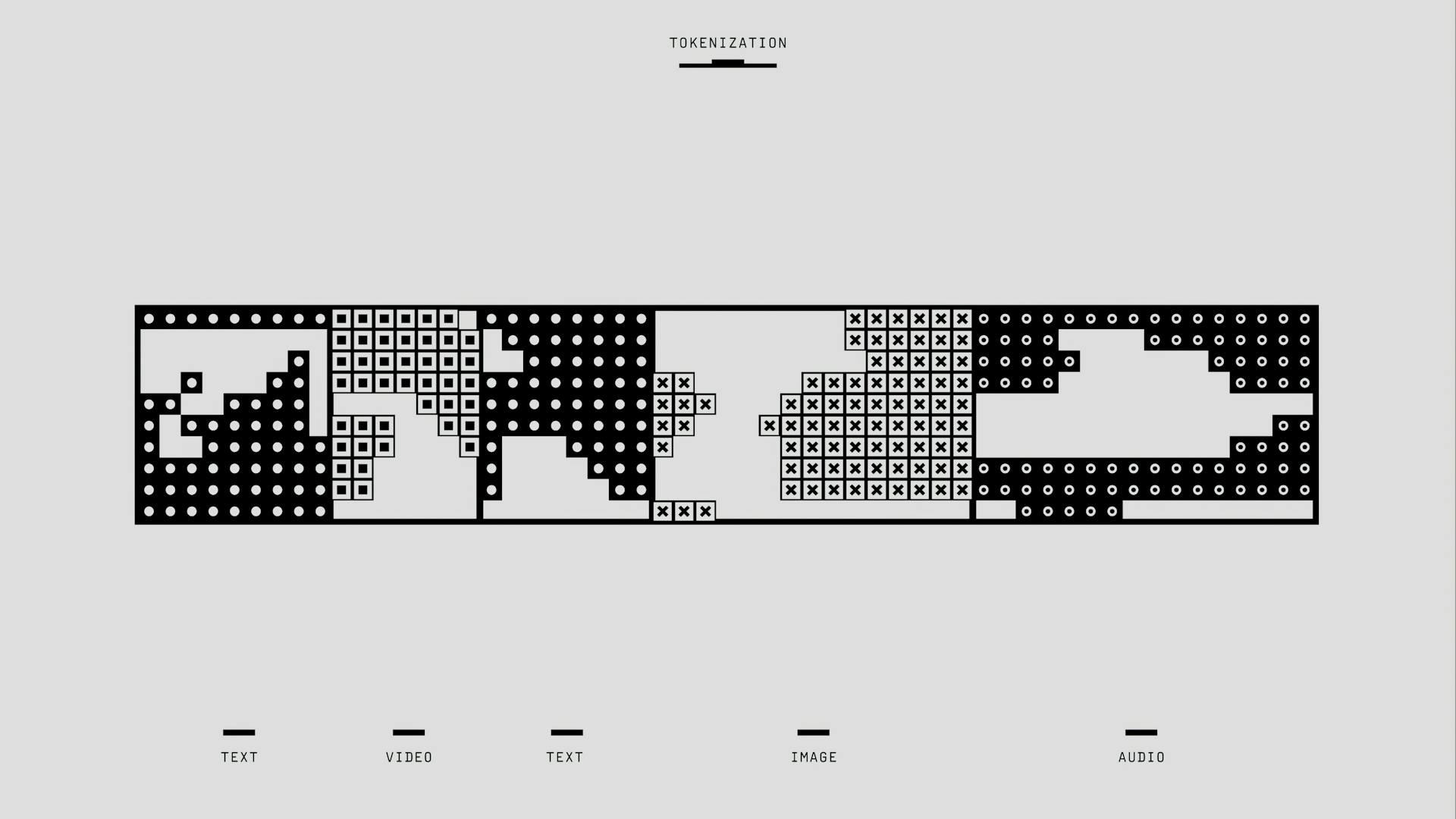
Authentication is a crucial aspect of any web application, and Django Rest Framework (DRF) provides several built-in authentication classes to make it easier to secure your API.
