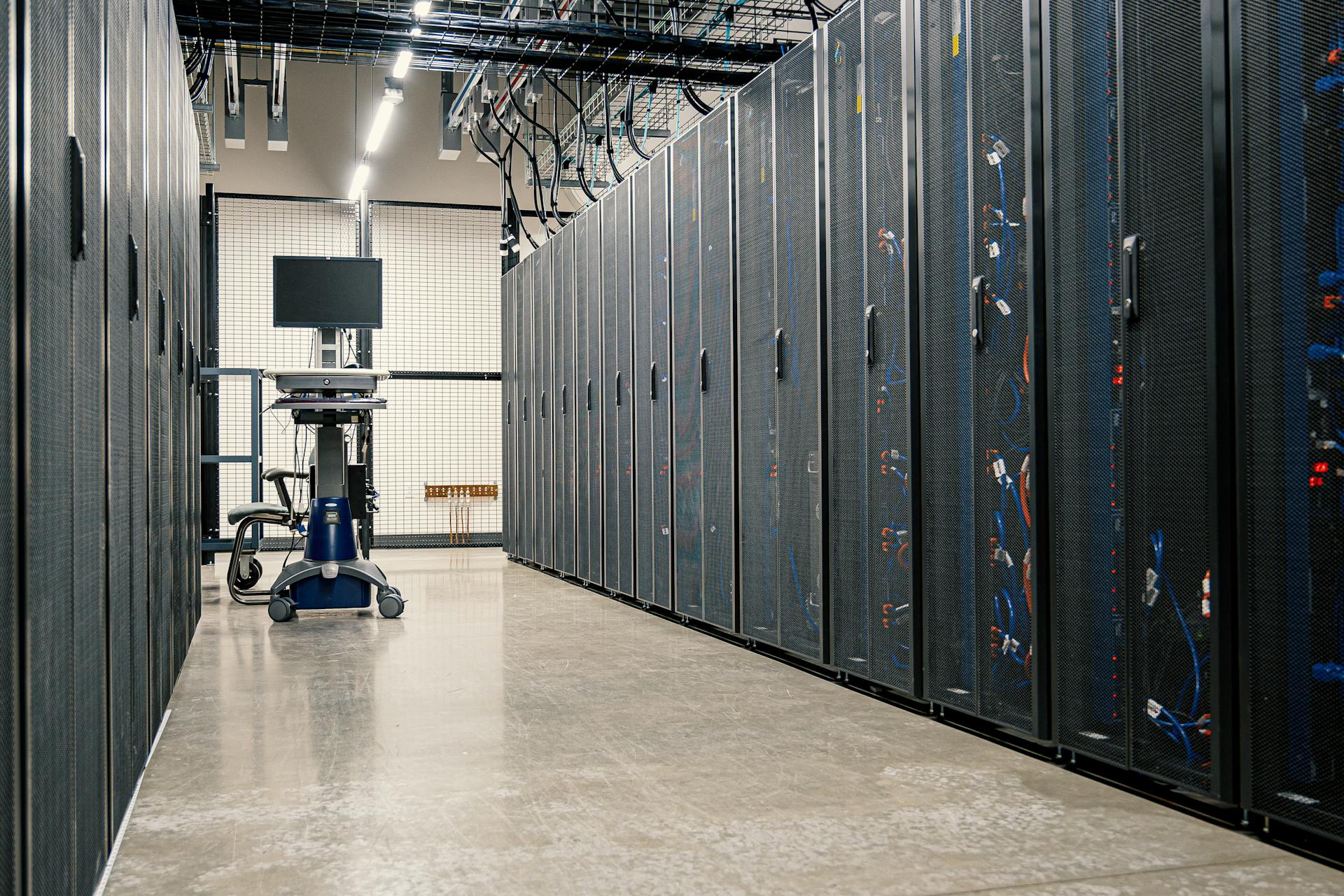
Developing Node.js applications on Azure can be a game-changer for businesses, offering scalability, reliability, and cost-effectiveness.
