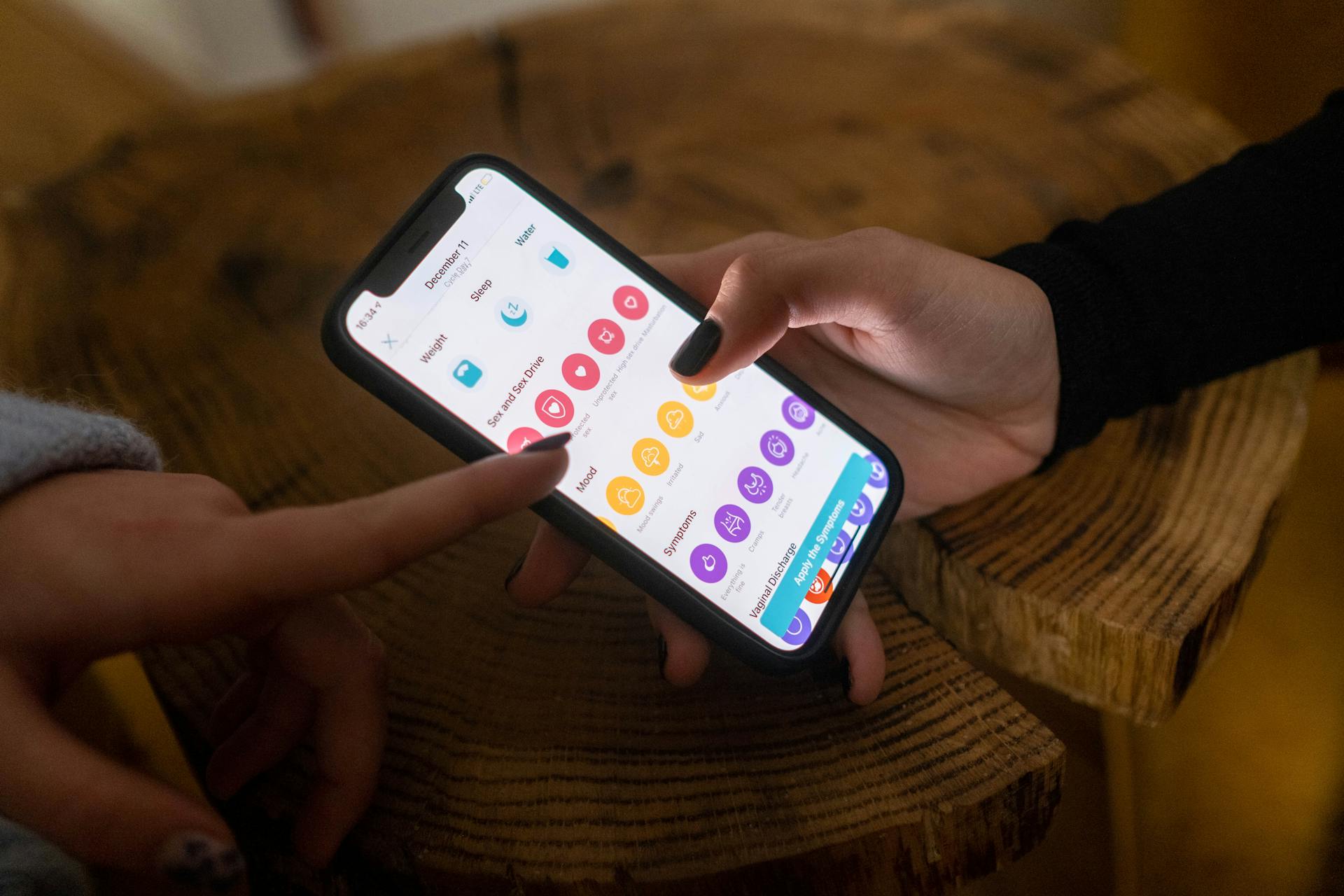
Using searchparams in Next.js can be a game-changer for better performance.
Using searchparams in Next.js allows for automatic optimization of static pages, which can lead to faster page loads and improved user experience.
By leveraging searchparams, developers can avoid unnecessary re-renders and server-side rendering, resulting in improved performance and reduced latency.
This approach is particularly useful for applications with a large number of pages or complex routing systems.
Recommended read: Nextjs Pages
Implementing URL Parameters in Next.js
Implementing URL Parameters in Next.js is a straightforward process. You'll need to have some understanding of Next.js routing and how to create a Next.js project. To learn more, check out OpenReplay’s articles on Routing and Getting started with Next.js.
To implement URL query parameters in a Next.js project, you'll need to import the useRouter hook from the 'next/router' package. This hook allows you to access the current URL and its query parameters. For example, you can use the router.query object to access the query parameters.
