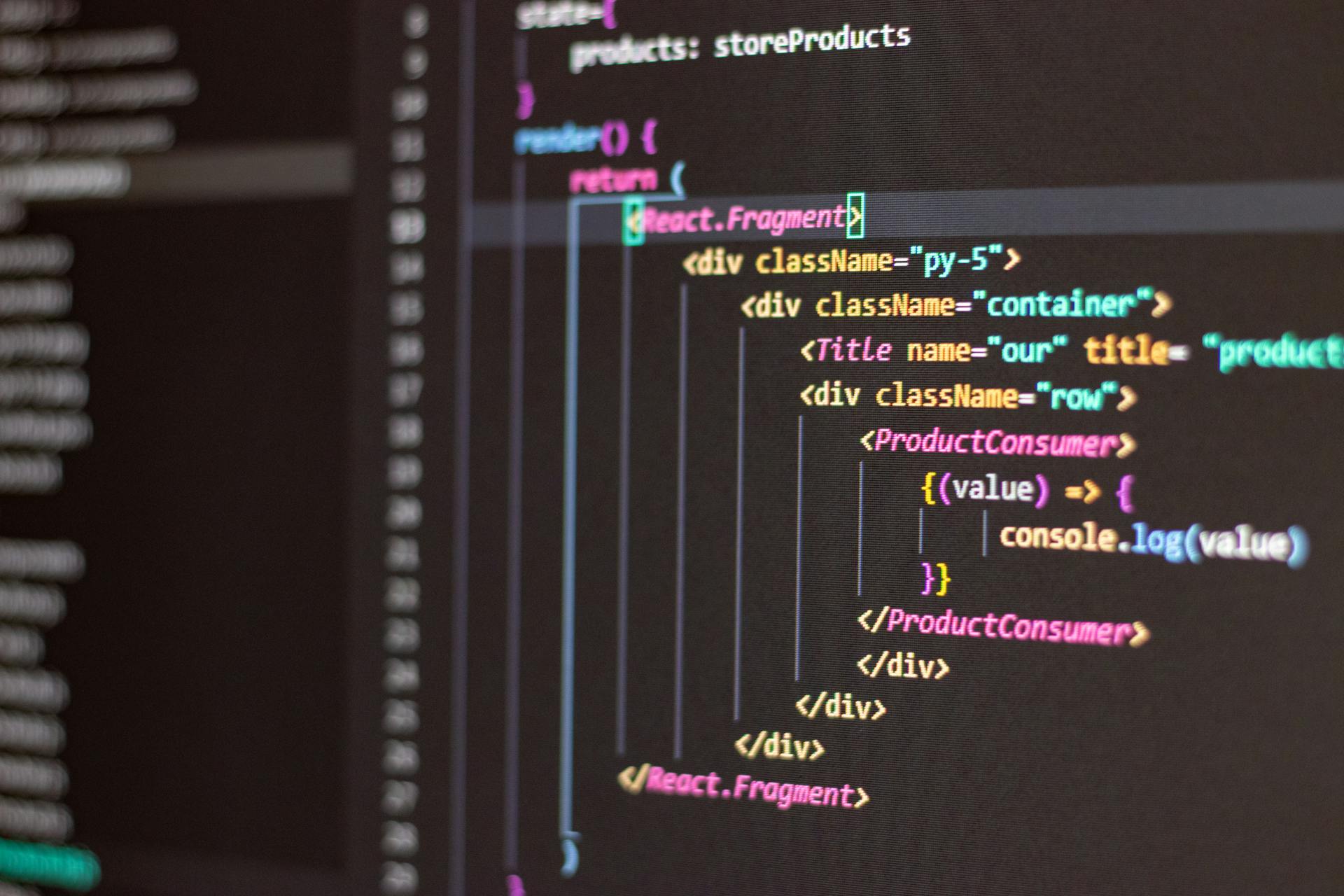
Next Js Axios is a powerful combination that can significantly improve the efficiency of API request handling in Next.js applications.
Axios is a popular JavaScript library used for making HTTP requests in Next.js, and it's particularly useful for handling API requests in a more efficient way.
