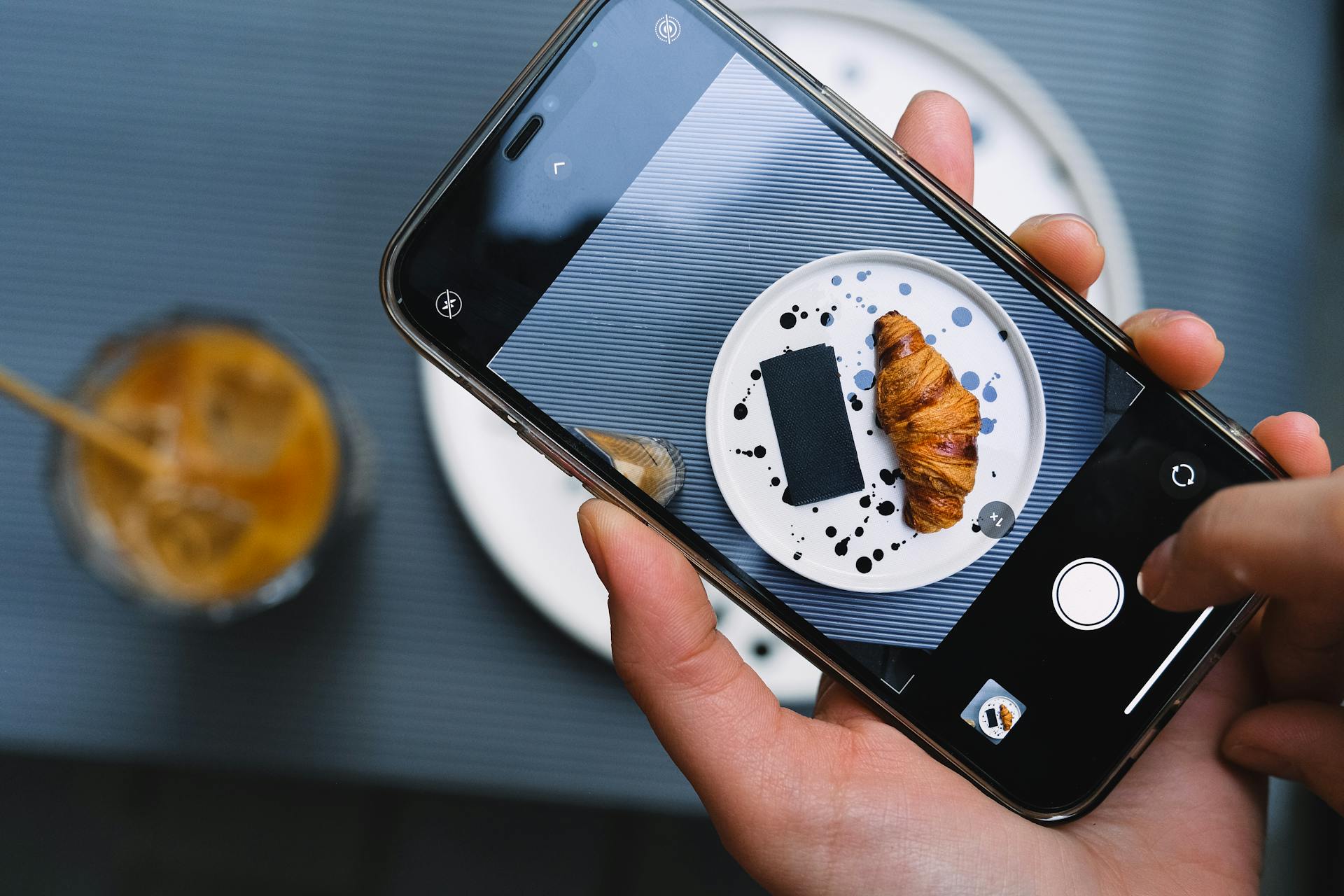
Next.js Image features make it easy to get started with image optimization. You can use the built-in Image component to optimize images for web use.
To use the Image component, you need to import it from the 'next/image' module. This is a simple step that sets the foundation for image optimization.
