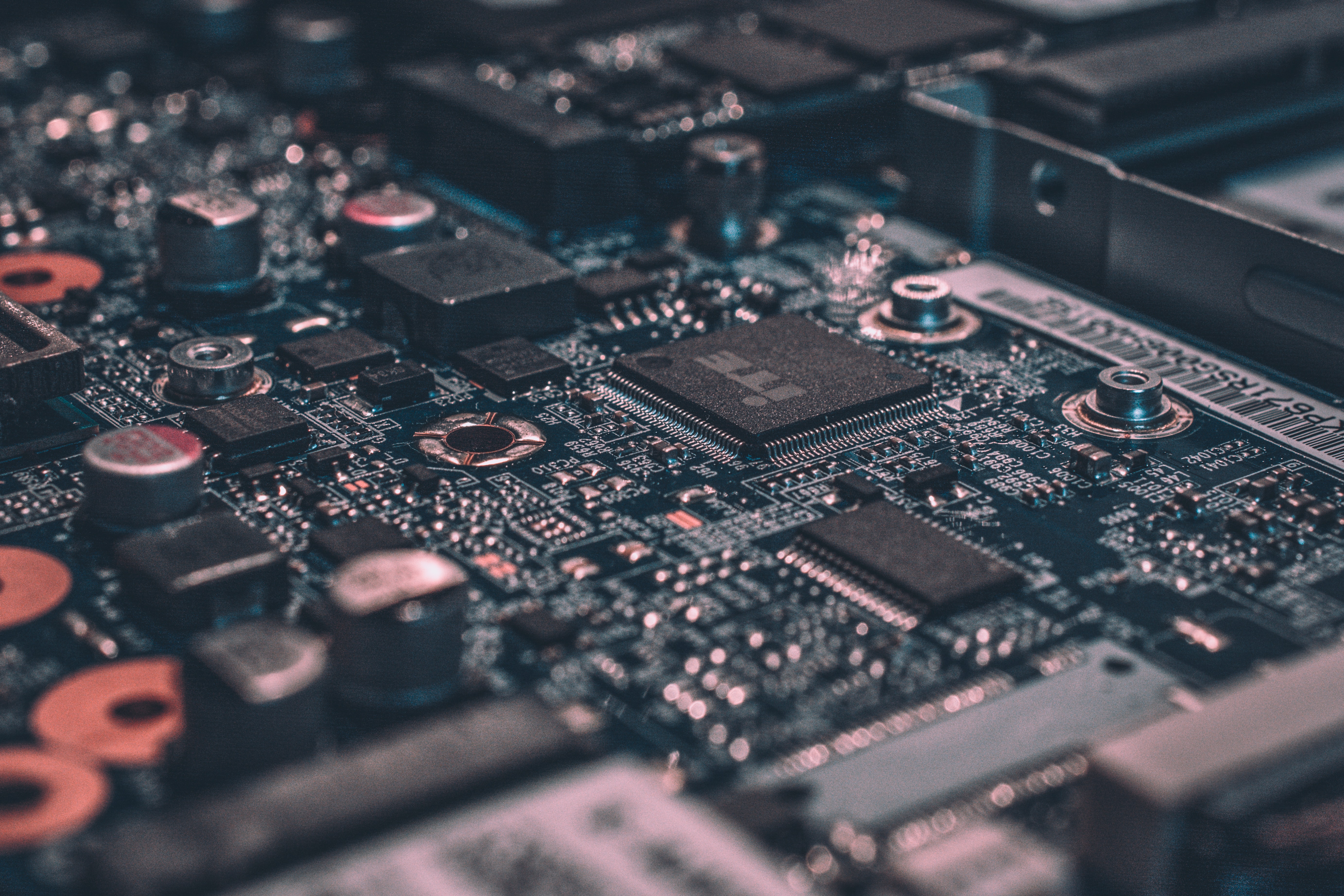
When creating a form in PHP, you will often need to specify what kind of data the form will accept and how to process it. In this tutorial, we will look at how to store data collected from a form in a MySQL database using PHP.
When a user submits a form, the data is sent to the server where PHP can access it. In order to store the data in a database, we first need to create a database and a table to store the data. For this tutorial, we will assume that you have access to a MySQL database server and know how to create databases and tables.
We will also assume that you have a basic understanding of HTML forms and how they work. If you need a refresher on HTML forms, you can check out our tutorial on creating a basic HTML form.
