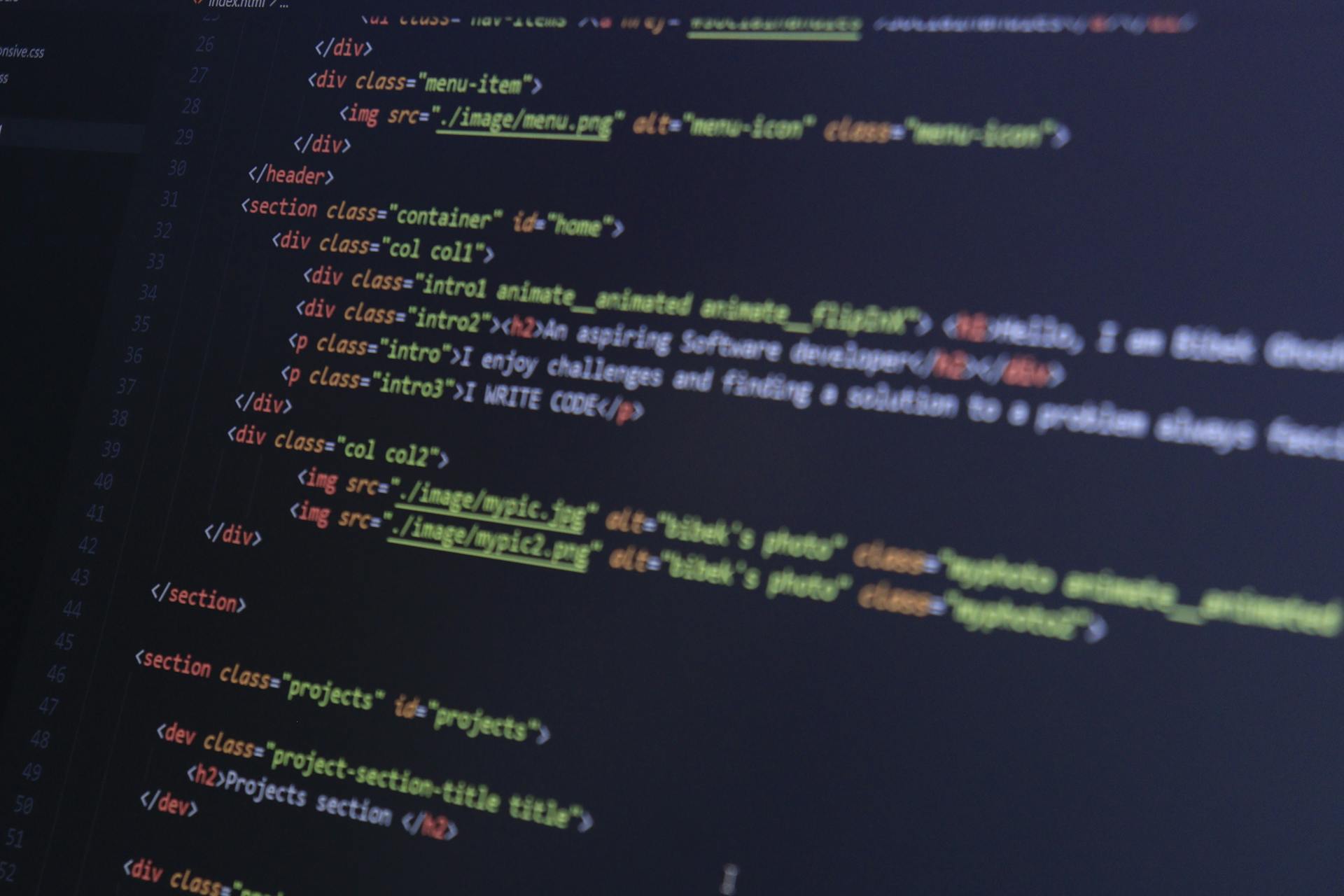
Clerk Nextjs streamlines user management and authentication, making it easier to manage users and their access levels.
