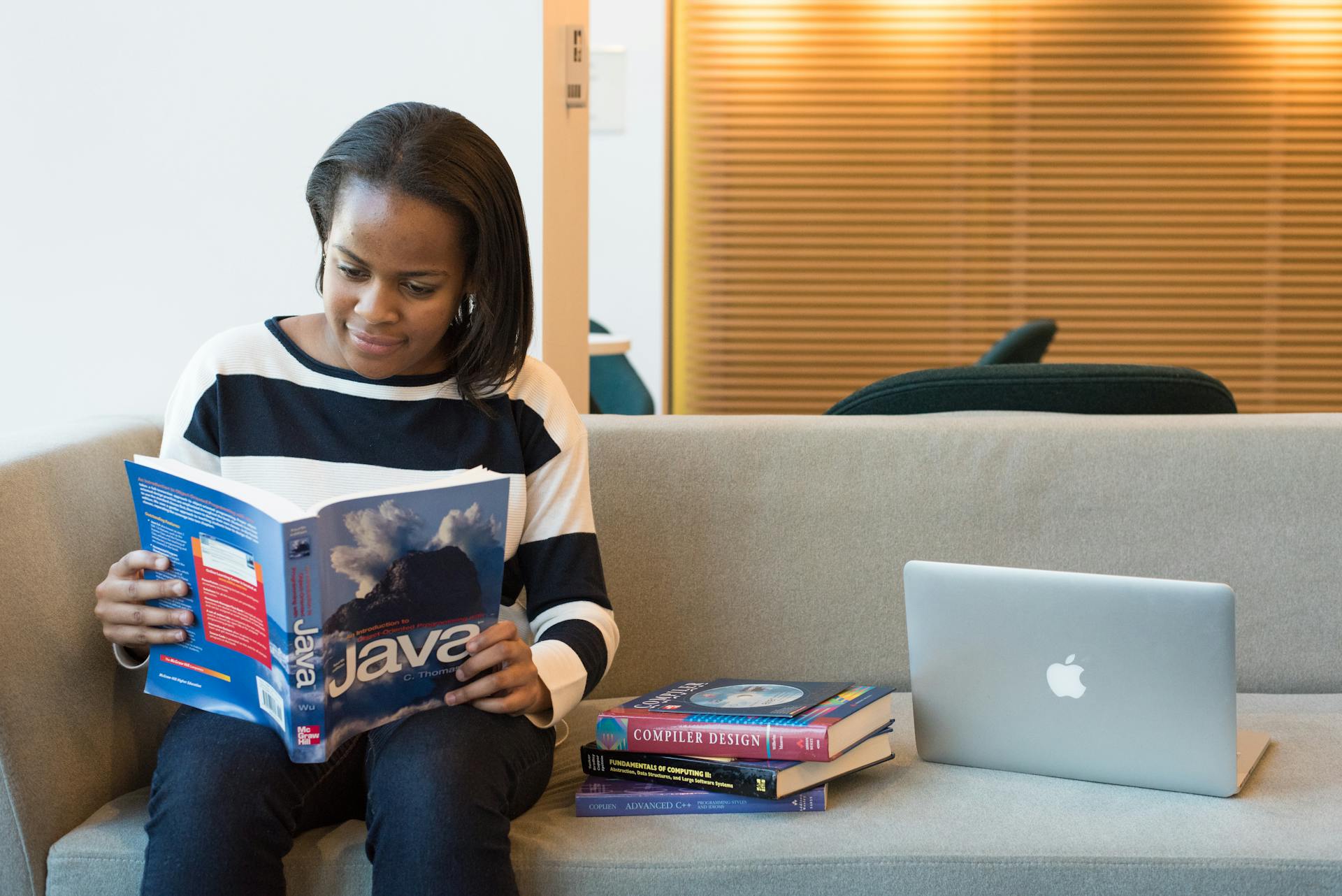
Here's the intro section for the article:
Maven is a popular build tool for Java projects that allows for easy management of dependencies and reproducible builds.
To host these artifacts securely, you can use AWS S3, a cloud-based object storage service that provides a highly durable and available storage solution.
AWS S3 offers a scalable and cost-effective solution for storing and serving artifacts, making it an ideal choice for Java Maven projects.
By integrating Maven with AWS S3, you can ensure that your artifacts are stored securely and can be easily accessed by your team and other stakeholders.
Worth a look: Aws S3 Storage Cost
Setting Up AWS S3
To set up AWS S3, start by creating a bucket and adding objects to it. You can find instructions on how to do this by visiting a specific link.
