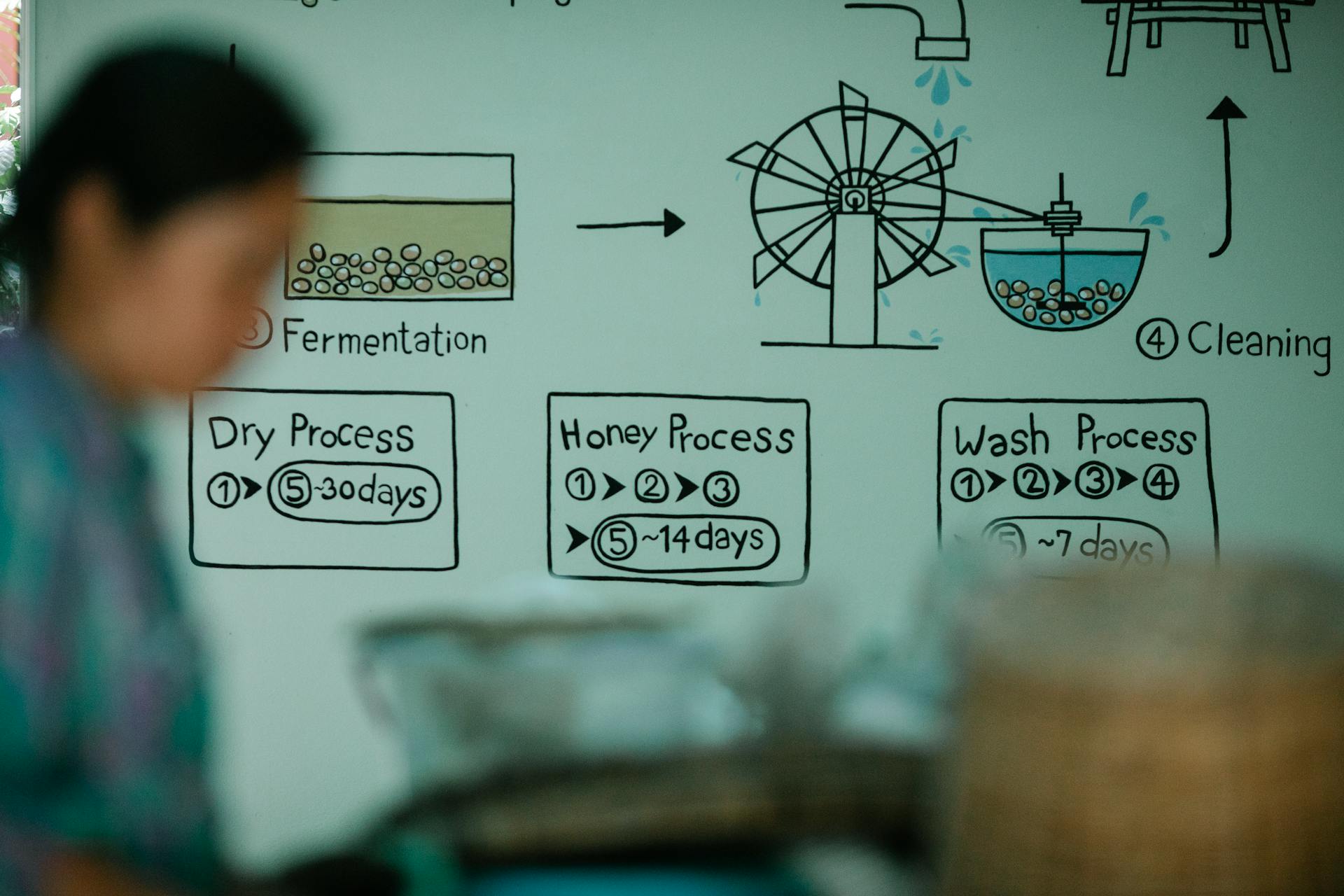
To get started with Azure MSAL React for your React applications, you'll need to install the necessary packages. Install the @azure/msal-react package using npm or yarn.
Azure MSAL React provides a simple way to authenticate users in your React applications. This is achieved through the use of a library that handles authentication for you.
To use Azure MSAL React, you need to create an instance of the PublicClientApplication class, passing your client ID and the redirect URI to the constructor. The client ID is the identifier for your Azure AD application.