You can use the TokenAuthentication class to authenticate users with a token-based system, where users receive a token upon successful login, which is then used to authenticate subsequent requests.
To use TokenAuthentication, you'll need to install the djangorestframework-simplejwt library, which provides a simple and secure way to handle JSON Web Tokens (JWT).
In DRF, the SessionAuthentication class is used to authenticate users using their session data, which is stored in the user's browser.
Installation and Setup
To enable JWT authentication in your Django project, you need to install Django Rest Framework and Simple-JWT. Add them to your requirements.txt or install them directly using pip.
You can install Django Rest Framework and Simple-JWT by adding 'rest_framework' and 'rest_framework_simplejwt' to your project's INSTALLED_APPS in the settings.py file.
You can also set up a default authentication scheme globally using the DEFAULT_AUTHENTICATION_CLASSES parameter. This allows you to establish a standard authentication method across your entire project.
You might like: Install Django Project
Installing
Installing Django Rest Framework and Simple-JWT is the first step to enabling JWT authentication in your Django project. You can add them to your requirements.txt or install them directly using pip.
To install them, you need to add 'rest_framework' and 'rest_framework_simplejwt' to your project's INSTALLED_APPS in the settings.py file. This is a crucial step in setting up the framework for JWT authentication.
Expand your knowledge: How to Add Sitemap to Django Project
Configuring
Configuring your Django project for authentication involves several steps. You can configure the default authentication classes globally using the DEFAULT_AUTHENTICATION_CLASSES parameter. This allows you to define the authentication schemes for your entire project.
To enable JWT authentication, you'll need to install Django Rest Framework and Simple-JWT. Add them to your requirements.txt or install them directly using pip. Next, add 'rest_framework' and 'rest_framework_simplejwt' to your project's INSTALLED_APPS in the settings.py file. This will enable JWT authentication for your project.
You can also configure the authentication scheme per view or per viewset basis using the APIView class. Alternatively, you can use the @api_view decorator with function-based views. This gives you more flexibility in defining authentication schemes for specific views or viewsets.
Curious to learn more? Check out: Jwt Azure Ad Authentication
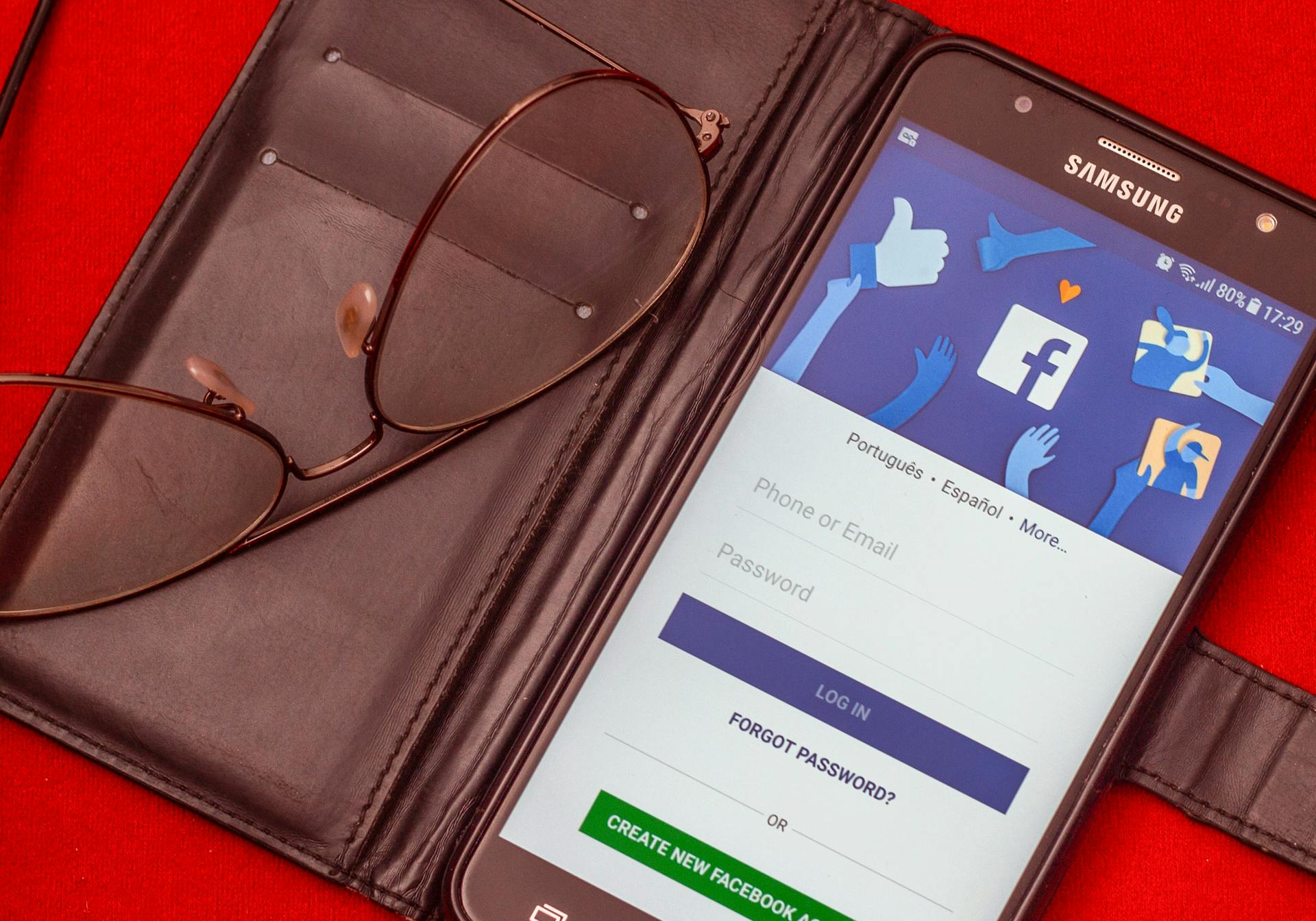