By using Axios, you can simplify your code and reduce the number of lines needed to make API requests, making it easier to manage and maintain your codebase.
Axios also provides a lot of features out of the box, such as automatic JSON data parsing and error handling, which can save you a lot of time and effort.
What Is
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized web applications.
Next.js provides a built-in API route feature that allows you to create API routes using Node.js.
Axios is a lightweight HTTP client library that makes it easy to send HTTP requests and interact with APIs.
Next.js and Axios can be used together to create a powerful API client for your Next.js application.
Axios supports both promise-based and async/await-based APIs, making it easy to write code that's both readable and maintainable.
Next.js provides a built-in support for Axios, making it easy to use Axios in your Next.js application.
Axios is a widely-used library with a large community and extensive documentation, making it a great choice for building robust and scalable APIs.
Next.js and Axios can be used together to create a robust and scalable API client for your Next.js application.
Intriguing read: Nextjs Spa
Architecture and Features
Next.js Axios offers a promise-based architecture, making it simpler to write asynchronous code. This architecture enables you to use .then() and .catch() for handling successful and unsuccessful responses, respectively.
Axios is also compatible with async/await, making your asynchronous code even more readable. This means you can write cleaner and more efficient code.
Fetch, on the other hand, is based on Promises, allowing you to use “then” and “catch” methods to deal with successful or unsuccessful operations. You can also make your code cleaner and more readable with “async/await.”
If this caught your attention, see: Next Js Architecture
Fetch allows for customization in a less direct manner, but you can specify settings such as the HTTP method to use, headers to include, and body data to send in a separate configuration object when calling the fetch() method.
Here are some key features to consider:
- Promises and async/await: Fetch is based on Promises, allowing you to use “then” and “catch” methods.
- Modifying requests and responses: Fetch allows for customization in a separate configuration object.
- Error handling: Fetch considers a request successful as long as it completes, regardless of the HTTP status code returned.
Promise-Based Architecture
Axios uses JavaScript promises, making it simpler to write asynchronous code. This allows for the use of .then() and .catch() for handling successful and unsuccessful responses.
The promise-based architecture of Axios enables you to write more readable asynchronous code by utilizing async/await. This is a significant advantage over traditional callback-based approaches.
Axios provides a clean and simple syntax, making it easy to understand and use. It explicitly names HTTP methods through its API, like axios.get() or axios.post(), making the code self-explanatory.
Consider reading: Use Client Nextjs
Interceptors
Interceptors are a powerful feature that allows you to run your code or modify the request/response before it's handled by then or catch. This is especially useful for tasks such as attaching authentication tokens to requests and handling errors globally.
You can use interceptors to set global settings like headers, log information, or transform request and response data. Axios, in particular, provides a straightforward way to intercept and modify requests and responses. With Axios, you can specify settings such as the HTTP method to use, headers to include, and body data to send in a separate configuration object when calling the fetch() method.
Here are some key benefits of using interceptors:
- Attach authentication tokens to requests
- Set global headers
- Log information
- Transform request and response data
By using interceptors, you can simplify your code and make it more readable. You can also handle errors globally, which can be a big time-saver. For example, you can use a response interceptor to catch any errors that occur during the response and handle them accordingly.
In practice, interceptors can be used to create a custom Axios instance with request and response interceptors. This allows you to attach authentication tokens to requests and handle errors globally. By using interceptors, you can make your code more robust and easier to maintain.
Check this out: Nextjs Usecontext
Advantages and Use Cases
In Next.js, Axios has several advantages that make it a popular choice among developers. Axios provides a simpler syntax for beginners, making it easier to learn and use. This is especially true for new developers who are just starting out with web development.
Axios also comes with advanced features out-of-the-box, such as request and response interceptors, automatic JSON data transformation, and configurable timeouts. This can save developers a significant amount of time and effort.
One of the key benefits of using Axios is its strong community and ecosystem. With a large number of tutorials, guides, and third-party plugins available, developers can easily find solutions to common challenges and stay up-to-date with best practices.
Here are some scenarios where Axios might be a better choice:
- Rapid prototyping and development: Axios can save you time when you're working on a project with tight deadlines or just want to prototype an application quickly.
