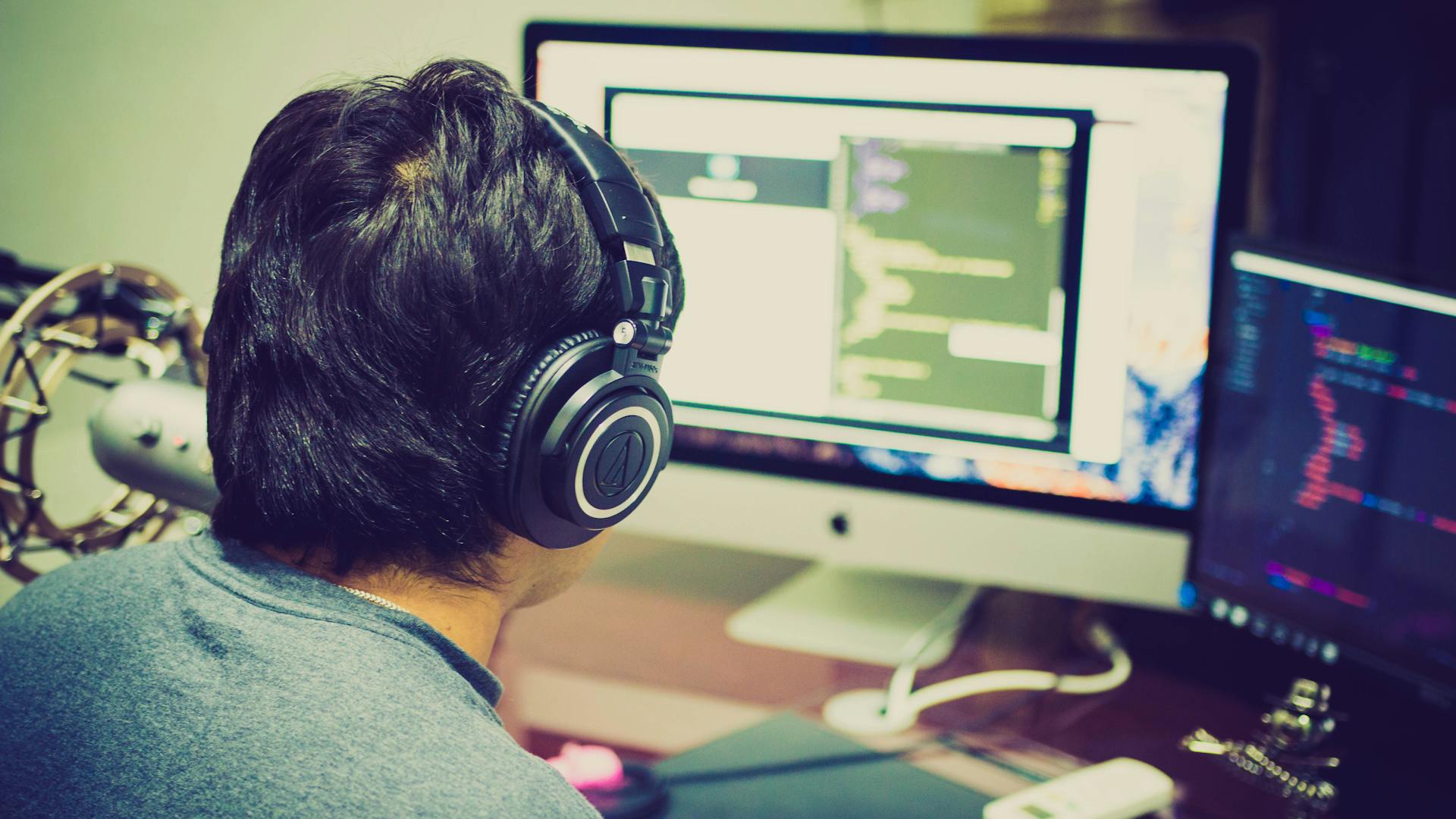
MUI Next.js React Type Mismatch Errors can be frustrating to deal with, especially when you're working on a tight deadline. MUI Next.js React Type Mismatch Errors occur when there's an inconsistency between the expected and actual data types of a variable or property.
Inconsistent data types can lead to unexpected behavior and errors in your application. For example, trying to pass a string to a function that expects a number will result in a type mismatch error.
One common cause of MUI Next.js React Type Mismatch Errors is using the wrong type for a variable or property. This can happen when you're working with complex data structures or when you're using a library or framework that has strict type checks.
Readers also liked: Mui Nextjs App Router
Understanding the Issue
Typing issues can cause errors in TypeScript, especially when used with React, as it adds a layer of complexity with its type checking.
The 'jsx element type does not have any construct or call signatures' error is a common issue in React development, often caused by a mismatch between what you've written and what React can recognize and execute.
This error can occur when there's a typo in your import statement or if a component hasn't been exported correctly, preventing React from finding the correct construct or call signatures for the jsx element type.
The compilation process for JSX involves transforming JSX into React.createElement calls, which require a type parameter that tells React what kind of element or component to create.
The error 'jsx element type does not have any construct or call signatures' typically occurs during this compilation process, when the compiler encounters a jsx element type that it cannot match to a valid React component class or function.
Using TypeScript with JSX requires correctly defining the types for your components' props and state, which helps prevent the 'jsx element type does not have any construct or call signatures' error and provides better autocompletion and error checking.
