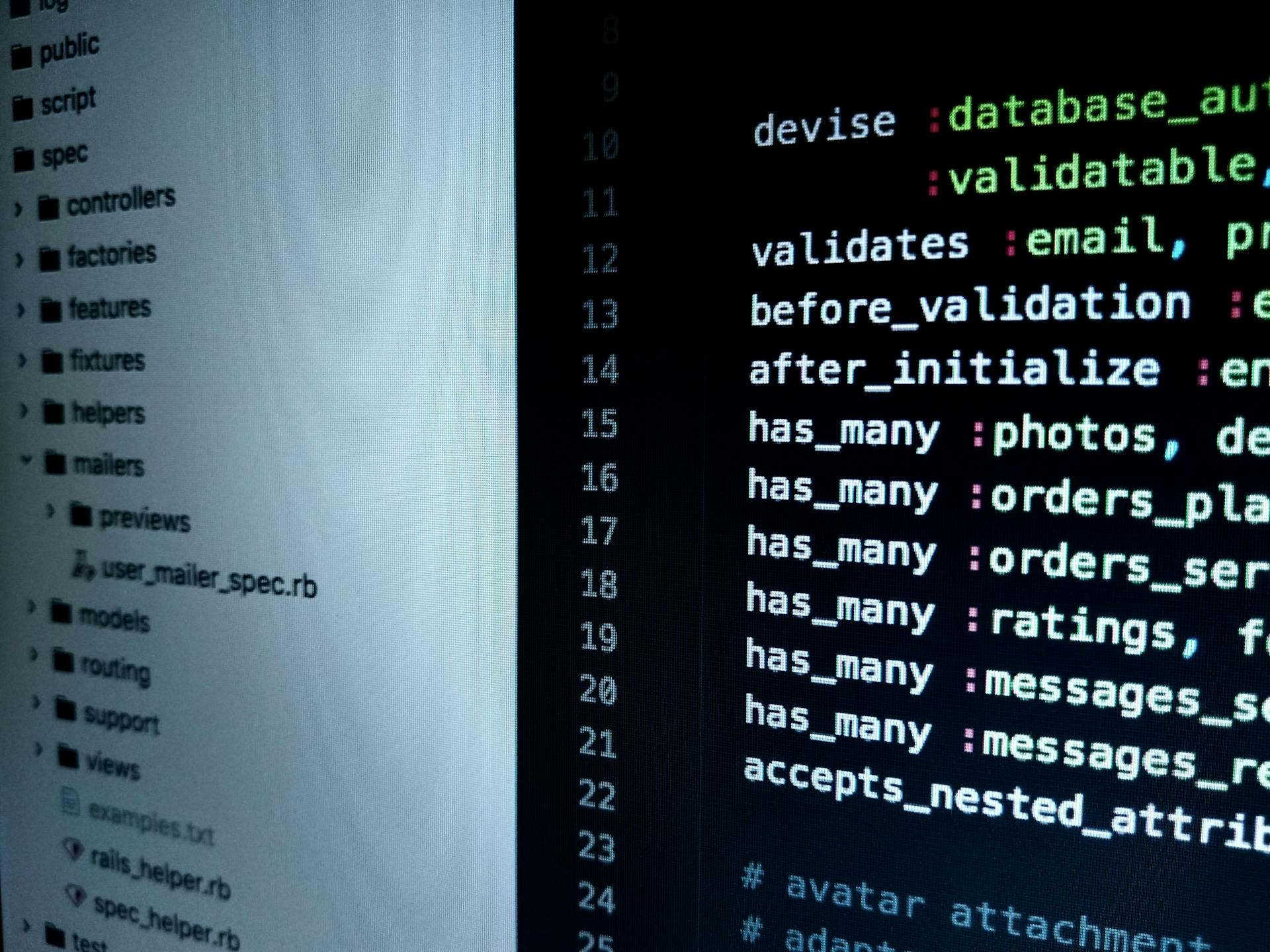
Azure SignalR is a Realtime Web Framework for Scalable Applications, allowing developers to build real-time web applications that can handle a large number of concurrent users.
It uses WebSockets under the hood to establish real-time connections between clients and servers, enabling features like live updates, chat, and collaboration.
With Azure SignalR, developers can focus on building their application without worrying about the underlying infrastructure, as it provides a scalable and fault-tolerant solution.
By using Azure SignalR, developers can create applications that can scale to meet the needs of their users, making it an ideal choice for applications that require real-time updates and high availability.
Intriguing read: Building Web Applications
Getting Started
To get started with Azure SignalR, you'll need an Azure subscription. You can sign up for a free trial or use your Visual Studio Subscription benefits when you create an account.