- Complex request and response manipulation: Axios makes intricate pre- or post-processing of requests and responses easier.
- Working with older browsers: Axios has a broader range of browser compatibility compared to Fetch. If your application needs to support older browsers, Axios is often the safer choice since it automatically includes polyfills.
- Advanced challenges: The robust community and ecosystem around Axios can be invaluable for projects where you anticipate running into challenges or needing specialized plug-ins.
In large-scale applications, Axios is often the better choice due to its advanced features and robust community support. However, for basic or small-scale applications, Fetch may perform better due to its native support and lower complexity.
Setup and Configuration
To set up Axios in a Next.js project, you can create a new project using `npx create-next-app my-app`. Then, install Axios by running `npm install axios`.
To create an Axios instance, you can use the `axios.create()` method. This instance allows you to define default configurations for your HTTP requests. You can set the `baseURL` to a centralized API endpoint, such as `config.API_HOST`, to avoid redundancy in your API URLs.
To configure Axios with interceptors, you can create a custom Axios instance with request and response interceptors. A request interceptor can check for a token in local storage and add it to the request headers, while a response interceptor can modify the response or handle errors globally.
Here are the key steps to create a custom Axios instance with interceptors:
- Creating an Axios instance with a base URL from environment variables.
- Adding a token from local storage to the request headers if available.
- Modifying the response or handling errors globally using a response interceptor.
Built-in CSRF Protection