Creating a Maven project is the next step, which can be done using a command that generates a Maven structure importing the necessary extensions, including the S3 Client extension. This will add the amazon-s3 extension to your pom.xml file.
The default setting for the maximum body size in Quarkus is 10,240K, which may limit your ability to upload multipart files larger than this size. If you need to upload larger files, you'll need to set this limit explicitly.
To create a JAX-RS resource, you'll need to define a configuration property for the name of the S3 bucket, which will be required by the request method. This can be done by defining a property like bucket.name in your resource class.
Here's a quick rundown of the configuration properties you may need to set:
- bucket.name: The name of the S3 bucket
- quarkus.http.limits.max-body-size: The maximum size of the body in bytes (default is 10,240K)
By following these steps and setting up the necessary configuration properties, you'll be well on your way to using AWS S3 with your Java Maven package.
Project Configuration
To get started with a Java Maven package for AWS S3, you'll need to configure your project. Add the S3 SDK to your project by including the following dependency, which will pull the S3 code from Maven Central and make it available for use in your local environment.
The default setting for quarkus.http.limits.max-body-size is 10240K, which may limit your ability to upload multipart files larger than the default. To upload larger files, you'll need to set this limit explicitly.
You can use the following command to create a Maven project, which will generate a Maven structure importing the RESTEasy Reactive/JAX-RS and S3 Client extensions. This will also add the amazon-s3 extension to your pom.xml.
Here are the steps to follow:
- Step 1: S3 Config
- Step 2: S3 Service
- Step 3: S3 Controller
Project Development
To start developing your project, you'll need to add the S3 SDK to your project by adding the following dependency, which will pull the S3 code from Maven Central.
The default setting for quarkus.http.limits.max-body-size is 10240K, which may limit your ability to upload multipart files larger than the default.
To create an application to manage a list of files, you'll need to follow these steps: S3 Config, S3 Service, and S3 Controller.
Here's a breakdown of each step:
- S3 Config: Set up the S3 Client to use in service.
- S3 Service: Create a service to request a list of all objects in a bucket.
- S3 Controller: Receive web requests to list all objects.
After completing these steps, start your application and head over to `http://localhost:8080/file/listAllObjects` in your browser to see a list of all your AWS Bucket objects.
This will list all your AWS Bucket objects, including the type and name of each object, such as an image of type png called "word-cloud".
You might like: Aws S3 Listobjects
Client Encryption Options
Let's dive into the world of client encryption options for your AWS S3 storage. You have three primary options to consider: SSE-S3, SSE-C, and SSE-KMS.
SSE-S3 is a fully-managed encryption option that shifts the trust onto AWS, requiring no work from you. Your keys are created and managed on your behalf.
SSE-C allows you to generate or provide your own key that S3 will implement, providing a layer of separation between AWS and your key generation infrastructure.
SSE-KMS is a hybrid option that lets you own a master key while still allowing AWS to perform cryptological functions on your behalf and manage data keys.
Here are the three options in a concise list:
- SSE-S3: Fully-managed encryption with AWS creating and managing keys.
- SSE-C: Customer-managed keys with S3 implementing your provided key.
- SSE-KMS: Customer-provided master key with AWS performing cryptological functions.
Note that when configuring the S3EncryptionClient builder, you'll need to use the KMSEncryptionMaterialsProvider.
Packaging and Deployment
Packaging your Java application is a straightforward process. Simply run ./mvnw clean package in your terminal.
This command will create a JAR file that can be run with java -jar target/quarkus-app/quarkus-run.jar.
If you're using a native executable, you'll need to install GraalVM first. Then you can create a native executable binary by running ./mvnw clean package -Dnative, which will take some time depending on your system.
To use the AWS CRT-based S3 client, you'll need to add the io.quarkiverse.amazon.s3.runtime.S3Crt qualifier.
Broaden your view: Aws Glue Create Table from S3
Sources
- https://www.geeksforgeeks.org/how-to-list-all-aws-s3-objects-in-a-bucket-using-java/
- https://codetinkering.com/aws-s3-encryption-java-api/
- https://docs.quarkiverse.io/quarkus-amazon-services/dev/amazon-s3.html
- https://medium.com/axel-springer-tech/using-an-aws-s3-bucket-as-a-maven-repository-sounds-juicy-but-is-it-worth-the-effort-6be1578b25b
- https://dzone.com/articles/host-your-maven-artifacts-using-amazon-s3
Featured Images: pexels.com