You'll also need to configure the authentication settings for your application, such as the authority and the scopes you want to request. The authority is the URL of the Azure AD endpoint you're using, and the scopes determine the permissions you're requesting from the user.
A different take: Client Secret Azure Ad
Azure MSAL Configuration
When dealing with Azure MSAL configuration, it's essential to understand the differences between common and single-tenant apps. For common tenants, you only need to specify the clientId in your configuration object.
In a single-tenant app, you'll need to provide the authority field, which includes the audience specification, also known as the tenant ID. The authority field will look like this: "https://your-tenant-id.b2clogin.com".
You may also need to provide the knownAuthorities array if you're using a separate authority or identity server. For example, if your authority field is "https://your-tenant-id.b2clogin.com", your knownAuthorities field would be ["login.microsoftonline.com"].
Check this out: Azure App Id
App Configuration
To set up your Azure MSAL React app, you'll need to configure it properly. This involves understanding the configuration object, which is used to specify the authentication flow and other settings.
The configuration object can be specified using the Configuration type, which is available when using TypeScript in your project. This helps you see the available fields to add to your configuration.
When using the common cloud instance, your configuration might look like the example above, where you redirect the user to a route called root after successful authentication. This route can then check if the user is logged in and redirect them accordingly.
Discover more: Azure Auth Json Website Azure Ad Authentication
Specifying Your Audience in Code/Configuration
You should specify an audience in your code or configuration, as many tenants and applications will have guest users. This ensures your app behaves as expected.
MSAL assumes the common tenant by default, but if you're dealing with a single-tenant app, you need to provide the authority field with the audience specification, also known as the tenant ID.
You can specify the audience using one of the following values: the Microsoft Entra authority audience enumeration, the tenant ID, or one of the placeholders as a tenant ID in place of the Microsoft Entra authority audience enumeration.
MSAL will throw a meaningful exception if you specify both the Microsoft Entra authority audience and the tenant ID.
To specify a personal Microsoft account audience, you should set the app registration audience to Work and school accounts and personal accounts, and set the audience in your code/configuration to AadAuthorityAudience.PersonalMicrosoftAccount (or TenantID = "consumers").
A fresh viewpoint: Azure Msal
Here's a summary of the audience specification options:
Adding Packages
To get started with app configuration, we first need to add the necessary packages to our project.
The first package we'll add is @azure/msal-react, the library itself.
This library is a crucial part of the app configuration process, allowing us to authenticate users without a backend server.
The second package we need to add is @azure/msal-browser, a peer dependency that enables authentication without a backend server.
This package works in conjunction with @azure/msal-react to provide a seamless user authentication experience.
By adding these two packages, we're setting the stage for a robust and secure app configuration.
You might like: Azure Msal Angular
Switch to API
If you're looking to switch to a more modern API, you should consider MSAL.js. MSAL.js has replaced ADAL.js and offers improved functionality and security features.
Some of the public methods in ADAL.js have equivalents in MSAL.js, which can make the transition easier. Here are some key differences:
MSAL.js also offers new methods that are not available in ADAL.js, such as loginPopup and loginRedirect. These new methods can help simplify the authentication process.
For your interest: React Js Deploy Nginx in Azure Web App
Initialization and Setup
To initialize the public client application, you need to create an instance of PublicClientApplication, which expects a configuration file that provides information unique to your Azure app. This configuration file can contain fields like Client ID, Authority, and Redirect URI.
The Client ID is a mandatory field that identifies your Azure app, while Authority and Redirect URI are optional fields that specify the cloud instance and URL for signing users in.
To specify the cloud instance, you can use the identity instance, which defaults to Azure public cloud instances if not explicitly specified. If you want to restrict login to any Microsoft Entra account, use https://login.microsoftonline.com/organizations instead.
Here are the mandatory fields in the configuration file:
- Client ID — mandatory
- Authority — optional
- Redirect URI — optional
To initialize the authentication flow, you need to acquire the MSAL instance using the useMsal hook. This can be done in two ways: either in a popup or by using a redirect. The redirect option is preferable due to issues with popup blockers and Internet Explorer.
If this caught your attention, see: Access Azure Key Vault Using Service Principal C#
Initializing the Public Client
To initialize the public client application, you'll need to create an instance of the PublicClientApplication class, which requires a configuration file. This file contains information unique to your Azure app, such as the Client ID, Authority, and Redirect URI.
The configuration file must include the Client ID, which is a mandatory field. The Authority and Redirect URI are optional fields that can be included if needed.
