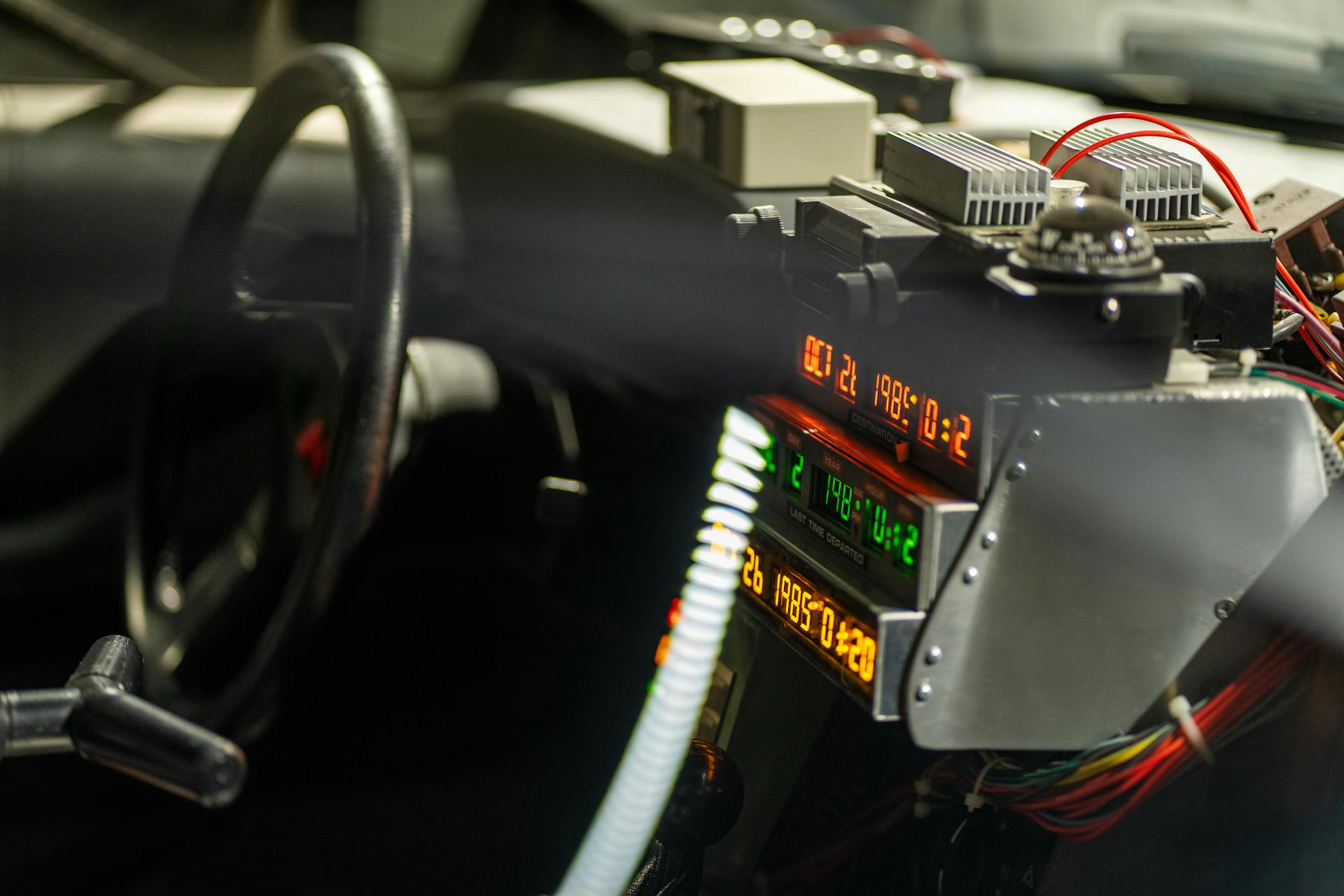
Developing a full-stack Next.js CRUD application with Supabase and Prisma is a powerful combination. Supabase provides a PostgreSQL database, and Prisma allows for a robust ORM (Object-Relational Mapping) system.
