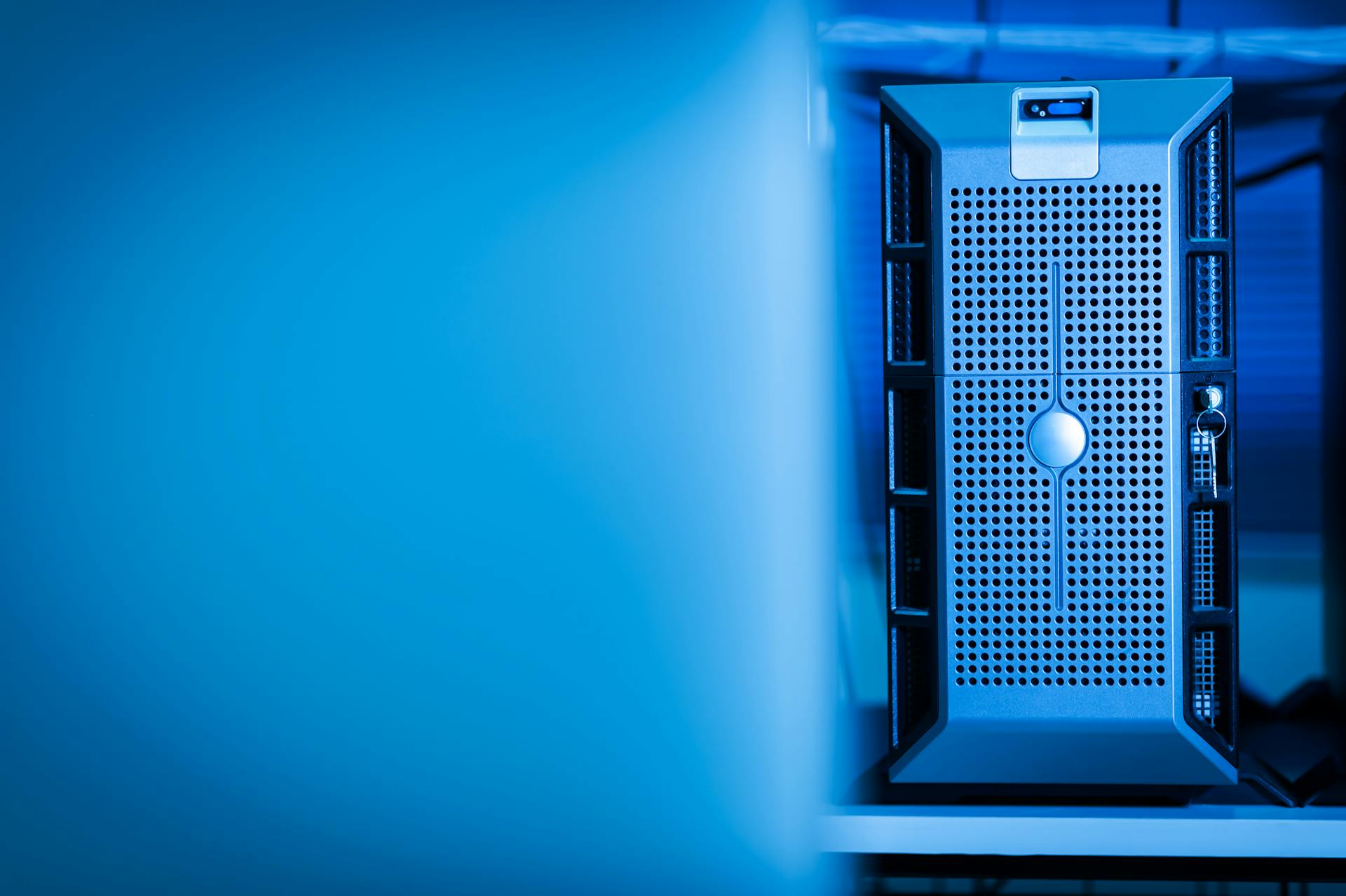
Managing S3 buckets with Terraform can be a breeze, especially when you understand the basics. S3 buckets can be created and configured directly in Terraform, making it easy to manage them.
You can create an S3 bucket in Terraform using the aws_s3_bucket resource, which allows you to specify the bucket name, region, and other properties.
