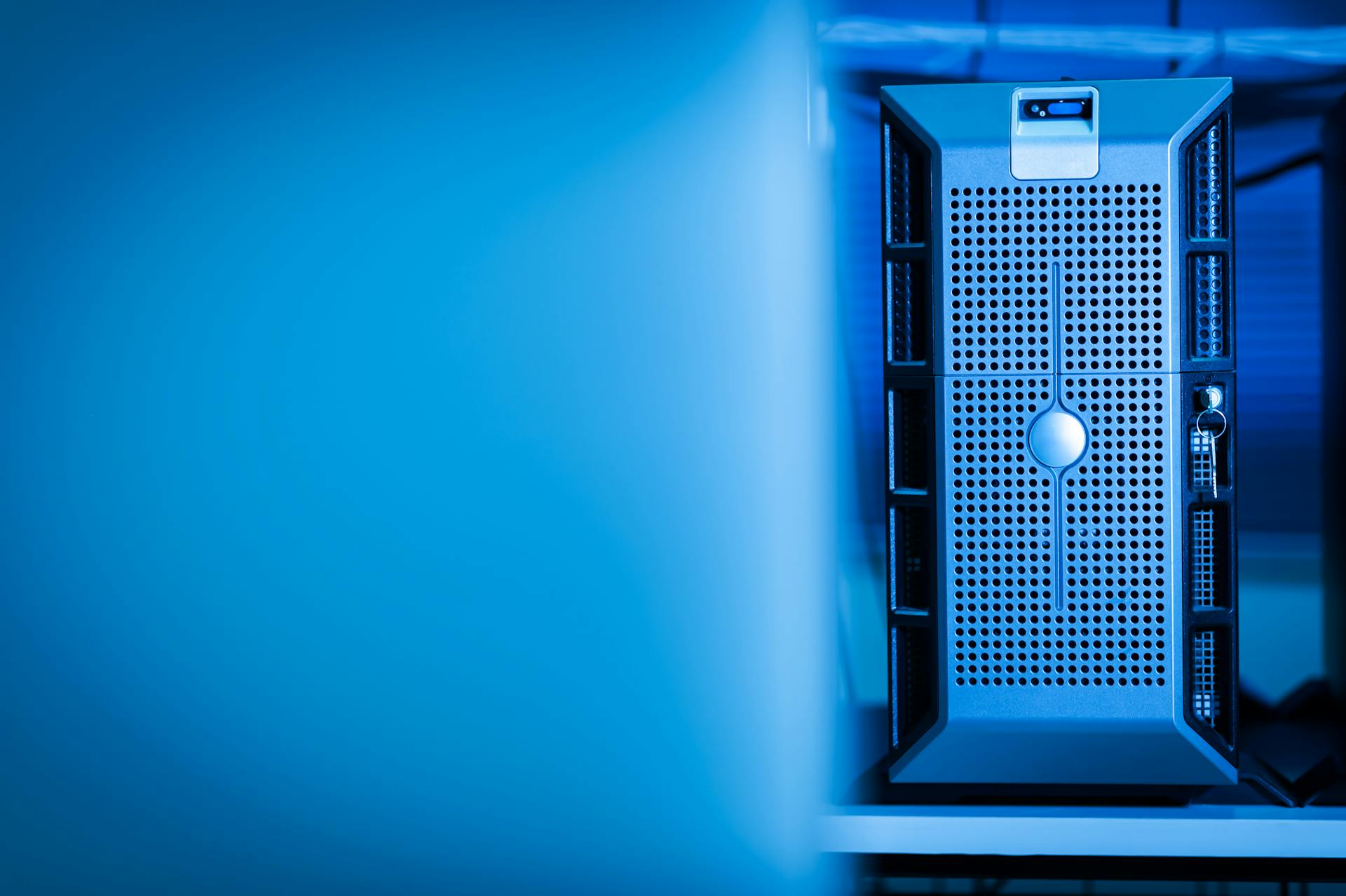
To get started with Azure Functions CLI, you'll need to install the Azure Functions Core Tools. This can be done by running the command `npm install -g azure-functions-core-tools@3` in your terminal.
The Azure Functions CLI is built on top of the Azure Functions Core Tools, which provide a set of commands for creating, deploying, and managing Azure Functions.
The CLI is designed to work with the Azure Functions runtime, which is a serverless compute platform provided by Azure.
To verify the installation, run the command `func --version` in your terminal.
On a similar theme: Azure Functions Core Tools
Azure Functions CLI Basics
You can list all function apps in a resource group using the command `az functionapp list`. This will display the default host name and state for all function apps, as well as show you which ones are running.
To get a list of functions in a specific function app, use `func azure functionapp list-functions`. This is a quick way to see what functions are available in your app.
If you need to install Functions extensions, you can use `func extensions install`. This command supports various options, such as installing a specific extension package version or using a custom NuGet feed source.
Related reading: Azure App Insights vs Azure Monitor
Commands
Creating a function app is as simple as using the `az functionapp create` command. You'll need to specify the name of the resource group, geographic location, and functions runtime stack.
To list all function apps in a specific resource group, use `az functionapp list`. You can also use this command to view the default host name and state for all function apps, or to list all running function apps.
If you want to check the available built-in stacks for function apps, use `az functionapp list-runtimes`. You can limit the output to just Windows or Linux runtimes.