Axios has a built-in mechanism to protect against Cross-Site Request Forgery attacks, enhancing the security of your applications.
This means you don't have to worry about manually implementing CSRF protection, saving you time and effort.
Axios takes care of this for you, so you can focus on building your application without worrying about security vulnerabilities.
Setting Up
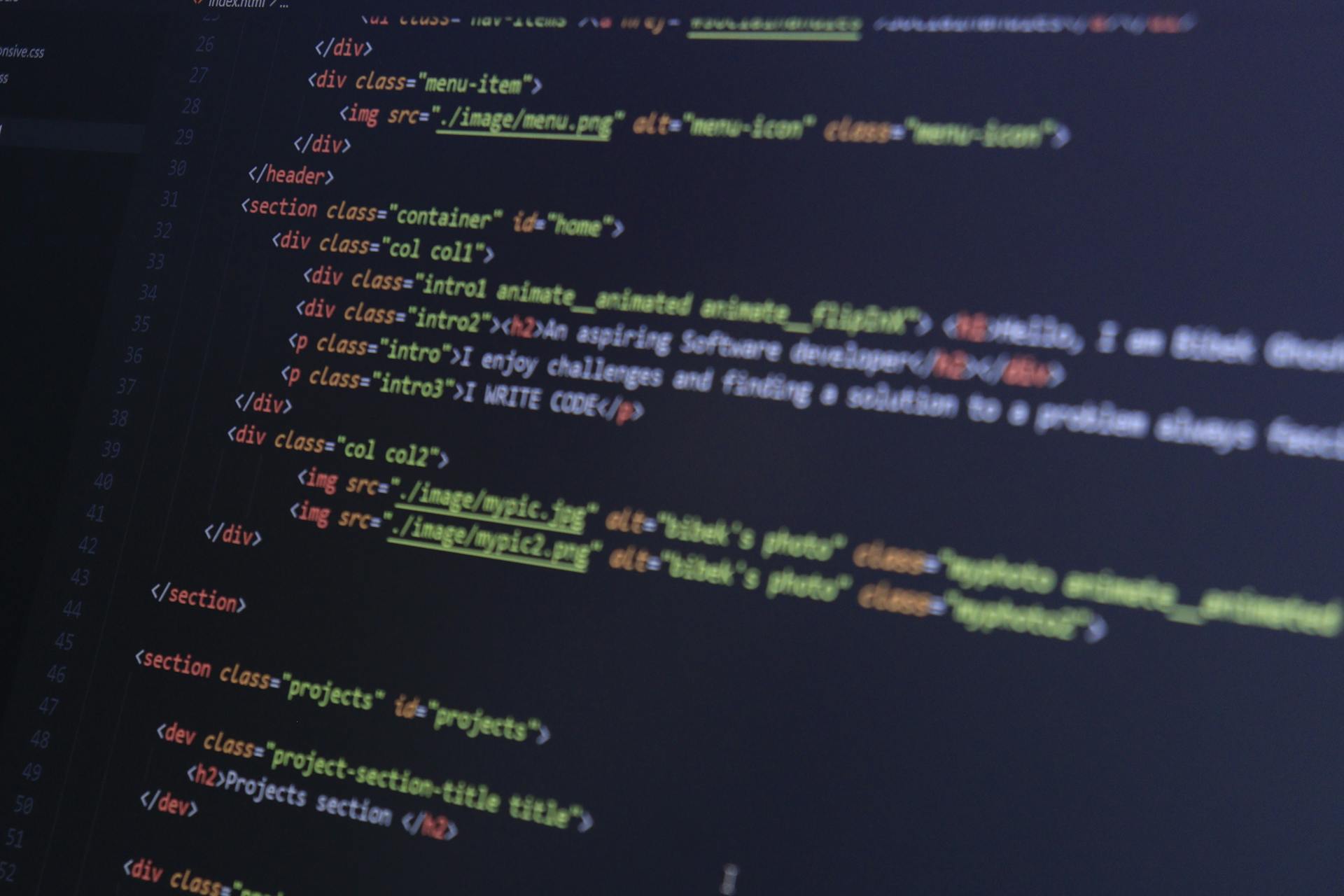
Setting Up Axios in a Next.js Project is a breeze. You can create a new project using `npx create-next-app my-app`, and then install Axios by running `npm install axios` or `yarn add axios`.
To get started with Axios in a Next.js project, you'll need to create a custom Axios instance. This can be done by creating a new file, such as `utils/axiosInstance.js`, where you can configure the instance with a base URL from environment variables.
A custom Axios instance can be created with request and response interceptors, which allow you to run code or modify the request/response before they are handled by `then` or `catch`. This is especially useful for tasks such as attaching authentication tokens to requests and handling errors globally.
You can also create a custom Axios instance by creating a new file, such as `./src/libs/utils/api.js`, where you can create an axios instance using `axios.create()`. This instance allows you to define default configurations for your HTTP requests.
Take a look at this: New Nextjs Project Typescript
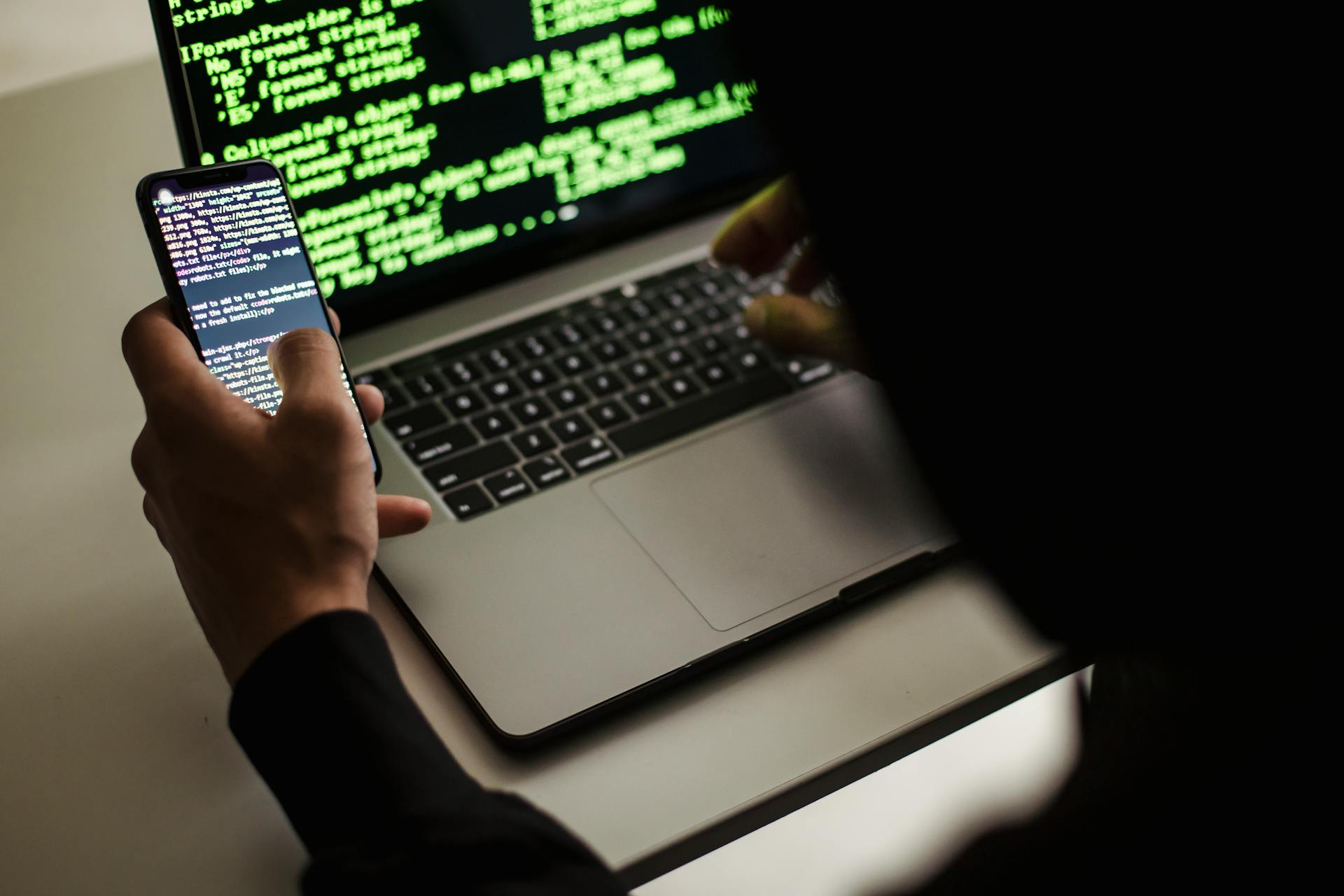
Here's a summary of the steps to create a custom Axios instance:
- Create a new file, such as `utils/axiosInstance.js`, to configure the instance with a base URL from environment variables.
- Create a new file, such as `./src/libs/utils/api.js`, to create an axios instance using `axios.create()`.
- Define default configurations for your HTTP requests, such as setting a base URL and timeout.
