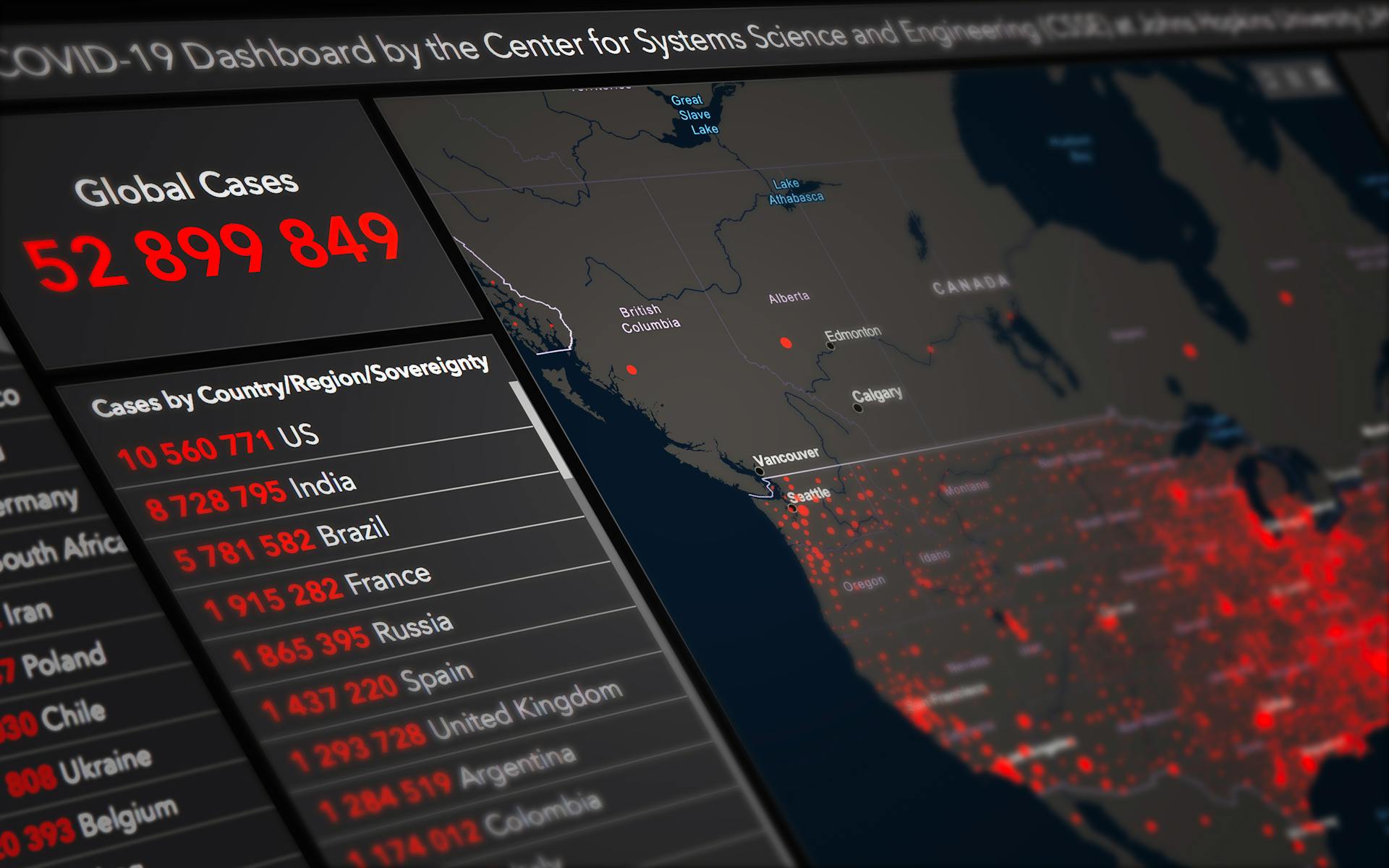
Nextjs Experimental App is a feature that allows developers to test and experiment with new features and APIs without affecting their production app. This is done by enabling the experimental app in the next.config.js file.
The experimental app is a separate instance of the app that runs alongside the production app. It's a way to test new features and APIs without affecting the live app.
To enable the experimental app, you need to add the following code to your next.config.js file: `module.exports = { experimental: { appDir: true } }`. This tells Next.js to create a separate instance of the app.
This allows you to test new features and APIs in a safe and controlled environment.
You might like: How to Build Next Js App for Production
Routing and App Structure
In Next.js 13, the new App Router works alongside the Pages Router to support incremental adoption and provide new features like server-side rendering and static site generation.
The App Router is controlled via folders inside the app directory, where a folder structure like app/profile/settings/page.jsx takes care of rendering the /profile/settings route.