To manually install Functions extensions in a non-.NET project or in a C# script project, use `func extensions install`. This command supports various options, such as specifying the path of the directory containing extensions.csproj, enabling C# scripting, or updating existing extensions.
You can also use `func azure functionapp list-functions` to return a list of the functions in a specified function app.
On a similar theme: Azure Linux Function App
Here's a summary of the commands mentioned:
Using `func settings list` will output a list of settings in the Values collection in the local.settings.json file, including connection strings. You can use the `--showValue` option to display the actual, unmasked values.
For another approach, see: Azure Cli Commands List
Init
The `func init` command is a great way to create a new Azure Functions project. It creates a new project in a specific language, either in a new folder or the current one.
You can specify the language using the `--language` option, which is currently supported for Node.js with options `typescript` and `javascript`. Alternatively, you can use `--worker-runtime` with values `javascript` or `typescript` to achieve the same result.
If you want to create a Dockerfile for your project, you can use the `--docker` or `--docker-only` options. These options are automatically supported for C#, JavaScript, Python, and PowerShell functions, but you'll need to manually create the Dockerfile for Java functions.
Check this out: Azure Function C
Some other options you can use with `func init` include `--force` to overwrite existing files, `--source-control` to create a git repository, and `--model` to set the programming model for your target language.
Here are the supported values for the `--worker-runtime` option:
- csharp
- dotnet
- dotnet-isolated
- javascript
- node
- powershell
- python
- typescript
- custom
- Maven (for Java)
Keep in mind that if you don't specify the `--worker-runtime` option, you'll be prompted to choose your runtime during initialization.
Consider reading: Azure Functions Runtime Is Unreachable
New
Creating a new function in Azure Functions is straightforward with the func new command. It's a great way to get started with a project, and you can even choose from a variety of templates.
You can run func new without the --template option, and you'll be prompted to select a template. In version 1.x, you'll also need to choose the language.
The func new action supports several options, including --authlevel, which lets you set the authorization level for an HTTP trigger. You can choose from function, anonymous, or admin.
Here are the options you can use with func new:
In version 2.x and later, you don't need to specify the language with --language, because it's defined by the worker runtime. The --name option is used to set the function name.
Install Core Tools
To install Core Tools, you'll need to download the installer based on your operating system. For Windows, you can choose between a 64-bit or 32-bit installer, with the 64-bit version being recommended for Visual Studio Code debugging.
You'll need to uninstall any old versions of Core Tools from Add Remove Programs before installing the latest version.
For macOS, you'll need to install Homebrew if it's not already installed, and then install the Core Tools package using the following commands: `brew tap azure/functions` and `brew install azure-functions-core-tools@4`. If you're upgrading from version 2.x or 3.x, you may also need to run `brew link --overwrite azure-functions-core-tools@4`.
For Ubuntu/Debian Linux distributions, you can use APT to install Core Tools. The specific commands to run are not provided in the article section, but you can find more information in the Core Tools readme.
Here are the installation methods for each operating system:
Restart (Appservice-Kube Extension)
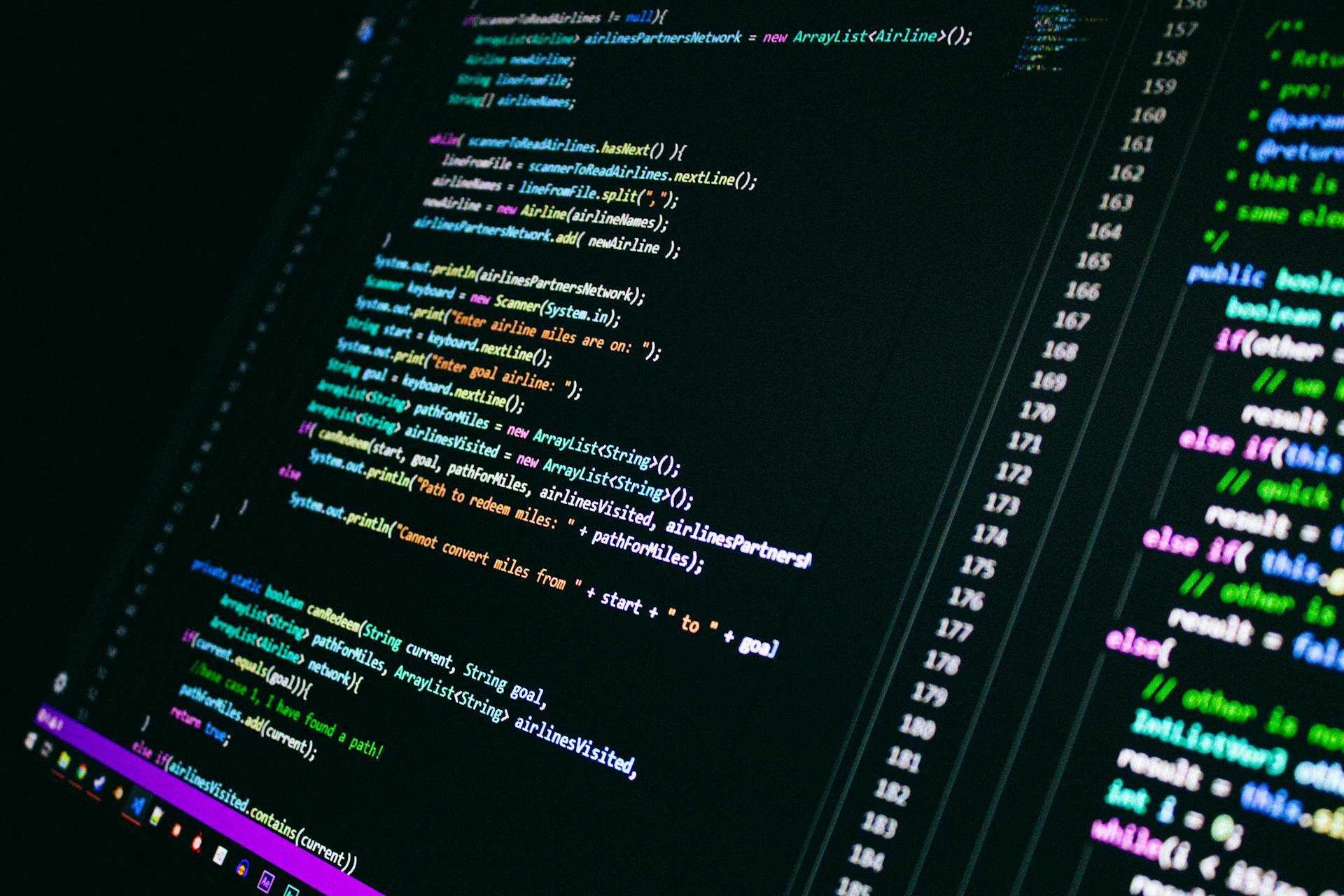
When using the Azure Functions CLI, you'll want to know how to restart your function app. The command to do this is az functionapp restart.
