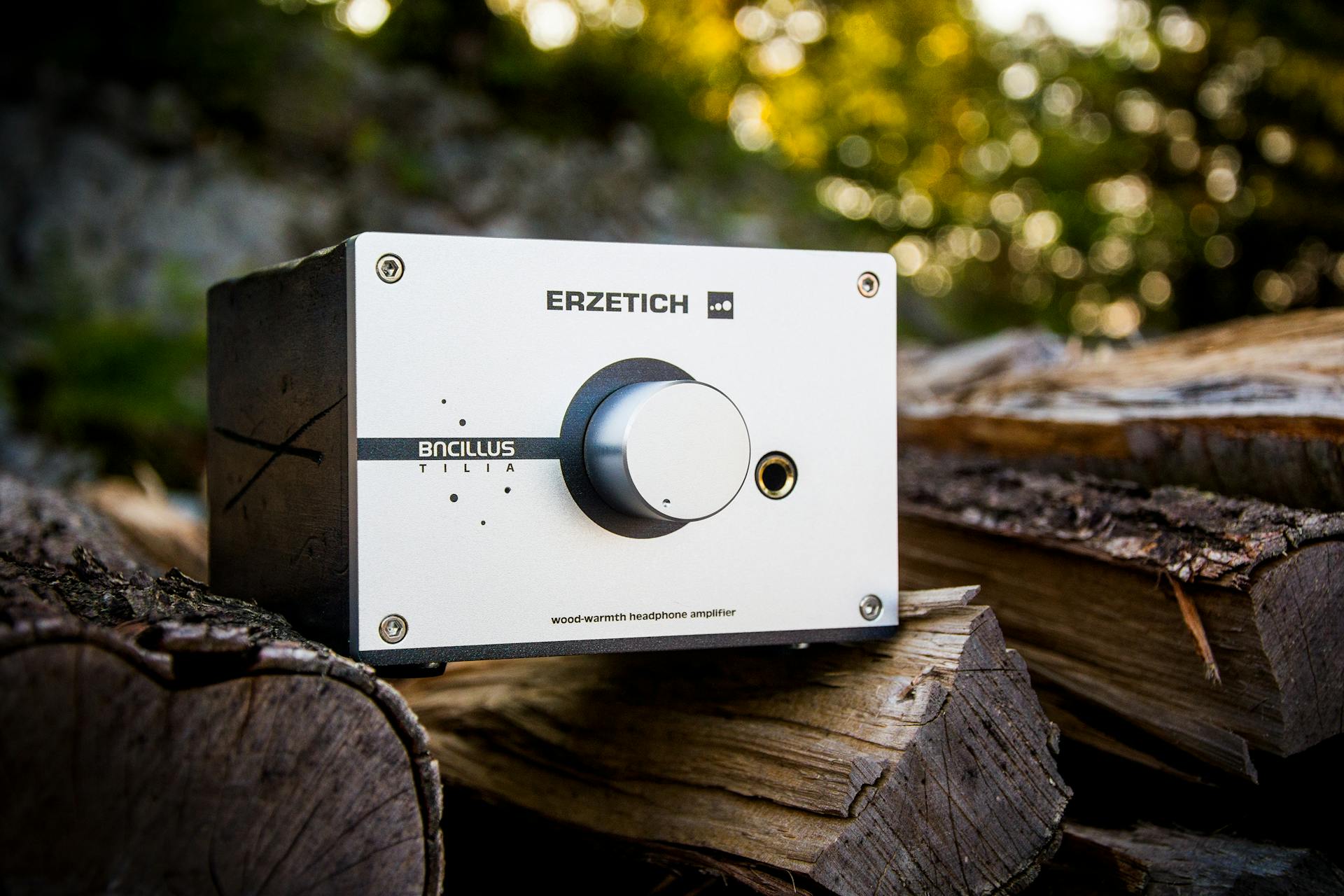
AWS Amplify Gen2 makes it easy to upload files to an S3 bucket, but you need to configure it correctly. To start, you'll need to create an S3 bucket and grant it the necessary permissions.
You can create an S3 bucket using the AWS Management Console, AWS CLI, or AWS SDKs. Make sure to choose a unique name for your bucket to avoid conflicts with other buckets.
To grant permissions to your S3 bucket, you'll need to create an IAM policy. This policy will define the actions that your Amplify app can perform on the bucket, such as uploading and deleting files.
In the IAM policy, you can specify the S3 bucket's ARN (Amazon Resource Name) to ensure that the policy only applies to that bucket. This helps prevent accidental access to other buckets.
If this caught your attention, see: Next Js Aws Amplify Gen2 Architecture Diagram
AWS Amplify Setup
To set up AWS Amplify, you'll need to install the Amplify CLI globally. Run the command `npm install -g @aws-amplify/cli` in your terminal to get started.
You might like: Aws Amplify vs S3
The Amplify CLI is the command-line interface that allows you to interact with AWS services from your terminal. It simplifies the process of integrating with various AWS services, including storage, authentication, and APIs.
To configure Amplify, you'll need to sign in to your AWS account and create a new IAM user with the necessary permissions. Follow the prompts to set up your AWS credentials and configure Amplify.
Here are the steps to configure Amplify:
- Run `amplify configure` in your terminal
- Follow the prompts to sign in to your AWS account
- Create a new IAM user with the necessary permissions
Once you've configured Amplify, you can create a new project using the Amplify CLI. To do this, run the command `amplify init` in your terminal and follow the prompts to set up your project.
