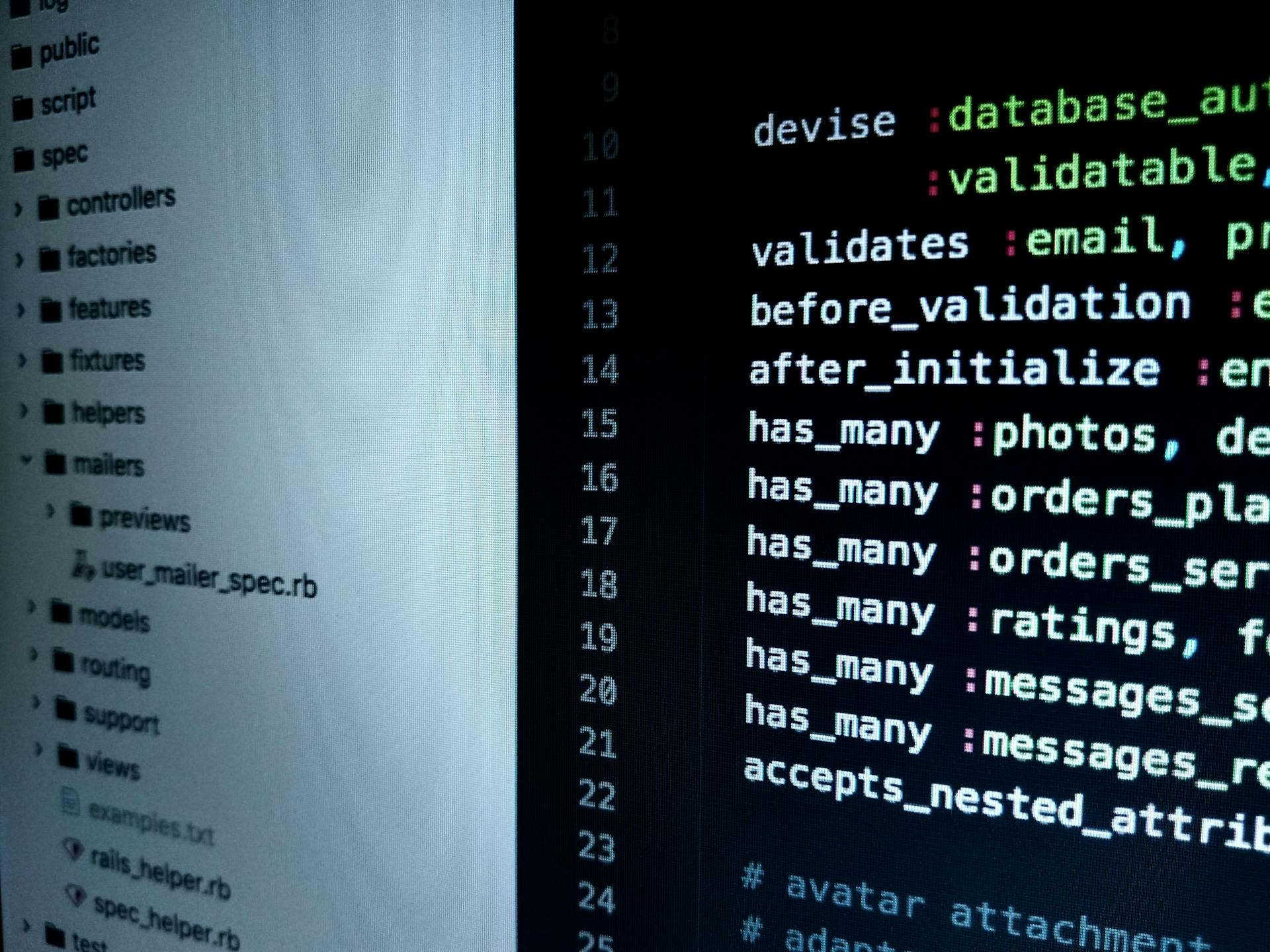
Integrating Knex with Next.js is a game-changer for efficient data management.