Azure Functions can be used to create serverless APIs, which are perfect for handling sudden spikes in traffic or requests.
By integrating Redis with Azure Functions, you can build real-time data pipelines that can handle high-volume data streaming.
This combination enables you to process and analyze large amounts of data in a highly scalable and efficient manner.
Prerequisites for Developing
To develop Azure Node.js applications, you'll need a few essential tools in place.
First and foremost, you'll need an Azure subscription, which you can create for free.
You'll also need Node.js installed on your computer, which you can do by following the instructions on how to install Node and npm on a Windows computer.
Here are the specific requirements for developing Azure Functions:
- Node.js LTS
- Azure Functions Core Tools
It's worth noting that having the latest version of Node.js and Azure Functions Core Tools will ensure you have the best possible experience.
Here's a summary of the prerequisites you'll need:
- The @azure/functions npm package version 4.3.0 or later.
- Azure Functions runtime version 4.28 or later.
- Azure Functions Core Tools version 4.0.5530 or a later version.
Make sure your function app is running on the latest version of the Azure Functions runtime before upgrading your Node.js version.
Readers also liked: Azure Version Control
Azure Services
Azure Functions can be integrated with other Azure services to create powerful and scalable applications. This integration allows you to leverage the strengths of each service to build robust solutions.
You can create functions that are triggered by other Azure services using trigger bindings, or use the Azure SDKs for more control and coordination.
To integrate Azure Functions with other Azure services, you can use the following methods:
- Low-code functions: Use trigger bindings to create functions that are triggered by other Azure services.
- High-code functions: Use the Azure SDKs to coordinate and control other Azure services.
Azure Functions can also be used to build serverless APIs, refactor Node.js Express APIs, and upload and analyze files with Blob Storage.
Prerequisites
To get started with Azure Services, you'll need to meet some prerequisites. You can create an Azure subscription for free, so don't worry about costs.
To install Node.js, which is required for Azure Services, follow the instructions on the Microsoft website for installing Node.js on Windows.
You'll also need to install the Azure Functions Core Tools, which can be used to develop and test Azure Functions. Specifically, you'll need Node.js LTS and the Azure Functions Core Tools.
To ensure compatibility, make sure you have the correct versions of the @azure/functions npm package, Azure Functions runtime, and Azure Functions Core Tools. The minimum required versions are:
- The @azure/functions npm package version 4.3.0 or later.
- Azure Functions runtime version 4.28 or later.
- Azure Functions Core Tools version 4.0.5530 or a later version.
Managed Redis Instance
A Managed Redis Instance is a powerful tool for caching data in Azure. You can create one in the Azure portal by selecting Create a resource and then Databases > Azure Cache for Redis.
To get started, sign in to the Azure portal and select Create a resource. On the New page, select Databases and then Azure Cache for Redis. You'll then configure the settings for your new cache, including the subscription, resource group, DNS name, location, cache type, and more.
The DNS name must be unique in the region and must be a string of 1 to 63 characters that contains only numbers, letters, and hyphens. The name must start and end with a number or letter, and it can't contain consecutive hyphens.
Worth a look: Create Sample Azure Function for .net Frame Work
You can choose from different cache types, including Standard and Premium, which determine the performance and feature parameters of the cache. The cache size also varies depending on the SKU you select.
Once you've configured the settings, select Next: Networking and choose a connectivity method for the cache. Then, select Next: Advanced and verify or select an authentication method, such as Microsoft Entra ID with managed identities.
Here are the settings you'll need to configure when creating a Managed Redis Instance:
Integrate with Other Services
Integrate with other Azure services to unlock the full potential of your applications. With Azure Functions, you can create functions that are triggered by other Azure services or that output to other Azure services using trigger bindings.
You can use low-code functions for a more straightforward integration experience. This approach allows you to create functions that interact with other Azure services without needing to write a lot of code.