The Image component automatically optimizes images by compressing them and resizing them to the correct dimensions. This helps reduce the file size and improve page load times.
With the Image component, you can easily add the 'loading' attribute to specify how the image should be loaded. This attribute can be set to 'eager', 'lazy', or 'auto'.
On a similar theme: Why Use Nextjs
Benefits and Features
The Next.js Image component is a powerful tool for optimizing images in your app. It automatically optimizes images on-demand as they are requested by users.
One of the key benefits is automatic image optimization, which ensures images are served in the most efficient format based on the user's device and browser capabilities. This can significantly improve the loading times of your Next.js app.
Lazy loading is another feature that loads images only when they enter the viewport, improving loading times. Images are loaded lazily by default, making them load only when needed.
The Next.js Image component also ensures responsive images by adapting to different screen sizes, providing an optimal experience across devices. This is achieved by setting the layout attribute to responsive.
The component can display a placeholder until the image is fully loaded, improving the perceived performance of your Next.js app. This is a nice touch that makes your app feel more responsive and engaging.
Here are some of the key benefits of using the Next.js Image component:
- Automatic Image Optimization
- Lazy Loading
- Responsive Images
- Placeholder Support
Optimization Techniques
The Next.js Image component automatically optimizes images on-demand, as they are requested by users, serving images in the most efficient format based on the user's device and browser capabilities.
Compressing images can significantly reduce their file size without sacrificing quality. Tools like ImageOptim, TinyPNG, or online services can help with this.
Optimizing file size is crucial, as large images can slow down your website. Consider compressing your photographs to lower their file size without drastically losing quality.
Using the right format is also essential. Current picture formats like WebP offer superior compression than conventional formats like PNG and JPG. However, ensure fallbacks for browsers that do not support these formats.
To minimize the performance impact of background images, consider the following tips:
- Optimize File Size
- Choose the Right Format
- Use Appropriate Dimensions
- Implement Responsive Images
- Consider Using CSS3 Effects
The Next.js Image component serves smaller images to mobile devices by creating multiple sizes for the same image and serving the most appropriately sized image for the user's device.
You can use the sizes property to tell the browser what percentage of the viewport's width the image should fit. For example, to display images at full width on mobile screens, in a two-column layout on tablets, and in a three-column layout on desktops, you could set the sizes property as follows:
sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw"
The component automatically compresses images for peak performance, improving a website's overall performance. This helps save bandwidth by reducing image file sizes and the amount of data the browser needs to download during rendering.
Related reading: Next Js Performance Analyzer
Adding and Handling Images
Adding background images in Next.js can be accomplished through various methods, each with its own set of advantages and considerations.
You can use inline styles for quick customizations or external CSS/SCSS for a more traditional approach, or CSS-in-JS libraries for component-scoped styles.
To display an image using the Next.js Image Component, use its relative path in the component’s src property if your image is stored in the public directory.
Adding Background
Adding background images in Next.js is a breeze, and you can do it through inline styles for quick customizations or external CSS/SCSS for a more traditional approach. Either way, Next.js is flexible enough to accommodate your preferred workflow.
You can use inline styles to add background images quickly, but keep in mind that this method can get cluttered if you have multiple styles to apply. On the other hand, external CSS/SCSS allows for more organization and maintainability.
If you prefer a more component-scoped approach, you can use CSS-in-JS libraries, which are perfect for large-scale applications. These libraries allow you to write styles as JavaScript functions, making it easier to manage complex styles.
To make your background images responsive, you can employ several strategies, including using CSS media queries, flexible box layouts, or grid systems. These techniques ensure that your background images scale and reposition correctly across different screen sizes.
Here are some key considerations for making your background images responsive:
- Use relative units like percentages or ems to make your background images scale with the viewport.
- Use media queries to apply different styles based on screen size or device orientation.
- Consider using flexible box layouts or grid systems to make your background images adapt to different screen sizes.
Performance Considerations
Background images can significantly enhance the visual appeal of your Next.js app, but they can also have a notable impact on performance if not handled correctly.
Large file sizes can slow down your app, leading to longer load times and a poor user experience. Optimizing images is crucial to prevent this.