By using Knex with Next.js, you can simplify your database interactions and focus on building a robust application.
With Knex, you can create a unified interface for interacting with your database, reducing the complexity of managing multiple database connections.
This integration also enables you to write database-agnostic code, making it easier to switch between different databases if needed.
By leveraging Knex's powerful features, you can streamline your database operations and improve the overall performance of your Next.js application.
Recommended read: Nextjs Spa
Database Operations
To get data from a database server-side in Next.js, you create a getServerSideProps() function that imports the db function from your database file and uses it to retrieve data.
You can create a select query using the .select() method, which takes an optional array of columns for the query, defaulting to * if none are specified. This returns an array of objects selected from the database.
To insert data into a database, you can use the .insert() method, which takes a hash of properties to be inserted into the row, or an array of inserts. If you want to return the added rows with specified columns, you can pass an array to the returning argument.
A unique perspective: Nextjs Cookies
Get Data from Database
Getting data from a database is a crucial part of any application. Next.js allows you to get data server-side using the getServerSideProps() function.
To establish a reliable connection between your Next.js app and a MySQL database, you can create a getServerSideProps() function that imports the db function from a file and uses it to retrieve data from the database.
You can create a select query using the .select() method, which takes an optional array of columns for the query, eventually defaulting to * if none are specified. This method returns an array of objects selected from the database.
The select query will resolve with an array of objects, which can be inferred based on the columns being selected, as long as the select arguments match exactly the key names in the record type.
If you want to retrieve specific columns, you can pass an array of columns to the .select() method, like this: .select(['id', 'text']). This will return an array of objects with only the id and text columns.
To insert data into the database, you can use the .insert() method, which takes a hash of properties to be inserted into the row, or an array of inserts, to be executed as a single insert command. This method returns the added rows with specified columns.
Recommended read: Next Js Getserversideprops
Join Raw
You can use a raw query with Knex.js to execute SQL commands, and it's a convenient way to specify joins between tables.
The join builder in Knex.js can be used to specify joins between tables, with the first argument being the joining table, the next three arguments being the first join column, the join operator, and the second join column, respectively.
To join tables, you can use the join method, passing in the joining table, the first join column, the join operator, and the second join column, like this: join(table, first, [operator], second).
You can also use a function as the second argument for the join query, and use on with orOn or andOn to create joins that are grouped with parentheses.
For nested join statements, you can specify a function as the first argument of on, orOn, or andOn.
To use a literal value in a join instead of a column, use knex.raw.
The rightJoin method is also available, which is similar to join but joins on the right side of the table.
Upsert
Upsert is a powerful database operation that allows you to insert new data while updating existing records, all in a single command.
The .upsert method is implemented for CockroachDB and creates an upsert query by taking a hash of properties or an array of upserts to be executed as a single command.
You can pass an array of upserts to execute multiple updates in a single command, making it a convenient option for bulk operations.
If you need to retrieve the added rows, you can pass the returning array with the desired columns, such as 'id' and 'title', to get the updated data.
The .update method is also used for upsert operations, where you can specify a key and value to update, or use the .update(data) method to update multiple records at once.
The returning array can be used to retrieve the updated data, just like in the .upsert method.
For another approach, see: Nextjs App Route Get Ssr Data
Row Number
Row Number is a function that adds a row_number() call to your query. It's a powerful tool for ordering and partitioning data.
You can use Row Number with a string syntax, which includes an alias, an orderByClause, and an optional partitionByClause. The alias can be set to a falsy value if not needed.
The Raw Syntax allows you to use a rawQuery, which can be a complex query. This syntax is useful when you need to perform a specific query.
Function Syntax is also available, which takes a function as an argument. This syntax is flexible and allows you to use a custom function to generate the row numbers.
In practice, I've found that using Row Number can greatly simplify complex queries and improve performance. By adding a row_number() call, you can easily order and partition your data.