Discover more: Azure Code
For more control, you can use the Azure SDKs to coordinate and control other Azure services. This high-code approach gives you fine-grained control over the integration process.
To integrate with other Azure services, you can use trigger bindings to connect your functions to other services. This enables you to create functions that are triggered by events in other services.
Here are some examples of integrating with other Azure services:
By integrating with other Azure services, you can create more powerful and scalable applications that can handle a wide range of tasks and workflows.
Cloud Environment
In the Azure cloud environment, you can set and use Application settings, such as service connection strings, and expose them as environment variables during execution.
You can add, update, and delete function app settings in several ways, including through the Azure portal, Azure CLI, or Azure PowerShell.
Changes to function app settings require your function app to be restarted.
Here are the ways to add, update, and delete function app settings:
Identity and Authentication
Microsoft Authentication Library (MSAL) allows you to acquire security tokens from Microsoft identity to authenticate users.
To authenticate users, you can use the JavaScript Azure identity client library, which uses MSAL to provide token authentication support. Install this library using npm.
You can create a new Node.js app using Microsoft Entra ID, which involves adding environment variables for your Host name and Service Principal ID.
Here are the environment variables you need to add:
- AZURE_MANAGED_REDIS_HOST_NAME
- REDIS_SERVICE_PRINCIPAL_ID
These environment variables are used to connect to an Azure Managed Redis instance using the cache host name and key.
Install JavaScript Identity Client Library
To install the JavaScript Azure identity client library, you'll need to use npm, the package manager for Node.js.
The library you want to install is the Microsoft Authentication Library (MSAL), which allows you to acquire security tokens from Microsoft identity to authenticate users.
You can install the library using the command npm install @azure/identity.
Broaden your view: Pip Install Azure
Microsoft Entra ID
Microsoft Entra ID is a powerful tool for managing identities and authentication. It's a Microsoft service that allows you to securely authenticate and authorize users, devices, and applications.
Microsoft Entra ID access tokens have a limited lifespan, averaging 75 minutes, which means you need to refresh the token to maintain a connection to your cache. This is demonstrated in Example 2, where a script is used to reauthenticate and reconnect to the Azure Cache for Redis.
To use Microsoft Entra ID, you need to create a service principal or user, and obtain the object ID, also known as the Service Principal ID. This ID is used as the username when connecting to the Azure Cache for Redis, as shown in Example 1.
You can use the `DefaultAzureCredential` class to construct a token credential from the Identity library, which is then used to fetch a Microsoft Entra token for authentication. This token is used as the password when connecting to the Azure Cache for Redis.
You might like: Access Token Azure
Here are some common settings you need to configure for your Azure Function to keep it secure:
- Configuration settings
- API CORS - configure your client domains, don't use *, indicating all domains
- TLS/SSL setting for HTTPS - enable HTTPS only in the TLS/SSL settings
- Deployment Slots - create a deployment slot, such as stage or preflight and push to that slot
Microsoft Entra ID also provides a way to refresh the token when it expires, which is essential for maintaining a connection to the cache. This is demonstrated in Example 2, where a script is used to reauthenticate and reconnect to the Azure Cache for Redis.
Functions
Azure Functions are a great way to create serverless APIs with Node.js. You can create durable, stateful functions that retain state or manage long-running functions in Azure.
To register a function, you need a function.json file located in a folder one level down from the root of your app, and a JavaScript file that exports your function. The function should be declared as an async function, and you can specify configuration specific to the trigger directly on the options argument.
You can run your functions from a package file to speed up the cold start process, especially for Node.js apps with large dependency trees. This can help reduce the time it takes for your function app to start up after a period of inactivity.
Access a Cache
To access a cache, you need to connect to it from your application. This can be done using Microsoft Entra ID or access keys. I recommend using Microsoft Entra ID for optimal security and ease of use.
