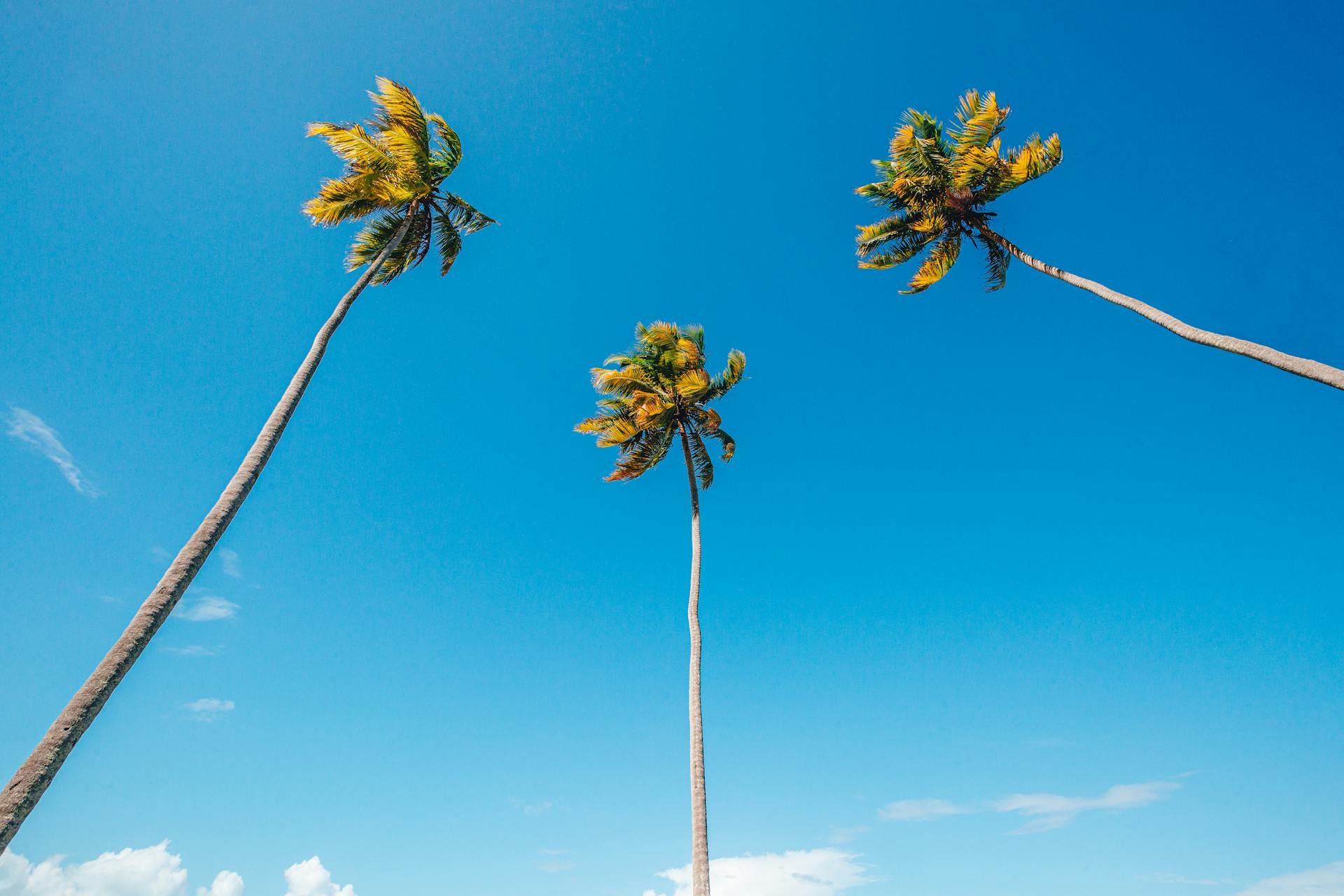
Ionic Azure integration is a game-changer for mobile app developers. By leveraging Azure Active Directory (AAD), you can easily implement secure login and user management in your Ionic apps.
