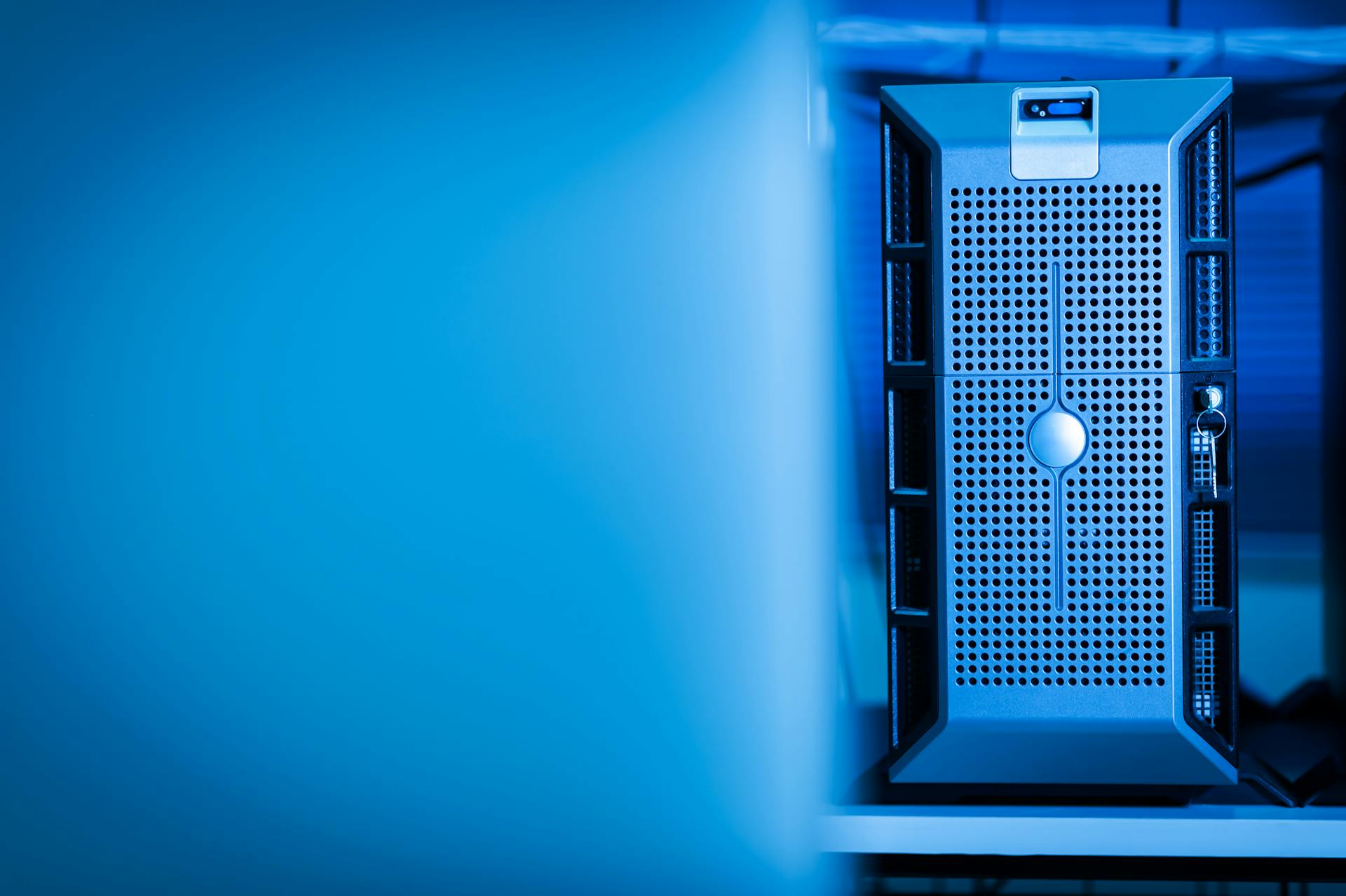
To start using AWS S3 with Golang, you'll need to install the AWS SDK for Go, which can be done using the go get command.