Expand your knowledge: Query in Nextjs
Column Info
Column Info is a crucial aspect of understanding your database. You can retrieve information about a column or the entire table using the .columnInfo() method.
The method returns an object with various keys, including defaultValue, type, maxLength, and nullable. This information can be useful for understanding the structure of your database.
For example, if you want to know the default value for a specific column, you can use the .columnInfo(columnName) method. This will return an object with the default value, type, maxLength, and nullable information for that column.
Here's a breakdown of the information you can expect to receive from the .columnInfo() method:
Understanding the column info can help you make informed decisions about your database design and operations.
Where Not Exists
In a database, "Where Not Exists" is a clause used in SQL queries to return records that do not exist in another table.
This clause is particularly useful when you need to find records that are not linked to another table, such as finding customers who do not have any orders.
For example, if you have a table of customers and another table of orders, you can use "Where Not Exists" to find customers who do not have any orders.
Expand your knowledge: Next Js Table
The syntax for this clause is similar to the "Where Exists" clause, but with a "Not" keyword added to negate the condition.
In the context of database operations, "Where Not Exists" is a powerful tool for finding missing links between tables.
By using this clause, you can identify records that are not related to other tables, which can be useful for data analysis and reporting.
For instance, if you have a table of employees and another table of departments, you can use "Where Not Exists" to find employees who do not belong to any department.
Query Optimization
Query Optimization is a crucial aspect of building high-performance applications with Knex and Next.js.
Using Knex's built-in query builder, you can optimize queries by reducing the number of database calls.
For example, with Knex's `whereIn` method, you can fetch multiple records with a single database call.
This can significantly improve the performance of your application, especially when dealing with large datasets.
By leveraging Knex's query optimization techniques, you can create more efficient and scalable applications.
Broaden your view: Nextjs Performance
On Conflict
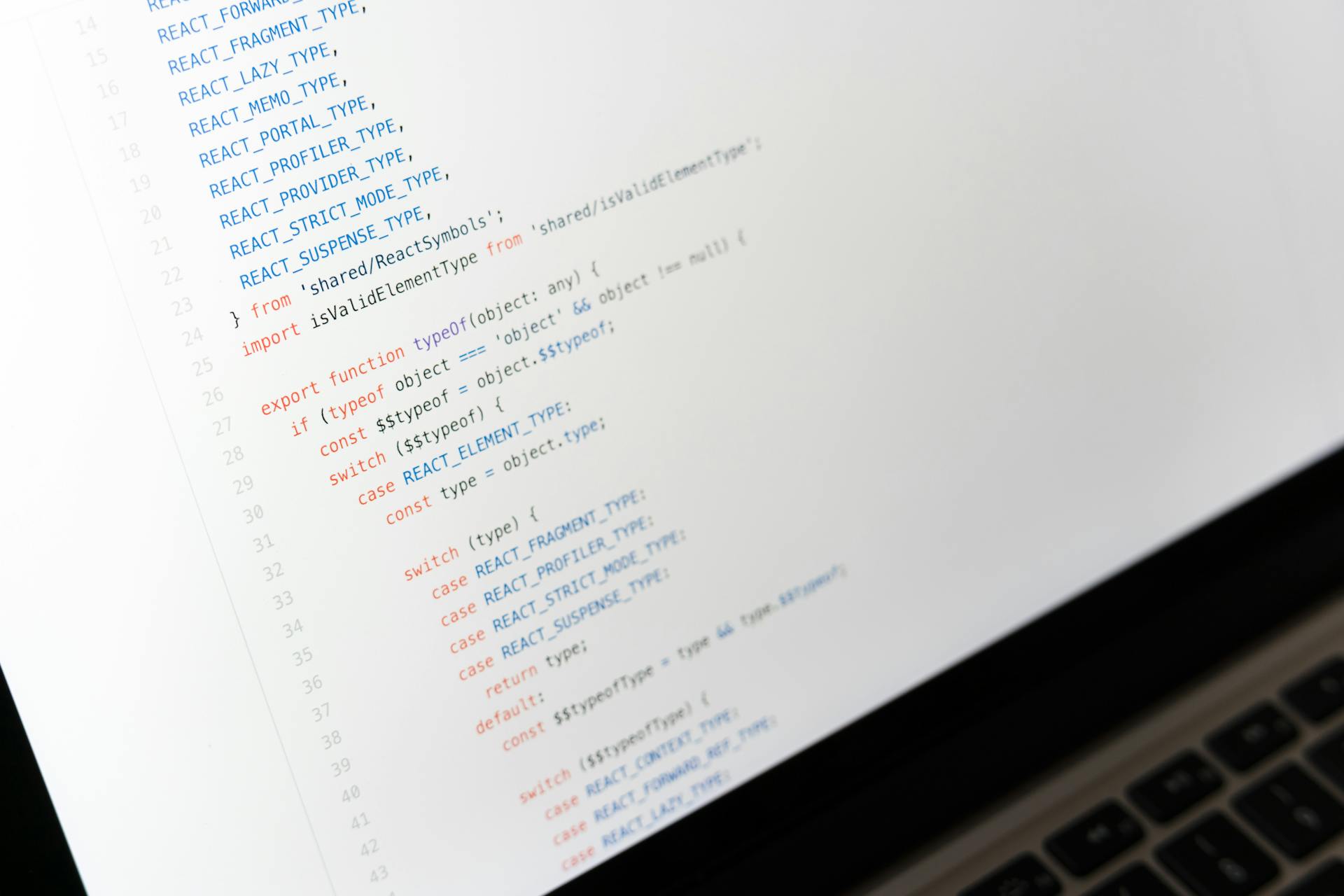
The onConflict modifier is implemented for PostgreSQL, MySQL, and SQLite databases. It specifies alternative behavior in case of a conflict, which occurs when a table has a PRIMARY KEY or a UNIQUE index on a column and a row being inserted has the same value as an existing row.