You can use a Node.js app to access a cache, and Azure Managed Redis (preview) instance is supported. To create a Node.js app, you can follow the instructions in Example 2.
To use Microsoft Entra ID authentication, you need to use a managed identity to authorize requests against your cache. This provides superior security and ease of use over shared access key authorization.
Here are the steps to follow for Microsoft Entra ID authentication:
- Use a managed identity to authorize requests against your cache.
- Provide the managed identity to your Node.js app to connect to the cache.
After you've created your Node.js app and connected to the cache, you can start using the cache to store and retrieve data. You can monitor the progress of the deployment on the Azure Cache for Redis Overview pane, and when the Status displays Running, the cache is ready to use.
What Is a Function?
A Function is a logical unit that contains all related functions in a single geographic location, allowing you to group functions together for easier management.
You can select from many common functions or create your own, giving you the flexibility to tailor your Function to your specific needs.
A Function resource can contain a single function or many functions, which can be independent of each other or related with input or output bindings.
This means you can have multiple functions working together seamlessly, or use them independently as needed.
The Function resource settings include typical serverless configurations such as environment variables, authentication, logging, and CORS.
Durable, Stateful Functions
Durable Functions are a game-changer for long-running tasks in Azure. They retain state, allowing you to manage complex workflows with ease.
You can create your first durable function in JavaScript, making it an ideal choice for developers who are already familiar with the language.