Efficient image loading can significantly improve the speed and responsiveness of your web pages, contributing to a better user experience and potentially higher SEO rankings.
Limitations When Using
The Next.js Image component is a powerful tool for optimizing images in your app, but it's not without its limitations. It doesn't support CSS background properties like background-size, background-position, or background-repeat, making it difficult to properly style background images.
Worth a look: Nextjs Set Background Image
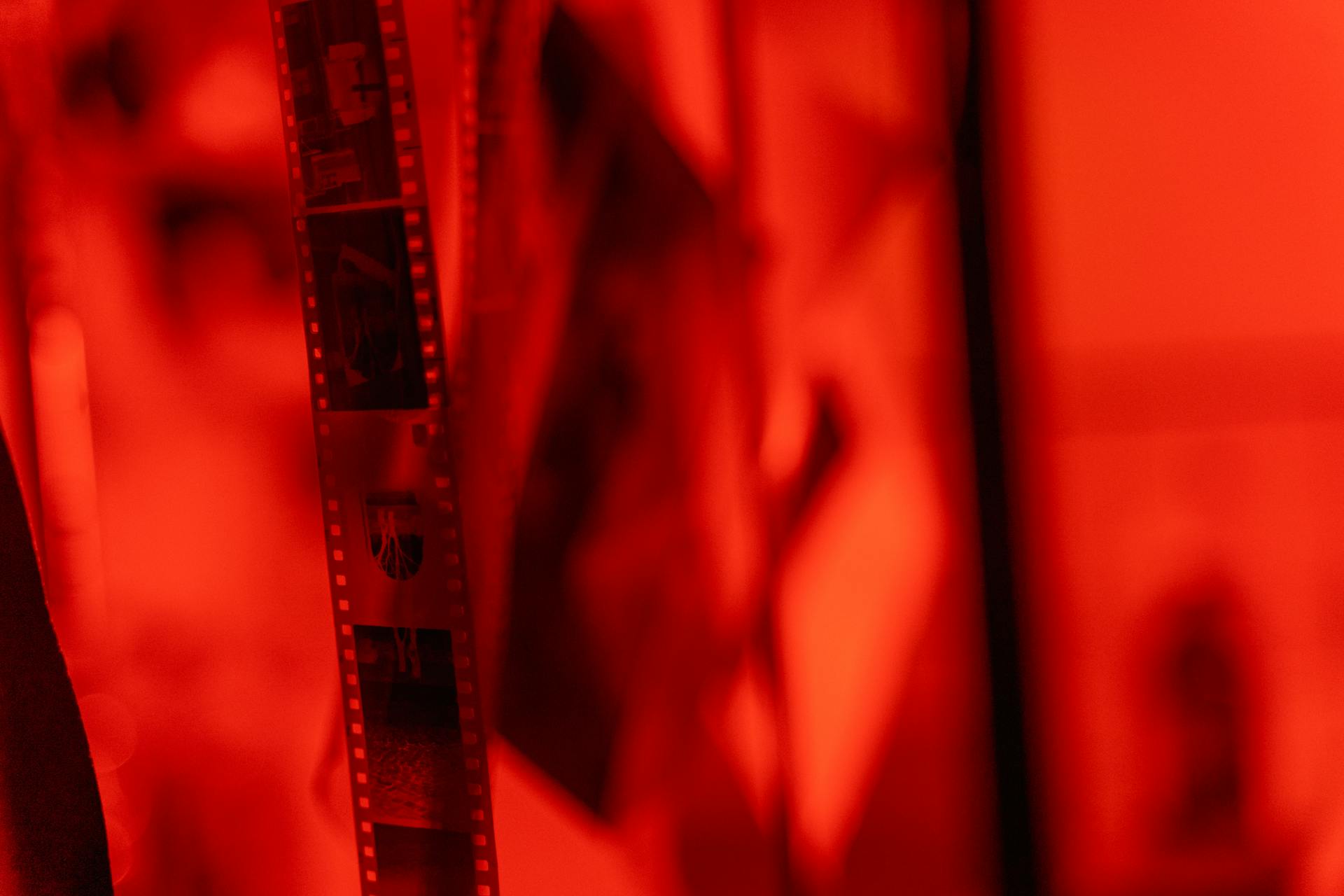
These limitations can make it challenging to achieve the desired layout and design for your app. For instance, background images often need to cover the entire background of an element or the whole page, which can be tricky to achieve with the Image component.