BasicAuthentication is a built-in authentication scheme that uses HTTP Basic Authentication. It's usually used for testing purposes only. If you're planning to use BasicAuthentication in a real project, make sure your API is accessible only through HTTPS and that clients always request the username and password upon login, never storing them in a permanent storage.
Here are the authentication schemes you can use:
- BasicAuthentication
- JWT Authentication
- Custom authentication classes
Remember to configure your authentication scheme globally, per view, or per viewset basis according to your project's needs.
User System and Token Generation
To set up a user system and generate tokens in Django REST Framework, you'll need to create a user authentication system, such as using Django's built-in User model or a custom user model. This can be done by creating a custom user model, as shown in Example 1.
You can also use the built-in User model, but be aware that it has some limitations. To generate tokens, you'll need to create an endpoint that returns an access token and a refresh token when a user logs in. This can be done using the `TokenAuthentication` scheme, as described in Example 3.
To automate token generation, you can use a signal to generate a token for each user when they are created. This can be done by overriding the `post_save` signal, as shown in Example 4.
Here's a summary of the steps to set up a user system and generate tokens:
User System Creation
Creating a user authentication system is a crucial step in using JWT authentication. You can use Django's built-in User model or create a custom user model.
To create a custom user model, you can use Django's built-in User model as a reference. Django's built-in User model is a good starting point, but you may need to add or modify fields to suit your application's needs.
A custom user model can be defined with fields like username, email, and password. Here's a sample custom user model: Django's built-in User model or create a custom user model.
You can use the following code to create a custom user model:
```python
from django.contrib.auth.models import AbstractBaseUser, PermissionsMixin
from django.db import models
class User(AbstractBaseUser, PermissionsMixin):
username = models.CharField(max_length=255, unique=True)
email = models.EmailField(unique=True)
password = models.CharField(max_length=255)
```
Token Generation and Handling
You can generate JWT tokens for users when they log in, and handle token generation and refresh using a specific endpoint. This endpoint will return an access token and a refresh token when a user logs in.