- Use the custom Axios instance in your Next.js components or pages to make API requests.
By following these steps, you can easily set up Axios in a Next.js project and start making API requests with ease.
Data
Data is a crucial aspect of any application, and setting it up correctly can make a huge difference in how your app performs. You can use Axios to intercept requests and responses, which is useful for setting global settings like headers or logging information.
Axios automatically parses JSON responses, so you don't need to call .json(). This feature can save you a lot of time and effort when working with JSON data.
Axios also supports request and response interceptors, allowing you to modify requests or responses globally. This is a powerful feature that can be used for a variety of tasks, such as adding authentication tokens to requests or logging responses and errors.
Here are some key benefits of using Axios for data fetching:
- Automatic JSON Parsing: Axios automatically parses JSON responses, eliminating the need for .json() calls.
- Automatic Error Handling: Axios automatically rejects the promise for HTTP errors (non-2xx responses), simplifying error handling.
- Simpler Configuration: Axios provides a more intuitive API for setting up headers, query parameters, and other request options.
- Interceptors: Axios supports request and response interceptors, allowing you to modify requests or responses globally.
Sources
- https://scrapingrobot.com/blog/fetch-vs-axios-in-next-js/
- https://sandeepbansod.medium.com/effortless-api-request-handling-in-next-js-with-axios-a-comprehensive-guide-8b424ce403c5
- https://www.getfishtank.com/insights/comprehensive-guide-to-data-fetching-in-nextjs
- https://devsarticles.com/fetch-vs-axios-in-next-js-complete-guide
- https://javascript.plainenglish.io/effortless-next-js-data-fetching-with-swr-and-axios-a197517af7c1
Featured Images: pexels.com