To overcome these limitations, developers often resort to traditional CSS techniques to implement background images. This can be a good solution, but it's essential to manually optimize background images to ensure high performance and fast loading times.
Here are some key limitations to keep in mind when using the Next.js Image component for background images:
- CSS Background Properties: The Image component does not support CSS background properties like background-size, background-position, or background-repeat.
- Layout Restrictions: Background images often need to cover the entire background of an element or the whole page, which can be challenging to achieve with the Image component.
- Complex Styling: When you need to overlay text or other elements on top of a background image, using the Image component can become complex.
Performance Considerations
Large file sizes can significantly slow down your app, leading to longer load times and a poor user experience.
Background images can have a notable impact on performance if not handled correctly.
Optimized images lead to fast-loading websites, which means users get the best experience possible.
Using next/image minimizes Cumulative Layout Shift (CLS) by ensuring images are loaded with appropriate width and height dimensions.
Efficient image loading can significantly improve the speed and responsiveness of your web pages, contributing to a better user experience and potentially higher SEO rankings.
Large file sizes, improper formats, and unoptimized delivery can slow down your app, leading to longer load times and a poor user experience.
Intriguing read: Next Js Build Time Slow
Speed Up Your Site with Contentful
The Next.js Image Component is a game-changer for improving page load times. It reduces cumulative layout shifts and provides responsive images that scale across devices and screen sizes.
Pairing it with Contentful takes it to the next level. Contentful's high-speed CDN ensures images and content are delivered quickly, even to mobile users.
Contentful can resize images and deliver them from its CDN. This means your site will load faster and provide a better experience for users.
You can integrate Contentful with Next.js to see the benefits for yourself. Sign up for a free Contentful account to get started.
Using your favorite tech stack, language, and framework with Contentful is a breeze. You can build a blog with Next.js, Tailwind, and Contentful, and even follow a tutorial to get started.
Responsive Images
Responsive images are crucial for ensuring your Next.js app looks great on all devices. Next.js offers several strategies for making background images scale and reposition correctly across different screen sizes.
To implement responsive images in a Next.js application, you can use the built-in next/image component. This component automatically optimizes images for different screen sizes and resolutions, ensuring your web pages load quickly and efficiently.
The next/image component can be used to display a single image or responsive images with different variations optimized for different screen sizes. You can use the fill property to ensure your Next.js Image Component fills the parent container, but using fill alone will cause your image to stretch, and does not preserve the original aspect ratio.
To make your image fully responsive, you need to set position: relative on its parent element, as well as either a width and height or an aspectRatio. The aspect ratio of the image is preserved using the aspectRatio property of the Next.js Image Component.
Here are some tips for optimizing background images:
- Optimize File Size: Compress your photographs to lower their file size without drastically losing quality.
- Choose the Right Format: Use current picture formats like WebP, which offer superior compression than conventional formats like PNG and JPG.
- Use Appropriate Dimensions: Don't use images that are larger than they need to be.
- Implement Responsive Images: Serve different image sizes for different device resolutions and screen sizes.
- Consider Using CSS3 Effects: Sometimes, you can achieve visually appealing backgrounds using CSS gradients, shapes, and patterns.
You can use the srcSet attribute to specify multiple image sources and implement art direction with Next.js. This allows for more creative control over how images are presented based on the user's screen size or other factors.
The Next.js Image Component automatically generates the srcSet for you, so you don't need to define it manually. However, you can control which image sizes are used in the srcSet by configuring your next.config.js file.
Lazy Loading and Preloading
Lazy loading is a technique that defers the loading of images until they are about to enter the viewport, reducing the page's initial load time and improving its overall performance. Next.js image component has built-in support for lazy loading, making it easy to implement.
Lazy loading can be turned off for a particular image by setting the loading attribute to "eager". This means the image will load immediately, rather than waiting until it enters the viewport.
The next/image component automatically uses lazy loading, which means images are only loaded as they approach the viewport. This reduces initial page load time and saves bandwidth.