Here's a summary of the steps to set up AWS Amplify:
Now that you've set up AWS Amplify, you can start building your full-stack application. In the next section, we'll explore how to add file upload functionality to your application using AWS S3.
File Upload Component
To create a file upload component, you'll need to install the react-dropzone library. This library simplifies file selection, making it easier to handle file uploads.
Create a new component called FileUpload.js to handle file uploads. This component will be responsible for displaying the file upload interface and handling the file upload process.
Here's a step-by-step guide to creating the FileUpload component:
1. Install the react-dropzone library by running the command `npm install react-dropzone` or `yarn add react-dropzone`.
2. Create a new file called FileUpload.js and add the necessary code to create the file upload component.
3. Use the react-dropzone library to simplify file selection and handling.
By following these steps, you'll be able to create a file upload component that integrates seamlessly with your AWS Amplify application.
Intriguing read: Aws Upload File to S3 Api Gateway
S3 Bucket Configuration
To configure your S3 bucket, start by choosing a globally unique name that's DNS-compliant. This will ensure your bucket is easily accessible and identifiable.
The name you choose must be unique, and AWS will notify you if it's already in use. Select the region that's geographically closest to your users for improved latency.
You might like: S3 Bucket Name
For general-purpose storage, the default storage class is usually sufficient. However, you can choose a different storage class based on your specific requirements.
To define who has access to your bucket, you can keep the default settings for now. This will allow you to simplify the setup process and focus on other aspects of your project.
A fresh viewpoint: Aws S3 Storage Cost
Create Bucket
Creating a new bucket is a straightforward process. You'll need to choose a globally unique name for it, which must be DNS-compliant.
The name you choose will be used to access your bucket, so pick something memorable. If the name you want is already in use, AWS will notify you.
Select the region that's geographically closest to your users for improved latency. This will help ensure that your data is stored and retrieved quickly.
The default storage class is usually suitable for general-purpose storage. If you need more specific storage options, you can choose from the available classes.
To keep things simple, you can stick with the default permissions for now. This will allow you to focus on other aspects of your S3 bucket configuration.
For more insights, see: Aws S3 Storage Types
Phase 1: S3 + CloudFront
Setting up your S3 bucket is a crucial step in configuring your storage solution. Amazon S3 is a great tool for storing videos and other files, and it integrates well with CloudFront for efficient and secure delivery.
To enable cross-origin resource sharing, CORS needs to be configured on your S3 bucket. This allows your React app to upload files to the bucket, even when hosted on AWS Amplify. Browsers prevent cross-domain interactions by default, but CORS allows legitimate cross-origin requests.
Securing your S3 bucket against unauthorized access requires more than just CORS configuration. You'll need to adjust the CloudFront configuration and attach a specific bucket policy that grants the necessary access. This will ensure that your bucket is secure and only accessible to authorized users.
Amazon CloudFront serves as the distribution network for delivering videos to users efficiently and with minimal latency. It has a global network of edge locations that cache your content, reducing the time it takes for users to access your files.
You might like: Aws S3 Sync Specific Files
To access your bucket from CloudFront, you'll need to enable Origin access control in the CloudFront settings. This setting governs your content's access, delivery, and security, and allows for detailed specification of caching policies.
Limiting the Allowed HTTP Protocols to GET and HEAD only will ensure that your distribution is only intended for video retrieval. Additionally, enabling the "Redirect HTTP to HTTPS" option will automatically upgrade HTTP requests to HTTPS, enhancing security and user experience.
After creating the distribution, you'll need to update the bucket policy of your source S3 bucket to allow the distribution to access the videos. This will involve adding a specific policy that grants the necessary access to CloudFront.
Here's an interesting read: Aws Cloudfront with S3
Presigned URL Logic
To implement presigned URL logic, you'll need to create a service to obtain a presigned URL from your backend.
Update the api.js file to create this service. This is done by implementing the necessary logic to obtain a presigned URL from your backend.
Related reading: Aws S3 Presigned Url
To integrate the FileUpload component with the presigned URL logic, you'll need to update the main component (App.js). This involves integrating the FileUpload component with the presigned URL logic obtained from your backend.
Create a service to obtain a presigned URL from your backend, which will be used to update the main component (App.js) to integrate the FileUpload component with the presigned URL logic.
For more insights, see: Aws S3 Upload File App Typescript
Frequently Asked Questions
How do I upload to a bucket folder on S3?
To upload to an S3 bucket folder, sign in to the AWS Management Console and navigate to the S3 console, then select the bucket and choose the "Upload" option.
Sources
- https://medium.com/@vanishree1698/aws-amplify-file-upload-9446c709d92d
- https://medium.com/@vjraghavanv/building-a-react-app-for-uploading-files-to-amazon-s3-with-amazon-amplify-and-cognito-2ee52f33aed1
- https://blog.devgenius.io/full-stack-tutorial-secure-file-upload-with-presigned-urls-in-react-and-node-js-aws-s3-2eb2643b168c
- https://aws.plainenglish.io/integrating-aws-api-gateway-and-aws-s3-using-aws-cdk-41118c1b01c3
- https://tutorialsdojo.com/building-a-simple-video-hosting-service-using-amazon-cloudfront-amazon-s3-and-aws-amplify/
Featured Images: pexels.com