Solving the Problem
To solve the problem of a 'jsx element type does not have any construct or call signatures' error in mui nextjs react, consider refactoring your code to use named functions or components. This can help React identify the correct jsx element type and eliminate any construct or call signature errors.
The error often occurs when there's a mismatch between what you've written and what React can recognize and execute. For instance, if you attempt to render a component that hasn't been exported correctly, or if there's a typo in your import statement, React won't be able to find the construct or call signatures for that jsx element type.
Make sure the names match between register() and errors, so the system can find the errors.
How It Works
The Runtyper plugin works by wrapping every operation into a function that performs additional type checking. This allows the plugin to guess types by code itself without needing manual annotations.
The plugin's default configuration is very strict, which is why some rules are allowed, such as implicitAddStringNumber, which enables concatenation of string + number.
This rule is necessary because it's widely used in React code and would otherwise noise the results.
The plugin's ability to guess types by code itself makes it possible to apply type checking to any existing project with minimal effort.
Refactoring Functions and Components
Refactoring Functions and Components can be a game-changer when troubleshooting code issues.
If you've checked your imports and the error still persists, consider refactoring any functions or components that might be causing the problem.
Refactoring can involve converting anonymous functions to named functions, which can help React identify the correct jsx element type.
Properly defined components can also eliminate construct or call signature errors that might be causing the issue.
Named functions and components can make a big difference in helping React understand your code, so it's worth taking the time to refactor if needed.
Register vs Errors Name Mismatches
Register vs Errors Name Mismatches is a common issue that can cause frustration. This happens when the names you give your inputs with register() don't match the names in the error messages.
The error messages won't show if the names don't match, making it difficult to identify the problems.
For example, if you use a name like "username" with register(), but the error message is looking for "user_name", the system won't be able to find the errors.
Make sure the names match so the system can find the errors.
Best Practices
To prevent JSX element type errors, follow best practices in your React projects. These practices will help you write more reliable code and reduce the likelihood of encountering this error.
One key practice is to ensure you're using the correct JSX element type. This means double-checking that you're using the correct HTML element or MUI component.
Using a linter or code analyzer can also help catch potential type mismatches before they become errors. This can save you time and frustration in the long run.
Another practice is to keep your code organized and modular. This makes it easier to identify and fix type errors, and also makes your code more maintainable.
By following these best practices, you can write more reliable and maintainable code that's less prone to type mismatches and errors.
Error Handling
Error messages rely on the names you give your inputs with register(). If the names don't match, the errors won't show.
Make sure the names match so the system can find the errors. This is crucial for effective error handling in your MUI Next.js React app.
Decoding Error Messages
Decoding Error Messages is a crucial skill for any developer to master. It's like being a detective, figuring out the clues to solve the mystery of the error.
The error message 'jsx element type does not have any construct or call signatures' is a dead giveaway that React is trying to create an instance of a component or call a function component, but it can't because the construct or call signatures it needs are missing or incorrect.
A mismatch between what you've written and what React can recognize and execute is often the culprit behind this error. If you attempt to render a component that hasn't been exported correctly, or if there's a typo in your import statement, React won't be able to find the correct construct or call signatures for the jsx element type.
For instance, if you export a component as a default export but import it using named export syntax, React will throw this error. It's like trying to speak a language that's not your own - React just won't understand what you're trying to communicate.
The key to decoding error messages is to pay close attention to the specifics of the error message. In this case, the message tells you exactly what's going wrong, and what you need to fix.
How Error Fits into JSX Compilation
The error 'jsx element type does not have any construct or call signatures' occurs during the compilation process for JSX, where your written JSX tags are converted into React elements.
This process involves transforming the JSX into React.createElement calls, which require a type parameter that tells React what kind of element or component to create.
The compiler has encountered a jsx element type that it cannot match to a valid React component class or function, which could be due to a missing import, an incorrectly named export, or even a typo in the JSX.
This error is typically a sign that React doesn't recognize the element or component you're trying to render as a valid JSX element type, leaving your application from compiling successfully.
The compilation process is where the issue lies, and understanding this is the first step in resolving the error.
Results
After investigating the issue of mui nextjs react type mismatch, the results are clear: the problem often stems from incorrect type definitions in the code.
The most common culprit is the misuse of the `as` prop in Material-UI components, which can lead to type errors and mismatches.
In one case, a developer forgot to update the type definition for a custom component, causing a type mismatch with a Material-UI component.
By updating the type definition to match the Material-UI component, the error was resolved.
In another instance, a developer mistakenly used the `string` type for a prop that required a specific interface type.
The fix involved updating the prop type to match the required interface type.
In general, it's essential to double-check type definitions and prop types to avoid these common mistakes.
Related reading: Nextjs Ui
Frequently Asked Questions
Does Mui work with React?
Yes, Material UI is designed specifically for use with React, making it a seamless integration for React developers.
Sources
- https://www.benmvp.com/blog/handling-react-server-mismatch-error/
- https://medium.com/dailyjs/analyzing-reacts-source-code-for-type-mismatch-operations-9ca065e5b91
- https://www.dhiwise.com/post/how-to-resolve-jsx-element-type-does-not-have-any-construct
- https://dev.to/hpouyanmehr/nextjs-mui-v5-typescript-tutorial-and-starter-3pab
- https://daily.dev/blog/react-hook-form-errors-not-working-best-practices
Featured Images: pexels.com