This will allow you to interact with S3 from your Golang application.
The AWS SDK for Go provides a simple and efficient way to access S3, making it easy to perform common operations such as listing objects and uploading files.
To get started, you'll need to create an S3 client instance, which can be done using the S3.New function.
Intriguing read: Aws S3 Sdk Nodejs
Setting Up AWS Golang S3
To get started with AWS Golang S3, you'll need to have an AWS account.
You should already have an IAM user with full s3 bucket permission, which is a crucial step in accessing S3 resources.
To set up your AWS Golang S3 environment, ensure you have the following prerequisites met:
- You should already have an AWS Account.
- You should have an IAM user with full s3 bucket permission.
Prerequisites
Before you start setting up AWS Golang S3, you need to have a few things in place. You should already have an AWS Account, which is a requirement to access AWS services.
To access S3, you'll need an IAM user with full s3 bucket permission. This will give you the necessary permissions to create and manage S3 buckets.
Here are the specific prerequisites you need to meet:
- You should already have an AWS Account.
- You should have an IAM user with full s3 bucket permission.
Constants
In AWS Golang S3, you'll often work with constants that control the behavior of your uploads and downloads.
The DefaultDownloadConcurrency is the default number of goroutines to spin up when using Download(). This setting is crucial for optimizing performance.
You can also adjust the DefaultDownloadPartSize, which is the default range of bytes to get at a time when using Download(). A larger part size can improve performance, but may also increase memory usage.
For uploads, the DefaultUploadConcurrency is the default number of goroutines to spin up when using Upload(). This setting can significantly impact the speed of your uploads.
The DefaultUploadPartSize is the default part size to buffer chunks of a payload into. This setting can affect the balance between upload speed and memory usage.
Here's an interesting read: Download File from Aws S3
There's also a limit to the number of parts in a multi-part upload on Amazon S3: MaxUploadParts. Be sure to stay within this limit to avoid errors.
The MinUploadPartSize is the minimum allowed part size when uploading a part to Amazon S3. This setting ensures that your uploads meet the minimum requirements for Amazon S3.
Working with S3
The Uploader function in S3 intelligently buffers large files into smaller chunks and sends them in parallel across multiple goroutines.
You can configure the buffer size and concurrency through the Uploader's parameters, giving you fine-grained control over the upload process.
The Uploader function also allows you to pass in request options that will be applied to all API operations made with this uploader, using the WithUploaderRequestOptions helper function.
A different take: Invoke Aws Lambda Function Sam with S3 Trigger
GetBucketRegion
GetBucketRegion is a useful function that allows you to get the region for a bucket.
It attempts to get the region using the regionHint to determine which AWS partition to perform the query on. The request won't be signed or use your AWS credentials.
Explore further: Aws S3 Cross Region Replication
NewDownloader
Working with S3 requires a flexible and efficient way to download objects, and that's where NewDownloader comes in. It creates a new Downloader instance to download objects from S3 in concurrent chunks.
You can customize the downloader behavior by passing in additional functional options. This allows you to tailor the downloader to your specific needs.
To use NewDownloader, you need a client.ConfigProvider to create an S3 service client. This is satisfied by a session.Session, which also happens to be a ConfigProvider.
NewDownloader requires a client.ConfigProvider to function properly.
Object
Working with S3 involves understanding how to upload and manage objects.
You can use the UploadObject method to return the BatchUploadObject at the current batched index, which is a feature added in inv1.9.0.
To upload objects, you'll need to use an iterator, specifically the UploadObjectsIterator.
This iterator allows you to upload objects in batches, making it more efficient than uploading individual objects.
If this caught your attention, see: Aws S3 Listobjects
User
As an S3 user, you're in control of how your uploads are handled.
You can configure the buffer size and concurrency through the Uploader's parameters, allowing you to fine-tune the upload process for your specific needs.
The Uploader instance's parameters can be modified to change the buffer size and concurrency, giving you flexibility in how your uploads are processed.
You can pass in request options to be applied to all API operations made with a specific uploader using the WithUploaderRequestOptions helper function.
