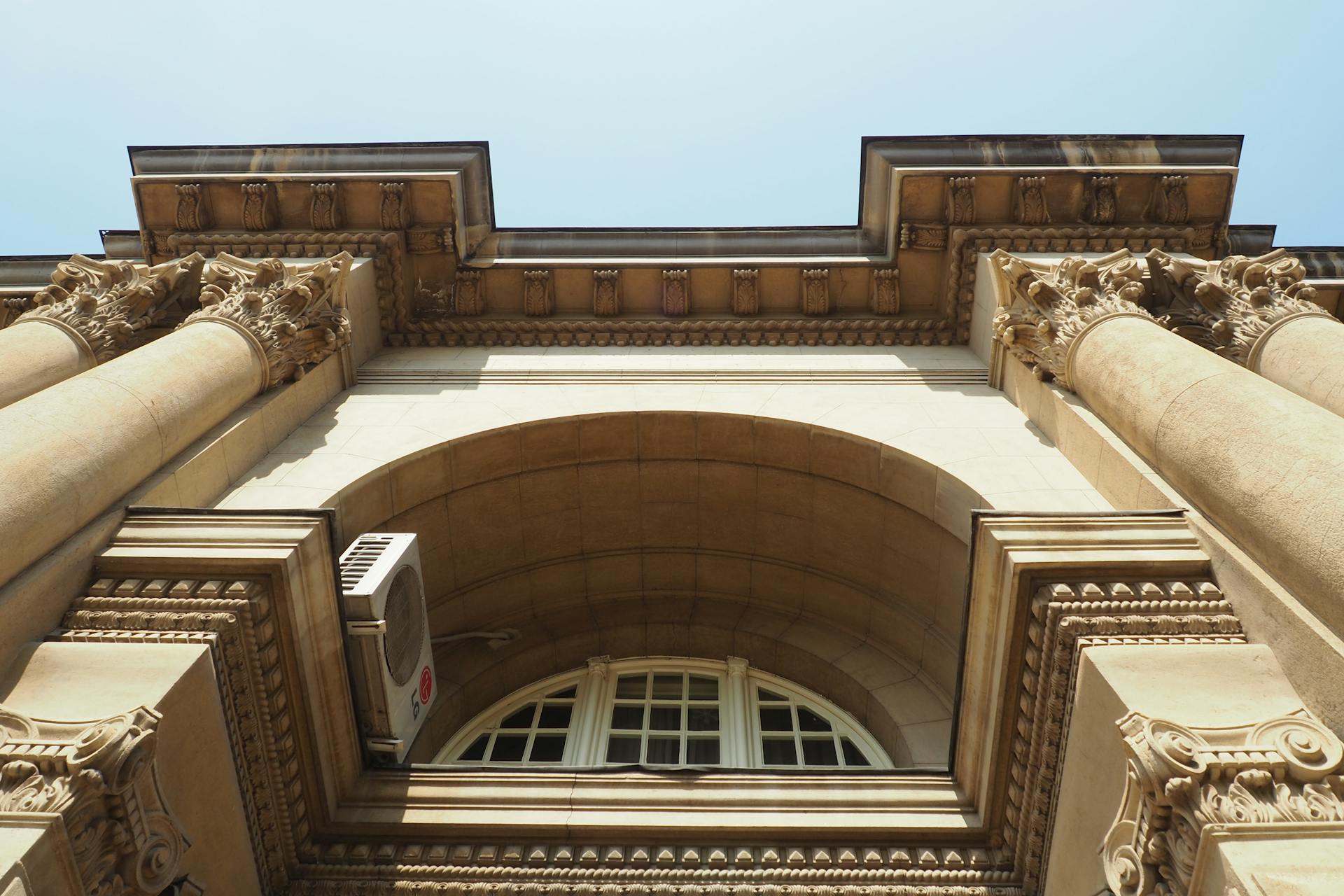
Java does not have a built-in mechanism for printing in columns, but there are a few ways to accomplish this. One way is to use the DecimalFormat class, which allows you to specify the number of decimal places to use, and the width of the field. For example, the following code will print a number in a field that is 10 characters wide, with 2 decimal places:
DecimalFormat df = new DecimalFormat("#.##"); System.out.println(df.format(1.2345));
This will output:
1.23
Another way to print in columns is to use the printf method, which is part of the java.io.PrintStream class. This method allows you to specify the width of the field, and the number of decimal places to use. For example, the following code will print a number in a field that is 10 characters wide, with 2 decimal places:
System.out.printf("%10.2f", 1.2345);
This will output:
1.23
You can also use the String.format method to format a string for output. This method works like the printf method, but it returns a string instead of printing it to the console. For example, the following code will format a number in a field that is 10 characters wide, with 2 decimal places:
String s = String.format("%10.2f", 1.2345); System.out.println(s);
This will output:
1.23
What is the easiest way to print in columns in Java?