The identity instance used for signing users in can be either the public cloud or national cloud. If no explicit instance is specified, msal-react will default to the Azure public cloud instances, which cover most use cases.
Here are the fields you'll need to include in the configuration file:
- Client ID — mandatory
- Authority — optional
- Redirect URI — optional
By following these steps, you'll be able to initialize the public client application and set up the necessary configuration for authentication.
Prerequisites
Before you can start setting up your Microsoft Entra app, you need to ensure you meet the necessary prerequisites.
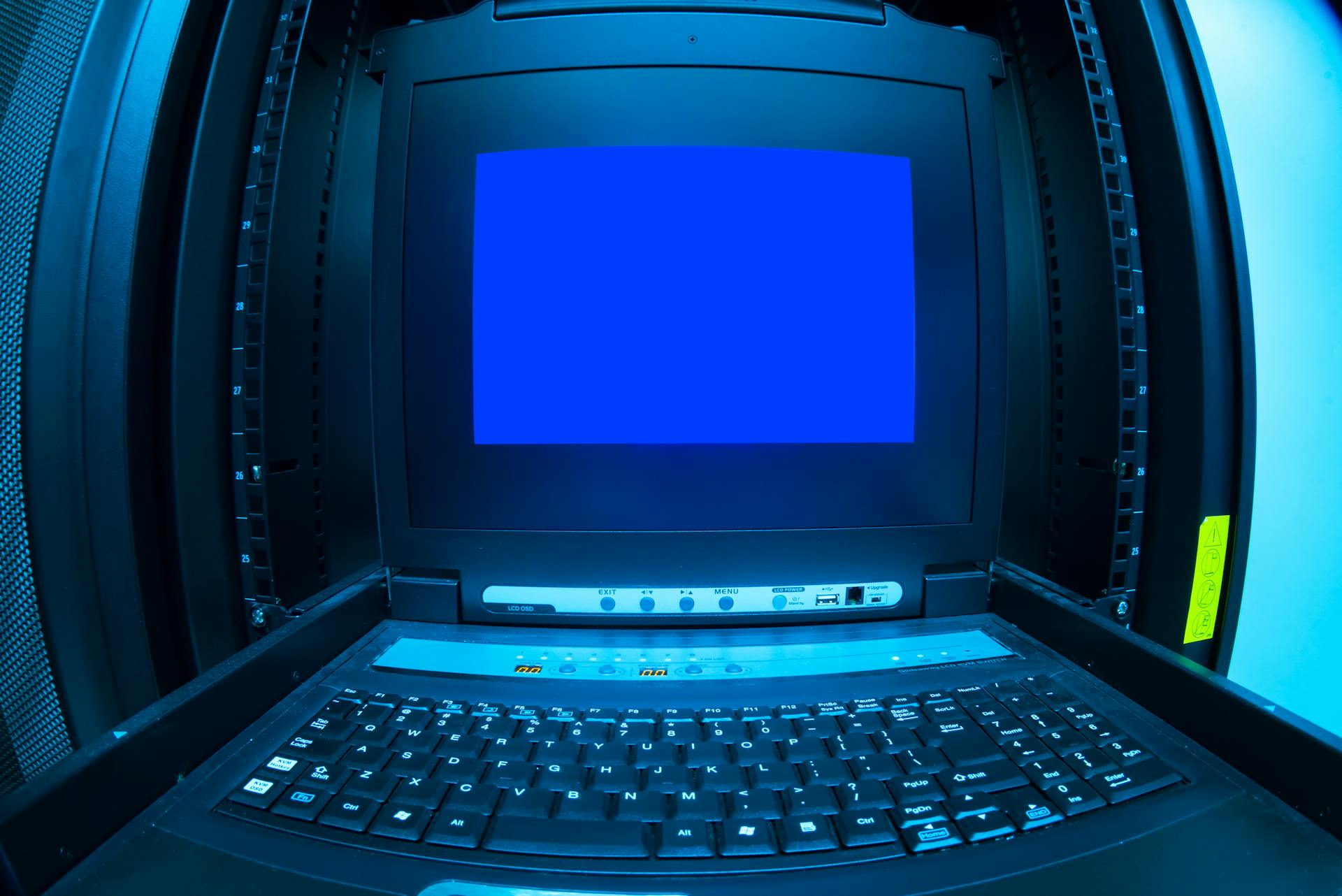
First and foremost, you must set the Platform / Reply URL Type to Single-page application on the App Registration portal. This is crucial, as having other platforms added in your app registration can lead to redirect URI restrictions.
