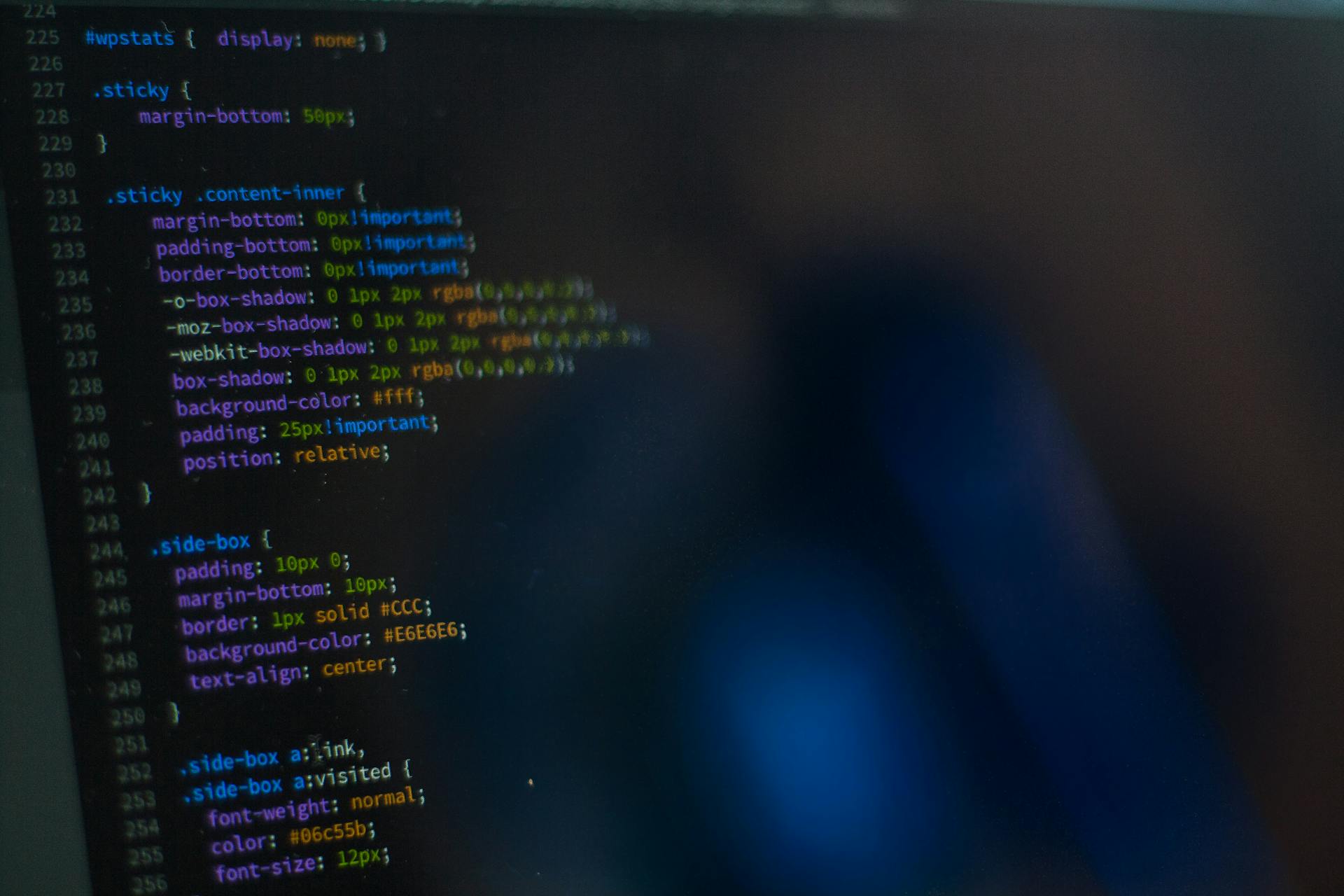
CSS selectors are a fundamental part of web development, allowing you to target specific elements on a webpage with precision.
They work by using a set of rules to identify elements, which can be based on their tag name, class, ID, or attributes.
For example, using the CSS selector `div#myDiv` will select the element with the ID "myDiv" that is a div element.
In contrast, CSS selectors are specific to web development and are not used in other programming languages or contexts.
CSS selectors are also much faster than XPath expressions, which can be a significant advantage in large-scale web applications.
However, CSS selectors can be limited in their ability to target complex elements, such as those with nested structures or dynamic content.
This is where XPath comes in, offering a more powerful and flexible way to target elements on a webpage.
A fresh viewpoint: Web Page Design and Development
What Is a Locator?
A locator is a crucial tool in test automation that helps select specific elements in the Document Object Model (DOM) tree of a web page. It's used by test automation tools to locate and interact with elements during test execution.
Related reading: Css Selector the Last 2 Child Elements
Locators are essential because they allow automation tools to find almost every component or element on the webpage. This is done by matching the element based on the provided locator expression.
There are two main types of locators: XPath Locator and CSS Locator. These two types have been around for many years and are supported by all major browsers, frameworks, and programming languages.
