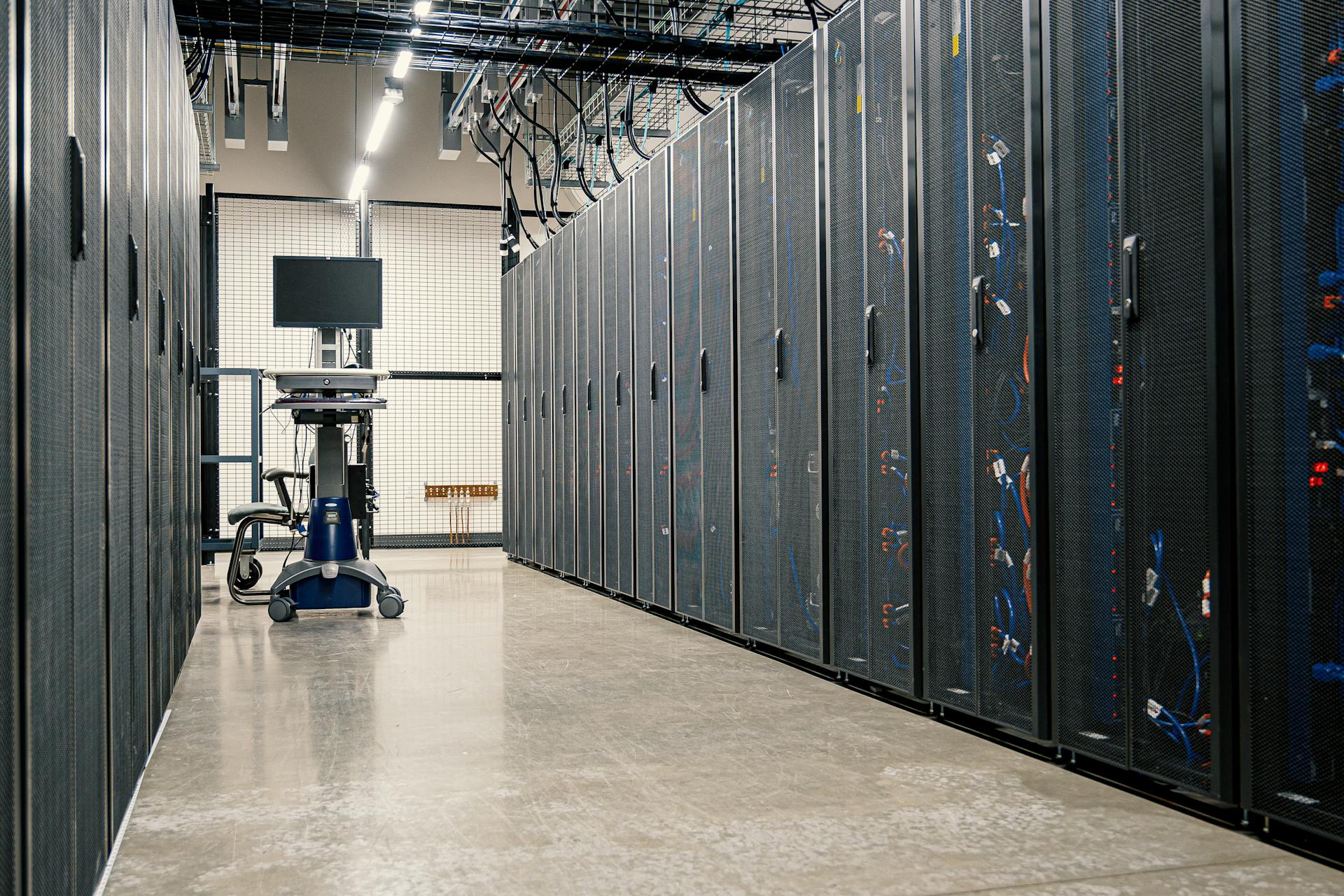
The AWS SDK for Node.js is a powerful tool for interacting with Amazon S3, allowing you to easily manage your cloud storage needs.
To get started, you need to install the AWS SDK for Node.js using npm or yarn.
You can then import the SDK and use it to create an S3 client, which is the main interface for interacting with S3.
This client allows you to perform a wide range of operations, including listing buckets, uploading files, and deleting objects.
See what others are reading: Next Js Software Staging Environment Aws Amplify Gen 2
Prerequisites
To get started with the AWS SDK for Node S3, you'll need a few things set up. First, you'll need an AWS account with access keys.
To use the AWS SDK for Node S3, you'll also need NodeJS and NPM installed on your local machine.
You should have a basic understanding of JavaScript, NodeJS, and AWS S3.
Here are the specific prerequisites:
- An AWS account with access keys.
- NodeJS and NPM installed on your local machine.
- A basic understanding of JavaScript, NodeJS, and AWS S3.