Java is a versatile programming language that enables developers to create a wide range of applications. One common use for Java is to create programs that print text in columns.
There are a few different ways to print text in columns in Java. The easiest way is to use the System.out.printf method. This method allows you to specify the width of each column, as well as the alignment of the text within the column.
For example, the following code will print the text "Hello, world!" in two columns, with the text aligned to the left in the first column and to the right in the second column:
System.out.printf("%-10s%10s%n", "Hello,", "world!");
Hello, world!
If you need to print a lot of text in columns, you can also use the DecimalFormat class. This class allows you to format numbers in a variety of ways, including in fixed-width columns.
For example, the following code will print a table of numbers in three columns, with the numbers aligned to the right in each column:
DecimalFormat df = new DecimalFormat("000");
for (int i=1; i<=10; i++) {
System.out.println(df.format(i) + " " + df.format(i*i) + " " + df.format(i*i*i));
}
001 001 001
002 004 008
003 009 017
004 016 032
005 025 061
006 036 100
007 049 147
008 064 200
009 081 261
010 100 324
There are a few things to keep in mind when printing text in columns in Java. First, the width of each column must be specified in characters. Second, the text will be aligned within the column according to the specified alignment (left, right, or center).
Finally, it is important to note that the Java language does not include a built-in method for printing text in columns. However, there are several third-party libraries that provide this functionality.
How can I print in columns without using a loop?
There are a few ways that you can print in columns without using a loop. One way is to use the column command in your word processor. For example, in Microsoft Word, you can go to the Layout tab and click on Columns. From there, you can choose how many columns you want and whether you want them to be equal width or not.
Another way to print in columns without using a loop is to use a table. You can create a table with as many columns as you want and then just enter the text into the cells.
Finally, you can use CSS to create a multi-column layout. This is a bit more complex, but if you want complete control over your layout, it is worth learning.
What is the best way to format my data before printing in columns?
The best way to format your data before printing in columns is to use a tool like Microsoft Excel. This will allow you to select the data you want to print, and then format it into columns. You can also use this tool to add headers and footers, and to change the margins.
If you want to print your data in a different order than it is currently in, you can use the "Sort" function in Excel. This will allow you to choose the order in which you want the columns to appear.
Once you have your data formatted the way you want it, you can print it out using the "Print" function in Excel.
How do I ensure that my columns are evenly spaced?
There are a few ways to ensure that your columns are evenly spaced. One way is to use a ruler or measuring tape to measure the space between each column, and then adjust the spacing as needed so that they are all even. Another way is to use a column grid system, which is a series of vertical lines that you can use as a guide to help you keep your columns evenly spaced. There are also a few software programs that can help you create evenly spaced columns, or you can use a template that already has evenly spaced columns.
If you want to ensure that your columns are evenly spaced, one of the best ways to do so is to use a ruler or measuring tape. Measure the space between each column, and then adjust the spacing as needed so that they are all even. This is a simple and effective way to make sure your columns are evenly spaced.
Another way to ensure that your columns are evenly spaced is to use a column grid system. A column grid system is a series of vertical lines that you can use as a guide to help you keep your columns evenly spaced. This is a great option if you want to ensure that your columns are evenly spaced, but you don’t want to have to measure each column individually.
There are also a few software programs that can help you create evenly spaced columns. These programs can be a great option if you want an easy way to create evenly spaced columns.
If you want to ensure that your columns are evenly spaced, you can also use a template that already has evenly spaced columns. This is a great option if you don’t want to measure each column individually or use a software program.
All of these options are great ways to ensure that your columns are evenly spaced. Choose the option that works best for you and your project.
How can I print in columns if my data is in a 2D array?
There are a few different ways that you can print in columns if your data is in a 2D array. One way is to use the built-in print function in Python. This function takes a 2D array as an argument and prints it out in columns. Another way is to use the NumPy function np.array2string. This function also takes a 2D array as an argument and prints it out in columns.