Now that we have our database and table set up, we can start writing the PHP code to store our form data. The first thing we need to do is connect to our database server. We do this using the mysql_connect() function. This function takes four parameters: the server name, the username, the password, and the database name.
Once we have connected to the database server, we need to select the database that we want to use. We do this using the mysql_select_db() function. This function takes two parameters: the connection to the database server and the database name.
Now that we are connected to the database and have selected the correct database, we can start writing the code to insert our form data into the database. We will be using the MySQL INSERT INTO statement to do this.
The INSERT INTO statement takes two parameters: the table name and the values to insert. The table name is the name of the table that we want to insert the data into. The values to insert are the data that we want to insert into the table.
In our case, we want to insert the data from our form into the table. The data from our form is contained in the $_POST array. This array contains all of the data that was sent from the form.
To insert the data from our form into the database, we need to loop through the $_POST array. For each item in the array, we need to insert it into the database.
We do this using the mysql_query() function. This function takes two parameters: the connection to
Worth a look: Cloud Data Store
How do I store a radio button value in a database using PHP?
When creating a web application that requires the storage of data, it is important to consider how that data will be stored. In some cases, data can be stored in a database, such as MySQL. In other cases, data can be stored in a file, such as a text file or an XML file.
In the case of storing a radio button value in a database, there are two options. The first option is to store the value as a string in the database. The second option is to store the value as an integer in the database.
When storing the value as a string, the value is stored in the database as-is. This means that if the value of the radio button is "on", the string "on" will be stored in the database. If the value of the radio button is "off", the string "off" will be stored in the database.
When storing the value as an integer, the value is converted to an integer before it is stored in the database. This means that if the value of the radio button is "on", the integer 1 will be stored in the database. If the value of the radio button is "off", the integer 0 will be stored in the database.
The decision of how to store a radio button value in a database should be based on the needs of the application. If the application needs to know whether the radio button was selected or not, it is best to store the value as an integer. If the application only needs to know the string value of the radio button, it is best to store the value as a string.
Suggestion: When Storing Products It Is Important to
What is the best way to store a radio button value in a database using PHP?
Storing a radio button value in a database using PHP is best done using a hidden form field. This field can be populated with the value of the radio button when the form is submitted. The value can then be inserted into the database.
A radio button indicates a choice of two or more mutually exclusive options. The user selects one option by clicking the radio button. Only one option can be selected at a time. If the user clicks a different radio button, the previous selection is deselected.
To submit a form that contains a radio button, the user must click the submit button. The value of the radio button is submitted to the server when the form is submitted.
When the form is submitted, the value of the radio button is passed to the PHP script. The script can then insert the value into the database.
The value of the radio button is stored in the database as an integer. The value is typically 1 for the first radio button, 2 for the second radio button, and so on.
The hidden form field is the best way to store a radio button value in a database using PHP. The hidden form field can be populated with the value of the radio button when the form is submitted. The value can then be inserted into the database.
For more insights, see: When I Young I Listen to the Radio?
How can I store a radio button value in a database using PHP?
Radio buttons are a type of input element that allows the user to select one option from a set of options. The options are typically presented as a set of checkboxes, with each option being represented by a checkbox. When the user selects an option, the checkbox for that option is checked.
When a radio button is selected, its value is stored in the database. To store the value of a radio button in a database, you need to use the name attribute of the radio button. The name attribute is used to identify the radio button so that when it is submitted, its value can be retrieved from the database.
To store the value of a radio button in a database, you first need to create a database table that will hold the values of the radio buttons. The table should have a column for the radio button value, as well as a column for the name of the radio button.
Next, you need to create a PHP script that will insert the radio button value into the database table. The script should first get the value of the radio button from the $_POST array. The value of the radio button is stored in the value attribute of the radio button.
Next, the script should get the name of the radio button from the $_POST array. The name of the radio button is stored in the name attribute of the radio button.
Finally, the script should insert the radio button value and name into the database table.
After the script has inserted the radio button value into the database, you can then retrieve the value from the database and display it on a web page. To do this, you first need to create a PHP script that will query the database for the radio button value.
The script should then display the radio button value on the web page.
For another approach, see: Azure Key Value Store
How do I store multiple radio button values in a database using PHP?