To use the App Router, you can create various types of components in the app directory using a specific filename convention: pages are defined as page.tsxlayouts are defined as layout.tsxtemplates are defined as template.tsxerrors are defined as error.tsxloading states are defined as loading.tsxnot found pages are defined as not-found.tsx
This allows you to colocate your files and define any type of component in the app directory without it becoming a page, unlike the older Pages Router.
Explore further: Nextjs Pages
Router Comparison
The Pages Router is a familiar concept for those who've worked with previous Next.js versions, where any file created inside the pages directory acts as a route in the UI.
If you've worked with previous versions of Next.js, you might already be familiar with the Pages Router. Any file created inside the pages directory would act as a route in the UI. For example, pages/home.jsx would take care of the /home route.
The new App Router is designed to work alongside the Pages Router to support incremental adoption.
The App Router provides new features like server-side rendering and static site generation.
The Pages Router and App Router can coexist, allowing for a gradual transition to the new App Router.
Worth a look: Next Js Folder Structure
Routing
Routing in Next.js 13 is a game-changer. The new App Router works alongside the Pages Router to support incremental adoption and provide new features like server-side rendering and static site generation.
You can control routing with the App Router by using folders inside the app directory. Each folder represents a route, and the UI for that route is defined by a page.jsx file inside the folder.
Worth a look: What Is .next Folder in Next Js
Routing with the App Router is controlled via folders, just like files in the pages directory. The UI for a particular route is defined by a page.jsx file inside of the folder.
The new App Router allows you to colocate your files, making it easier to define any type of component in the app directory without it becoming a page.
Here's a breakdown of the conventional filenames you can use in the app directory:
- pages are defined as page.tsx
- layouts are defined as layout.tsx
- templates are defined as template.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
- not found pages are defined as not-found.tsx
You can use the layout.tsx file to define a UI that is shared across multiple pages. A layout can render another layout or a page inside of it.
A layout can render another layout or a page inside of it, and its state is preserved when the route changes.
Server-Side Rendering
Server-Side Rendering is a game-changer in Next.js, especially with the new --experimental app. With Server Components as the default, you can render pages faster and reduce the amount of JavaScript sent to the client.
Server Components are a new type of React component that run on the server and return compiled JSX, which is sent to the client. This is a big shift from traditional React components that run on both the server and client.
Server Components can import client components, making it easy to mix and match the two. However, Server Components have some constraints, such as not being able to use React hooks, Context, or browser-only APIs.
Here are some benefits of using Server Components:
- Render pages faster
- Reduce the amount of JavaScript that needs to be sent to the client
- Improve the routing performance of server-rendered pages
Server Components are useful for rendering the skeleton of a page, while client components handle the interactive bits. This approach improves performance and reduces the amount of JavaScript needed on the client.
Additional reading: Next Js Debugging
App Directory and File Setup
The app directory in Next.js 13 is an experimental feature that allows us to build Next.js apps in a new way. It coexists with the pages directory and can be used to incrementally migrate an existing project to the new directory structure.
Broaden your view: Src Directory Nextjs
To set up the app directory, you'll need to copy the example's .env.local.sample file, rename it to .env.local, and set the NEXT_PUBLIC_WORDPRESS_URL to your WordPress site's URL. You can keep the NEXT_PUBLIC_URL set to http://localhost:3000 for now.
The new directory structure allows us to colocate files, making it easier to define any type of component in the app directory without it becoming a page. This means we can place components for a specific page right in the folder where it's defined, without adding a new path segment to the routing.
Here's a list of the types of components we can define in the app directory using conventional filenames:
- pages are defined as page.tsx
- layouts are defined as layout.tsx
- templates are defined as template.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
- not found pages are defined as not-found.tsx
Layout Tsx File
The layout.tsx file is a powerful tool in Next.js that allows you to define a UI shared across multiple pages. This file can render another layout or a page inside of it, and its state is preserved whenever a route changes to any component within the layout.
Explore further: Nextjs Server Actions File Upload
You can use the layout.tsx file to create a consistent look and feel across your app by defining common UI elements and components. This file can be used to render another layout or a page inside of it, making it a great way to organize your code and improve maintainability.
To create a layout.tsx file, simply create a new file with the name layout.tsx in your pages directory. This file will serve as the top-level layout for your app.
Here are some key benefits of using the layout.tsx file:
- Shared UI components: You can define common UI elements and components in the layout.tsx file, making it easy to reuse them across your app.
- State preservation: When you navigate to a new route, the state of the layout.tsx file is preserved, ensuring that your app remains consistent and user-friendly.
- Flexibility: You can use the layout.tsx file to render another layout or a page inside of it, giving you flexibility in how you organize your code.
By using the layout.tsx file, you can create a robust and maintainable app that meets the needs of your users.
