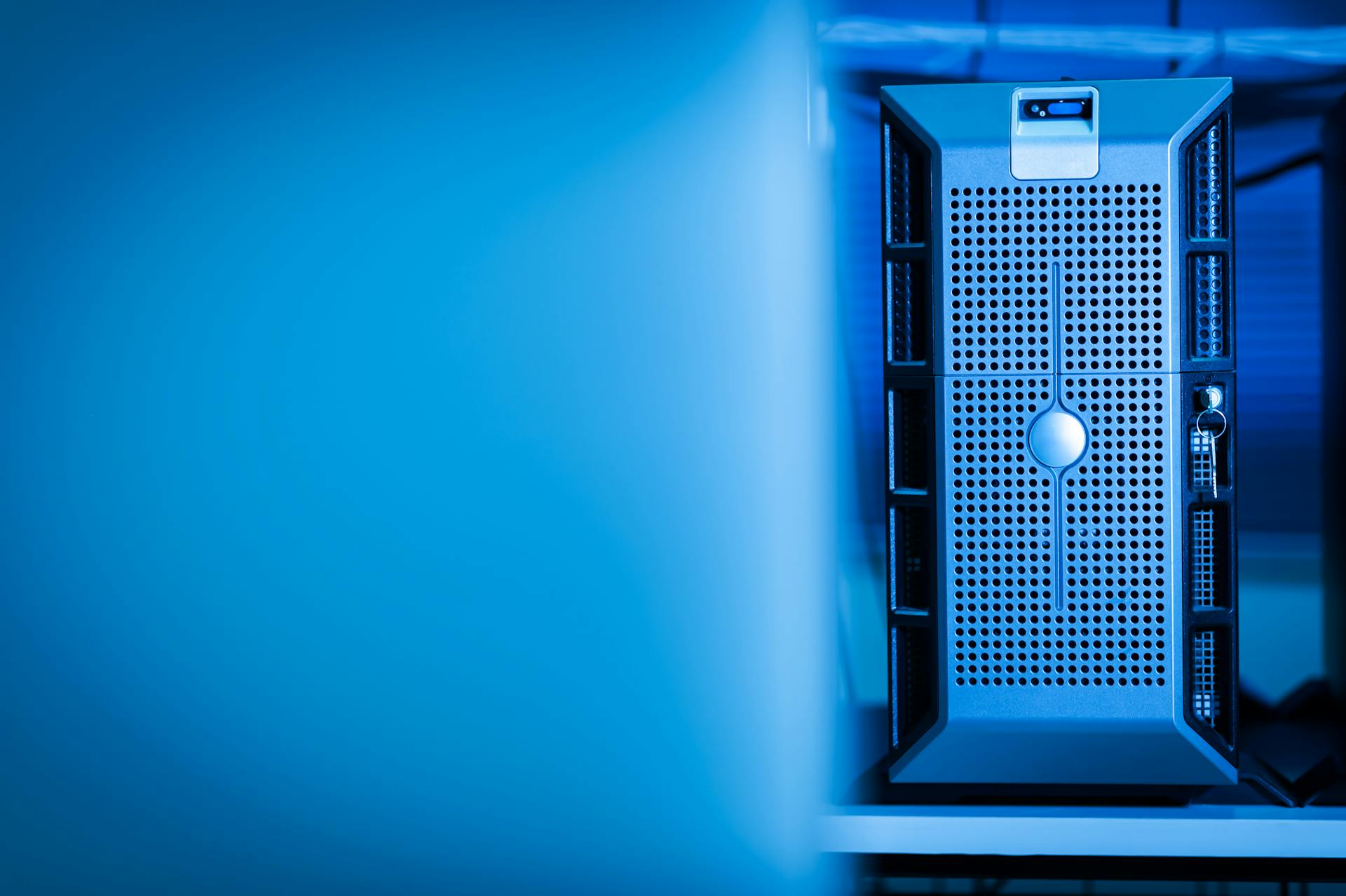
Azure Functions PowerShell is a powerful tool that allows you to create serverless functions using PowerShell scripts.
You can create a new Azure Functions project in Visual Studio by selecting the "Azure Functions" template and choosing "PowerShell" as the runtime.
To get started with Azure Functions PowerShell, you'll need to install the Azure Functions Core Tools using npm. This will give you access to the az function command, which you can use to create and manage your functions.
In the next step, we'll walk you through creating a simple Azure Functions PowerShell project using Visual Studio.
Check this out: Create Sample Azure Function for .net Frame Work
Configuring Azure Functions
Configuring Azure Functions is a crucial step in setting up your PowerShell-based Azure Functions. You'll need to finalize the Function App configurations in the Azure Portal.
To do this, go back to your function app and click on Configuration. Add the following application settings:
Next, you'll need to configure the function app to use PowerShell 7. Open the local.settings.json file and confirm that a setting named FUNCTIONS_WORKER_RUNTIME_VERSION is set to ~7. If it's missing or if it's set to another value, update the contents of the file.
If this caught your attention, see: Set Subscription Context Azure Powershell
Managed Identity Authentication
Managed Identity Authentication is a convenient and secure way to access Azure resources. It's managed by the platform, which significantly simplifies developers' lives.
To enable Managed Identity for your Azure Function, go to the "Identity" section, select "System assigned", and set the status to "On". This is extremely simple.
You can either use the managed identity directly for authentication or use it to fetch the certificate for authentication from an Azure Key Vault. Either way, the managed identity always needs to be enabled.
To authenticate with the Azure Function managed identity, you can use PnP.PowerShell, which supports authenticating to SharePoint Online with Azure managed identities.