Setup and Installation
To get started with AWS SDK for NodeJS and S3, you need to set up an S3 bucket in your AWS account. Create an S3 bucket by logging in to your AWS account and navigating to the S3 console, then click on the “Create bucket” button.
Discover more: Create S3 Bucket Aws
An S3 bucket is a container for storing objects, such as files, in the Amazon S3 cloud. To create an S3 bucket, you need to enter a unique name for your bucket, select a region, and choose the default settings for versioning, logging, and permissions.
To interact with AWS services using NodeJS, you need to install the AWS SDK for NodeJS. Run the following command in your terminal: "To install the AWS SDK for NodeJS, run the following command in your terminal:"
The AWS SDK provides a set of JavaScript libraries for accessing and managing AWS services, including Amazon S3. To install the AWS SDK for NodeJS, you can also use the command "Install AWS-SDK" and run it in your project terminal.
The AWS SDK helps to reduce the coding by providing us with JavaScript objects for AWS services. It's essential to install the AWS SDK for NodeJS to form the connection with S3.
Here are the steps to create an S3 bucket:
- Log in to your AWS account and navigate to the S3 console.
- Click on the “Create bucket” button.
- Enter a unique name for your bucket, select a region, and choose the default settings for versioning, logging, and permissions.
- Click on the “Create bucket” button to create the bucket.
Uploading Files
You can upload a file to S3 using NodeJS and the AWS SDK. To do this, you need to use the S3 API to create a new object in your S3 bucket and upload the file data to it.
The AWS SDK for JavaScript provides a simple function for uploading a file to Amazon S3. The function takes two parameters: the local file to be uploaded and the destination S3 bucket.
Here are the best practices for uploading files to Amazon S3:
- Use Server-Side Encryption (SSE) to encrypt your files at rest, providing an additional layer of security.
- Set Content-Type explicitly for your files to ensure proper handling by browsers and applications.
- Use Transfer Acceleration for faster file uploads.
You can upload a file to an AWS S3 bucket by running a command like `node upload.js webiny-s3-bucket-testing index.txt`. This will upload the file `index.txt` to the S3 bucket `webiny-s3-bucket-testing`.
Here are the parameters you need to configure the upload:
- `client`: The destination of the file, which in this case is the S3 bucket.
- `params`: An object containing the name of the S3 bucket, the file name, the access control list, and the file content.
- `queueSize`: The number of parts to be processed simultaneously, which defaults to 4.
- `partSize`: The size of each part that is processed, which defaults to 5MB.
If the upload is successful, the promise resolves with an object containing information about the uploaded file, including the file name, location, and metadata.
Here's an interesting read: Aws S3 Upload File App Typescript
Troubleshooting
If you're experiencing issues with your AWS SDK Node.js S3 integration, check if the IAM role or user has the necessary permissions to access the S3 bucket.
Make sure the AWS region is correctly specified in your code to avoid errors.
Incorrect region settings can cause issues with S3 bucket access, so double-check your code.
Verify that the S3 bucket is in the same region as your AWS account to ensure seamless integration.
If you're using a VPC, ensure that the S3 bucket is configured to allow access from the VPC.
Check the AWS SDK Node.js version you're using, as some versions may have known issues with S3 integration.
Outdated SDK versions can cause problems, so consider updating to the latest version.
If you're still experiencing issues, try checking the AWS SDK Node.js documentation for troubleshooting guides specific to your scenario.
A fresh viewpoint: Aws S3 Vpc Endpoint
Nodejs Setup
To set up Nodejs for AWS SDK, start by creating an S3 bucket in your AWS account. This is done by logging in to your AWS account, navigating to the S3 console, and clicking on the “Create bucket” button.
To interact with AWS services using Nodejs, you need to install the AWS SDK for Nodejs. This can be done by running the command in your terminal: install the AWS SDK for Nodejs.
Suggestion: Aws Cross Account S3 Access
You can install the @aws-sdk package using npm or yarn, and then import it in your Nodejs code to use its functionality. The AWS SDK provides a set of JavaScript libraries for accessing and managing AWS services, including Amazon S3.
To confirm your AWS SDK setup, you can check the version of the SDK you have installed. The latest version (v3.x) of AWS SDK is recommended for use.
Worth a look: Aws Sdk S3
Install Nodejs
To get started with Nodejs, you'll need to install the AWS SDK for Nodejs, which provides a set of JavaScript libraries for accessing and managing AWS services.
You can install the AWS SDK for Nodejs by running the following command in your terminal: `To install the AWS SDK for Nodejs, run the following command in your terminal:`. This will give you access to the tools you need to interact with AWS services.
If you already have @aws-sdk installed and configured, you can skip this step. But if you're starting from scratch, this is where you begin.
A unique perspective: Aws Cli for S3
Nodejs Set Up
To set up Nodejs, start by setting up an S3 bucket in your AWS account, which is a container for storing objects in the Amazon S3 cloud. An S3 bucket is created by logging in to your AWS account, navigating to the S3 console, and clicking on the “Create bucket” button.