There are multiple ways to store radio button values in a database using PHP. One way is to use the implode() function. This function returns a string containing all the values from an array. In this example, we have an array of radio button values. The implode() function will return a string containing all the values, separated by commas.
Another way to store radio button values in a database using PHP is to use the serialize() function. This function returns a string containing all the information from an array. In this example, we have an array of radio button values. The serialize() function will return a string containing all the information, including the indexes and keys.
Lastly, you can store radio button values in a database using PHP by creating your own function. In this example, we create a function called store_radio_values(). This function will take an array of radio button values and store them in a database. We use the mysqli_query() function to execute our SQL query.
Whichever method you choose to use, storing radio button values in a database using PHP is a simple task.
Expand your knowledge: Why Are Values Important in a Company
What is the best way to store multiple radio button values in a database using PHP?
When building a form that includes radio buttons, you'll often want to store the selected value in a database for later use. In PHP, this can be done by first accessing the radio button's value from the $_POST superglobal array. This can be done like so:
$selected_radio = $_POST['radio'];
Once you have the value of the selected radio button, you can insert it into a database table just like you would with any other value. In general, it's best to store radio button values in a table with a separate column for each button. This makes it easy to query the database later to see which option was selected.
If you need to store multiple radio button values in a single database column, you can do so by concatenating the values together into a single string. For example, if you had a series of radio buttons for choosing a color, you could store the values in a single database column like this:
$selected_colors = $_POST['color1'] . ',' . $_POST['color2'] . ',' . $_POST['color3'];
You can then insert the string of selected values into a database table. When retrieving the data later, you can use the explode() function to split the string back into an array of individual values.
Overall, the best way to store radio button values in a database depends on your specific needs. If you only need to store a single value, then a separate column for each button is the best option. If you need to store multiple values in a single column, then concatenating the values into a string is the way to go.
A fresh viewpoint: Why Is Customer Lifetime Value Important
How can I store multiple radio button values in a database using PHP?
In order to store multiple radio button values in a database using PHP, it is important to understand how radio buttons work. Radio buttons are HTML elements that allow the user to select one option from a set of options. When the user clicks on a radio button, the value of the radio button is sent to the server.
The value of a radio button is determined by the value attribute of the element. Radio buttons are used to submit forms because they are small and easy to use. However, radio buttons have one major limitation: they can only submit one value.
This can be a problem when you want to submit multiple values from a single form. For example, let's say you have a form with two radio buttons, "Yes" and "No". The user can only select one of these options, but you want to store both values in the database.
One way to solve this problem is to use an array. An array is a data structure that can store multiple values. In PHP, you can create an array by using the array() function. The syntax for this function is as follows:
array(value1, value2, value3, ...)
To use this function, you would first create an array with the two values you want to store, "Yes" and "No". You would then loop through each value in the array and insert it into the database.
Here's an example of how you would do this:
$values = array("Yes", "No");
foreach($values as $value) {
// insert value into database
}
This code would insert both "Yes" and "No" into the database.
Another way to store multiple values from a radio button is to use the implode() function. This function takes an array and converts it into a string. The syntax for this function is as follows:
implode(separator, array)
The separator is a character that will be used to separate the values in the string. For example, if you wanted to separate the values with a comma, you would use this syntax:
implode(",", array)
Here's an example of how you would use the implode() function to store multiple radio button values in a database:
$values = array("Yes", "No");
$value_
For more insights, see: Radio Button Styling Css
How do I store a radio button value in a database using PHP and MySQL?
When designing a form that collects information from a user, you may find yourself needing to use radio buttons. Radio buttons are defined with the HTML tag, using the "radio" type. Radio buttons allow the user to select one of a set of mutually exclusive options. The value attribute of the tag is what will be stored in the database.
When the form is submitted, the value of the selected radio button will be sent to the server. In PHP, you can access the value of the radio button with the $_POST superglobal variable. For example, if the name of the radio button is "color", you would access the value with $_POST['color'].
Once you have the value of the radio button, you can store it in a database using PHP and MySQL. To do this, you will need to connect to the database using the mysqli_connect() function. Then, you can use the mysql_query() function to execute an SQL INSERT statement. Be sure to use proper SQL syntax and escape any user input to avoid SQL injection attacks.