To restart your function app, you'll need to use the az functionapp restart command, which is part of the appservice-kube extension.
This command is particularly useful when you've made changes to your function app's configuration or code and want to apply them. Simply run az functionapp restart and your changes will be live.
The appservice-kube extension is a powerful tool that allows you to manage your Azure Functions deployments from the command line.
Update
Updating a function app is a straightforward process. You can do this by using the command "az functionapp update".
The command takes several options, including specifying the name or resource ID of the plan to update the function app with. This is a key step, as it allows you to customize the plan for your function app.
For your interest: How to Update Azure Cli Version
You can also remove properties or elements from a list using the "--remove" option. For example, you can remove a property from a list by specifying the index of the element to remove.
Updating an object involves specifying a property path and value to set. This can be done using the "--set" option, where you specify the property path and value to set.
A fresh viewpoint: Azure Cli Set Subscription
Creating Azure Functions
To create an Azure Function, you'll need to use the az functionapp create command. This command requires the function app's name to be unique and able to produce a FQDN as AppName.azurewebsites.net.
When enabling local git, you'll need to choose a geographic location where your function app will be hosted. You can use az functionapp list-flexconsumption-locations to view available locations.
To use the command, you'll also need to specify the name or ID of your subscription. You can configure the default subscription using az account set -s NAME_OR_ID.
A fresh viewpoint: .net 8 Azure Functions
Required Parameters
Creating Azure Functions requires a specific set of parameters to be set up correctly.
You'll need to specify the deployment storage account authentication type. This is a crucial step in ensuring secure access to your Azure Functions.
The name of the container app environment is also required. This will determine how your app is deployed and managed.
You'll need to set the minimum number of replicas when creating a function app on a container app. This ensures your app is always running with the required level of redundancy.
The OS type for the app to be created must be specified. This will determine the underlying operating system used by your Azure Functions.
A functionapp app service plan is also required, either by name or resource ID. If you're using an existing plan, make sure to use the full resource ID if it's in a different resource group.
You'll also need to provide a name or resource ID of an existing log analytics workspace to be used for the application insights component. This will help you monitor and troubleshoot your Azure Functions.
A Storage Account is required for non-kubernetes function apps, either by name in the provided Resource Group or by Resource ID in a different Resource Group.
Asynchronous deployment is also a required parameter. This ensures your Azure Functions are deployed efficiently and with minimal downtime.
Take a look at this: Docker Azure Cli Container
Create
To create an Azure function, you can use the Azure CLI command `az functionapp create`. This command requires several parameters, including the function app's name, geographic location, and subscription name. The name of the function app must be unique and able to produce a unique FQDN as AppName.azurewebsites.net.
You can enable local Git by running the `az functionapp create` command with the `--git` option. To view available locations, use the `az functionapp list-flexconsumption-locations` command. You can also use the `az account set` command to configure the default subscription.
If you want to create a function app in an app service Kubernetes environment, you can use the `az functionapp create` command with the `--kubernetes` option. This requires a name for the existing App Insights project, geographic location, and name or ID of the custom location.
When creating a function project locally, you can use the `func init` command to create a functions project in a folder named LocalFunctionProj. This command requires a runtime and target framework, such as `dotnet-isolated` and `net8.0`. You can then add a function to your project using the `func new` command, specifying the function's trigger and auth level.
To create supporting Azure resources for your function, you need to create a resource group, storage account, and function app. You can use the `az group create` command to create a resource group, and the `az storage account create` command to create a storage account. The function app can be created using the `az functionapp create` command, specifying the resource group, consumption plan location, runtime, and functions version.
Here are the required parameters for creating a function app:
- Deployment storage account authentication type
- Name of the container app environment
- Minimum number of replicas when creating a function app on container app
- Set the OS type for the app to be created
- Name or resource ID of the function app app service plan
- Name of an existing log analytics workspace to be used for the application insights component
- Storage account in the provided resource group or resource ID of a storage account in a different resource group
Here's a summary of the commands you can use to create an Azure function:
Note that these commands require specific parameters, such as the function app's name, geographic location, and subscription name. Be sure to check the documentation for each command to ensure you have the correct parameters.