This setup enables you to create, read, update, and delete data with ease. In fact, we'll be using Prisma's auto-generated API client to interact with our database.
With Supabase, you can create a PostgreSQL database instance in minutes, and Prisma will generate a schema for you based on your database structure. This means you can get started with building your application quickly.
A different take: Nextjs Prisma Docker
Project Setup
To set up your Next.js project for CRUD operations, you'll first need to create a database. This can be done by running a command in the MongoDB shell to switch to a new database called my_blog, which will be used to store blog posts.
The first step is to initialize your Next.js app using create-next-app and install the MongoDB driver. This will give you a solid foundation for building your web application.
Broaden your view: Mongodb Nextjs
Next, you'll need to structure your app into pages, components, models, and lib directories. This includes creating dynamic routes for your pages, such as Home and Blog, as well as a Layout component in components/layout.js that will handle the header, footer, and render children.
Here's a breakdown of the key directories and files you'll need to create:
- pages: for your dynamic routes, such as Home and Blog
- components: for your Layout component and other reusable components
- models: for your MongoDB models, such as your blog post schema
- lib: for your utility functions and other library code
Next.js CRUD
Next.js CRUD is a powerful combination that enables developers to build robust, scalable web applications quickly and efficiently. Next.js is a widely used React framework that enables server-side rendering and simplified page routing, while MongoDB is a popular NoSQL database known for its flexible document data model, scalability, and high performance.
To create a CRUD API in Next.js, you can start by creating a new Next.js project and installing the necessary dependencies. You can then create a MongoDB database instance and set up a connection to it using Mongoose. This will allow you to interact with the database using Mongoose methods.
Expand your knowledge: Next Js Set Cookie
One of the key steps in creating a CRUD API is to set up the API routes that handle create, read, update, and delete operations. In Next.js, you can create API routes by creating new files in the `src/pages/api` directory. For example, you can create a `products` directory and add files for each CRUD operation, such as `add.js`, `delete.js`, `list.js`, and `update.js`.
Here is a summary of the CRUD API routes:
- `add.js`: Handles create operations
- `delete.js`: Handles delete operations
- `list.js`: Handles read operations
- `update.js`: Handles update operations
When creating a CRUD API, it's also important to consider authentication and authorization. In the example provided, the CRUD API routes are not authenticated, so it's expected to get an error when trying to access the routes.
To create a product data, you can use the `add.js` file to create a new product record in the database. You can also use the `update.js` file to update an existing product record. The `delete.js` file can be used to delete a product record from the database.
Here is an example of how to create a product data using the `add.js` file:
```javascript
// src/pages/api/products/add.js
import { NextApiRequest, NextApiResponse } from 'next';
import { MongoClient } from 'mongodb';
const mongoClient = new MongoClient('mongodb://localhost:27017');
const db = mongoClient.db();
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
For your interest: Next Js File Structure
const product = req.body;
const result = await db.collection('products').insertOne(product);
return res.json(result);
}
```
This code creates a new product record in the `products` collection of the database using the `insertOne` method. The `req.body` object contains the product data that is being created.
In conclusion, Next.js CRUD is a powerful combination that enables developers to build robust, scalable web applications quickly and efficiently. By following the steps outlined in this article, you can create a CRUD API in Next.js that interacts with a MongoDB database instance using Mongoose methods.
A unique perspective: Using State in Next Js
Database Connection
To connect your Next.js app to a database, you'll need to set up a connection to MongoDB. This can be done by creating a mongodb.js file that sets up a reusable database connection.
To connect a database to Next.js, you'll need to follow a few key steps. First, you'll need to create a database connection using Mongoose, a popular ODM for working with MongoDB.