First, create an Azure SignalR resource. If you're not familiar with creating Azure resources, follow the step-by-step guide for creating a SignalR resource using the Azure portal. You can also use the Azure CLI, Azure PowerShell, or Azure Resource Manager (ARM) templates to create a SignalR resource.
Additional reading: Webflow Subscription
To quickly create a SignalR resource in Azure, you can deploy our sample template by clicking a link. After the instance is deployed, open it in the portal and locate its Settings page. Change the Service Mode setting to Serverless.
Alternatively, you can start with the .NET Core SDK. Install the latest version to get started.
To proceed, download or clone the AzureSignalR-sample GitHub repository.
Explore further: Sample Web Page Design
Authentication and Security
Authentication is crucial for your Azure SignalR Service to function properly. To authenticate your client, you'll need to use a connection string, which can be found in the Azure Portal or by using the Azure CLI or Azure PowerShell snippet.
For local development, store the connection string in the local.settings.json file. When deployed, use the application settings to set the connection string.
Azure SignalR Service supports different authentication providers, including Azure Active Directory (Azure AD) and custom authentication. To use Azure AD authentication, select Azure Active Directory in the Azure SignalR Service settings, configure the Azure AD tenant ID, client ID, and client secret, and obtain these values when you register your application in Azure AD.
Curious to learn more? Check out: How to Use Inspect Element to Find Answers
Security and Authentication
To secure your SignalR Service, you'll need to implement authentication and authorization mechanisms. Azure SignalR Service can work with Azure Active Directory (Azure AD) for authentication if needed.
You can select your SignalR Service in the Azure portal and go to the "Authentication" section. Choose an authentication provider, such as Azure AD or custom authentication, that suits your needs.
Azure AD authentication requires configuring settings like the Azure AD tenant ID, client ID, and client secret, which can be obtained when registering your application in Azure AD.
To define access control rules for your SignalR service, you can use the [Authorize] attribute in your SignalR hub methods or implement custom authorization logic based on the authentication information.
Here are the steps to configure Azure AD authentication:
- Click on “Azure Active Directory” in the Azure SignalR Service settings.
- Configure Azure AD authentication settings, including the Azure AD tenant ID, client ID, and client secret.
- Save Configuration and update your client application to obtain Azure AD tokens and pass them to the SignalR service when connecting.
Core Concepts
Azure SignalR is a real-time web framework that enables bi-directional communication between web servers and clients. It's built on top of the ASP.NET Core framework.
SignalR uses WebSockets, Server-Sent Events, and Long Polling to establish and maintain connections with clients. This allows for efficient and scalable communication.
With Azure SignalR, you can easily add real-time functionality to your web applications, making it perfect for live updates, live chat, and other interactive features.
Realtime Web Framework
SignalR Service client is a library that provides SignalR server functionalities in a serverless style, allowing for real-time web communication.
It's a game-changer for web applications that require instant updates, like live chat or stock market feeds.
The SignalR Service client offers serverless capabilities, making it a great choice for applications with variable traffic patterns.
This approach helps reduce infrastructure costs and improves scalability.
The SignalR client, on the other hand, is a more traditional approach that requires a server to handle real-time communication.
It's still a great option for many applications, especially those with predictable traffic patterns.
In general, the choice between SignalR Service client and SignalR client depends on the specific needs of your application.
Binding Info
Binding Info is a crucial aspect of working with Azure SignalR Service.
SignalR Connection Info input binding is a feature that makes it easy to generate the token required for SignalR clients to initiate a connection to Azure SignalR Service. To learn more, follow the Azure SignalR Connection Info input binding tutorial.
Curious to learn more? Check out: Web Dev Services
The SignalR trigger allows a function to be executed when a message is sent to Azure SignalR Service. This trigger is a powerful tool for real-time communication.
To use the SignalR trigger, you need to define a hub class with methods that can be called by the client.
Here are some key methods to include in your hub class:
- BroadcastMessage: This method broadcasts a message to all clients.
- Echo: This method sends a message back to the caller.
These methods use the Clients interface provided by the ASP.NET Core SignalR SDK, giving you access to all connected clients and allowing you to push content to your clients.
Trigger
Triggering functions in Azure Functions is a powerful feature that allows you to automate tasks and respond to events in real-time.
The SignalR trigger is a type of trigger that allows a function to be executed when a message is sent to Azure SignalR Service.
You can also trigger functions when a SignalR client gets connected or disconnected by applying the SignalRTrigger attribute to the InvocationContext parameter. This is useful for logging connection IDs or performing other actions when a client connects or disconnects.