Durable Functions are specifically designed to handle long-running tasks, which can be a challenge for traditional functions. They can persist data and pick up where they left off, even after a restart or failure.
By using durable functions, you can simplify your code and focus on the logic of your application, rather than worrying about the underlying infrastructure.
Registering a Function
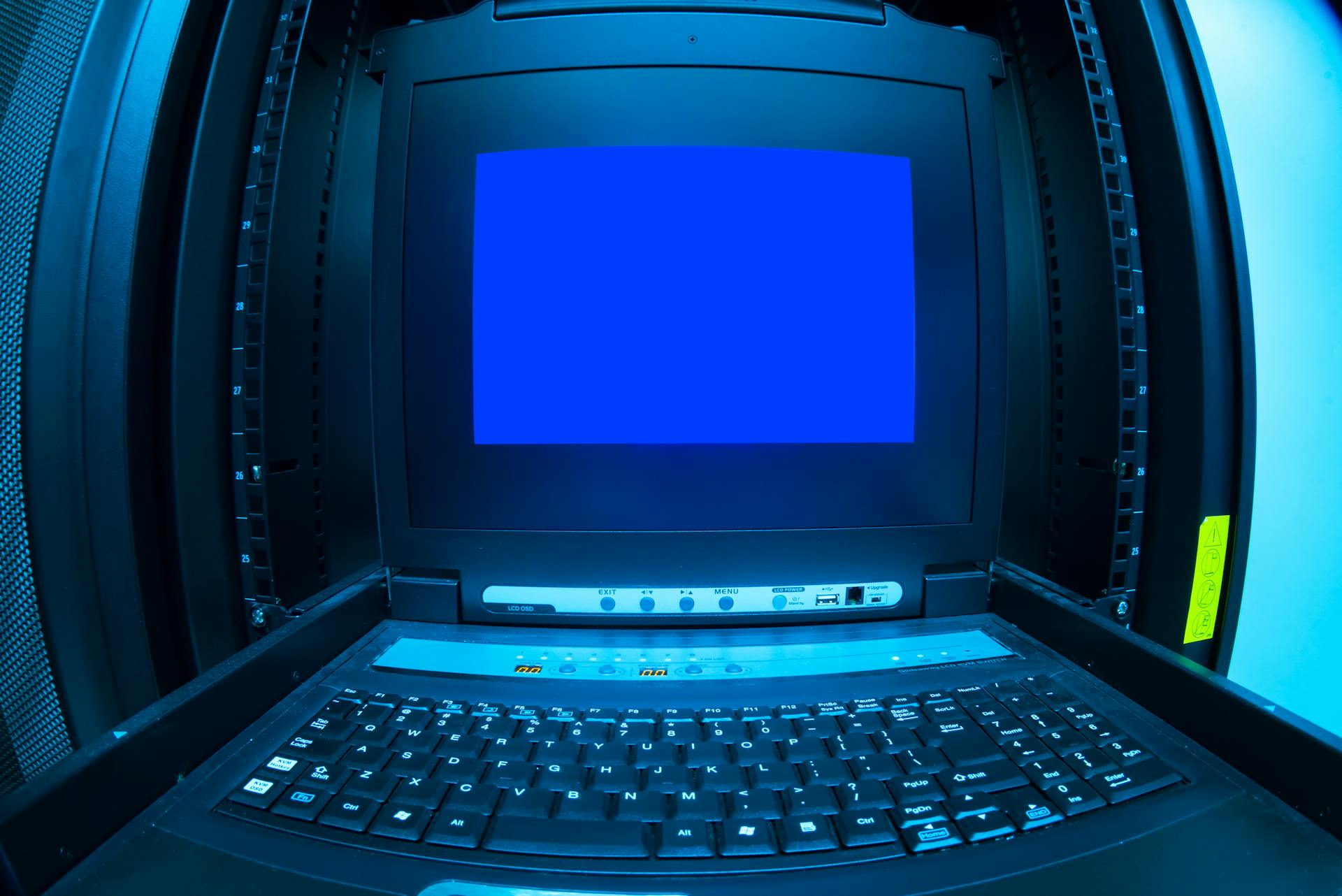
To register a function, you need a function.json file located in a folder one level down from the root of your app.
The v3 model looks for an index.js file in the same folder as your function.json, unless you're using TypeScript, in which case you must use the scriptFile property in function.json to point to the compiled JavaScript file.
You can export a synchronous function, but it's recommended to use an async function, which is the default.
The function you export should be declared as an async function, and it's passed an invocation context as the first argument and your inputs as the remaining arguments.
Your function is passed an invocation context as the first argument and your inputs as the remaining arguments.
You can register a function from any file in your project, as long as that file is loaded (directly or indirectly) based on the main field in your package.json file.
For your interest: Azure Auth Json Website Azure Ad Authentication
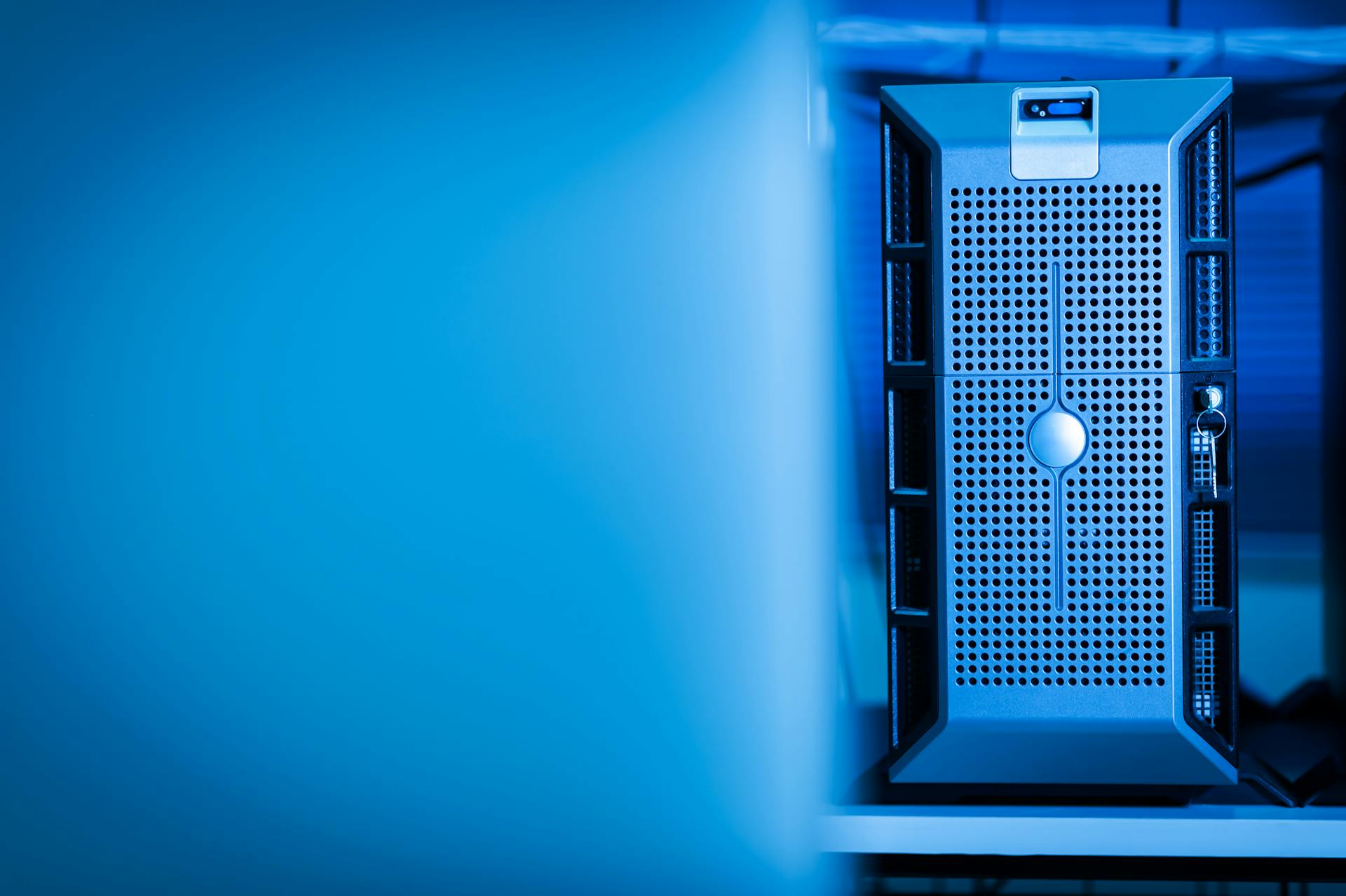
To customize the file location or export name of your function, see configuring your function's entry point.
Here are some examples of how to register functions from different file locations:
The first argument when registering a function is the function name, and the second argument is an options object specifying configuration for your trigger, your handler, and any other inputs or outputs.
Language Node Arguments
In Node.js, you can access function arguments in two ways. You can use the arguments passed to your function directly, or you can access them as properties of the context.bindings object.