To generate tokens automatically for each user, you can use the post_save signal to create a token for each user when they're created. This is useful if you want to ensure that each user has a token as soon as they're created.
If you've already created users, you can generate tokens for them using the API endpoint. This is useful if you want to provide a way for clients to obtain a token using their username and password.
Here's a list of the steps to follow to generate tokens automatically:
- Perchase the post_save signal to create a token for each user when they're created.
- Use the API endpoint to generate tokens for existing users.
Here's an example of how to use the API endpoint to generate a token:
- Send a POST request to the API endpoint with the user's credentials.
- The API will return an access token and a refresh token.
You can also use the Django admin interface to create tokens for users. This is useful if you want to create tokens for a large number of users at once.
User
To create a user authentication system, you can use Django's built-in User model or create a custom user model. A sample custom user model is shown in Example 2.
You can also create your own authentication scheme by creating a subclass of BaseAuthentication and overriding the .authenticate(self, request) method. This method should return a tuple (user, auth) if authentication is successful, or None otherwise.
If you want to raise an exception instead of returning None, you can call AuthenticationFailed in the .authenticate() method. It's generally a good practice to follow this approach:
- If no authentication attempt was made, return None. Other authentication schemes that are also used will be checked.
- If an authentication attempt was made but failed, call AuthenticationFailed. An error response will be returned immediately, regardless of any permission checks and without checking other authentication schemes.
You can also override the .authenticate_header(self, request) method if you want to return a string that will be used as the value of the WWW-Authenticate header in the HTTP 401 Unauthorized response.
Here's a simple table to illustrate the possible outcomes of the .authenticate() method:
Note that when your custom authentication scheme is called through the .user or .auth properties of the request object, you may see AttributeError being converted to WrappedAttributeError. This is necessary to prevent the original exception from being suppressed by accessing an external property. Python doesn't recognize that the AttributeError comes from your custom authentication scheme, and instead considers it to be an attribute error on the request object. These errors should be fixed or otherwise handled by your authentication scheme.
Authentication Workflow
Django Rest Framework authentication uses JSON Web Tokens (JWT) to verify user credentials. JWT Authentication Workflow is a crucial part of this process.
The server issues two tokens to the user after verifying the credentials: an Access Token and a Refresh Token. These tokens are stored securely by the frontend of your application.
Access Tokens are short-lived, expiring after 10-15 minutes, depending on the configuration. This prevents misuse of the token if an attacker gains access to it.
Intriguing read: Django Rest Framework Jwt
The frontend proceeds to refresh the token by sending the Refresh Token to the "refresh" endpoint after the Access Token expires. This ensures seamless authentication.
Customization of the JWT workflow is possible through Simple JWT. You can define SIMPLE_JWT in your settings to adjust the behavior to your business needs.
Access and Refresh token expiration time can be customized, and even refreshing the Refresh Token is supported. This flexibility allows you to tailor your authentication workflow to your specific use case.
Frequently Asked Questions
How to use token authentication in Django Rest Framework?
To use token authentication in Django Rest Framework, navigate to the "Token" section and create a token, then replace placeholders in your API URL with the actual token key and endpoint URL. Replace 'your-token-key' and 'http://localhost:8000/api/your-endpoint/' with your actual token and URL.
What is Django Rest Auth?
Django Rest Auth is a library that provides REST API endpoints for user authentication and registration. It simplifies the process of creating secure and scalable authentication systems for web applications.
Sources
- https://www.guguweb.com/2022/01/23/django-rest-framework-authentication-the-easy-way/
- https://www.remoteinning.com/blog/how-to-use-jwt-authentication-with-django-rest-framework
- https://blog.devgenius.io/jwt-authentication-in-django-rest-framework-with-simple-jwt-a-comprehensive-guide-f2ba860f1365
- https://ilyachch.gitbook.io/django-rest-framework-russian-documentation/overview/navigaciya-po-api/authentication
- https://learnbatta.com/blog/authentication-in-django-rest-framework-81/
Featured Images: pexels.com