Preloading images above the fold is also a crucial technique for improving performance. By setting the priority property on critical images, you can ensure they are preloaded and ready to display as soon as the page loads.
Here are some benefits of using lazy loading and preloading with Next.js image component:
- Improved initial page load time
- Reduced bandwidth usage
- Improved overall performance
- Better Core Web Vitals
Data from a community survey performed by the Next.js team showed that 70% of respondents use the image component in production, and they saw improved Core Web Vitals.
Implementing Art Direction with Multiple Sources
Implementing art direction with multiple sources is a key feature of the Next.js image component. This allows you to serve different images based on the device or context.
You can use the Next.js image component to implement art direction by specifying multiple images as sources. For example, you can use the `src` prop to specify the image source for different screen sizes or resolutions.
Here are some ways to implement art direction with multiple sources:
The Next.js image component automatically optimizes images for different screen sizes and resolutions, ensuring your web pages load quickly and efficiently. By implementing art direction with multiple sources, you can improve the user experience and enhance the visual appeal of your web pages.
Installation and Setup
To get started with Next.js image optimization, you'll need to install Next.js and its project dependencies. This can be done with a simple command that scaffolds a new project with all the necessary files and configurations.
Next.js comes with the Image Optimization API, so you can start serving optimized images immediately without additional packages. This is a big time-saver and a key benefit of using Next.js for image optimization.
To begin working on your application, navigate to your project directory after the installation is complete.
Installing Project Dependencies
To install project dependencies, start by creating a new Next.js application in your project directory using a simple command that scaffolds a new project with all the necessary files and configurations.
This command will set up your project with everything you need to get started.
Your Application
Your application's security is crucial, and setting up a domain configuration in your next.config.js file is essential to protect it from malicious users.
To configure your Next.js application, you need to specify a domain's configuration, which allows you to define trusted sources of images. This is particularly important when serving images from remote servers.
With the domain configuration in place, your application will only serve images from the specified domains, preventing unmatched hostnames from compromising your application.
Readers also liked: Speed up a Next Js Application Fetching Data
Boosts Productivity
Using modern image formats like WebP or AVIF can significantly boost productivity by offering better compression and quality than traditional formats like JPEG or PNG.
With Next.js, you can automatically serve images in these formats using the next/image component, making it easier to optimize your images without sacrificing quality.
Implementing lazy loading with the next/image component reduces initial page load time and saves bandwidth, allowing your users to start interacting with your site sooner.
Prioritizing critical images using the priority attribute for above-the-fold images can improve the Largest Contentful Paint (LCP) metric, which is essential for performance.
By utilizing caching strategies, you can serve images quickly to repeat visitors and reduce the load on your server.
Here are some specific benefits of using the next/image component:
- Images will load faster, even with poor internet connections.
- The alt attribute is now required, enforcing accessibility best practices for images.
- The component ships less client-side JavaScript, reducing layout shifts and providing faster loading.
- It supports native browser lazy loading, leading to better performance.
Other Features and Tools
The Next.js image component is quite impressive, and there's more to it than just its benefits and features. It provides a built-in image component, next/image.
One of the standout features of next/image is its ability to handle image optimization out of the box. This means you don't need to worry about manually compressing images or configuring complex optimization tools.
Next/image also offers a simple and intuitive API that makes it easy to use and integrate into your application. This API is designed to be flexible and adaptable to different use cases.
Worth a look: Next Js Api Routes
The component's API allows you to specify a wide range of options, including the image's src, alt text, and layout. This level of customization gives you the flexibility to tailor the component to your specific needs.
By using next/image, you can significantly improve the performance and user experience of your application. This is especially important for applications with high traffic or complex layouts.
Sources
- https://www.dhiwise.com/post/how-to-add-a-background-image-in-nextjs-a-comprehensive-guide
- https://nextjs.org/docs/pages/api-reference/components/image
- https://www.dhiwise.com/post/how-to-implement-next.js-responsive-image-features
- https://www.contentful.com/blog/nextjs-image-component/
- https://prismic.io/blog/nextjs-image-component-optimization
Featured Images: pexels.com