If you want to print in columns without using a built-in function, you can use a for loop. Loop through each row of the 2D array, and then loop through each element in the row. Print each element in a separate column. You can use the string format() method to control the number of decimal places that are printed.
It is also possible to print in columns using the pandas library. The pandas library is a Python library for data analysis. The pandas library has a function called to_string that can be used to print data in columns. The to_string function takes a 2D array as an argument and prints it out in columns.
What is the difference between print and println when printing in columns?
The Java programming language provides two basic ways to print information: println and print. println displays information on the screen and then starts a new line, while print displays information on the screen without starting a new line.
This can be important when printing in columns, as you may want each piece of information to appear on a separate line. In this case, you would use println to print each piece of information, followed by a line break. Alternatively, you could use print to print all of the information on the same line, separated by a space or other character.
So, to summarize, the main difference between println and print is that println starts a new line after printing the information, while print does not.
How can I print in columns if I have a lot of data?
If you have a lot of data that you need to print out in columns, there are a few different ways that you can go about doing this. One option is to use a word processing program such as Microsoft Word or Google Docs, and use the built-in tools to format your data into columns. Another option is to use a spreadsheet program such as Microsoft Excel or Google Sheets, and use the built-in tools to format your data into columns. Finally, you can use a specialised printing program such as Adobe InDesign or QuarkXPress, which will allow you to more easily format your data into columns.
What are some common errors when printing in columns in Java?
There are a few common errors that can occur when printing in columns in Java. One error is that the data may not be aligned properly. This can happen if the data is not in the correct format or if the data is not spaced correctly. Another error that can occur is that the columns may not print correctly. This can happen if the column widths are not set correctly or if the data is not in the correct order.
How can I troubleshoot errors when printing in columns in Java?
When you are printing in columns in Java, there are a few common errors that can occur. Here are some tips on how to troubleshoot these errors:
1. Make sure that you are using the correct print method. If you are trying to print in columns, you should be using the printColumns method. This method will take care of formatting your data so that it prints in the correct format.
2. Make sure that your data is in the correct format. The printColumns method expects your data to be in an array of strings. If your data is not in this format, you will need to convert it before you can print it in columns.
3. Make sure that you have the correct number of columns. The printColumns method requires you to specify the number of columns that you want to print. If you do not specify the correct number of columns, your data will not print in the correct format.
4. Make sure that you have the correct width for each column. The printColumns method allows you to specify the width of each column. If you do not specify the correct width, your data will not print in the correct format.
5. Make sure that you have the correct alignment for each column. The printColumns method allows you to specify the alignment for each column. The options for alignment are left, right, and center. If you do not specify the correct alignment, your data will not print in the correct format.
6. Make sure that you have the correct padding for each column. The printColumns method allows you to specify the padding for each column. The options for padding are left, right, and center. If you do not specify the correct padding, your data will not print in the correct format.
7. Make sure that you have the correct number of decimal places. The printColumns method allows you to specify the number of decimal places. If you do not specify the correct number of decimal places, your data will not print in the correct format.
8. Make sure that you have the correct character for the decimal point. The printColumns method allows you to specify the character for the decimal point. If you do not specify the correct character, your data will not print in the correct format.
9. Make sure that you have the correct character for the thousands separator. The printColumns method allows you to
Frequently Asked Questions
Which method should we use to print the statements in Java?
The print () method is used to print text on the console.
How to print 2D array in Java?
Method #2 – Using System.out.println() Method. We can also print the 2D array using System.out.println(). Code: /** Prints the 2D arrays ar using System.out.println() method */public class PrintUsingSystemOut {public static void main(String[] args) {System.out.println("==========================");for (int i = 0; i < ar.length; i++) How to print 2D array in java?
How to print statements on the console in Java?
In general, we use the print () method to print a statement on the console. However, if we want to format the output differently or send it to a different destination, then we can use the println () method. Both methods will print the same statement on the console but they provide different formatting options and destinations. The following example prints "Hello, World!" on the console. print("Hello, World!");
What is the use of printf () method in Java?
The printf () method is used to format the string and to pass it to the print (), println (), or pprint () methods of the OutputStream class.
How to print a statement in Java?
In Java, we can use the println () method to print the statement. Let us consider an example: System.out.println("Java is a great language"); This will print "Java is a great language" on the screen.