A conflict can be resolved by silently ignoring the error or updating the existing row with new data. This is achieved by using .onConflict().ignore() or .onConflict().merge() respectively.
You must specify the columns that will be used to resolve the conflict, which can be the table's PRIMARY KEY or have a UNIQUE index on them. For PostgreSQL and SQLite, this is a requirement, while MySQL will ignore the specified columns and always use the table's PRIMARY KEY.
In PostgreSQL and SQLite, you can use knex.raw(...) function to specify a condition when you have a partial index.
Memoize
Memoize is a technique that can help optimize queries by storing the results of expensive function calls and reusing them when the same inputs occur again. This can be particularly useful in a Next.js environment where the db injector might get reinitialized.
To implement memoization, you can use a simple variable to store the connection instance, as shown in the example. The variable cachedConnection is created to store the connection instance, and if it doesn't exist, a new one is created and referred to by it.
The cached connection is almost always returned to the handler, but it's not a guarantee due to potential reinitialization of the db injector. This can lead to a connection hanging out with Knex for longer than intended.
Group By Raw
In database querying, the ability to group data by specific criteria is crucial for obtaining meaningful insights.
The .groupByRaw method is a powerful tool for achieving this.
It allows you to add a raw group by clause to the query, giving you fine-grained control over the grouping process.
This can be particularly useful when working with complex queries or data sets that require custom grouping logic.
The method takes a single argument, sql, which specifies the raw group by clause to be added to the query.
This means you can write custom SQL code to define the grouping criteria, giving you maximum flexibility and control.
Explore further: Nextjs Neon Sql
OrderBy
The OrderBy clause is a powerful tool for sorting query results. It can be added to a query using the orderBy function.
The column or columns to be ordered can be specified as a string or a list of strings and objects. This flexibility makes it easy to order multiple columns.
The direction of the order can be specified, but it's not required. The nulls option can be used to determine where null values are placed in the sorted order.
In a single column order, the column can be a string.
Featured Images: pexels.com