With Terraform, you can also configure bucket policies, which define the permissions and access controls for your S3 bucket. This is a crucial step in securing your data.
Terraform's state management feature ensures that your S3 bucket is created and updated according to your configuration, even if you make changes to your code.
Creating and Managing S3 Bucket
Creating and managing an S3 bucket using Terraform is a straightforward process. You can start by installing Terraform and setting up your AWS credentials.
To create an S3 bucket, you'll need to specify the region and bucket name in your Terraform configuration file, typically named `main.tf`. You can also set the access control list (ACL) to private to restrict access to the bucket.
Here's a step-by-step guide to creating an S3 bucket using Terraform:
- Install Terraform and set up your AWS credentials.
- Create a new directory for your Terraform configuration and add a `main.tf` file.
- In the `main.tf` file, specify the region and bucket name, and set the ACL to private.
- Initialize the working directory with the `terraform init` command.
- Run the `terraform plan` command to preview the changes Terraform will make to your infrastructure.
- Review the output to ensure that Terraform will create the resources as expected.
- Run the `terraform apply` command to create the S3 bucket.
By following these steps, you can easily create and manage an S3 bucket using Terraform.
Create with Resource
To create an S3 bucket using Terraform, you'll need to specify the region and bucket name. The region can be any valid AWS region, such as "us-east-1". The bucket name must be unique across all of AWS.
You can use the aws_s3_bucket resource to create an S3 bucket. This resource requires three main attributes: region, bucket, and acl. The acl attribute sets the access control list for the bucket, and you can set it to private to restrict access.
To create a Terraform configuration file, you'll need to create a new directory and add a file named main.tf. This file will contain your Terraform configuration for creating an S3 bucket. You can define an AWS provider specifying the region and a basic S3 bucket resource.
Consider reading: S3 Bucket Naming
Here's an example of how to create an S3 bucket using Terraform:
- Install Terraform and set up AWS credentials.
- Create a new directory and add a file named main.tf.
- Define an AWS provider specifying the region and a basic S3 bucket resource.
- Run the terraform init command to initialize the working directory and download the required providers.
- Run the terraform apply command to create the S3 bucket.
Here's an example of the Terraform code snippet:
```terraform
resource "aws_s3_bucket" "example" {
region = "us-east-1"
bucket = "example-bucket"
acl = "private"
}
```
This code creates an S3 bucket named "example-bucket" in the "us-east-1" region with a private access control list.
To upload files to an S3 bucket, you can use the aws_s3_bucket_object resource. This resource requires the bucket ID, key, and source attributes. The key attribute determines the name of the file after it has been uploaded to the bucket, and the source attribute specifies the path to the file.
Here's an example of how to upload files to an S3 bucket using Terraform:
- Create a new file called document.txt within the terraform-s3 directory and add some sample text.
- Update the main.tf file with the following code:
```terraform
resource "aws_s3_object" "example" {
bucket = "example-bucket"
key = "document.txt"
source = "/path/to/document.txt"
}
```
This code uploads the document.txt file to the S3 bucket named "example-bucket" with the key "document.txt".
To apply the new changes, you can run the terraform plan command to preview the changes Terraform will make to your infrastructure. If everything looks good, you can proceed to the next step and run the terraform apply command to upload the files to the S3 bucket.
Here's a list of the main attributes required to create an S3 bucket using Terraform:
By following these steps and using the correct Terraform resources, you can create and manage S3 buckets using Terraform.
Delete
Deleting an S3 bucket is a straightforward process. You can simply run the universal $ terraform destroy command to delete all the resources you've created previously.
Terraform makes it easy to delete resources in the reverse chronological order, starting from the most recent ones. This means that test2.txt, test2.txt, and finally the bucket spacelift-test1-s3 will be deleted.
Deletion is always easy when using Terraform.
A different take: Aws S3 Sync --delete
S3 Bucket Configuration
You can create a new S3 bucket using Terraform by specifying the region and bucket name in the aws_s3_bucket resource. For example, you can use the following code to create an S3 bucket in the us-east-1 region with the name my-unique-bucket-name.