Storage Fetch-Connection-String
Storage Fetch-Connection-String is a crucial step in creating Azure Functions. You can use the func azure storage fetch-connection-string command to get the connection string for your Azure Storage account.
A unique perspective: Azure Cli Upload File to Blob Storage
The connection string is essential for your Azure Function to access storage resources. For more information, see Download a storage connection string.
You can use the connection string to authenticate and authorize your Azure Function to access your Azure Storage account. This is typically done through environment variables or configuration settings.
Managing Azure Functions
Managing Azure Functions is a breeze with the Azure Functions CLI. You can connect to streaming logs for your function app in Azure using the `func azure functionapp logstream` command.
The default timeout for this connection is 2 hours, but you can change it by adding an app setting named SCM_LOGSTREAM_TIMEOUT with a timeout value in seconds.
If you're using a Linux app in the Consumption plan, you'll need to use the `--browser` option to view logs in the portal, as this feature isn't supported yet. This option will open Azure Application Insights Live Stream for your function app in the default browser.
For another approach, see: Azure Function Timeout
List-Consumption-Locations
To list available locations for running function apps, you can use the az functionapp list-consumption-locations command.
This command is a quick way to see where you can deploy your function apps, and it's especially useful if you're planning to deploy to a specific region.
You can run this command from the Azure CLI, and it will give you a list of available locations for running function apps.
Each location has its own set of pricing and features, so it's worth checking the list to see which ones meet your needs.
By using az functionapp list-consumption-locations, you can make informed decisions about where to deploy your function apps.
See what others are reading: Azure Logic App vs Function
Durable Delete-Task-Hub
If you're using Azure Functions, you might need to delete storage artifacts in your Durable Functions task hub. You can do this with the delete-task-hub action.
You can specify the storage connection string to use with the --connection-string-setting option. This is optional, but it's a good idea if you have multiple storage accounts set up.
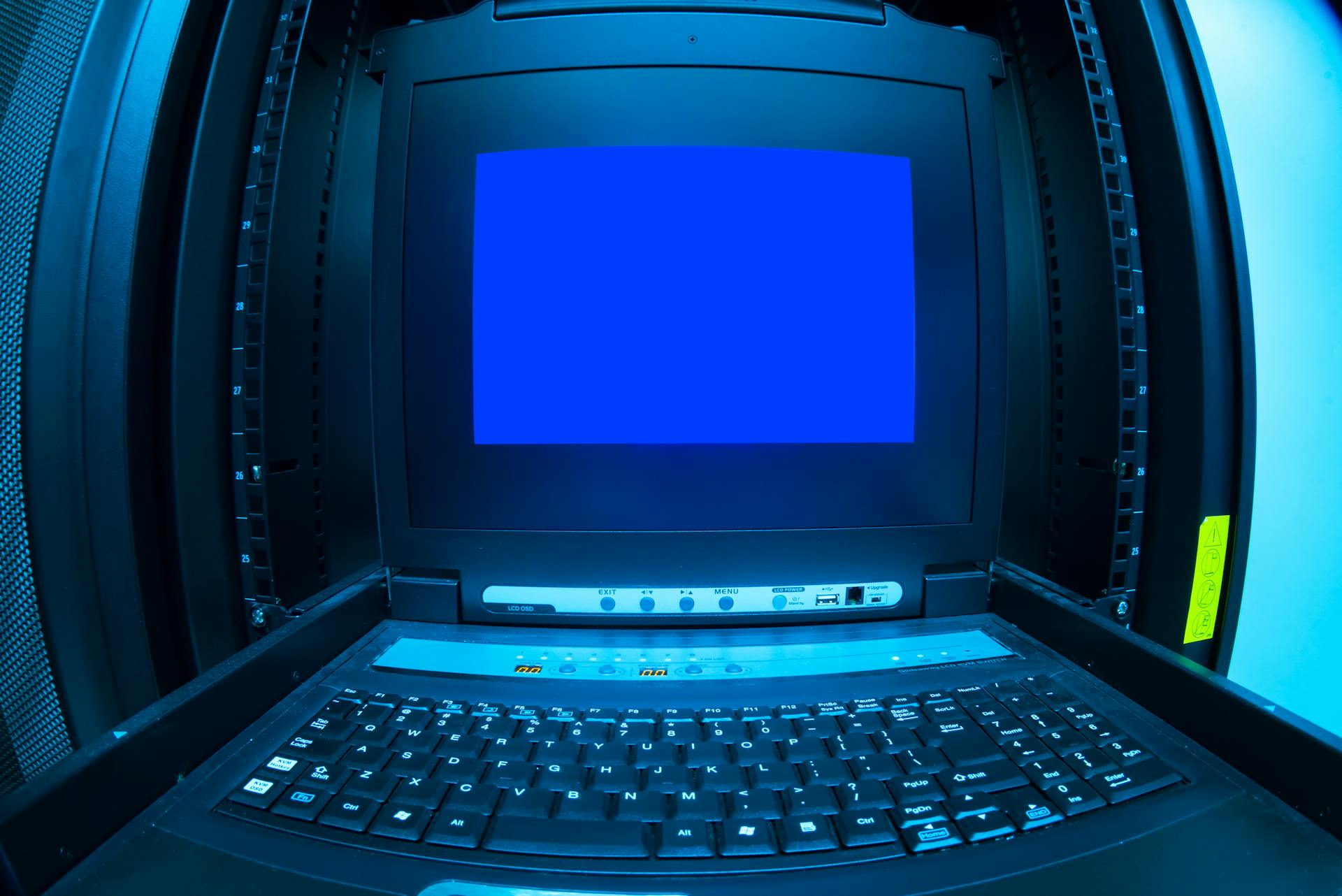
The --task-hub-name option is also optional, but it allows you to specify the name of the Durable Task Hub to use.