The recommended way to access function arguments is by using the arguments passed to your function. This is done by listing the arguments in the same order they're defined in function.json. You can then use these arguments in your function code.
You can also access function arguments as properties of the context.bindings object. This is done by using the key matching the name property defined in function.json.
Here's an example of how to access function arguments using both methods:
- As arguments passed to your function: Use the arguments in the same order that they're defined in function.json. The name property defined in function.json doesn't need to match the name of your argument, although it's recommended for the sake of organization.
- As properties of context.bindings: Use the key matching the name property defined in function.json.
For example, if your function.json file contains the following bindings:
```
{
"name": "myTrigger",
"type": "http",
"direction": "in"
},
{
"name": "myInput",
"type": "blob",
"direction": "in"
},
{
"name": "myOtherInput",
"type": "queue",
"direction": "in"
}
```
You can access these arguments in your function code like this:
```
module.exports = async function (context, myTrigger, myInput, myOtherInput) { ... };
```
Or, you can access them as properties of the context.bindings object like this:
```
module.exports = async function (context) {
context.log("This is myTrigger: " + context.bindings.myTrigger);
context.log("This is myInput: " + context.bindings.myInput);
context.log("This is myOtherInput: " + context.bindings.myOtherInput);
};
```
You might enjoy: Azure Azure-common Python Module
Retry Context
The retryContext object is a crucial part of our functions, and it has a few key properties that are worth noting. The retryContext object has the following properties.