App Directory
The app directory is a new way to build Next.js apps, released in Next.js 13. It's an experimental feature that coexists with the pages directory, allowing you to incrementally migrate an existing project to the new directory structure.
The app directory is a whole new routing system underneath, much more powerful than the current one. It allows you to colocate your files, which means you can define any file in the app directory without it becoming a page component.
For your interest: Trpc Nextjs App Directory
You can place your components for a specific page right in the folder where it's defined, making it easier to organize your code. For example, you could place your components for a specific page in a folder named after the page.
The app directory also allows you to create new layouts, loading files, and pages without adding a new path segment to the routing. This means that all pages under a certain directory will be accessed from the root path, without the need for a separate path segment.
Here are the different types of components you can define in the app directory using conventional filenames:
- pages are defined as page.tsx
- layouts are defined as layout.tsx
- templates are defined as template.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
- not found pages are defined as not-found.tsx
Note that server components do not need a notation to be defined as such in the app directory. They are the default component type in the new app directory, and can only use server-side APIs.
Local File Setup
To set up your local file, start by copying the example's .env.local.sample file and renaming it to .env.local.
See what others are reading: File Upload Next Js Supabase
You'll need to update the NEXT_PUBLIC_WORDPRESS_URL to your WordPress site's actual URL.
The NEXT_PUBLIC_URL is set to http://localhost:3000 by default, but you can leave it as is for now if you're working on a local development environment.
This set-up is a crucial step in getting your app directory and file structure in place.
Enhanced Router Features
The nextjs --experimental app brings a lot of exciting changes to the routing system. The new App Router works alongside the Pages Router to support incremental adoption and provide other new features like server-side rendering and static site generation.
You can now create various types of components in the app directory using a specific filename convention. This is a big improvement over the current routing system, which required a specific directory structure to define pages, API handlers, and so on.
Here's a breakdown of the new filename conventions:
Enhanced Router
The Enhanced Router in Next.js is a game-changer. It allows us to define various types of components in the app directory using a specific filename convention.
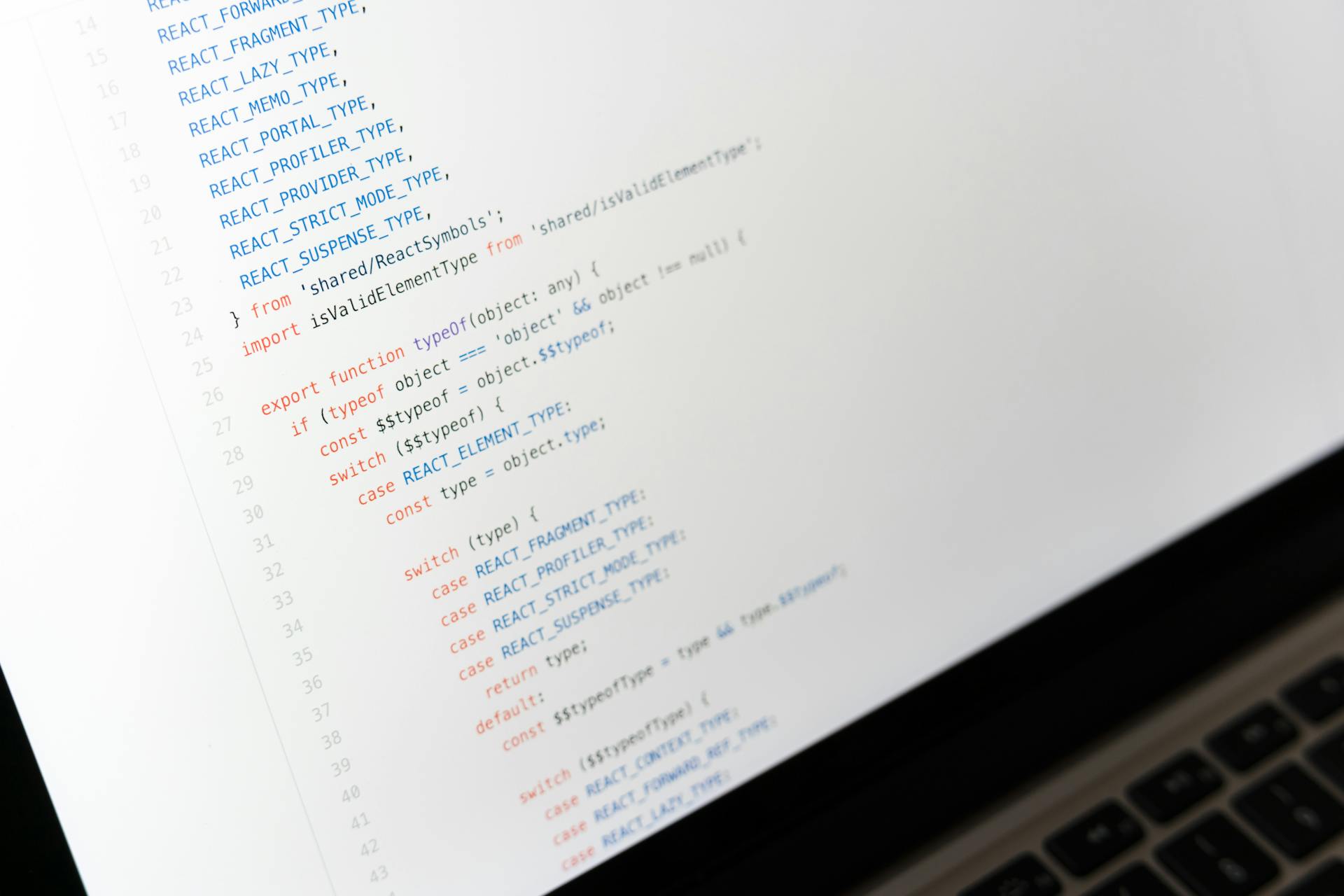
Using conventional filenames, we can create different types of components in the app directory. This is a big improvement over the current routing system, which requires us to use a specific directory structure to define pages, API handlers, and so on.