To interact with AWS services using Nodejs, you need to install the AWS SDK for Nodejs. The AWS SDK provides a set of JavaScript libraries for accessing and managing AWS services, including Amazon S3. To install the AWS SDK for Nodejs, run the following command in your terminal:
```
npm install @aws-sdk/client-s3
```
You can use the latest version (v3.x) of AWS SDK, which can be installed by running the following command:
```
npm install @aws-sdk/client-s3@latest
```
If you already have @aws-sdk installed and configured, you can skip to the next section. To set up AWS credentials, you need to provide your access keys to the AWS SDK. You can obtain your access keys from the AWS console by following these steps:
- Log in to your AWS account and navigate to the IAM (Identity and Access Management) console.
- Click on the “Users” tab and select your IAM user.
- Click on the “Security credentials” tab and expand the “Access keys” section.
- Click on the “Create access key” button to generate a new access key pair.
- Download the access key file and keep it in a safe place.
Once you have obtained your access keys, you can set them up in your Nodejs code using the AWS SDK. The easiest way to do this is to set them up as environment variables, like this:
```
AWS_ACCESS_KEY_ID=your_access_key_id
AWS_SECRET_ACCESS_KEY=your_secret_access_key
```
By following these steps, you can set up Nodejs and start using AWS services.
Add an Argument
Adding an argument to a function can be a simple yet effective way to make it more flexible and reusable. You might need to pass additional information to a function, like a bucket argument in a service with multiple buckets.
Having default values for arguments can also be helpful, especially if you only plan to use one bucket. This way, you can still add the bucket argument if you switch from the default.
In some cases, you might need to add a second argument to a function, like the deleteFolder function which takes a second bucket argument. This allows you to work with multiple buckets in your service.
You might enjoy: Aws S3 Copy Multiple Files
S3 Operations
S3 Operations are a crucial part of working with AWS SDK in Node.js, and understanding how to perform them is essential for any developer.
You'll need to configure the SDK and create an AWS S3 service object to start listing objects in an S3 bucket. This is a repetitive task that you'll encounter often when working with S3.
Listing Objects in an S3 Bucket is a common operation that involves providing the bucket name from which you want to read the objects. The result will be a list of objects (files) or a failure message.
To list objects in an S3 bucket, you'll need to use a script like the one in the listObjects.js file. This script will take the bucket name as input and return a list of objects or a failure message.
You can use the AWS S3 service object to list objects in an S3 bucket, but you'll need to configure the SDK first. This involves creating an AWS S3 service object, which can be a bit repetitive but is necessary for most S3 operations.
Check this out: Aws S3 List_objects
Access and Security
Double-check your AWS credentials if you encounter an "InvalidAccessKeyId or SignatureDoesNotMatch" error, as this usually indicates an issue with your access key ID and secret access key.
To resolve an "Access Denied" error, verify that your IAM user has the necessary permissions to upload files to the specified S3 bucket. This ensures that your user account has the correct permissions to perform the desired action.
Set Up Credentials
To access your AWS account programmatically, you need to provide your access keys to the AWS SDK. You can obtain your access keys from the AWS console by following these steps:
- Log in to your AWS account and navigate to the IAM (Identity and Access Management) console.
- Click on the “Users” tab and select your IAM user.
- Click on the “Security credentials” tab and expand the “Access keys” section.
- Click on the “Create access key” button to generate a new access key pair.
- Download the access key file and keep it in a safe place.
Once you have obtained your access keys, you can set them up in your NodeJS code using the AWS SDK. The easiest way to do this is to set them up as environment variables.
Access Denied
Access Denied issues can be frustrating, but they're often easily solvable. One common cause of Access Denied is when your IAM user doesn't have the necessary permissions to access a specific S3 bucket. This can happen when you're trying to upload files to a bucket and you get an "Error 3: Access Denied" message.
To fix this, you need to verify that your IAM user has the correct permissions. This is a simple step that can save you a lot of time and hassle in the long run.
Discover more: Aws Access Denied S3
Access Points
To access the data you store on S3, you need an S3 Access Point, which is an endpoint attached to a bucket for performing S3 object operations.
Each access point has distinct permissions and network controls that S3 applies for any request made through it.
Access points are used to perform operations on objects, but not on buckets.
You can use access points to manage data access with S3, and to learn more about it, you can check out the source for managing data access with S3 access points.
Discover more: Aws S3 Access Point
Sources
- https://saturncloud.io/blog/how-to-upload-a-file-to-amazon-s3-with-nodejs/
- https://www.codemzy.com/blog/delete-s3-folder-nodejs
- https://www.webiny.com/blog/get-started-with-aws-s3/
- https://www.freecodecamp.org/news/how-to-upload-files-to-aws-s3-with-node/
- https://blog.emumba.com/amazon-s3-using-node-js-271b365a24b4
Featured Images: pexels.com