Here are the options you can use with the delete-task-hub action:
Logs
Managing Azure Functions requires a good understanding of how to log and monitor your functions. You can use the func logs action to do this.
The func logs action supports various options to customize its behavior. One of these options is the --platform option, which allows you to specify the hosting platform for your function app. Supported options include kubernetes.
You can also use the --name option to specify the function app name in Azure. This is a crucial step in setting up logging for your function app.
Here are the supported options for the func logs action:
Logstream
Logstream is a powerful tool for monitoring and debugging Azure Functions. You can connect your local command prompt to streaming logs for the function app in Azure using the command "func azure functionapp logstream".
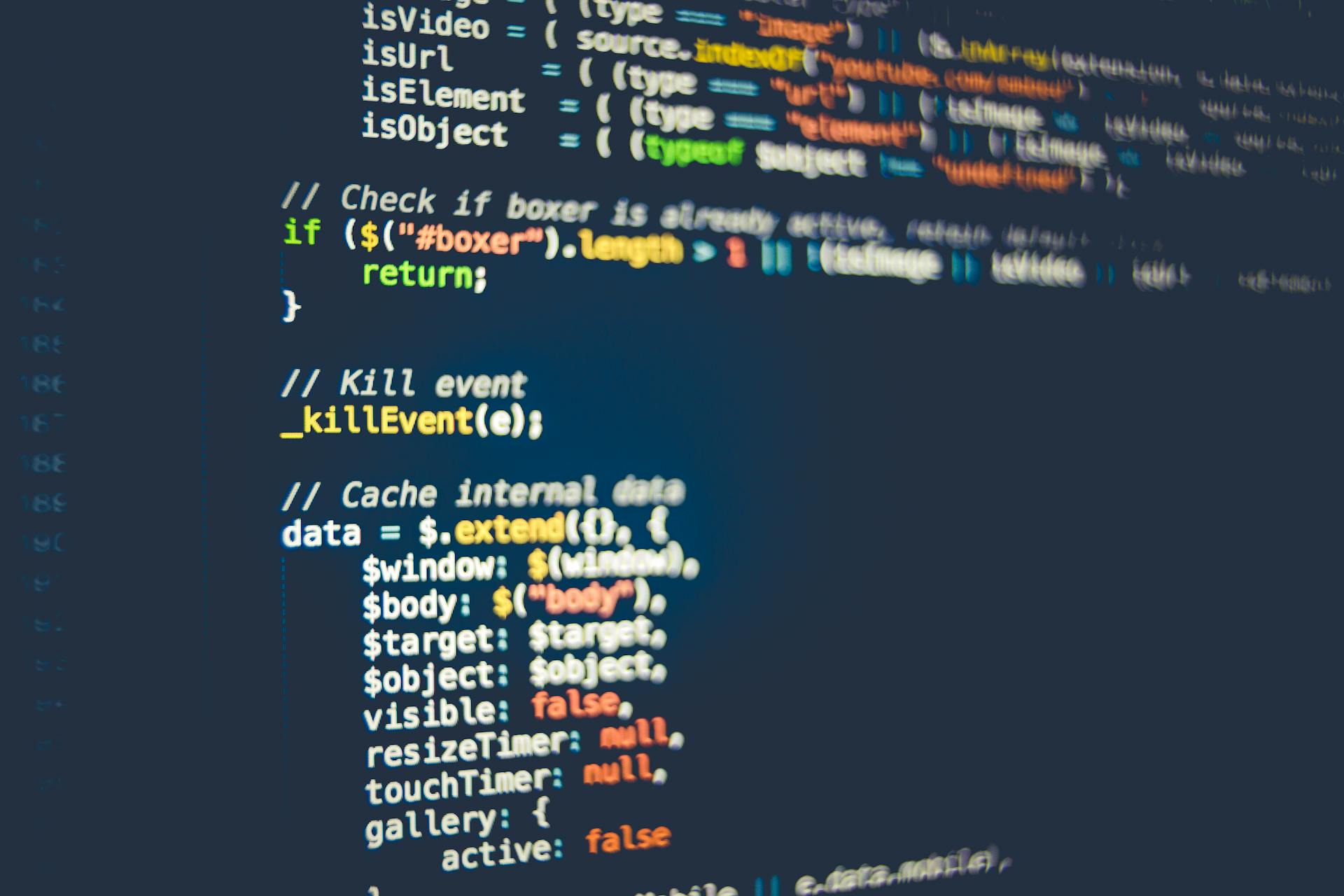
The default timeout for the connection is 2 hours, but you can change this by adding an app setting named SCM_LOGSTREAM_TIMEOUT, with a timeout value in seconds.
If you're using a Linux app in the Consumption plan, you're out of luck with the logstream feature. In this case, you'll need to use the --browser option to view logs in the portal.
You can use the --browser option to open Azure Application Insights Live Stream for the function app in the default browser.