With Clerk Nextjs, you can easily manage users and their permissions, ensuring that only authorized users have access to sensitive areas of your application.
Clerk Nextjs provides a robust authentication system that handles user authentication, password recovery, and more.
Next.js Setup
To set up a Next.js application, you'll want to start by installing the latest version of Next.js using the create-next-app CLI tool. This tool will automatically set up and configure the Next.js project, including the default folder structure and files.
To begin, run the command `create-next-app clerk-passwordless-demo` in your preferred directory. Then, change into the newly created project folder and start the development server with the command `npm run dev`. The application will be assigned localhost:3000 by default, so open it in your browser to see what you've got.
The default folder structure and files created by the CLI tool include a /components folder in the src/app/ folder, which is where you'll create your Header component. To create the Header component, add a new file called Header.tsx to the /components folder and add the code below.
Additional reading: How to Start Next Js Project
Here's a list of the default pages created by the CLI tool:
- Home page: This is the entry point to the application.
- Auth page: This is where authentication takes place.
- Main page: This page should be loaded when authentication completes.
To create the Home page, replace the template code in the page.tsx file with the code below. This will define a default Home component with some sample content.
To create the Auth page, add a new /auth folder to the src/app/ folder and add a dynamic route for the Auth page. Then, add a page.tsx file to the /auth folder and add the code below. This will define a simple component for the Auth page.
To style the application, open the globals.css file in the src/app/ directory and replace all the styles defined in it with the styles in the GitHub repository.
Here's an interesting read: What Is .next Folder in Next Js
Authentication
Authentication is a crucial aspect of any Next.js application, and Clerk makes it a breeze. With Clerk, you can implement both password-based and passwordless forms of authentication, and in this context, we'll focus on passwordless.
Clerk provides a set of Next.js specific components and helpers that make adding user management a breeze. To get started, you'll need to install Clerk's Next.js package and add it to your Next.js project's dependencies.
To protect your application with Clerk, you'll need to wrap your application in the ClerkProvider component. This component provides access to the current session and user context. The ClerkProvider component is easily customizable and can be integrated with popular front-end and back-end frameworks.
Clerk also provides third-party integrations and SDKs for popular frameworks, making it easy to get up and running. With Clerk, you can implement one-time passwords, custom authentication links, and social sign-in.
Here are some key features of Clerk's authentication:
- One-time passwords
- Custom authentication links
- Social sign-in
- Passwordless authentication
- Password-based authentication
By using Clerk, you can focus on your primary business and let Clerk handle the complexity of authentication. With Clerk, you can redirect users to the Clerk sign-up page, and after successfully signing up, they'll be automatically redirected to your application.
To create a custom sign-in and sign-up page, you'll need to add additional environment variables to your project. This will tell Clerk where your signing and signup pages will be hosted in your application. Clerk will use these environment variables in their custom components SignIn and SignUp components.
Here's an example of how to configure the environment variables:
- `CLERK_SIGN_IN_PAGE_PATH`: The path to your sign-in page
- `CLERK_SIGN_UP_PAGE_PATH`: The path to your sign-up page
Once you've configured the environment variables, you can create a custom sign-in and sign-up page using the SignIn and SignUp components from the @clerk/nextjs package.
To protect your routes, you'll need to use the authMiddleware function provided by Clerk. This function will redirect users to the Clerk sign-in page if they're not authenticated. You can configure the authMiddleware function to protect specific routes by passing an array of route paths.
Here's an example of how to configure the authMiddleware function:
```javascript
import { authMiddleware } from '@clerk/nextjs';
export default authMiddleware({
publicRoutes: ['/sign-in', '/sign-up'],
});
```
Prerequisites and Configuration
To get started with Clerk Next.js, you'll need a Clerk account. You can sign up for a free trial on Clerk's website and create a new application.
Make sure to enable the Username attribute for your Application in Clerk, as it will be used later to access user data.
You'll also need an Ably account, which has a generous free tier that makes it easy to add user management to your application.