Here are the two types of locators:
A locator expression is used to match the desired element based on its attributes, such as id, class, name, or other HTML attributes. This expression is used by automation tools to find the specific element on the webpage.
Related reading: How to Find Css Selector
Types of Locators
There are several types of locators used in Selenium, including className, name, id, tagName, linkText, partialLinkText, XPath, and CSS selector.
The most commonly used locators are XPath and CSS selector, which offer several advantages over other types of locators.
We can use the following locators to identify elements on a web page: id, className, name, tagName, linkText, partialLinkText, XPath, and CSS selector.
Discover more: Css Name Selector
Selenium supports eight different types of locators: className, name, id, tagName, linkText, partialLinkText, XPath, and CSS selector.
Here are the different types of locators and their syntax:
The syntax for XPath locators is //tagname[@attribute='value'], while the syntax for CSS selectors is tagname[attribute='value'].
The eight types of locators supported by Selenium are: className, name, id, tagName, linkText, partialLinkText, XPath, and CSS selector.
We can use the following Selenium methods to pass the values from the example column into: driver.find_element_by_xpath(), driver.find_element_by_css_selector(), driver.find_element_by_id(), driver.find_element_by_name(), driver.find_element_by_class_name(), driver.find_element_by_link_text(), driver.find_element_by_partial_link_text(), and driver.find_element_by_tag_name().
Curious to learn more? Check out: Css Select Data Attribute in Pseudo Selector
Creating a Locator
Creating a locator is a crucial step in automating a web application using Selenium. You can create a locator using either XPath or CSS selectors, both of which are supported by modern browsers.
CSS selectors are almost as powerful as XPath, but some browsers might not fully support all of the CSS selectors you need. This is why it's essential to consider the browser you're using when deciding between XPath and CSS selectors.
Consider reading: Convert Xpath to Css Selector
To create a CSS selector, you can use the browser developer's tools, which are available in Mozilla Firefox, Microsoft Edge, and Google Chrome. These tools help you create CSS selectors by allowing you to inspect the DOM tree and select the desired element.
One of the benefits of using CSS selectors is that they are relatively easy to write and maintain, especially when compared to XPath expressions. However, it's essential to keep your locator expressions short and robust to ensure they survive incoming front-end changes.
Here are some best practices to keep in mind when writing locator expressions:
- Avoid using absolute expressions, which start from the root of the HTML element and can be prone to breaking with changes in the DOM.
- Use relative expressions instead, which are easier to write and more robust.
- Don't use any information that's likely to change in the future.
Here is a cheatsheet for writing Selenium locators:
By following these best practices and using the cheatsheet, you can create effective and robust locator expressions that will help you automate your web application with ease.
Selector vs XPath
Now that we've explored the basics of CSS selectors and XPath, let's dive into the key differences between them.
The main difference between a CSS selector and an XPath is that a CSS selector is used to select elements based on their style, while an XPath is used to select elements based on their position and structure.
Both CSS selectors and XPath can be used to locate elements on a webpage, but they have different approaches. CSS selectors are generally faster and more efficient, while XPath can be more flexible and powerful.
Explore further: Css Selector Select Child of Parent
vs
When choosing between a CSS selector and an XPath, the direction of traversal is a key consideration. CSS selectors allow only unidirectional flow, meaning you can only traverse from parent to child.
In contrast, XPath enables bidirectional flow, allowing traversal from both child to parent and parent to child.
CSS selectors are generally faster than XPath, making them a better choice in terms of speed and performance. However, XPath provides axes to solve complicated selector problems, which CSS doesn't.
Here's a comparison of the two:
CSS selectors also have a simpler syntax, making them easier to learn and use. They include a single element in their structure, which makes them more readable as they grow.
Selecting with Style
CSS selectors are a popular option for element selection in test automation, allowing testers to select elements based on their HTML tags, classes, attributes, or other CSS properties.
They have a simple syntax, including a single element in their structure, making them easy to learn and use.
CSS selectors are widely used in web development to apply styles to specific elements, but they can also be used for element selection in test automation.
Their performance is the same or faster compared to XPath, making them a viable option.
However, CSS selectors only allow unidirectional flow, making it difficult to traverse from child to parent, unlike XPath.
Here are some of the key advantages of using CSS selectors:
- Simple syntax
- Performance is the same or faster compared to XPath
- Easier to learn and use
While they have their advantages, CSS selectors also have some disadvantages, particularly when it comes to handling complex element locators.
Advantages and Performance
CSS selectors have several advantages over XPath, making them a preferred choice for test automation. They are faster compared to XPath, which can be computationally expensive and impact overall performance.
One key benefit of CSS selectors is their unidirectional search capability, allowing them to search for elements in the DOM tree more efficiently. This makes them ideal for applications where performance is critical.
CSS selectors also have an advantage when it comes to maintenance. They are less prone to breaking when new element-level changes are introduced, unlike XPath which tends to break in such situations.