Delete
Deleting Azure Functions and Settings is a crucial part of managing your applications. You can delete a function app using the command "az functionapp delete".
This command requires you to provide one or more resource IDs, which should be complete resource IDs containing all information of 'Resource Id' arguments. You can either provide --ids or other 'Resource Id' arguments.
To keep the app service plan empty, you can use the --keep-empty-app-service-plan option. The name of the slot is also required, and it defaults to the production slot if not specified.
Worth a look: Azure Function Service Bus Trigger
You can also specify a timeout for the deployment operation in milliseconds using the --timeout option. If you're migrating a function app from Premium to Consumption, you'll need to provide a --plan option.
When you need to remove an existing setting from the local.settings.json file, you can use the "func settings delete" command. This command supports the --connectionString option, which removes the name-value pair from the ConnectionStrings collection instead of the Values collection.
Recommended read: Azure Function Local Settings Json
Deploying and Running Azure Functions
Deploying and running Azure Functions is a straightforward process. You can deploy a provided artifact to Azure functionapp using the `az functionapp deploy` command. This command is in preview and under development, but it's a great way to deploy a war file asynchronously.
To deploy a war file, you'll need to specify the path of the artifact to be deployed, which can be a local file or a URL. You can also specify the name of the function app to deploy to and whether to restart the web app after deployment. The command also supports cleaning the target directory prior to deploying the file(s).
Here are the supported options for the `az functionapp deploy` command:
You can also run the function locally by starting the local Azure Functions runtime host from the LocalFunctionProj folder using the `func start` command. This will allow you to test and debug your function before deploying it to Azure.
List-Flexconsumption-Runtimes
When working with Azure Functions on the Flex Consumption plan, you can list the available built-in stacks for function apps using the command az functionapp list-flexconsumption-runtimes.
This command is useful for limiting the output to just the runtimes available in a specified location.
To get started, you'll need to run this command in your terminal or command prompt, and it will display the list of available runtimes.
You can use this command to plan and prepare your function app for deployment, ensuring you're using the correct runtime for your needs.
Note that the output of this command will be specific to the location you specify, so make sure to include that information when running the command.
By using this command, you can streamline your deployment process and avoid potential issues down the line.
Readers also liked: Azure Function Disappeared after Deployment
Run
To run your Azure Functions, you can start the local Azure Functions runtime host from the LocalFunctionProj folder using the command `func start`. This will make your function available at a URL like `http://localhost:7071/api/HttpExample`.
The output will show you the URL of your function, which you can copy and paste into a browser to test it. If you don't see your function listed, it's likely because you started the host from outside the project's root folder.
To stop the host, simply press Ctrl+C and choose y to confirm.
If you want to run your function with specific options, you can use the `func run` command with various flags. For example, you can use `--content` to pass inline content to the function, or `--debug` to attach a debugger to the host process.
Here are some options you can use with `func run`:
Azure Functions Extensions
Azure Functions Extensions are a powerful way to extend the functionality of your Azure Functions. You can install extensions manually using the `func extensions install` command.
The `func extensions install` command supports various options, including `--configPath`, `--csx`, `--force`, `--output`, `--package`, `--source`, and `--version`. For example, you can install version 5.0.1 of the Event Hubs extension in the local project using this command.
You can also use the `func extensions sync` command to install all extensions added to the function app. This command supports options like `--configPath`, `--csx`, and `--output`. Regenerating a missing `extensions.csproj` file is also a feature of this command.
Here's a summary of the options supported by `func extensions install`:
Extensions Install
Installing extensions for Azure Functions can be a bit tricky, but don't worry, I've got you covered. You can manually install extensions using the `func extensions install` command.
The `func extensions install` command supports several options, including `--configPath`, which specifies the path to the directory containing the `extensions.csproj` file. You can also use `--csx` to support C# scripting projects.
One important thing to note is that you should not use `func extensions install` for compiled C# projects, as you should use standard NuGet package installation methods instead, such as `dotnet add package`. If you're using Core Tools, make sure you have the .NET SDK installed.
Here's a list of the supported options for `func extensions install`:
For example, to install version 5.0.1 of the Event Hubs extension in the local project, you can use the following command: `func extensions install --package Microsoft.Azure.WebJobs.Extensions.EventHubs --version 5.0.1`.
Related reading: Azure Function Change Runtime Version
Extensions Sync
The Extensions Sync feature is a powerful tool that allows you to install all extensions added to your function app with just a few clicks.
You can use the sync action to install extensions, and it supports several options to customize the process. For example, you can specify the path of the directory containing the extensions.csproj file using the --configPath option.
The sync action also supports C# scripting (.csx) projects with the --csx option. This is useful if you're working with C# projects and want to take advantage of the sync action.
The output path for the extensions can be specified using the --output option. This allows you to control where the extensions are installed on your system.
Here's a summary of the options supported by the sync action:
Regenerating a missing extensions.csproj file is also supported by the sync action. However, if an extension bundle is defined in your host.json file, no action will be taken.
Durable Functions
Durable Functions is a powerful feature in Azure Functions that allows you to orchestrate tasks and workflows. You can create and manage orchestration instances using the Azure Functions CLI.
To get started with Durable Functions, you can use the `func durable` command, which provides several subcommands for managing orchestration instances. For example, you can use the `get-instances` subcommand to retrieve a list of orchestration instances, including their status and creation time. You can also use the `start-new` subcommand to start a new instance of an orchestrator function.
