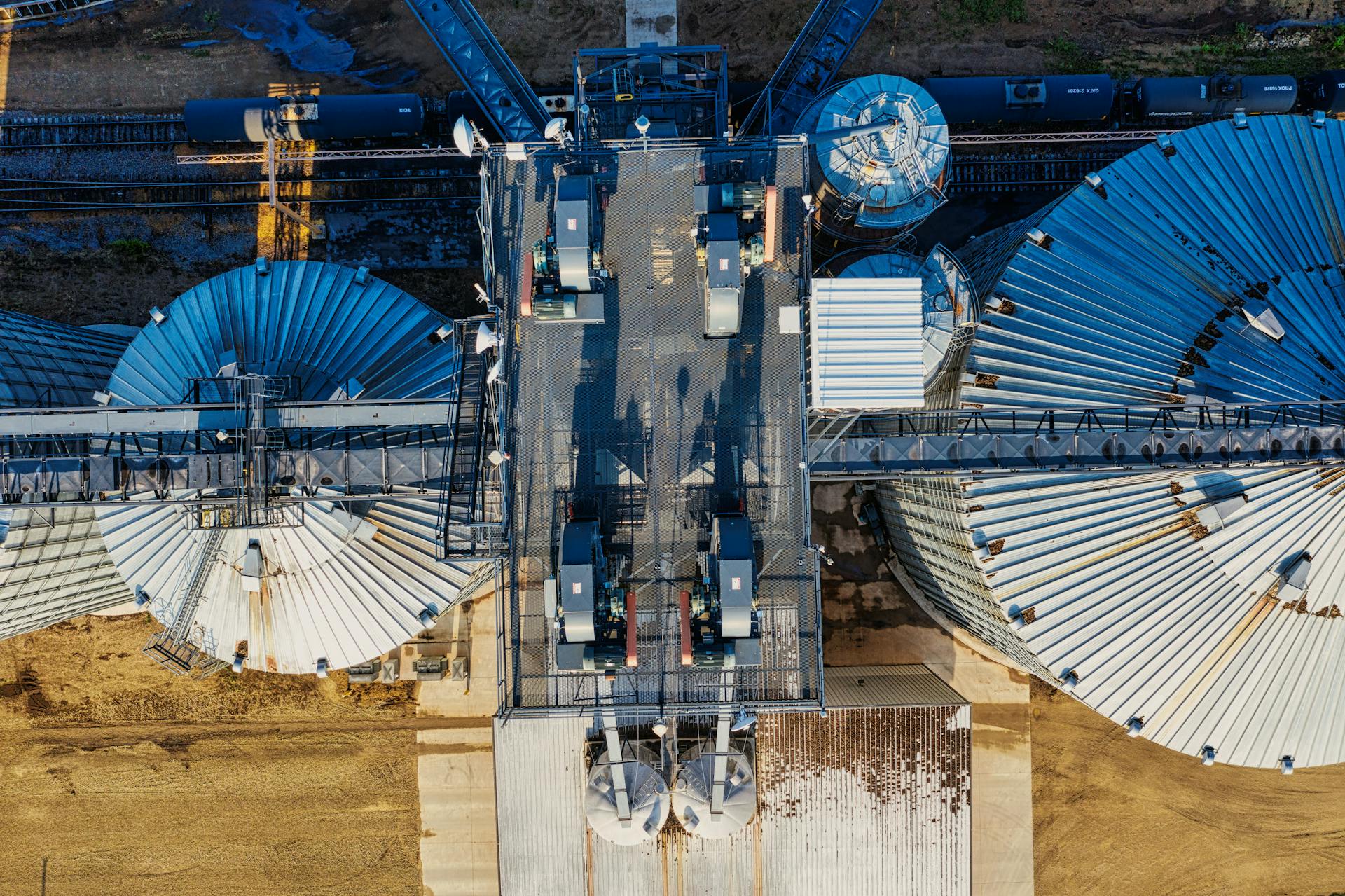
To set up the Cloudinary Upload API with NextJS, you'll first need to create a Cloudinary account and obtain an API key. This key will be used to authenticate API requests.
Cloudinary offers a free plan, making it accessible to developers of all levels. With the free plan, you can upload up to 100MB of files per day.
NextJS provides a built-in API route for handling file uploads, which you'll need to configure to work with Cloudinary. This involves installing the @cloudinary/server-sdk package and importing the Cloudinary library in your NextJS API route.
The Cloudinary Upload API allows you to upload files from your NextJS application to Cloudinary's servers, where they can be stored and managed.
Worth a look: Nextjs Proxy Api Route
Setting Up Cloudinary
To set up Cloudinary, you'll need to create an account on their website. This will give you access to your Cloudinary dashboard.
Cloudinary provides a unique API key for each account, which you'll use to authenticate your API requests. This key is a crucial part of the upload process, so be sure to store it securely.
To get started with the Cloudinary Upload API in Next.js, you'll need to install the cloudinary package using npm or yarn. This will allow you to use Cloudinary's API in your Next.js application.
Cloudinary supports various image and video formats, including JPEG, PNG, GIF, and MP4. You can also use their API to upload and manage other types of files.
In the next step, you'll need to configure your Next.js application to use the Cloudinary Upload API. This involves setting up your API keys and configuring the upload settings in your Cloudinary dashboard.
Creating API Route
To create an API route for uploading images to Cloudinary, you'll need to start by creating a helper function to upload files, which will return a promise for easier usage.
This function should be wrapped with a try-catch-finally block to handle any potential errors.