Broaden your view: When Was Azure Launched
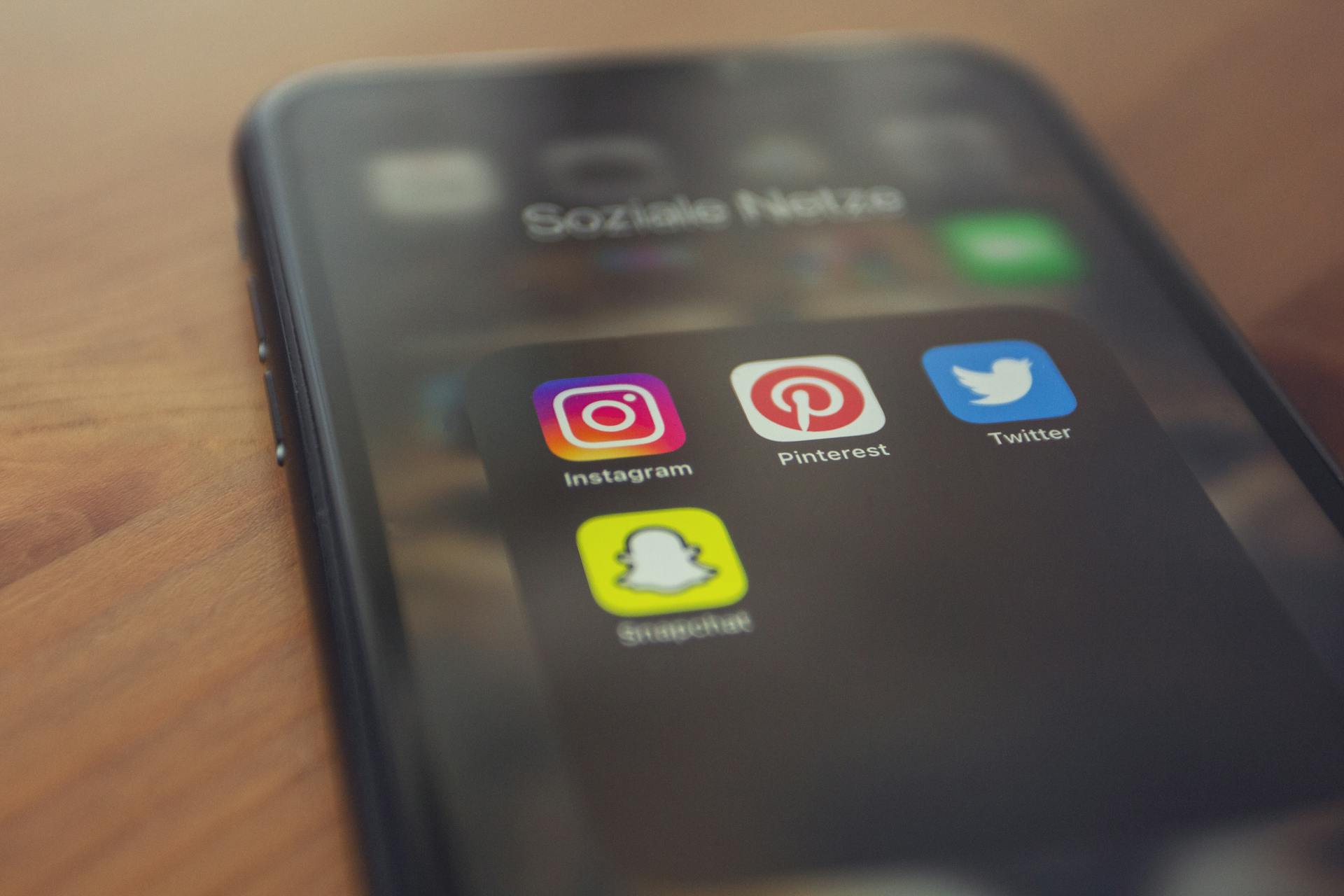
To trigger a function when a SignalR client sends a message, you can apply the SignalRTrigger attribute to the InvocationContext parameter, and the SignalRParameter attribute to each parameter whose name matches the parameter name in your message.
Triggering functions based on SignalR events can be a game-changer for real-time applications, allowing you to respond quickly and efficiently to user interactions.
You can use the SignalR trigger to execute a function when a message is sent to Azure SignalR Service, or when a SignalR client sends a message. This can be useful for logging message content, performing actions based on message data, or triggering other workflows.
Additional reading: Web Based Application Programming Language
Scalability and Monitoring
Scalability is key to handling varying workloads, and Azure SignalR Service offers two scaling methods: vertical scaling, which increases capacity by changing the pricing tier, and horizontal scaling, which adds more instances to distribute the load.