You can also authenticate with a self-signed certificate, which you'll store in an Azure Key Vault. Your function app will fetch the certificate from the key vault using its managed identity.
To create an Entra ID application registration, go to the Azure Portal, click on Microsoft Entra ID, and select App registrations. Click on New registration, name the app registration after your function app, and click on Register.
Related reading: Azure Devops Use Service Connection in Powershell Task
You'll need to configure the application registration with permissions and a certificate. Copy the Application (client) ID to Notepad, go to the API permissions blade, and assign the necessary permissions. Then, go to the Certificates & secrets blade.
To finalize the Function App configurations, go back to your function app in the Azure Portal, click on Configuration, and add the following application settings:
To grant permissions to your system-assigned managed identity, go to the Subscription > Access control (IAM) > Add > Add role assignment > Select Reader Role > Find managed identity by the Function App’s name > Save.
PnP PowerShell
PnP PowerShell is a powerful tool for automating tasks in Azure Functions, but it can be a bit tricky to set up. You'll need to use version 2 of PnP PowerShell Core, which supports managed identity authentication to SharePoint Online.
To use PnP PowerShell in Azure Functions, you'll need to add it as a required module to load. This can be done by referencing the PnP.PowerShell module in the requirements.psd1 file, which can be found in the App files blade of your Function App resource in the Azure Portal.
On a similar theme: Do You Need Powershell for Azure
You'll also need to install the Azure modules, specifically Az.Accounts and Az.ManagedServiceIdentity, to enable managed identity authentication. It's recommended to install these modules explicitly rather than the entire Az module collection to avoid potential issues with dependencies and timeouts.
Here's a quick reference to get you started:
- Use version 2 of PnP PowerShell Core for managed identity authentication.
- Add the PnP.PowerShell module to the requirements.psd1 file.
- Install Az.Accounts and Az.ManagedServiceIdentity modules explicitly.
Create Local Project
To create a local project for PnP PowerShell, you'll first need to create a local Azure Functions project using Visual Studio Code.
Open the command palette in Visual Studio Code by selecting F1 (or Ctrl/Cmd+Shift+P), and then enter and select Azure Functions: Create New Project.
You'll be prompted to select a language for your function app project, so choose PowerShell to create a local PowerShell Functions project.
Next, select Azure Functions v4, as this version is required for the project.
You can skip selecting a template for your project's first function for now, as you'll add functionality later.
Finally, select how you'd like to open your project, and choose Open in current window to open Visual Studio Code in the folder you selected.
Visual Studio Code will install Azure Functions Core Tools if required and create a function app project in a folder, including the host.json and local.settings.json configuration files.
A fresh viewpoint: Azure Function Local Settings Json
Editing Files
Editing files is a breeze with PnP PowerShell, and you can do it directly in the Azure Portal. You can edit files like host.json and requirements.psd1 under the "App files" section on the Function App page.
If you're just experimenting or doing a proof of concept, you might not need to set up and edit files locally. In that case, you can do it all in Azure Portal.
Files like run.ps1 and function.json can be edited under the "Code + Test" section of the function's page. This is super convenient and saves you time.
Editing files in Azure Portal is a great way to avoid setting up local files and publishing to Azure. It's a more streamlined process that gets you up and running faster.
Take a look at this: Azure Blob Storage Move Files between Containers C#
Create Self-Signed Certificate
To create a self-signed certificate, you'll need to execute a script that generates the certificate files in the same directory where you run the script.
Adjust the $commonName and $password variable values to match your needs.
The script will generate the necessary certificate files.
Make sure to run the script in the correct directory to avoid any issues.
After executing the script, you'll have your self-signed certificate ready for use.
You might enjoy: How to Run Locally an Azure Funtion
Using PnP PowerShell
You can use PnP PowerShell to interact with SharePoint Online, and it's a great tool to have in your toolkit. PnP PowerShell is a set of PowerShell modules that allow you to perform various tasks, such as creating and managing SharePoint sites, lists, and libraries.
To use PnP PowerShell, you'll need to create an Azure Function App, which can be done by following the instructions in the "Create an Azure Function App" section. This will give you a basic function app that you can use as a starting point.
One of the challenges of using PnP PowerShell in Azure Functions is finding a stable build that allows you to use some of the newer PnP PowerShell modules. As Alans points out in the comments, it can be tricky to find a build that works.
To authenticate with PnP PowerShell, you can use a self-signed certificate, which can be stored in an Azure Key Vault. This allows your function app to fetch the certificate and use it to authenticate with SharePoint Online.
Related reading: Azure Functions .net 8
Here are the steps to add PnP PowerShell to the required modules to load:
- Go to the Function App resource in Azure Portal
- Open the App files blade
- Select requirements.psd1 from the dropdown
- Add a reference to the PnP.PowerShell module
- Remember to Save the changes
Note that managed identity authentication requires version 2 of PnP.PowerShell, while authentication with a certificate is also possible in version 1.
You can also use a script to downgrade the PowerShell version to 7.0, which is required for PnP PowerShell to work correctly. This can be done by running a script that is available on GitHub.
Note that you'll need to have the Azure modules (Az.Accounts and Az.ManagedServiceIdentity) installed in order to use managed identity authentication.
Sources
- https://demiliani.com/2024/01/30/list-all-azure-functions-with-older-runtime-with-powershell/
- https://ochzhen.com/blog/az-powershell-module-in-azure-functions
- https://laurakokkarinen.com/how-to-use-pnp-powershell-on-azure-functions-with-application-permissions/
- https://tech.nicolonsky.ch/azure-functions-powershell-modules/
- https://learn.microsoft.com/en-us/azure/azure-functions/durable/quickstart-powershell-vscode
Featured Images: pexels.com