You'll then create a route handler to receive the image file or blob sent by the client, which can be done using the fetch API.
The route handler should also be wrapped with a try-catch block to catch any exceptions.
Next.js provides a formData method that helps retrieve the file easily, eliminating the need for Multer.
You can use this formData method to send the image file to Cloudinary for upload.
Explore further: Nextjs Image
Uploading Images
You can create an API route in Next.js to upload images to Cloudinary using a helper function that returns a promise. This function can be used to handle the file shared in the API call by the client.
To create the route, you'll need to wrap all the async code with try-catch-finally blocks to ensure proper error handling. You can use the Next.js .formData method to retrieve the file easily, eliminating the need for a library like Multer.
Here are the steps to follow:
- Create a form component in your Next.js application using the useState hook to manage the file and filename state.
- When the user selects a file, set the file state and display the filename in the label element.
- When the form is submitted, create a new FormData object and append the selected file and Cloudinary upload preset to it.
- Make a POST request to the Cloudinary API using the Axios library, passing in the form data as the request body.
Note: Replace your_cloud_name with your own Cloudinary cloud name and YOUR_UPLOAD_PRESET_NAME with your own preset name.
Uploading Images
You can upload images to Cloudinary using a helper function that returns a promise, making it easier to use. This function is a good starting point for creating a route handler to receive image files.
To create a route handler, you can use the Next.js .formData method, which helps retrieve the file easily. This method eliminates the need for a library like Multer.
For your interest: Routes in Nextjs
If you're coming from a Node.js background, you might be familiar with using Multer, but in this case, Next.js provides a more straightforward solution.
Here are some key points to consider when uploading images:
- You can use a custom return type for the helper function based on your use case.
- The route handler should be wrapped with a try-catch block to handle any errors.
- You can compress your image before uploading to Cloudinary if needed.
- The Next.js .formData method makes it easy to retrieve the file from the client-side code.
By following these steps and using the right tools, you can efficiently upload images to Cloudinary and get the image URL.
Uploading Images to Next.js via REST API
You can upload images to Next.js using Cloudinary's REST API, and it's actually quite straightforward.
To start, you'll need to create an upload preset in Cloudinary's dashboard. This preset will determine how your uploads are handled. For unsigned uploads, you'll want to select the Unsigned Signing Mode.
In your Next.js application, you'll need to create a form that allows users to select an image file. You can use the `useState` hook to manage the file and filename state.
When the form is submitted, you'll need to create a new FormData object and append the selected file and Cloudinary upload preset to it. Then, make a POST request to the Cloudinary API using the Axios library, passing in the form data as the request body.
Related reading: Nextjs save Data Pulled from Api Using State Context
Here are the key steps to follow:
- Create an upload preset in Cloudinary's dashboard with Unsigned Signing Mode
- Create a form in your Next.js application to select an image file
- Create a new FormData object and append the selected file and Cloudinary upload preset to it
- Make a POST request to the Cloudinary API using Axios, passing in the form data as the request body
By following these steps, you can upload images to Next.js using Cloudinary's REST API.
Image Upload Options
Cloudinary offers a free plan for basic users, which is a great option for those on a budget. You can use their REST API to upload images, and it's a fairly straightforward process.
You can use Cloudinary's widget for a drag-and-drop solution, or you can create a custom solution using their API. The API flow involves a Next.js Dropzone component calling a local Next.js Cloudinary endpoint that generates a signature and upload URL.
The signature is composed of the timestamp and Cloudinary's apiSecret, so you don't need to worry about sorting or parsing query parameters. However, if you want to include transformations, you'll need to add them to the signature string and sort them alphabetically, as per the Cloudinary documentation.
Cloudinary accepts both SHA1 and SHA256 links, but it's recommended to use SHA256 due to the security concerns with SHA1.
To apply transformations when uploading an image, you can use Cloudinary's Incoming Transformations feature. This allows you to resize and optimize images on the fly, without having to save the raw image.
Here are some common transformation options:
- Resize: You can resize images to a specific width or height.
- Format quality: You can set the format quality to be automatic, which will choose the best quality for the image.
When using signed uploads, you can also include transformations via the SDK. This allows you to apply transformations to your images without having to upload the raw image first.
Image Upload and Moderation with Next.js
To upload images to Cloudinary with Next.js, you can create an API route using the `fetch` API to send the image file from the client-side. This route handler should be wrapped in a try-catch block to handle any errors that may occur.
You can use the `formData` method in Next.js to retrieve the file easily, eliminating the need for a library like Multer. The client-side code should be wrapped in a try-catch-finally block to ensure that any resources are properly cleaned up.
In Next.js, you can also use the `Dropzone` component to call a local Next.js Cloudinary endpoint that generates and returns the necessary upload parameters. The endpoint should be wrapped in a try-catch block to handle any errors that may occur.
To upload the image to Cloudinary, you can use the `fetch` API to post the file to Cloudinary's REST API, along with the signature data from the Next.js local API. Upon a successful upload, you can garner the secure URL from the response and return it to the client-side.
Sources
- https://medium.com/@varchasvipandey/nextjs-and-cloudinary-app-router-integration-ad7fd2e0fdb2
- https://www.obytes.com/blog/cloudinary-in-nextjs
- https://medium.com/@brent_73702/uploading-images-to-cloudinary-for-next-js-via-their-rest-api-85f20efcccfd
- https://spacejelly.dev/posts/how-to-programmatically-upload-images-to-cloudinary-in-react-next-js
- https://cloudinary.com/blog/next-js-cloudinary-upload-transform-moderate-images
Featured Images: pexels.com