We can now define pages as page.tsx, layouts as layout.tsx, templates as template.tsx, errors as error.tsx, loading states as loading.tsx, and not found pages as not-found.tsx. This makes it much easier to organize our code and create complex applications.
Here's a quick rundown of the filename conventions:
- pages are defined as page.tsx
- layouts are defined as layout.tsx
- templates are defined as template.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
- not found pages are defined as not-found.tsx
This new approach to routing makes it much easier to create complex applications with multiple types of components. We can colocate our files in the app directory, which makes it easier to organize our code and reduce complexity.
Redirect Side-Effect
Redirects in Next.js can throw errors, which is a bit of a gotcha. The new `redirect` function has a return type of `never`, meaning it won't return a value, and if you catch the error, you need to be careful to follow the redirect.
Take a look at this: Next Js Redirect
To manipulate the response, for example by setting cookies, you can use the `NextResponse` object. This utility is exported by the Next.js package and gives you more control over the redirect process.
If you want to redirect the user to a different page, you need to be careful to follow the redirect thrown by the error. This is where the `NextResponse` object comes in handy.
Data Handling and Fetching
Fetching data in Next.js can be done using the getAuthClient function, which is part of the @faustwp/experimental-app-router package. This function is specifically designed for use in React Server Components (RSC).
You can also use the getAuthClient function in the Next.js App Router from an RSC, and it's used in an example project under the folder my-account.
To load data in Layout Components, you can use the new use hook, which is an experimental hook in React that uses Suspense to fetch data on the server. This allows you to write asynchronous code in a synchronous way.
On a similar theme: Rsc Nextjs
Here are the steps to fetch data in Layout Components using the use hook:
- Fetch the data in the layout component using the use hook.
- Conditionally render the ProfileDropdown component based on the data.user property.
Alternatively, you can make the component an async component and use async/await to fetch the data from getData.
Broaden your view: Nextjs Script Async
Fetching Data
Fetching data in Next.js can be achieved through various methods. One approach is to use the getAuthClient function, specifically designed for use in React Server Components (RSC), or the getAuthClient function for making authenticated requests in the Next.js App Router from an RSC.
The getAuthClient function is part of the @faustwp/experimental-app-router package, and an example of its use can be found in the example project under the folder my-account.
To fetch data in Layout Components, the new use hook can be used, an experimental hook in React that uses Suspense to fetch data on the server.
Here's a step-by-step guide on how to fetch data using the use hook:
- Fetch the data in the layout component using the use hook.
- Conditionally render the ProfileDropdown component based on the data.user property.
This approach allows us to write asynchronous code in a synchronous way, making it seem like we're fetching data in a synchronous manner.
Alternatively, we can make the component an async component and use async/await to fetch the data from getData.
Here's an interesting read: Nextjs App Route Get Ssr Data
Handling Webhooks
Handling webhooks is a common use case for API routes, and getting the raw body request is now much simpler.
In Next.js App Router, you can get the raw body request by using the request.text() method. This makes it easier to handle webhooks compared to the Pages routing system.
You can define a server action from a client component, but it needs to be exported from a separate file and imported in the client component. The file should have the keyword use server at the top.
There are multiple ways to call the server action from the client, including defining the action as the action property of a form component, calling the action from a button component using the formAction property, calling the action using the useTransition hook (if it mutates data), or simply calling the action like a normal function (if it does not mutate data).
Here are the ways to call the server action from the client:
- Defining the action as the action property of a form component
- Calling the action from a button component using the formAction property
- Calling the action using the useTransition hook (if it mutates data)
- Simply calling the action like a normal function (if it does not mutate data)
Sources
Featured Images: pexels.com