The `get-instances` subcommand supports several options, including `--continuation-token`, `--connection-string-setting`, `--created-after`, `--created-before`, `--runtime-status`, `--top`, and `--task-hub-name`. These options allow you to filter and customize the list of instances returned.
Here's a summary of the `get-instances` subcommand options:
Durable Get-Instances
Durable Get-Instances is a powerful feature in Durable Functions that allows you to retrieve instances based on various criteria.
You can limit the number of records returned in a given request using the --top option.
The --created-after option lets you get instances created after a specific date/time (UTC), which must be in ISO 8601 format.
If you need to get instances created before a specific date/time (UTC), use the --created-before option.
To get instances whose status match a specific status, such as running, completed, or failed, use the --runtime-status option with one or more space-separated statuses.
You can use the --task-hub-name option to specify the name of the Durable Functions task hub to use.
Here are the available options in a table format:
Durable Purge-History
Durable Purge-History is a powerful tool that helps manage the storage of orchestration instance state, history, and blob storage for Durable Functions. It's essential to use this feature to maintain a clean and organized environment.
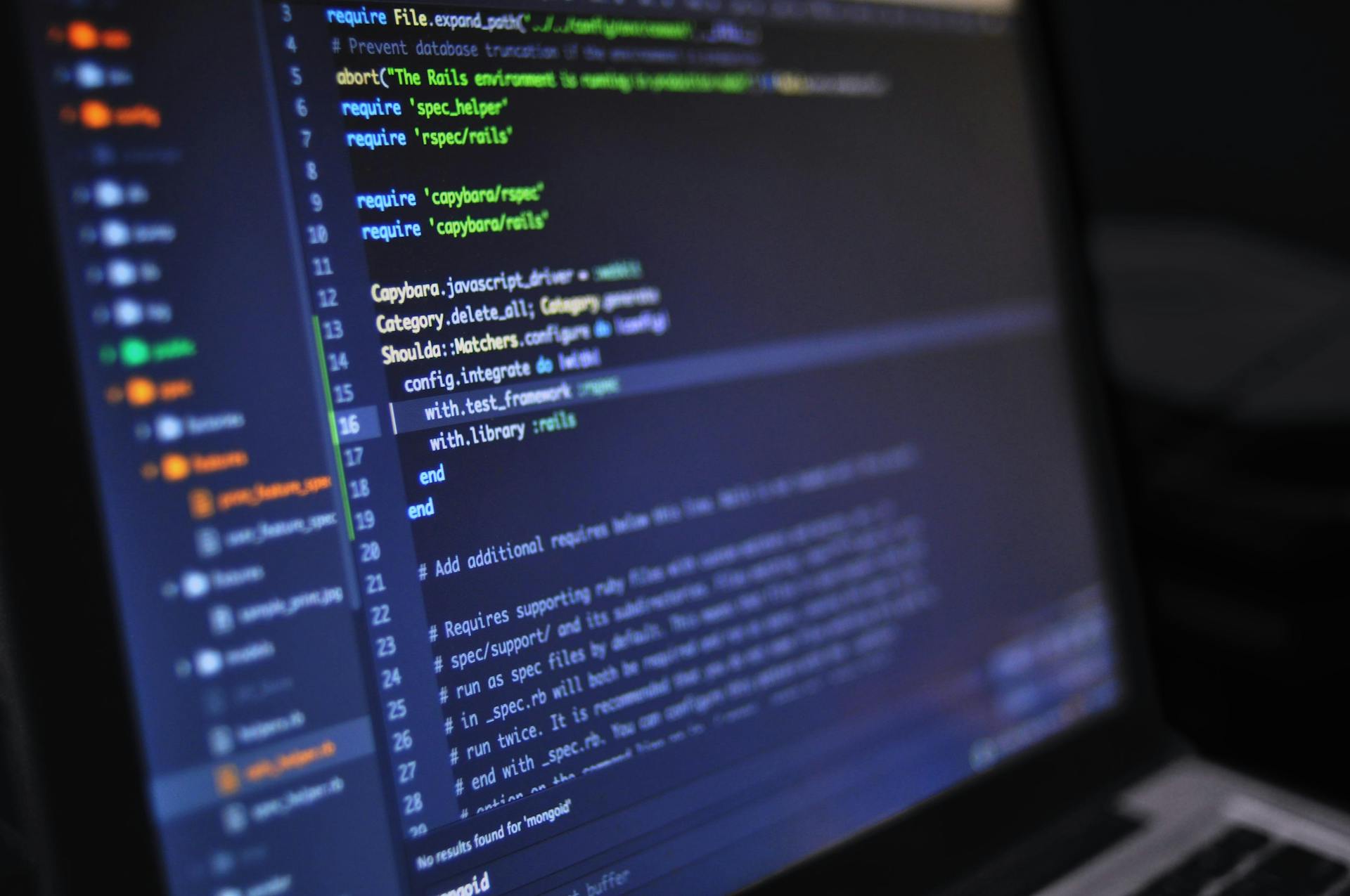
You can use the purge-history action to delete orchestration instance state, history, and blob storage for orchestrations older than a specified threshold. This is a great way to free up storage space and keep your Durable Functions environment running smoothly.
The purge-history action supports several options that allow you to customize the deletion process. One of these options is --connection-string-setting, which specifies the name of the setting containing the storage connection string to use.
Another option is --created-after, which allows you to delete the history of instances created after a specific date and time. You can use any ISO 8601 formatted datetime value for this option.