Explore further: Next Js Spa
Prerequisites
To get started with building this application, you'll need to have an Ably account. You can sign up for a free trial if you don't already have one.
Having a Clerk account is also essential, and fortunately, Clerk has a generous free tier that makes it easy to add user management to your application.
Make sure to enable the Username attribute for your Application when creating your Clerk account, as we'll be using that later to access user data.
You'll need to locate your Clerk API Keys, which can be found in the API Keys section of your Clerk application's dashboard.
Similarly, you'll need to find your Ably API key in the API Keys section of your Ably application's dashboard.
Lastly, ensure you have Node 18 installed on your system, as it's the minimum required version for this project. You can check your current Node version by running the command node -v from your terminal or command line.
Broaden your view: Next Js Dashboard
Create Schema and Database Connection
To create a schema and database connection, start by defining a user_messages schema in a file named src/app/db/schema.ts. This file should use the types provided by Drizzle ORM.
Additional reading: File Upload Next Js Supabase
Next, create a file named src/app/db/index.ts to use Neon's serverless driver with Drizzle ORM. This allows you to export the Drizzle instance as a singleton that other modules can import and reuse.
You'll also need to create a .env.local file in the root of your Next.js project directory and add your database URL from the Neon Console as an environment variable named DATABASE_URL.
Discover more: Nextjs Server Actions File Upload
Example and Integration
To integrate Clerk into a Next.js application, you can use the ClerkProvider component, which encapsulates the application to offer active session and user context to Clerk's hooks and other components.
The ClerkProvider component should be wrapped around the entire application, making its capabilities accessible from anywhere within the application. This is achieved by importing and wrapping the ClerkProvider component around the body of the application.
To add a protection layer across the application, Clerk SDK exposes a middleware called authMiddleware. This middleware can be used to implement protection across the application, redirecting all attempts to access it to Clerk's default authentication page.
If this caught your attention, see: Nextjs Middleware Firebase Auth
The authMiddleware function can be customized to make some pages public by passing an array of routes that should be public. For example, the Home and Auth pages can be made public by passing them as an argument to the authMiddleware function.
To complete a full authentication circle, sign-up, sign-in, sign-out, and user account management functionalities need to be implemented in the demo application. This involves updating the main page to include these functionalities.
By combining the Clerk middleware with other middlewares like the one provided by next-intl, you can ensure that protected routes are handled appropriately and then potentially redirect or rewrite incoming requests.
For your interest: Next Js Pages
Localization and Content
Localizing your page content can sometimes be tricky, especially when dealing with locale prefixes in your pathname.
If your page content isn't localized despite the pathname containing a locale prefix, it's very likely due to your middleware not running on the request.
A potential fallback from i18n/request.ts might be applied if the middleware doesn't run.
Broaden your view: Middleware Next Js
You should explicitly return a locale from getRequestConfig to recover from this error, especially if you're using a setup without i18n routing.
If no locale is available after getRequestConfig has run, next-intl will invoke the notFound() function to abort the render.
Consider adding a not-found page due to this, as it's a common issue.
Solution Architecture
Let's break down the solution architecture for a Clerk and Next.js application. Vercel will provide serverless hosting for your Next.js application.
Your Next.js application will direct users to Clerk for authentication, which will then redirect them to your application once they've completed the authentication flow using a supported provider like Google or Discord OAuth.
Clerk will handle the authentication process, allowing users to access protected pages and routes in your application.
You can read their session data as needed to obtain the user ID and query or associate data with the authenticated user in your Neon Postgres database.
Additional reading: Nextjs App Route Get Ssr Data
Sources
- https://prismic.io/blog/nextjs-authentication
- https://bejamas.io/hub/guides/passwordless-authentication-with-clerk-in-nextjs
- https://next-intl-docs.vercel.app/docs/routing/middleware
- https://ably.com/blog/how-to-use-clerk-to-authenticate-next-js-route-handlers
- https://neon.tech/blog/nextjs-authentication-using-clerk-drizzle-orm-and-neon
Featured Images: pexels.com