One of the most important properties is the one that allows us to track the number of retries. The retryContext object has this property. It's a simple yet effective way to keep track of how many times our function has attempted to execute.
This property can be especially useful when debugging issues or optimizing our functions for performance. The retryContext object also has other properties, but this one is particularly useful in certain situations.
Develop Locally with Visual Studio Code
Developing Azure Functions locally with Visual Studio Code is a breeze. You can create your first function using Visual Studio Code, which simplifies many of the details with the Azure Functions extension.
This extension helps you create JavaScript and TypeScript functions with common templates. Serverless functions remove much of the server configuration and management, so you can focus on just the code you need.
To get started, you'll need Node.js LTS and the Azure Functions Core Tools. With Visual Studio Code, you can create and run your functions locally without any hassle.
Here's a brief overview of the Azure Functions with Node.js:
Local debugging is also a piece of cake with VS Code. It starts your Node.js process in debug mode automatically and attaches to the process for you. If you're using a different tool for debugging or want to start your Node.js process in debug mode manually, you can add "languageWorkers__node__arguments": "--inspect" under Values in your local.settings.json.
See what others are reading: Azure Function Local Settings Json
Setting Node Version
You can set your Node.js version using the Azure CLI or in the Azure portal. To update the Node.js version for your function app running on Windows, use the Azure CLI command az functionapp config appsettings set.
The WEBSITE_NODE_DEFAULT_VERSION application setting can be updated either by using the Azure CLI or in the Azure portal. This setting specifies the Node.js version to use, both at runtime and during automated package restore in Kudu.
To change the Node.js version, you can run the Azure CLI command az functionapp config appsettings set. This sets the WEBSITE_NODE_DEFAULT_VERSION application setting to the supported LTS version of ~20.
For function apps running on Linux, the Node.js version is set by the linuxfxversion site setting. This setting can be updated using the Azure CLI command az functionapp config set.
Broaden your view: Azure Cli Macos
Scaling and Concurrency
Azure Functions automatically monitors load and creates more host instances for Node.js as needed. This behavior is sufficient for many Node.js applications.
For CPU-bound applications, you can improve performance by using multiple language worker processes. You can increase the number of worker processes per host from the default of 1 up to a max of 10 by using the FUNCTIONS_WORKER_PROCESS_COUNT application setting.
Using multiple processes can lead to unpredictable behavior and increase function load times, so use this setting with caution. It's highly recommended to offset these downsides by running from a package file.
Azure Functions runs Node.js functions more efficiently on single-vCPU VMs. Using larger VMs doesn't produce the expected performance improvements.
Readers also liked: How to Connect to Azure Cosmos Db Using Connection String
Function Configuration
Function configuration is a crucial aspect of Azure Node.js development. You can define the threshold level to be used when tracking and viewing logs by setting the logging.logLevel property in the host.json file.
This property lets you define a default level applied to all functions, or a threshold for each individual function. To configure the function entry point, use the function.json properties scriptFile and entryPoint. The scriptFile property is required when you're using TypeScript and should point to the compiled JavaScript.
You can access environment variables using the standard Node.js pattern. For example, to access an app setting called NODE_ENV, use the following code. In the Azure cloud environment, you can set and use Application settings, such as service connection strings, and exposes these settings as environment variables during execution.
You can add, update, and delete function app settings in several ways, including the Azure portal, Azure CLI, and Azure PowerShell. Changes to function app settings require your function app to be restarted.
Note: The following table summarizes the ways to add, update, and delete function app settings:
Configure Server
You can configure your Node.js server to start with PM2, which is a production process manager that comes with the Node.js containers. PM2 provides a full-service app management platform.
For production or staging use, it's recommended to run your app with PM2. This will give you the benefits of a full-service app management platform.