You can connect Mongoose to MongoDB in Next.js by importing and connecting it to your database using the URI. This will allow you to use Mongoose's API to interact with your MongoDB database.
A different take: Connect Rpc Nextjs
Connecting Mongoose to MongoDB in Next.js is straightforward. You can do this by creating a new file, such as pages/api/db.js, and configuring Mongoose to connect to your locally running MongoDB instance.
Alternatively, you can use Prisma to connect your Next.js app to a Postgres database. To do this, you'll need to update the .env file with your database credentials and run the prisma migrate command to update the schema in the DB.
Here are the steps to connect to a Postgres database using Prisma:
To test if everything is working correctly, you can use Prisma Studio, a tool that comes with Prisma. Simply type `npx prisma studio` in your terminal and open localhost:5555 in your browser.
Mongoose works seamlessly with Next.js right out of the box. To get started, simply install the required dependencies, including Mongoose, and you'll be able to require('mongoose') in your Next.js API routes and models.
Broaden your view: Prisma Next Js
Database Operations
Database operations are crucial for any Next.js CRUD application. To implement CRUD operations, we need to connect our Next.js app to a database, such as Supabase.
For more insights, see: Next Js Db
We can implement CRUD operations by defining our endpoint, using the correct HTTP method, and passing in the necessary data. For example, to create product data, we can define an endpoint at /skus/add using POST, and use the preValidation hook to modify the body and create an Insert operation from it to our products table.
The four CRUD operations - Create, Read, Update, and Delete - are essential for managing data in our application. We can implement these operations using various methods, such as using HarperDB Custom Functions with secure Next.js endpoints as proxies. We can also use Mongoose models to interact with MongoDB through Mongoose's API.
Worth a look: Use Client Nextjs
Deleting
Deleting is a crucial aspect of database operations, ensuring that unnecessary data is removed to maintain data integrity and optimize performance.
You can delete a product by linking to a page with a query parameter that specifies the product to be deleted.
To confirm deletion, check if the results include "deleted hashes", which is your ID, indicating a successful update.
You might like: Nextjs App Route Get Ssr Data
Once a product is deleted, it should no longer be visible in your database.
Deleting a SKU is a straightforward process that involves using the Delete operation and associating it with an existing record.
You can test deletion by navigating to the Delete Product page and confirming your action.
To delete data, you can add a mutation query to your Todos/index.tsx file with delete functionality.
When testing deletion, make sure to update the DeleteIcon onClick functionality with the delete function.
Create Mongoose Models
Creating Mongoose models is a crucial step in building a robust database for your Next.js application.
You can create Mongoose models after connecting Mongoose to your MongoDB database.
Mongoose models can then interact with MongoDB through Mongoose's API.
This allows you to define schemas for your data and perform CRUD operations easily.
With Mongoose models, you can create, read, update, and delete data in your MongoDB database.
You can create models like this to manage your data.
Models like this can then interact with MongoDB through Mongoose's API.
Curious to learn more? Check out: Next Js Project
Security and Optimization
You can enable authentication and authorization in Next.js CRUD using a library like Next-Auth. This allows you to manage user sessions and protect routes with access control.
To optimize performance, consider using a caching mechanism like Redis or Memcached. This can reduce the load on your database and improve page load times.
Implementing a Content Delivery Network (CDN) can also help distribute static assets across different geographic locations, reducing latency and improving user experience.
If this caught your attention, see: Nextjs Usecontext
Securing Custom Function Requests with Serverless Functions
To secure custom function requests, Next.js serverless API functions can be used.
In order to make requests to custom functions securely, you need to know which location to make requests to. This is done by hitting an endpoint in the application.
You should not hit this endpoint in your browser, as it may impact the Table in your CRUD API, whether adding, updating, or deleting.
The code in the src/pages/api/products/update should be updated to include the ID of the product you want to update in the body.
When making a delete request, only pass in the ID from the body, just like when updating a product.
By following these steps, you can ensure that your custom function requests are secure and successful.