To configure S3 bucket replication using Terraform, you need to initialize the Terraform AWS Provider in two different regions, one for the source S3 bucket and the other for the destination. You can use an alias for each Terraform AWS provider block to distinguish between them easily.
To enable website configuration for an S3 bucket, you need to add a Bucket Policy and website configuration using the aws_s3_bucket_website_configuration resource block. For example, you can use the following code to enable website configuration for an S3 bucket named my-bucket.
Here are the required variables to enable website configuration for an S3 bucket:
- bucket_name
- index_document
- error_document
Note that you need to specify the bucket name, index document, and error document when enabling website configuration for an S3 bucket.
Create Configuration File
To create a Terraform configuration file for your S3 bucket, you'll need to create a new directory and inside that directory, create a file named main.tf. This file will contain your Terraform configuration.
The main.tf file should specify the AWS provider, including the region, and a basic S3 bucket resource. For example, you can use "us-east-1" as the preferred AWS region and "my-unique-bucket-name" as the name for the S3 bucket.
You'll also need to specify the name of the region, the name of the bucket, and the access control list (acl). The acl should be set to private. Here's a summary of the required information:
Once you've specified the required information, you can use the Terraform aws_s3_bucket resource to create the S3 bucket.
Versioning
Versioning is a crucial feature for managing multiple versions of an object in your S3 bucket. Enabling versioning helps you retain different versions of each object, which can be useful for tracking changes or maintaining a record of previous versions.
Discover more: Aws S3 Delete Object
To enable versioning, you'll need to modify your main.tf file. This is where you'll find the versioning block, which is a key component of enabling versioning on your S3 bucket.
The versioning block is included, with enabled set to true. This simple tweak enables versioning on your S3 bucket, which will keep multiple versions of each object stored in the bucket.
By enabling versioning, you'll be able to store and manage multiple versions of each object, making it easier to track changes and maintain a record of previous versions.
A different take: Aws Versioning S3
Configuring Encryption
Configuring encryption is a crucial step in securing your S3 bucket. You can choose from three types of server-side encryption (SSE) in Amazon S3: SSE-S3, SSE-KMS, and SSE-C.
To enable SSE-S3 encryption, you can use Terraform. This method encrypts each object with a unique key that is fully managed and rotated by the AWS S3 service. SSE-S3 uses the AES256 algorithm, which is one of the strongest encryption algorithms available.
For more insights, see: S3 Bucket Encryption
To implement SSE-S3 using Terraform, you need to create a simple S3 bucket using the aws_s3_bucket resource block. Then, you need to enable SSE-S3 type of encryption on the bucket by specifying the sse_algorithm parameter as AES256. This ensures bucket-level encryption.
Here are the key differences between SSE-S3 and SSE-KMS:
SSE-KMS encryption uses encryption keys managed by AWS Key Management Service (KMS) instead of AWS S3 service. To implement SSE-KMS using Terraform, you need to generate a Customer Master Key (CMK) using AWS KMS. Then, you need to enable SSE-KMS on your bucket by specifying the KMS Master Key (or the CMK previously generated) and the sse_algorithm parameter as aws:kms.
SSE-KMS provides more control over encryption keys and is recommended for sensitive data. However, it requires additional setup and management of KMS keys.
Related reading: Aws S3 Encryption
Configuring Lifecycle Rules
Lifecycle rules in Amazon S3 allow you to define automated actions for managing the lifecycle of objects stored in your S3 buckets. These rules help optimize storage costs, improve performance, and ensure compliance through the use of data retention policies.
Companies like Canva have successfully implemented lifecycle rules to manage infrequently accessed objects, resulting in significant cost savings - in their case, $3 million.
To implement lifecycle rules, you'll need to introduce a new configuration rule, which contains several important fields, including id, status, transition, storage_class, and expiration.
The id field is a unique identifier for the lifecycle rule, while the status field specifies whether the rule is enabled or disabled. In this example, the status is set to "Enabled".
The transition field defines the action to be taken on objects after a certain number of days, while the storage_class field specifies the target storage class for the objects. In this case, it's set to STANDARD_IA, representing the Standard-Infrequent Access storage class.
The expiration field defines the action to be taken on objects after a certain number of days. It's crucial to meticulously establish and examine lifecycle rules to ensure they align with your data retention and access needs.
