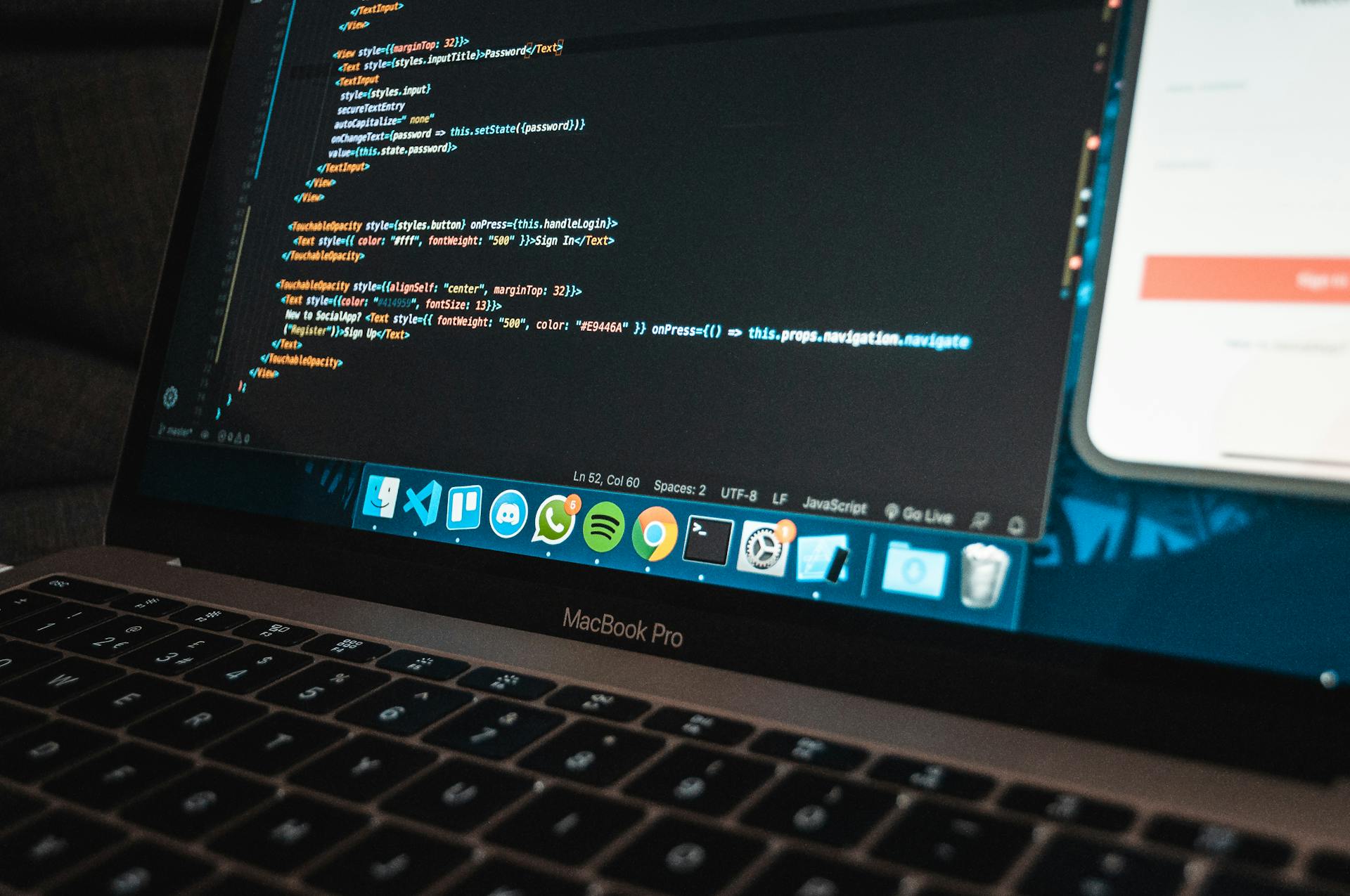
Next.js provides a built-in JSON parser to handle API data, making it easy to integrate with external APIs. This feature is especially useful for fetching data from APIs and rendering it on your Next.js pages.
The JSON parser in Next.js allows you to parse JSON data from APIs, making it easy to work with data in your Next.js applications. This is achieved through the use of the `json()` function.
Using the `json()` function, you can fetch data from APIs and parse it into a JavaScript object, which can then be used in your Next.js pages. This makes it easy to integrate with external APIs and display the data in a user-friendly way.
For another approach, see: Next Js Pages vs App
API Integration
API Integration is a crucial aspect of Next.js development, and it's essential to understand how to integrate APIs into your Next.js application. You can start by initializing your project using the default commands, such as `npx create-next-app {project-name}`.
To fetch data from an API, you'll need to create a file called `getdata.js` in the `lib` folder. This file should contain an async function that exports `getData()`, which will fetch data from your desired API link.
To use the `getData()` function, you'll need to import it in your `pages.js` file. You can do this by adding the line `import { getLocalData } from "@/lib/getdata"`.
Once you've imported the `getLocalData` function, you can create an async function in your home component to fetch the data using a variable. Here's an example of how you can do this:
- Create an async function in your home component and await your fetched data using a variable
- Return the following code to map your fetched data with your element: `{JSON.stringify(post)}`
Here's a step-by-step guide to API integration in Next.js:
- Initialize your project using `npx create-next-app {project-name}`
- Create a `lib` folder and add a `getdata.js` file
- In `getdata.js`, create an async function that exports `getData()`
- In `pages.js`, import the `getLocalData` function
- Create an async function in your home component to fetch the data using a variable
- Map your fetched data with your element using `{JSON.stringify(post)}`
By following these steps, you can successfully integrate APIs into your Next.js application.
Creating API Routes
Creating API Routes is a crucial step in building a full-stack web application with Next.js. To create an API Route, you need to create a file in the api folder found in the pages folder of your application.
A unique perspective: What Is .next Folder in Next Js
The api folder contains an example API route named hello.js, which returns a JSON response when accessed at localhost:3000/api/hello. Every API route or endpoint must export a default function that handles the requests made to that endpoint.
The function receives two parameters: req, an instance of http.IncomingMessage, and res, an instance of http.ServerResponse. These methods are familiar if you've worked with Node.js and Express.js before.
You can handle different HTTP request methods in an API route by writing the handler function with a switch statement, or using methods like if/else statements. To submit data from a form in your application and send it to an API route using a POST request method, you can create a form that sends post data to the API route.
With Next.js, you can access or store data in your database like you would if you were using a separate backend application. This is achieved through API Routes, which eliminate the need for creating an additional backend server in your full-stack web applications.
Related reading: Nextjs App Route Get Ssr Data
Type Safety
Type Safety is a crucial aspect of Next.js, especially when working with API Routes. Next.js provides automatic types to ensure your API Routes are type-safe.
You can take advantage of this feature without any extra setup, thanks to Next.js' zero-configuration approach.
However, keep in mind that you need to have integrated TypeScript in your Next.js project before using these features.
Sources
- https://dev.to/feeqcodes/display-json-data-in-nextjs-1hde
- https://refine.dev/blog/next-js-api-routes/
- https://blog.sentry.io/common-errors-in-next-js-and-how-to-resolve-them/
- https://upmostly.com/next-js/mastering-next-js-how-to-load-data-from-files-in-your-projects
- https://nextjs.org/docs/pages/building-your-application/routing/api-routes
Featured Images: pexels.com