You also need to make sure your redirect URIs don't overlap with other platforms, like Web. This is why it's essential to check the Redirect URI restrictions.
To make your app compatible with Internet Explorer, you'll need to provide polyfills for ES6 features that MSAL.js relies on, such as promises.
Lastly, if you haven't already, you'll need to migrate your Microsoft Entra apps to the v2 endpoint. This will ensure you're using the latest and most secure version of the platform.
A fresh viewpoint: Azure App Insights vs Azure Monitor
Configuration Object
The configuration object is a crucial part of using Azure MSAL React, and it's essential to understand how to set it up correctly.
You only need to specify the clientId in your configuration object if you're using the common tenant by default.
To configure a single-tenant app, you'll need to provide the authority field, which includes the audience specification, also known as the tenant ID.
The authority field will look like this: "https://your-tenant-id.b2clogin.com" if you're using a single-tenant app.
If you're using a separate authority or identity server, you'll need to provide the knownAuthorities array, which can include values like "login.microsoftonline.com".
You can check out the library docs for more information on the authority field.
The example configuration assumes you're using the common cloud instance and want to redirect the user to a route called "root" after successfully authenticating.
The config would look like this: { clientId: 'your_client_id', redirectUri: 'your_redirect_uri' }.
If you were to use login.live.com as an authority, your config would look like this: { clientId: 'your_client_id', authority: 'https://login.live.com' }.
Using TypeScript and specifying the Configuration type for the object will help you see the fields available to add to your configuration.
Consider reading: Azure Clientid
Auth Flow and User Management
Initializing the authentication flow is a crucial step in using Azure MSAL React. You can acquire the MSAL instance using the useMsal hook and then initialize the authentication in two ways – either in a popup or by using a redirect.
The popup method is not recommended as it can be blocked by popup blockers and has issues in Internet Explorer. The redirect option is preferable, allowing users to sign in or sign up on the Microsoft identity provider.
The configuration file is required to initialize the public client application object (pca). This file contains fields such as Client ID, Authority, and Redirect URI, which are mandatory or optional depending on the configuration.
Here are the mandatory fields in the configuration file:
- Client ID
The identity instance can be a public cloud or national cloud, and Azure MSAL React will default to the public cloud instance if not specified.
Auth Flow Initialization
Initializing the authentication flow is a crucial step in setting up user management for your application. You can initialize the authentication flow using the MSAL instance, which can be acquired using the useMsal hook.
There are two ways to initialize the authentication flow: using a popup or a redirect. The popup method keeps the user inside the app, but it's known to have issues in Internet Explorer and can be blocked by popup blockers.
The redirect option is preferable, as it takes the user to the Microsoft identity provider for signing in or signing up. To initialize the login redirect, you can press the "Sign in" text and use the loginRedirect method.
MSAL Angular v2 and MSAL React are wrappers libraries that expose the same public API as MSAL.js while offering framework-specific methods and components for streamlining authentication and token acquisition processes.
To initialize the public client application object (pca), you need to pass in a configuration file that contains information unique to your Azure app. The configuration file should include the Client ID, which is mandatory, as well as the Authority and Redirect URI, which are optional.
Here are the configuration file fields you should look over:
- Client ID — mandatory
- Authority — optional
- Redirect URI — optional
In ADAL.js and MSAL.js, you instantiate the AuthenticationContext and PublicClientApplication classes, respectively, using a configuration object that contains the clientId parameter at minimum. The authority URI defaults to https://login.microsoftonline.com/common if you don't specify it.
If you use the https://login.microsoftonline.com/common authority in v2.0, you will allow users to sign in with any Microsoft Entra organization or a personal Microsoft account (MSA).
You might like: Azure Computing
Handling User Signout
Handling user signout is a crucial aspect of the auth flow and user management. You can handle it by calling instance.logoutRedirect() to clear the browser cache.
The user will be navigated into the identity provider and then back into the app. This method should be used when a user logs out.
To configure the post-logout redirect location, you should also set a postLogoutRedirectUri in your config object. This will determine where the user is redirected after logging out.
You can add code to redirect the user after logging out by building on the code snippet above. Simply add the code below to redirect the user.