Here's a summary of the lifecycle rule fields:
By implementing lifecycle rules, you can optimize storage expenses by automatically transferring objects to a more affordable storage class or removing them when they become unnecessary.
S3 Bucket Security
S3 Bucket Security is a top priority for many businesses. You can control access to your S3 bucket using IAM policies, which can be attached to your bucket. This is a more secure approach than using ACLs.
AWS Identity and Access Management (IAM) allows you to control access to your S3 bucket. You can define IAM policies and attach them to your bucket. Here's a simple example: create an IAM user and grant full access to the S3 bucket.
To manage ACLs and block public access, you can use Terraform's aws_s3_bucket_public_access_block resource. By default, the value is false, which means we are allowing public ACL (Access Control List). If you want to restrict the public ACL, you have to set the value to true.
Here are some key settings to manage public access:
- block_public_acls = true
- block_public_policy = true
- ignore_public_acls = true
- restrict_public_buckets = true
Note that AWS does not recommend using ACLs for access management anymore. Instead, use S3 Bucket Policy, which we will cover in the next section.
Policies
Policies are a crucial aspect of S3 bucket security, and understanding how to manage them is essential for protecting your data.
You can define IAM policies and attach them to your S3 bucket to control access. For example, you can create an IAM policy to grant full access to the S3 bucket.
The process of creating IAM policies involves using the `aws_iam_policy_document` data source, as shown in Example 5. This allows you to define the necessary permissions for your S3 bucket.
There are two types of policies: IAM policies and S3 bucket policies. IAM policies are applied to IAM users or roles, while S3 bucket policies are applied directly to the S3 bucket.
To create an S3 bucket policy, you can use the `aws_iam_policy_document` data source, as shown in Example 8. This allows you to define the necessary permissions for your S3 bucket.
Here's a summary of the main differences between IAM policies and S3 bucket policies:
By understanding how to manage policies, you can ensure that your S3 bucket is secure and only accessible to authorized users.
Manage Public Access Block
Managing public access block is a crucial step in securing your S3 bucket. By default, the value of aws_s3_bucket_public_access_block is false, which means public ACL is allowed. You can restrict public ACL by setting the value to true.
To manage public access, you can use the aws_s3_bucket_public_access_block resource. This resource allows you to block public ACLs, public policies, and ignore public ACLs. You can also restrict public buckets.
Here's a breakdown of the options you can use:
By setting these options to true, you can effectively manage public access to your S3 bucket.
Kms Encryption in
Kms Encryption in S3 using Terraform is a powerful way to secure your data. This method uses encryption keys managed by AWS Key Management Service (KMS) instead of AWS S3 service.
You can implement SSE-KMS using Terraform by generating a Customer Master Key (CMK) using AWS KMS and then using it to encrypt your data in S3. To do this, you'll need to add the following code to the kms.tf file:
Intriguing read: S3 Bucket Key
First, we have created a new S3 bucket using the aws_s3_bucket resource block.
Next, we used the aws_s3_bucket_server_side_encryption_configuration resource block to enable SSE-KMS on our Bucket by specifying the KMS Master Key (or the CMK previously generated). We have also specified the sse_algorithm parameter as aws:kms. This ensures SSE-KMS is enabled at the bucket level.
Finally, we have used the aws_s3_object resource to upload the same object as in the previous section. However, this time, we have mentioned the server_side_encryption parameter as aws:kms so that SSE-KMS encryption gets applied to the uploaded object. This ensures SSE-KMS at the object level.
Here's a summary of the key steps:
- Generate a CMK using AWS KMS.
- Enable SSE-KMS on the bucket using the KMS Master Key.
- Specify the sse_algorithm parameter as aws:kms.
- Upload an object with SSE-KMS encryption enabled.
Sources
- https://spacelift.io/blog/terraform-aws-s3-bucket
- https://kodekloud.com/blog/how-to-create-aws-s3-bucket-using-terraform/
- https://hands-on.cloud/terraform-s3-examples/
- https://blog.awsfundamentals.com/using-s3-with-terraform
- https://plainenglish.io/blog/aws-s3-bucket-creation-and-management-with-terraform
Featured Images: pexels.com