To scale up, navigate to your SignalR service in the Azure Portal and click on "Scale-up." To scale out, click on "Scale-out" and specify the number of units you want to run in parallel.
Auto-scaling rules can be set to automatically adjust the number of units based on usage metrics, ensuring your service is always right-sized. You can create rules based on metrics like the number of connections or messages per second.
Monitoring and diagnostics are also crucial for tracking performance and troubleshooting issues. Azure SignalR Service provides diagnostic logs that capture information about service activities and usage.
To set up monitoring and diagnostics, navigate to your SignalR service in the Azure Portal and select "Diagnostic settings." You can choose to monitor categories like "Service and performance counters" or "Connectivity", and configure the settings accordingly.
Discover more: Cascading Style Sheet Rules
Scale and Optimize
Scaling your application is a crucial step in ensuring it can handle varying workloads. You can configure scaling options in the Azure Portal, specifically for the Azure SignalR Service you've created.
To scale up, you can increase the capacity of your service by changing the pricing tier. This will give your SignalR service more available resources.
Scaling up is done by clicking on "Scale-up" in the Azure Portal. You can scale out by adding more instances of your SignalR service, which helps distribute the load across multiple instances.
To scale out, click on "Scale-out" in the Azure Portal and specify the number of units you want to run in parallel. You can also configure auto-scaling rules to automatically adjust the number of units based on usage metrics.
Auto-scaling rules can be created based on metrics like the number of connections or messages per second. You can define the minimum and maximum number of units for auto-scaling by clicking on "Auto-scale" in the Azure Portal.
Fine-tuning how hubs and connections are managed can also help optimize your application. You can configure hub methods for load distribution and optimization, and implement connection management to disconnect idle connections when necessary.
See what others are reading: Azure Metrics
Monitoring and Diagnostics
Monitoring and diagnostics are crucial for tracking the performance and troubleshooting issues with your Azure SignalR Service. You can set up monitoring and diagnostics by clicking on your SignalR service in the Azure Portal.
To get started, navigate to the diagnostic logs that capture information about service activities and usage. In the Azure Portal, go to your SignalR service and select "Diagnostic settings" under "Settings."
Choose the diagnostic categories you want to monitor, such as "Service and performance counters" or "Connectivity." You can also select a destination for the diagnostic logs, typically Azure Monitor, and configure the settings accordingly.
Save the diagnostic setting and configure metrics collection to understand the performance and usage of your SignalR service. Azure SignalR Service provides metrics like connections, messages sent, and messages received.
Set up alerts to be notified of critical issues based on metric thresholds, diagnostic logs, or other conditions. You can create alerts based on specific criteria, such as metric thresholds, conditions, and alert actions.
By integrating Application Insights with your SignalR service, you can gain a deeper understanding of your application's performance, including interactions with the SignalR service. You can choose to link an existing Application Insights resource or create a new one.
Expand your knowledge: Azure App Insights vs Azure Monitor
Frequently Asked Questions
Is SignalR deprecated?
SignalR is deprecated by Microsoft, meaning it's no longer actively maintained or updated. For the latest real-time web functionality, consider using Azure SignalR Service or other recommended alternatives.
What is the difference between Azure SignalR and socket io?
Azure SignalR is a library for ASP.NET developers that simplifies real-time web development, whereas Socket.IO is a more comprehensive framework for real-time applications with bidirectional communication capabilities. The key difference lies in their scope and complexity, with SignalR being a more streamlined solution and Socket.IO offering a more detailed and event-based approach.
Is Azure SignalR high availability?
Azure SignalR Service offers 99.9% availability, but it's a regional service that doesn't automatically fail over to another region in case of a region-wide outage. This means you may need additional redundancy to ensure high availability for your application.
What is the difference between Azure SignalR service serverless and default?
Key difference: Azure SignalR Service Serverless mode doesn't require a hub server, relying on the service to manage client connections, whereas Default mode requires a running hub server for connection management
Sources
- https://learn.microsoft.com/en-us/dotnet/api/overview/azure/microsoft.azure.webjobs.extensions.signalrservice-readme
- https://medium.com/@darshana-edirisinghe/implement-real-time-applications-using-azure-signalr-b1cd9dc39fd2
- https://www.nuget.org/packages/Microsoft.Azure.SignalR
- https://learn.microsoft.com/en-us/azure/azure-signalr/signalr-quickstart-dotnet-core
- https://dev.to/jayendran/securing-azure-signalr-azure-app-service-part-1-bko
Featured Images: pexels.com