Use Scopes Instead of Resources
The MSAL.js library supports only the v2.0 endpoint, which employs a scope-centric model to access resources.
One of the key advantages of this model is the ability to use dynamic scopes, allowing users to provide incremental consent to scopes. This means you can request permissions at the time you need them, rather than requiring users to consent to all permissions upfront.
The v2.0 endpoint uses the scope parameter to request permissions, giving you more flexibility in how you manage user access. This is a significant improvement over the v1.0 endpoint, which required you to register static scopes required by your application.
By using scopes instead of resources, you can build applications that are more user-friendly and flexible. This approach also allows you to request additional permissions at a later time, rather than requiring users to re-consent to all permissions.
Use Promises
In MSAL.js, promises are used instead of callbacks, which makes the code easier to read and maintain. This is a significant improvement over ADAL.js, where callbacks were used.
You can also use the async/await syntax that comes with ES8, which allows you to write asynchronous code that looks and feels like synchronous code.
MSAL.js is designed to work with promises, so you can use them to handle asynchronous operations in a more elegant way.
Broaden your view: Azure Msal Browser
Here's a list of some key differences between ADAL.js and MSAL.js:
In general, MSAL.js is designed to be more modern and flexible than ADAL.js, and using promises is a key part of that.
Use the Events API
The Events API is a useful tool for tracking the authentication process in your app. It can be used to update the user interface, show error messages, and check if any interaction is in progress.
MSAL.js (version 2.4 and above) introduces this API, which provides a way to listen for events related to authentication.
You can use event callbacks to update the UI, show error messages, and check if any interaction is in progress. For example, an event callback can be used to handle login process failures.
For performance reasons, it's essential to unregister event callbacks when they're no longer needed. This will help prevent unnecessary processing and keep your app running smoothly.
Related reading: Azure Events
React and Azure Integration
React and Azure Integration is a breeze with the right tools. You can use the msal-react library available through Azure AD B2C to integrate authentication into your React app.
To get started, you'll need to register your frontend app in the AzureAD portal. This involves selecting the "New Registration" option and entering your app's redirect URL, which can be http://localhost:3000 for development purposes.
Here are the steps to register your app:
Once your app is registered, you can start integrating msal-react into your frontend app.
Effective Audience
Effective audience is a crucial aspect of integrating React with Azure. The effective audience for your application will be the minimum (if there's an intersection) of the audience you set in your app and the audience that's specified in the app registration.
To get an app to sign in users with only personal Microsoft accounts, you need to configure both app registration settings and code settings. This means setting the app registration audience to Work and school accounts and personal accounts, and setting the audience in your code/configuration to AadAuthorityAudience.PersonalMicrosoftAccount (or TenantID="consumers").
Here's a quick rundown of the required settings:
By following these steps, you can ensure that your React application effectively targets the correct audience and allows users to sign in with personal Microsoft accounts.
Project Integration
To integrate msal-react in your project, you'll need to have pre-existing experience with developing web apps using React.
You must also have set up a project and successfully integrated the authentication service on your backend.
The frontend app must be registered in the AzureAD portal, which involves going to portal.azure.com, into Azure Active Directory, then over to App Registrations, and selecting the “New Registration” option.
You can use http://localhost:3000 as the redirect URL for the purpose of this tutorial.
After completing these steps, you can start integrating msal-react in your frontend app.
Additional reading: How to Connect to Aks from Azure Portal
Wrapping the App
To integrate Azure AD with your React app, you need to wrap your app in the MsalProvider component. This is a requirement because the library uses the Context API.
This wrapper enables all components in your app to use the authentication result, which is crucial when building your router to limit access to certain areas of the app only to authenticated users.
The MsalProvider component is a wrapper that simplifies the authentication process, allowing you to focus on building your app rather than handling authentication complexities.
You can use the MsalProvider component to wrap your entire app or specific components that require authentication. This flexibility makes it easy to integrate Azure AD into your React app.
By using the MsalProvider component, you can streamline the authentication process and make it easier to manage authentication across your app.
Here's a brief overview of the benefits of using the MsalProvider component:
Sources
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-client-application-configuration
- https://blog.logrocket.com/using-msal-react-authentication/
- https://taerimhan.com/calling-microsoft-graph-using-azure-msal-react-from-pcf-control/
- https://learn.microsoft.com/en-us/entra/identity-platform/sample-v2-code
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-compare-msal-js-and-adal-js
Featured Images: pexels.com