Here are some key differences between CSS selectors and XPath:
CSS selectors are also more readable and maintainable than XPath, especially when they become complicated. This makes them a better choice for large test suites where readability is crucial.
Best Practices and Tools
When working with CSS selectors and XPath, it's essential to follow best practices to ensure efficient and accurate automation. Use meaningful and descriptive variable names to avoid confusion and make your code more readable.
CSS selectors are generally faster and more efficient than XPath, especially for large datasets. This is due to the way CSS selectors are parsed and evaluated by the browser.
To optimize your CSS selectors, focus on using specific and unique selectors that target the exact elements you need. Avoid using overly broad selectors that may match more elements than intended.
Readability
Readability is a crucial aspect of writing efficient and maintainable code.
XPath syntax can be complex and overwhelming, especially for beginners.
CSS selectors, on the other hand, have a simpler and more readable syntax, making it easier for testers to construct and maintain selector expressions.
This is because CSS selectors use familiar HTML tag, class, and attribute representations, which are easier to understand and work with.
As a result, CSS selectors are generally more intuitive and easier to learn than XPath.
Writing Locator Expressions Best Practices
Writing locator expressions is a crucial part of automating web browsers with Selenium, and there are some best practices to keep in mind.
Avoid using information that's likely to change in the future, like IDs or absolute paths, as they can break your script when the website updates.
Using short and robust locators will ensure your script survives more incoming front-end changes.
Short locators are easier to write and maintain, making them a better choice than absolute expressions.
Relative expressions are a good option, as they're easy to write and more robust than absolute expressions.
Here's a cheatsheet for writing Selenium locators:
Use Cases and Compatibility
XPath enjoys wider compatibility with different document types, including both XML and HTML, making it a better choice for working with XML documents or applications that use XML-like structures.
CSS selectors, on the other hand, are primarily designed for HTML documents and may not work as expected with XML.
The choice between XPath and CSS selectors ultimately depends on the specific use case and the attributes available in the DOM tree, with XPath being well-suited for complex scenarios that require bidirectional traversal or working with XML documents.
Use Cases
When working with web scraping, the choice between XPath and CSS selectors depends on the specific use case.
XPath is well-suited for complex scenarios that require bidirectional traversal or working with XML documents.
CSS selectors are a good choice for most common use cases, especially when an element can be uniquely identified by its id, class, or other attributes.
If an element's id, class, or attributes make it easily identifiable, CSS selectors provide a reliable and efficient way to select the element.
You might like: Css Selector Multiple Attributes
Compatibility
XPath has wider compatibility with different document types, including both XML and HTML. This makes it a more versatile choice for working with various types of documents.
Both XPath and CSS selectors are widely supported in test automation libraries, programming languages, and modern browsers. But it's worth noting that CSS selectors are primarily designed for HTML documents.
CSS selectors may not work as expected with XML documents or applications that use XML-like structures. This is because they're not designed to handle the complexities of XML.
Worth a look: Css Not Class
Selecting and Extracting
CSS selectors can select elements based on their HTML tags, classes, attributes, or other CSS properties.
Both CSS selectors and XPath can be used to select elements, but they have different approaches. CSS selectors are widely used in web development to apply styles to specific elements.
To extract text from elements, you can use the text() function in XPath. For example, the XPath //h2 returns the element, and the text() function can be used to extract the text inside it.
You can also extract the value of any attribute using the @ symbol with the attribute name in XPath. For example, to extract the value of the href attribute from an anchor tag, you can use @href in the XPath.
XPath allows you to search for elements that contain specific text by using the text() function in square brackets. Note that this will be looking for a complete match.
For more insights, see: Css Selector That Targets a Specific Property Declaration
Browser Testing and Automation
Browser testing and automation is a crucial step in ensuring the accuracy of your XPath and CSS selectors. You can test your XPath or CSS selector in Chrome's JavaScript console by wrapping it in a statement.
To check the correctness of the XPath in the browser, there are two important points to consider. The XPath expression in the browser developer tool search box must match at least one element, and once you enter the valid XPath expression, the specific UI element must be highlighted.
If none of the above criteria meets, consider rewriting the XPath expressions. This can save you time and effort in the long run.
Check this out: Linux Console Web Browser
Test Automation
Test Automation is a crucial step in ensuring the quality of your application. It helps you identify and fix bugs early on, saving you time and resources in the long run.
Both CSS and XPath-based selectors are supported by most automation frameworks, but it's essential to know when to use each. Writing locators requires technical knowledge and proficiency, and creating stable and unbreakable locators is key.
Modern AI-based test automation frameworks like Testsigma cloud offer automatic construction of selectors in the background, making test engineers' tasks easier and increasing productivity. This tool records the selectors and provides an option to edit and customize locators, easing the testing process.
Frequently Asked Questions
Which locator is faster in Selenium CSS or XPath?
CSS Selector Locator is generally faster than XPath Locator in Selenium, but slower than ID Locator. This makes CSS Selector Locator a good choice for locating elements when the ID Locator is not available.
Sources
Featured Images: pexels.com