Broaden your view: Get Element Parameters Nextjs
Here's a step-by-step guide to implementing URL parameters in Next.js:
- Import the useRouter hook from 'next/router'
- Use the useRouter hook to access the current URL and its query parameters
- Use the router.query object to access the query parameters
- Use the getServerSideProps function to fetch data from the API route
By following these steps, you can easily implement URL parameters in your Next.js project.
Handling URL Parameters
URL parameters can be a better choice than useState in certain scenarios, especially when managing application state in a more versatile and shareable way.
A URL can have multiple parameters, and each parameter (key and value) is separated by an ampersand (&). For example, a search route URL might look like this: https://www.example.com/search?q=mens+t-shirt&size=3xl&color=white&sort=asc.
To implement URL parameters in Next.js, you'll need to understand the Next.js routing system and create a Next.js project. It's quite simple once you get started.
Handling edge cases in query parameters is crucial. This includes validating the incoming query parameters to make sure they are safe. Some edge cases to consider are undefined or null values, unexpected values, type checking, and security considerations.
Here are some edge cases to consider:
- Undefined or Null values: It’s important to always provide a fallback value for query parameters that are null or undefined.
- Unexpected values: In order to validate a user in your application using authentication tokens, it is important to sanitize URL query parameters to ensure they are valid.
- Type Checking: Make sure to validate query parameters against expected types and convert or parse values if necessary.
- Security Consideration: Use regular expressions to remove any special characters in query parameters.
To get the query params in the component, you can use the useRouter hook from the ‘next/router’ and then access the query parameters using router.query.
Take a look at this: Query in Nextjs
Accessing and Using URL Parameters
URL parameters are a powerful tool for managing application state in Next.js. They can be used to encode state information directly into the URL, making it easy for users to bookmark and share a specific page with others.
You can access URL parameters in Next.js by importing the useRouter function from the 'next/router' and creating a variable router. Then, using router.query, you can get the exact params used anywhere on the page.
To get a single query string value, you can use the .get() method of the URLSearchParams object. For example, to get the sort property value of name from the query string, you can simply use .get('sort').
You can also use the useSearchParams hook to access query params in a component. This hook returns an object with two properties: searchParams and setSearchParams. The searchParams property is an object with the query params, and the setSearchParams property is a function that updates the query params.
Suggestion: Using State in Next Js
Here are some edge cases to consider when using query parameters:
- Undefined or Null values: Provide a fallback value for query parameters that are null or undefined.
- Unexpected values: Sanitize URL query parameters to ensure they are valid.
- Type Checking: Validate query parameters against expected types and convert or parse values if necessary.
- Security Consideration: Use regular expressions to remove any special characters in query parameters.
Monitoring changes to a query string with useEffect is also a good practice. You can pass searchParams as a dependency into the useEffect hook, so that the code is executed every time a param changes.
Advanced Topics
In Next.js, you can use the `useSearchParams` hook to manipulate URL search parameters.
The `useSearchParams` hook returns an object with two properties: `searchParams` and `push`. The `searchParams` property is an object that represents the current search parameters, while the `push` property is a function that allows you to add or update search parameters.
You can use the `searchParams` object to access and manipulate individual search parameters.
To update a search parameter, you can use the `push` function, passing an object with the updated parameter and its new value.
For example, if you have a search parameter `q` with the value `hello`, you can update it to `world` by calling `push({ q: 'world' })`.
Readers also liked: How to Update Next Js
Performance and Optimization
The useSearchParamsState Hook is slower than the useState Hook, and it's due to the external nature of its state, which lives in the browser's APIs. This comes with a performance penalty.
Here's what happens every time we change state with the useSearchParams Hook:
- The History API is invoked by React Router.
- The browser updates the URL.
- React Router detects changes in the location.search and surfaces a new value for the application.
- The code in the application that depends on this value reacts.
This process is slower than just invoking useState and relying on a local variable. However, it's not overwhelmingly slower, and browsers are very fast these days.
Best Practices and Usage
To get the most out of usesearchparams in Next.js, use it in conjunction with getStaticProps to pre-render pages that rely on search parameters.
usesearchparams is best used for pages that don't have a lot of dynamic content, as it can slow down performance.
For example, if you're building a blog with a single page for each post, usesearchparams can be a good fit.
Always include the search parameters in your getStaticProps function to ensure they're properly handled.
Keep in mind that usesearchparams only works for static sites, so if you're building a dynamic site, you'll need to use a different approach.
When using usesearchparams, make sure to handle any errors that might occur, such as invalid search parameters.
If this caught your attention, see: Next Js Pages vs App
Sources
- https://blog.openreplay.com/state-management-in-react-using-url/
- https://ultimatecourses.com/blog/query-strings-search-params-react-router
- https://sourcefreeze.com/how-to-get-the-query-parameters-from-url-in-next-js/
- https://www.geeksforgeeks.org/next-js-functions-usesearchparams/
- https://blog.logrocket.com/use-state-url-persist-state-usesearchparams/
Featured Images: pexels.com