Worth a look: Next Js Custom Server
Aggregation Caching for Performance Optimization
Caching aggregated data in Redis can significantly reduce the load on your database.
This is particularly useful for applications that require frequent aggregations, such as displaying real-time analytics or charts.
To implement aggregation caching, you can use Next.js middleware to cache the aggregated data for a specified period.
For example, you can cache the aggregation result for 60 seconds, avoiding extra load on the database.
By implementing aggregation caching, you can ensure your application remains responsive and efficient, even with high traffic or complex queries.
Full-Stack Development
Combining MongoDB and Next.js is an excellent choice for launching full-stack web apps, as it brings intuitive, dynamic data storage from MongoDB and server-side rendering, static site generation, and API routes from Next.js.
MongoDB's flexible schemas rapidly adapt to changing data requirements, while Next.js pre-renders pages for snappy initial loads.
Together, they enable rapid iteration without the overhead of traditional SQL databases, making them a great choice for those looking to level up their JavaScript stacks.
Here are the key benefits of this stack:
- MongoDB's flexible schemas
- Next.js pre-renders pages
- Granular MongoDB indexes
- Next.js incremental static regeneration
Synergizing for Full-Stack Development
Synergizing MongoDB and Next.js creates an excellent stack for full-stack development. This combination rapidly adapts to changing data requirements with MongoDB's flexible schemas. Next.js pre-renders pages for snappy initial loads, making it ideal for building fast and scalable web applications.
Together, MongoDB and Next.js enable rapid iteration without the overhead of traditional SQL databases. This powerful combination brings intuitive, dynamic data storage and server-side rendering, SSG, and API routes to handle all frontend needs.
MongoDB's granular indexes optimize query performance, while Next.js incremental static regeneration scales well. This synergy makes it an excellent choice for launching full-stack web apps.
Here are the key benefits of this stack:
- MongoDB's flexible schemas
- Next.js pre-renders pages
- Granular MongoDB indexes
- Next.js incremental static regeneration
By leveraging these strengths, developers can build robust, scalable web applications with minimal backend configuration. Whether you're a seasoned developer or a beginner, this tech combo is worth exploring further.
Develop a Full-Stack Application with Supabase
Developing a full-stack application can be a daunting task, but with the right tools and knowledge, it can be a breeze. You can create a full-stack application quickly using Next.js and Supabase, which offer a powerful combination for building robust, scalable web applications with minimal backend configuration.
Related reading: Next Js Stack
To start, you'll need to set up a Next.js project, which can be done in just a few minutes. Once you have your project set up, you can create a Supabase project and connect it to your app.
Here's a quick rundown of the steps to connect Next.js to Supabase:
1. Create a new file called .env.local in the root of your Next.js project and add the Supabase URL and anonymous key.
2. Create a new file called utils/supabase.ts and add the Supabase client code.
3. Set up your database table in the Supabase project dashboard.
4. Modify the app/page.tsx file to fetch and display items from your Supabase database.
By following these steps, you'll have a functional web app that allows you to manage a list of items, demonstrating the core operations of any data-driven application.
Here are the key benefits of using Next.js and Supabase:
- Rapid development with minimal backend configuration
- Scalable and robust web applications
- Easy integration with Supabase database
- Fast and efficient data retrieval and manipulation
Whether you're a seasoned developer or a beginner, this combination of tools can help you create a full-stack application quickly and efficiently.
Sources
- https://www.harperdb.io/development/tutorials/create-a-crud-api-w-next-js-harperdb
- https://nextjsstarter.com/blog/mongodb-with-nextjs-a-developers-quickstart-guide/
- https://ilearnedathing.com/how-to-create-a-crud-app-using-nextjs-and-supabase
- https://www.cloudnweb.dev/2022/2/supabase-nextjs-authentication-crud
- https://dev.to/francescoxx/typescript-crud-api-with-trpc-4689
Featured Images: pexels.com