These options will not impact the original Uploader instance, so you can experiment and modify them without worrying about affecting the overall uploader settings.
Intriguing read: Aws S3 Sync Specific Files
S3 Operations
S3 Operations can be managed using environment variables stored in applications.yml, which is a common practice in Go development. This approach allows for easy configuration switching between different environments.
To download files from S3, you'll need to create a session and a downloader instance. Then, open the file you want to download and save its instance in a variable. Finally, call the download function of the downloader by providing the bucket name and file name.
Here's a step-by-step guide to listing S3 buckets:
- Create a session.
- Create a new S3 Client.
- Get the list of the buckets.
- Print Names of buckets.
You can also use an AWSConfig struct to manage configs for environment variables related to AWS, which is useful for fetching S3 operations.
Listing Objects
You can list out objects present on S3 using ListObjectsV2. This will list down all the objects in specific bucket.
To manage configs for environment variables, you can use applications.yml. This is where you'll store information related to AWS required for fetching S3 operations.
Listing objects on S3 is a straightforward process that can be accomplished using ListObjectsV2. This is a key AWS service that helps you manage your S3 bucket contents.
A unique perspective: Aws S3 Listobjectsv2
DeleteObject
DeleteObject is a method that can be used to delete objects from a batched index.
In version 1.9.0, the DeleteObject method was added to the DeleteObjectsIterator function.
This method returns the BatchDeleteObject at the current batched index.
You can use the DeleteObject method to delete objects in a specific order, which can be useful for managing large datasets.
The DeleteObject method is only available in version 1.9.0 and later.
For more insights, see: Aws S3 Sync --delete
Coding Out Operations
Coding out operations on Amazon S3 can be a breeze with the right tools and knowledge. You can manage configurations for environment variables using applications.yml, which is a great way to keep your code organized.
To perform S3 operations, you'll need to create an AWSConfig struct that contains all the necessary information. This struct will serve as the foundation for your S3 operations.
One of the most powerful tools for S3 operations is the Downloader. With the Downloader, you can download objects from S3 in a concurrent manner, which is perfect for large files. You can also use the DownloadWithContext method to add deadlining, timeouts, and other features to your downloads.
Here's a step-by-step guide to downloading files using the Downloader:
- Create a session.
- Create a downloader instance.
- Open the file that you need to download and save its instance in a variable.
- Call the download function of the downloader by providing the bucket name and file name.
You can also use the UploadWithContext method to upload objects to S3, which allows you to configure the buffer size and concurrency of the upload process.
Batch operations are another important feature of S3 operations. With the BatchDelete and BatchUploadIterator interfaces, you can perform batch deletions and uploads of objects in a single operation.
Here's an example of how to use the BatchDelete interface:
- Create a batch delete object.
- Use the DeleteObject method to delete objects from the batch.
These are just a few examples of how you can code out operations on Amazon S3. With the right tools and knowledge, you can perform a wide range of S3 operations with ease.
You might enjoy: Aws S3 Batch Operations
Type Output
Type Output is an essential part of S3 Operations, as it provides a response from various calls, including the Upload() call.
The UploadOutput type represents a response from the Upload() call, giving you valuable information about the upload process.
In S3, UploadOutput is a crucial type that helps you understand the outcome of an upload operation.
Release
In release notes, you'll often find a mention of new features and improvements. For S3 Operations, a notable addition is the Err function in the UploadObjectsIterator, which was added in version 1.9.0.
This Err function will return an error, but don't worry if you're not sure what that means - it's just a way to satisfy a specific interface, and it will only return nil.
Sources
- https://www.mohitkhare.com/blog/aws-s3-golang/
- https://blog.stackademic.com/aws-s3-and-golang-ebc32887aee8
- https://pkg.go.dev/github.com/aws/aws-sdk-go/service/s3/s3manager
- https://towardsaws.com/end-to-end-devops-for-a-golang-web-app-docker-eks-aws-ci-cd-f7793e230ae9
- https://medium.com/geekculture/go-cafe-creating-and-adding-files-to-aws-s3-using-golang-b92eaa5f2081
Featured Images: pexels.com