Here is an example of how to store a radio button value in a database using PHP and MySQL:
$server = "localhost"; $username = "root"; $password = ""; $dbname = "myDB";
// Connect to the database $conn = mysqli_connect($server, $username, $password, $dbname);
// Get the value of the radio button $color = $_POST['color'];
// Execute an SQL INSERT statement $sql = "INSERT INTO table_name (column_name) VALUES ('$color')"; mysqli_query($conn, $sql);
// Close the connection mysqli_close($conn);
?>
In this example, we are storing the value of a radio button in a column of a database table. First, we connect to the database using the mysqli_connect() function. Then, we get the value of the radio button with the $_POST superglobal variable. Finally, we execute an SQL INSERT statement to store the value in the database.
Worth a look: Free Azure Sql Database
What is the best way to store a radio button value in a database using PHP and MySQL?
There are many ways to store a radio button value in a database using PHP and MySQL. The best way depends on the specific requirements of the project. For example, if the radio button is part of a form that will be submitted to a database, the value can be stored in the database when the form is submitted. If the radio button is part of a page that will be viewed by users, the value can be stored in a session variable or a cookie.
The value of a radio button can be stored in a database using PHP and MySQL in a number of ways. The best way depends on the specific requirements of the project. For example, if the radio button is part of a form that will be submitted to a database, the value can be stored in the database when the form is submitted. If the radio button is part of a page that will be viewed by users, the value can be stored in a session variable or a cookie.
When storing a radio button value in a database, it is important to consider the security of the data. Radio button values may contain sensitive information, such as passwords or credit card numbers. It is important to ensure that this data is stored in a secure database.
You might like: Data Lake Store
How can I store a radio button value in a database using PHP and MySQL?
When you submit a form that contains a radio button, the value of the radio button is sent to the server. You can then store that value in a database using PHP and MySQL.
To do this, you will first need to create a database table to store the values from the radio button. The table should have at least two columns - one for the value of the radio button, and one for the timestamp.
Next, you will need to create a PHP script that will insert the value of the radio button into the database table. The script should also check the value of the radio button before inserting it into the database. This will ensure that only valid values are stored in the database.
Finally, you will need to create a HTML form that will allow the user to select a radio button. The form should also contain a submit button.
When the user submits the form, the value of the radio button will be stored in the database.
Frequently Asked Questions
How do I get the radio button of a radio group?
In a form with radio groups, the radio buttons are represented by an $_POST or $_GET array.
How to get the value of a radio button with PHP code?
The name of a radio button is found in the form’s HTML element with an attribute called NAME. The value of a radio button can be accessed by using the PHP $_GET superglobal variable. If you want to set the value for a radio button, use the following code: value = 'Yes'; ?>
How to get the value of a radio button without iterating?
document.querySelector("#radioButton1").value
Where can I find the value property for radio buttons?
The value property for a radio button is found in the form’s source code, as demonstrated below: form . RadioButtons [ id ]. Value ;
What happens when a radio button is checked?
If a radio button is checked, the value of its value property will be sent to the server with the form. If the radio button isn't checked, no information is sent.
Sources
- https://stackoverflow.com/questions/17730937/php-getting-the-value-of-a-radio-button-and-storing-it-into-a-database
- https://www.fundaofwebit.com/post/how-to-insert-radio-button-value-into-database-in-php-mysql
- https://phpcoder.tech/question/store-radio-button-value-in-php-variable/
- https://t4tutorials.com/insert-values-in-database-with-radio-button-in-html-and-php/
- https://www.quora.com/How-do-you-store-the-value-of-a-radio-button-in-a-database
- https://www.folkstalk.com/2022/09/how-to-update-radio-button-value-in-database-using-php-with-code-examples.html
- https://stackoverflow.com/questions/39889070/getting-radio-button-value-and-storing-in-php
- https://www.c-sharpcorner.com/UploadFile/051e29/insert-value-from-radio-button-in-mysql-in-php/
- https://stackoverflow.com/questions/29542576/how-do-i-get-the-value-of-a-radio-button-in-php
- https://stackoverflow.com/questions/5472219/how-to-store-radio-button-value-in-database
- https://www.android-examples.com/php-insert-store-radio-button-clicked-value-to-mysql-database/
- https://www.sourcecodester.com/tutorials/php/4374/save-radio-button-value-using-phpmysql.html
- https://www.sitepoint.com/community/t/radio-button-value-save-to-database/14474
- https://alhadathtoday.com/how-to-store-radio-button-value-in-database-php/
Featured Images: pexels.com