This integration allows for seamless authentication, eliminating the need for users to remember multiple passwords. Azure's robust security features also ensure that user data is protected.
With Ionic Azure integration, you can take advantage of Azure's scalable and reliable infrastructure to store user data. This means your app can handle a large number of users without compromising performance.
By using Azure's user management features, you can easily manage user roles and permissions, ensuring that the right users have access to the right features.
Discover more: Azure Data Studio Connect to Azure Sql
Authentication
Authentication is a crucial aspect of building a secure Ionic app. You can create an Authentication Service for login functionality using Azure AD environment configurations added to the Ionic app.
To start, generate an AuthenticationService class using the ionic generate command. This class will encapsulate Azure AD and Ionic Auth Connect's login functionality.
Consider reading: Azure Auth Json Website Azure Ad Authentication
Open up src/app/services/authentication.service.ts and import Auth Connect, RxJs, and the Azure AD configuration objects. Extend the IonicAuth class to gain access to Auth Connect's functionality and instantiate it with the proper Azure configuration object.
Implementing login functionality is quite simple - define your own login method and call Auth Connect's login method. Upon successful login, Auth Connect calls the onLoginSuccess event, which you can override to run any additional logic.
To detect and act on the login status changing, subscribe to the status change observable in app.component.ts. Add a method that inspects the status of the login attempt and redirect the user accordingly.
When running as a web app, the user will be redirected to the Azure AD sign-in page. After successful sign-in, pass the browser's url to Auth Connect's handleCallback function to complete the login process.
To deploy to mobile, install Capacitor and run the following command to install the Auth Connect plugin. For the AUTH_URL_SCHEME variable, use the globally unique App Id you decided on when configuring the Azure AD app.
Configuration Details
To configure Azure Notification Hubs in your Ionic app, you'll need to add the cordova-azure-notification-hubs plugin to your project. This plugin allows your app to register for and process push notifications with Azure Notification Hubs.
The Capacitor Push Notifications plugin can intercept notifications, so you'll need to use both the cordova-azure-notification-hubs plugin and the Capacitor Push Notifications plugin in your Ionic 4 app to automatically register for and receive notifications.
Here are the configuration details you'll need to add to your Ionic app:
- clientID: Your app's Application (client) ID, found on the Overview page.
- redirectUri: The URI to redirect to after the user has logged in. Use "http://localhost:8100/login" for testing locally in a browser.
- scope: The scope property is used to unlock access to protected resources, such as read/write permissions. You'll need to add the Full Scope Value link created earlier to this property.
- logoutUrl: The URI to redirect to after the user has logged out. Use "http://localhost:8100/".
You can find the Full Scope Value link in the Azure AD B2C portal by navigating to the "Expose an API" page and clicking on the Scope you defined earlier.
Build and Deploy
To create a build pipeline in Azure DevOps, you can use the Azure Pipelines feature. This will allow you to automatically build, test, and deploy your App.
First, you'll need to select New pipeline or Create Pipeline, and then choose Github as the source code option. You can then choose to start from scratch and select Starter Pipeline.
The minimum pipeline structure will be displayed, and you can delete all lines and replace them with the following code to install, build, and test your App. This will generate the www artifact whenever it is successfully executed.
You can rename this pipeline to App Build CI by clicking Edit → Three dots → Triggers → YAML and editing the Name field. The build specification is responsible for installing, building, and testing the App, and generates the www artifact.
Here's a summary of the pipeline structure:
After creating the build pipeline, you can save and run it to see the build output and errors on your Azure DevOps dashboard.
Add Web Platform
To add a web platform to your app, navigate to Manage - Authentication and click the “Add a Platform” button. Here, you'll choose “single-page application” under Web applications.
Specify a web URL for Redirect URIs, such as http://localhost:8100/ along with your app's core login page name. For local testing, this is a good starting point.
Here's an interesting read: Microsoft Azure Websites
Under Logout URL, specify a web URL to redirect to once the user has logged out, like https://localhost:8100/along with your logout page name. This ensures a smooth logout experience.