If you're working on development, you can run your app with npm start. This is the recommended approach for development use only.
Alternatively, you can run your app with a custom command, which is suitable for either development or staging.
Here are the options for running your app:
Set Version
To set the version of your Node.js application, you should run a command in the Cloud Shell to set the WEBSITE_NODE_DEFAULT_VERSION to a supported version. This will ensure that your app uses a version of Node.js that is regularly patched and updated by the platform.
You can use the recommended "tilde syntax" to target the latest available version of Node.js 16 runtime on App Service. This is a good approach because the runtime is regularly updated, and targeting a specific minor version or patch is not recommended due to potential security risks.
To set the Node.js version in your project's package.json, you can use the following command:
Worth a look: Azure Functions Runtime Is Unreachable
This setting specifies the Node.js version to use, both at runtime and during automated package restore in Kudu.
Here are the supported versions of Node.js:
To update the Node.js version for your function app running on Windows, you can use the Azure CLI command `az functionapp config appsettings set`. This will set the WEBSITE_NODE_DEFAULT_VERSION application setting to the supported LTS version of ~20.
If your function app is running on Linux, you can use the `az functionapp config set` command to update the Node.js version. This will set the base image of the Linux function app to Node.js version 20.
You might like: Azure Linux Function App
Environment Variables
Environment variables are a convenient way to store operational secrets and environmental settings outside of your code. You can add them in both local and cloud environments.
You can access environment variables using the standard Node.js pattern, or through process.env in your function code. For example, to log the WEBSITE_SITE_NAME environment variable, you can use the following code.
Expand your knowledge: Azure Functions Environment Variables
Environment variables can be used to store connection strings, keys, endpoints, and other sensitive information. They're especially useful for profiling variables and other environmental settings.
Some Functions environment variables are specific to Node.js, such as those that allow you to access app settings set outside of your code. You can use these variables to access settings like NODE_ENV.
In App Service, you can set app settings outside of your app code and access them using the standard Node.js pattern.
Curious to learn more? Check out: Azure Environment Setup and Adf Setup
Configure Entry Point
The function.json properties scriptFile and entryPoint can be used to configure the location and name of your exported function.
To customize the file location or export name of your function, you must use the scriptFile property in function.json to point to the compiled JavaScript file.
You can use the entryPoint property to specify the name of your exported function, but it's not required unless you're using a specific configuration.
When using TypeScript, you must use the scriptFile property in function.json to point to the compiled JavaScript file.
You can use the following table to summarize the options for configuring the entry point of your function:
The entryPoint property is optional, but it can be useful if you need to specify a custom name for your exported function.
You can use the following example to see how to use the scriptFile property in function.json: "src/functions/*.js"
Check this out: How to Use Azure Key Vault C#
Frequently Asked Questions
What is an Azure Node?
An Azure Node is a virtual machine that handles a portion of your application's workload, with its size determining CPU cores, memory, and storage capacity. Understanding Azure Nodes is key to optimizing your application's performance and scalability.
How to deploy nodejs backend in Azure?
To deploy a Node.js backend in Azure, create a new web app by right-clicking App Services and selecting Create new Web App, then select Node as the runtime stack and Free (F1) pricing tier. This will get your Node.js backend up and running in Azure in no time.
How to install Node js on Azure?
To install Node.js on Azure, you need an active Azure subscription and then install Node.js and npm on your local machine. Verify the installation by running the command "node --version" in your terminal.
Sources
- https://learn.microsoft.com/en-us/azure/azure-cache-for-redis/cache-nodejs-get-started
- https://learn.microsoft.com/en-us/azure/developer/javascript/how-to/develop-serverless-apps
- https://learn.microsoft.com/en-us/azure/app-service/configure-language-nodejs
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-reference-node
- https://www.freecodecamp.org/news/how-to-deploy-node-js-app-on-azure/
Featured Images: pexels.com