You can also use --created-before to delete the history of instances created before a specific date and time. This option also accepts ISO 8601 formatted datetime values.
The --runtime-status option enables you to delete the history of instances whose status match a specific status. You can provide one or more space-separated statuses, including completed, terminated, canceled, and failed.
If you don't include --runtime-status, instance history is deleted regardless of status. This means you can choose to delete all instance history or just the history of instances that have a specific status.
Here are the supported statuses for --runtime-status:
You can also specify the name of the Durable Functions task hub to use with the --task-hub-name option. This allows you to manage the storage of orchestration instance state, history, and blob storage for specific task hubs.
Durable Rewind
Durable Rewind is a crucial feature in Durable Functions that allows you to rewind a specified orchestration instance.
To rewind an orchestration instance, you'll need to specify its ID, as well as the reason for rewinding it. This is a required step, and you can't proceed without providing both pieces of information.
The rewind action supports several options, including the ability to specify a storage connection string using a setting name. If you don't provide a storage connection string, Durable Functions will use the default one.
You can also specify the name of the Durable Functions task hub to use for the rewind operation. However, this is optional, and you can leave it out if you're not sure what to use.
Here are the options you can use with the rewind action:
Local Development and Testing
Local development and testing is where the magic happens. You can create a local function project with a single function using the `func init` command, specifying the runtime and target framework. For example, `func init LocalFunctionProj --worker-runtime dotnet-isolated --target-framework net8.0`.
To get started, you'll need to navigate into the project folder, which contains various files including configurations files like `local.settings.json` and `host.json`. These files are excluded from source control by default in the `.gitignore` file.
A function project is a container for one or more individual functions, and you can add a function to your project using the `func new` command. For instance, `func new --name HttpExample --template "HTTP trigger" --authlevel "anonymous"` creates an `HttpExample.cs` code file.
You can run your function locally by starting the Azure Functions runtime host from the project folder with the `func start` command. This will output the URL of your function, which you can copy and paste into a browser to test it.
Curious to learn more? Check out: Azure Auth Json Website Azure Ad Authentication
Create Local Project
To create a local project, start by running the func init command. This will create a functions project in a folder named LocalFunctionProj with the specified runtime.
The command to run is func init LocalFunctionProj --worker-runtime dotnet-isolated --target-framework net8.0. This will set up the necessary configurations for your project.
Navigate into the project folder by running cd LocalFunctionProj. This will take you to the folder containing various files for the project, including configurations files named local.settings.json and host.json.
local.settings.json is an important file that contains secrets downloaded from Azure, which is why it's excluded from source control by default in the .gitignore file.
To add a function to your project, use the func new command. This command will create a new function with the specified trigger and name.
Here are the steps to add a function to your project:
- Run the func new command with the --name argument and the --template argument, like this: func new --name HttpExample --template "HTTP trigger" --authlevel "anonymous"
- This will create an HttpExample.cs code file in your project folder.
Run Locally
To run your Azure Functions locally, you'll need to start the local Azure Functions runtime host. This can be done by running the command `func start` from the project folder.
You should see output that includes the following lines: "Now listening on: http://0.0.0.0:7071" and "Application started. Press Ctrl+C to shut down." This indicates that the host is up and running.
The host will also display the URL of your function, which will be in the format "http://localhost:7071/api/HttpExample". You can copy this URL and paste it into a browser to test your function.
Discover more: Http Triggered Azure Function
If you don't see your function listed, it's likely because you started the host from outside the project folder. In this case, use Ctrl+C to stop the host, navigate to the project's root folder, and run the `func start` command again.
Here are the steps to run your function locally in a concise format:
- Run `func start` from the project folder.
- Copy the URL of your function from the output.
- Paste the URL into a browser to test your function.
- Use Ctrl+C to stop the host when you're done.
By following these steps, you'll be able to test your Azure Functions locally and ensure they're working as expected before deploying them to the cloud.
Durable Start-New
Durable Start-New is a powerful tool for local development and testing. It allows you to start a new instance of an orchestrator function.
To use Durable Start-New, you'll need to specify the name of the orchestrator function to start. This is required and must be provided using the --function-name option. For example, if your function is named "myFunction", you would use the command "func durable start-new --function-name myFunction".
You can also specify the ID of an orchestration instance using the --id option. This is also required, so make sure to include it in your command. The ID is used to identify the specific instance of the function that you want to start.
You might enjoy: Durable Functions Azure
If you need to provide input to the orchestrator function, you can do so using the --input option. This input can be either inline or from a JSON file. If you're using a file, make sure to prefix the path to the file with an ampersand (@). For example, if your file is located at "path/to/file.json", you would use the command "func durable start-new --function-name myFunction --id myInstanceId --input @path/to/file.json".
Here's a summary of the Durable Start-New options:
Frequently Asked Questions
What is Azure command line?
The Azure command line is a set of commands that help you create and manage Azure resources. It's a powerful tool for automating Azure tasks and streamlining your cloud management experience.
Sources
- https://learn.microsoft.com/en-us/cli/azure/functionapp
- https://learn.microsoft.com/en-us/azure/azure-functions/create-first-function-cli-csharp
- https://www.npmjs.com/package/azure-functions-cli
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-core-tools-reference
- https://azure.github.io/AppService/2016/12/01/Running-Azure-Functions-Locally-with-the-CLI-and-VS-Code.html
Featured Images: pexels.com