Toggle “Access tokens” under Implicit Grant and click Configure to save your settings. This step is crucial for securing your app's authentication process.
You can add additional Redirect URIs by clicking the “Add URI” button under Single-page application settings. This allows you to set up different environments like staging or production.
Build and Deploy Pipelines
To create build and deploy pipelines, you can use Azure DevOps, which provides Azure Pipelines to automate the process. Azure Pipelines can be used to create three pipelines to build, test, and deploy your App.
You can create a Build and Release Pipeline in Azure DevOps, where the Build Pipeline generates one or more artifacts using the source code of the project, and the Release Pipeline deploys these artifacts.
Recommended read: Azure vs Azure Devops
To create a Build Pipeline, select New pipeline or Create Pipeline, and choose Github as the source code option. Then, select Starter Pipeline and delete all lines to add your own build specification. This pipeline will install, build, and test your App, generating the www artifact whenever it is successfully executed.
Here are the steps to create a Build Pipeline:
- Select New pipeline or Create Pipeline
- Select Github as the source code option
- Select Starter Pipeline
- Delete all lines and add your own build specification
You can also use Azure Pipelines to create a Release Pipeline, which deploys the artifacts generated by the Build Pipeline. To create a Release Pipeline, you can use the Azure Pipelines app, which will redirect you to the Github page to accept and install the app.
After creating the pipeline, you can save it and run it to see the build output and errors on your Azure DevOps dashboard.
For more insights, see: Azure App Insights vs Azure Monitor
User Management
Ionic Azure offers a robust user management system that simplifies the process of managing users for your application.
User roles and permissions are easily managed through the Ionic Azure portal, allowing you to assign specific roles and permissions to users based on their needs. This ensures that users have access to the features and data they require, while preventing unauthorized access to sensitive information.
By leveraging Ionic Azure's user management capabilities, you can streamline user onboarding and reduce the administrative burden associated with managing users.
Authentication Service for Login
To create an authentication service for login functionality, you can generate an AuthenticationService class using the ionic generate command. This class will encapsulate Azure AD and Ionic Auth Connect's login functionality.
The AuthenticationService class will need to import Auth Connect, RxJs, and the Azure AD configuration objects. It will also need to extend the IonicAuth class to access Auth Connect's functionality.
The login functionality is implemented by defining a login method that calls Auth Connect's login method. Upon successful login, Auth Connect calls the `onLoginSuccess` event, which can be overridden to run additional logic.
To detect and act on the login status changing, you can subscribe to the status change observable in the app.component.ts file. This will allow you to redirect the user to the Home page if the login is successful, or remain on the Login page if the login fails.
Here are the steps to implement the login functionality:
1. Import the AuthenticationService class in the Login page (src/app/login/login.page.ts).
2. Add a click handler to the Login button to call the AuthenticationService's login method.
3. Pass the browser's URL to Auth Connect's `handleCallback` function to handle the callback after successful sign-in.
By following these steps, you can create an authentication service for login functionality that integrates with Azure AD and Ionic Auth Connect.
View User Details
Viewing user details can be a game-changer for user management.
To display user profile details on the home page, you'll need to declare a new method in the AuthenticationService to retrieve the user's profile info from Azure.
Accessing all information via Auth Connect's `getIdToken` function is a key step in this process.
On the Home page, make the call to `getUserInfo` when the page loads.
Update the Home page's HTML template to display the user's profile info.
Sources
- https://techcommunity.microsoft.com/t5/microsoft-developer-community/using-azure-notification-hubs-in-apache-cordova-and-ionic-apps/ba-p/1056685
- https://techcommunity.microsoft.com/t5/apps-on-azure-blog/adding-azure-ad-b2c-login-to-a-hybrid-mobile-app-using-ionic/ba-p/1563740
- https://ionic.io/docs/appflow/cli/examples/azure-devops
- https://dev.to/carlosgit2016/creating-building-and-deploying-an-ionic-application-using-azure-devops-and-app-center-1bhj
- https://enxhileba.medium.com/how-to-login-ionic-app-with-azure-cloud-adb2c-3a48844316b4
Featured Images: pexels.com